JavaScript Set Collection Tutorial with Examples
1. Collections - Set
ECMAScript 6 introduces the 2 new data structures such as Map & Set. They are part of ECMAScript Collections Framework).
- Maps - This data structure allows you to store the "Key/Value". And you can access the value through the key or update a new value corresponding to a key.
- Sets - This data structure stores a list of elements not allowing duplicate, and not indexing elements.
In this post, I will introduce the Set to you.
Set
The Set is a data structure introduced in the ECMAScript6. The following is Set characteristics.
- Set is a list of elements not allowing iteration.
- Set doesn't index elements.
- Set is an ordered list, which means that an element which is added first will come first and an element that is added second will come second.
Constructors:
Create Set object through the constructor of the Set: class
new Set( [iterable] )
Parameters:
- iterable - If this object is passed to the constructor of the Set, all its elements will be added to the Set. On the contrary, if you don't specify this parameter, or its value is null, the Set object created will be empty.
Properties:
set-example.js
// Create a Set from an Array
var fruits = new Set( ["Apple","Banana","Papaya"] );
console.log(fruits); // Set { 'Apple', 'Banana', 'Papaya' }
Property | Description |
size | This Property returns element number of Set object |
set-size-example.js
// Create a Set from an Array
var fruits = new Set( ['Apple','Banana','Papaya'] );
var size = fruits.size;
console.log(size); // 3
for..of
You can use the for...of loop to loop through elements of Set.
set-for-of-loop-example.js
// Create a Set from an Array
var fruits = new Set( ["Apple","Banana","Papaya"] );
for(let fruit of fruits) {
console.log(fruit);
}
Output:
Apple
Banana
Papaya
2. The methods of Set
add(value)
Add an element to the end of Set if this element doesn't exists in Set and return this Set object.
set-add-example.js
// Create a Set from an Array
var fruits = new Set( ["Apple","Banana","Papaya"] );
// Append an Element to the Set and returns this Set.
var thisFruits = fruits.add("Cherry");
console.log(fruits); // Set { 'Apple', 'Banana', 'Papaya', 'Cherry' }
// 'Set' does not allow duplicates
fruits.add("Banana");
console.log(fruits); // Set { 'Apple', 'Banana', 'Papaya', 'Cherry' }
Output:
Set { 'Apple', 'Banana', 'Papaya', 'Cherry' }
Set { 'Apple', 'Banana', 'Papaya', 'Cherry' }
delete(value)
This method is used to remove an element out of the Set. The method returns true if it finds an element to be removed and on the contrary, it returns false.
set-delete-example.js
// Create a Set from an Array
var fruits = new Set( ["Apple","Banana","Papaya"] );
console.log(fruits); // Set { 'Apple', 'Banana', 'Papaya' }
// Delete an element:
var deleted = fruits.delete("Banana");
console.log("Deleted? " + deleted); // true
console.log(fruits); // Set { 'Apple', 'Papaya' }
clear()
Remove all elements out of Set.
set-clear-example.js
// Create a Set from an Array
var fruits = new Set( ["Apple","Banana","Papaya"] );
console.log(fruits); // Set { 'Apple', 'Banana', 'Papaya' }
// Remove all elements.
fruits.clear();
console.log(fruits); // Set { }
console.log("Size after clear: " + fruits.size);
entries()
This method returns a new Iterator object of which each entry contains an array with the 2 elements such as [value, value]. The order of entries still be kept unchanged like the order of elements in the Set object. (See the following illustration)
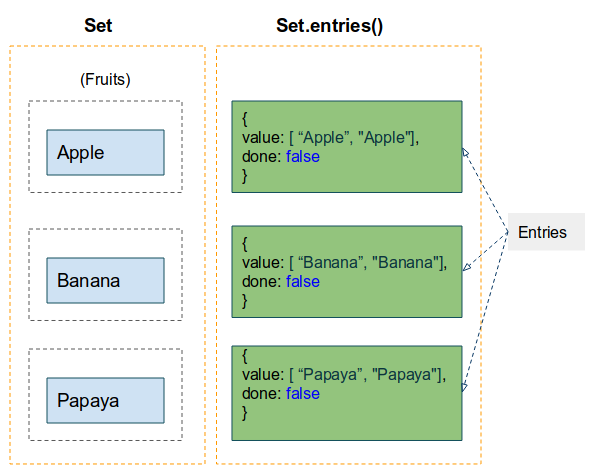
set-entries-example.js
// Create a Set from an Array
var fruits = new Set( ["Apple","Banana","Papaya"] );
console.log(fruits); // Set { 'Apple', 'Banana', 'Papaya' }
// Iterator Object:
var iteratorEntries = fruits.entries();
var entry;
while( !(entry = iteratorEntries.next() ).done ) {
console.log(entry.value); // [ 'Apple', 'Apple' ]
}
has(value)
This method tests whether a value given by a parameter exists in Set or not. Return true if existing, on the contrary, return false.
set-has-example.js
// Create a Set from an Array
var fruits = new Set( ["Apple","Banana","Papaya"] );
var has = fruits.has("Banana");
console.log(has); // true
forEach(callbackFn[, thisArg])
This method will call the callbackFn function one time for each element of Set.
mySet.forEach(callbackFn [, thisArg])
Parameters:
- callbackFn - This function will be called one time corresponding to each element of the Set object.
- thisArg - Parameter that is used as this when executing the callbackFn function.
set-forEach-example.js
// Create a Set from an Array
var fruits = new Set( ["Apple","Banana","Papaya"] );
console.log(fruits); // Set { 'Apple', 'Banana', 'Papaya' }
function showFruit(fruit, thisSet) {
console.log("Fruit: " + fruit);
}
// Or Call: fruits.forEach(showFruit);
fruits.forEach(showFruit, fruits);
key() & values()
The key() & values() method returns a new Iterator of which each element has the shape {value: aValue, done: false}.
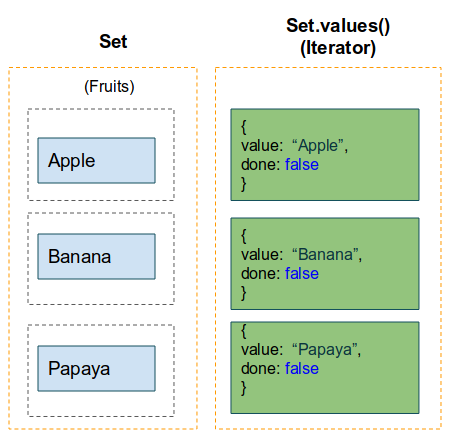
set-values-example.js
// Create a Set from an Array
var fruits = new Set( ["Apple","Banana","Papaya"] );
var fruitIterator = fruits.values();
var entry;
while ( !(entry = fruitIterator.next()).done) {
console.log(entry); // { value: 'Apple', done: false }
console.log(entry.value); // Apple
}
Output:
{ value: 'Apple', done: false }
Apple
{ value: 'Banana', done: false }
Banana
{ value: 'Papaya', done: false }
Papaya
mySet[Symbol.iterator]
Symbol.iterator is a special property of Set. By default, the value of mySet[Symbol.iterator] is the values() method of mySet.
set-symbol-iterator-example.js
// Create a Set from an Array
var fruits = new Set( ["Apple","Banana","Papaya"] );
// Same as: fruits.values()
var fruitIterator = fruits[Symbol.iterator]();
var entry;
while ( !(entry = fruitIterator.next()).done) {
console.log(entry); // { value: 'Apple', done: false }
console.log(entry.value); // Apple
}
3. WeakSet
Basically, the WeakSet is quite the same as Set, but it has the following differences:
- The WeakSet elements have to be objects, they can not be a primitive type.
- The WeakSet elements can be removed in the process of Garbage collection, which is an independent process to remove the objects no longer used in the program.
- WeakSet doesn't support property: size, therefore, you can not know how many elements it has.
- Many methods may be available in Set class but have no WeakSet, for example, values(), keys(), clear(),..
NOte: You can not use the for..of loop for WeakSet, and there is no way for you to iterate over the elements of WeakSet.
Create a WeakSet object.
new Set( [iterable] )
Parameters:
- iterable - If this object is passed to the constructor of the WeakSet, all its elements will be added to the Set. On the contrary, if you don't specify this parameter, or its value is null, the Set object created will be empty.
Methods:
The quantity of WeakSet methods is less than the quantity of Set methods:
- WeakSet.add(element)
- WeakSet.delete(element)
- WeakSet.has(element)
The elements in the WeakSet have to be objects, they can not be primitive types.
let w = new WeakSet();
w.add('a'); // TypeError: Invalid value used in weak set
let s = new Set();
s.add('a'); // Works
weakset-example.js
var element1 = {}; // An Object
var element2 = {foo: "bar"};
var element3 = {bar: "foo"};
var myWeakSet = new WeakSet( [element1, element2] );
myWeakSet.add(element3);
console.log(myWeakSet.has(element2)); // true
ECMAScript, Javascript Tutorials
- Introduction to Javascript and ECMAScript
- Quickstart with Javascript
- Alert, Confirm, Prompt Dialog Box in Javascript
- Quickstart with JavaScript
- JavaScript Variables Tutorial with Examples
- Bitwise Operations
- JavaScript Arrays Tutorial with Examples
- JavaScript Loops Tutorial with Examples
- JavaScript Functions Tutorial with Examples
- JavaScript Number Tutorial with Examples
- JavaScript Boolean Tutorial with Examples
- JavaScript Strings Tutorial with Examples
- JavaScript if else Statement Tutorial with Examples
- JavaScript Switch Statement
- JavaScript Error Handling Tutorial with Examples
- JavaScript Date Tutorial with Examples
- JavaScript Modules Tutorial with Examples
- The History of Modules in JavaScript
- JavaScript setTimeout and setInterval Function
- Javascript Form Validation Tutorial with Examples
- JavaScript Web Cookies Tutorial with Examples
- JavaScript void Keyword Tutorial with Examples
- Classes and Objects in JavaScript
- Class and inheritance simulation techniques in JavaScript
- Inheritance and polymorphism in JavaScript
- Undertanding Duck Typing in JavaScript
- JavaScript Symbols Tutorial with Examples
- JavaScript Set Collection Tutorial with Examples
- JavaScript Map Collection Tutorial with Examples
- Undertanding JavaScript Iterables and Iterators
- JavaScript Regular Expressions Tutorial with Examples
- JavaScript Promise, Async/Await Tutorial with Examples
- Javascript Window Tutorial with Examples
- Javascript Console Tutorial with Examples
- Javascript Screen Tutorial with Examples
- Javascript Navigator Tutorial with Examples
- Javascript Geolocation API Tutorial with Examples
- Javascript Location Tutorial with Examples
- Javascript History API Tutorial with Examples
- Javascript Statusbar Tutorial with Examples
- Javascript Locationbar Tutorial with Examples
- Javascript Scrollbars Tutorial with Examples
- Javascript Menubar Tutorial with Examples
- JavaScript JSON Tutorial with Examples
- JavaScript Event Handling Tutorial with Examples
- Javascript MouseEvent Tutorial with Examples
- Javascript WheelEvent Tutorial with Examples
- Javascript KeyboardEvent Tutorial with Examples
- Javascript FocusEvent Tutorial with Examples
- Javascript InputEvent Tutorial with Examples
- Javascript ChangeEvent Tutorial with Examples
- Javascript DragEvent Tutorial with Examples
- Javascript HashChangeEvent Tutorial with Examples
- Javascript URL Encoding Tutorial with Examples
- Javascript FileReader Tutorial with Examples
- Javascript XMLHttpRequest Tutorial with Examples
- Javascript Fetch API Tutorial with Examples
- Parsing XML in Javascript with DOMParser
- Introduction to Javascript HTML5 Canvas API
- Highlighting code with SyntaxHighlighter Javascript library
- What are polyfills in programming science?
Show More