JavaScript Web Cookies Tutorial with Examples
1. What is Web Cookie?
As you know, Web browsers and Server communicate each other through HTTP protocol. When you use access to a website from the browser, your request will be sent to the server and the server returns the content to the browser for displaying.
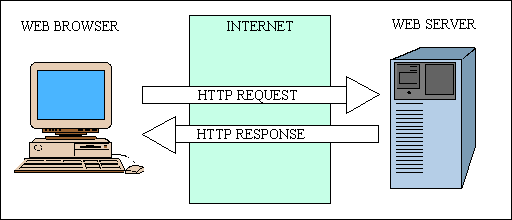
Cookie is a technique that helps a website store some information on your computer. It will save the information and traces you have ever accessed to that website.
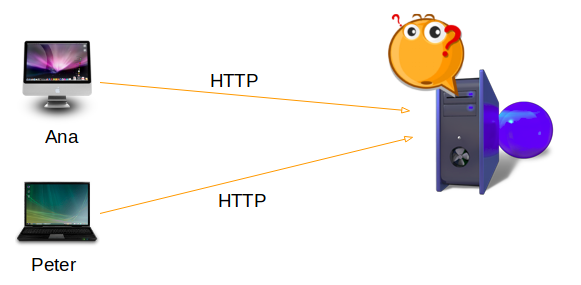
For simplicity, see the following situation:
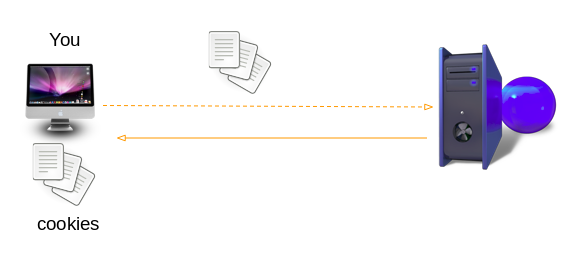
You access to a website that you have not ever visited, such as foo.com. Suppose this website supports 3 languages: English, French and Vietnamese. Because you have never visited this website before, the default English interface will be displayed. You reset other language such as French to display. The server responds to your request, and it also sends the browser a Cookie containing your preferred language - French.
You turn off the computer and visit this website the next day. When the request is sent to the server, the Cookies saved on your computer will be sent with the request, which will help the server determine your preferred language and return the content in such language.
What is Web Cookie?
- Cookie means a small piece of text, generated by the Web Server sent to your browser. The Cookie can be stored on memory or as files on a user's computer. Cookies may also be generated by Javascript running on your browser.
- Cookies stored on the client side will be included with the request every time when a user accesses any page of that website.
The main information of Cookie is a pair of Name/Value; for example:
SessionID = AAA22311
languagePreference = FR
headerColor = BLUE
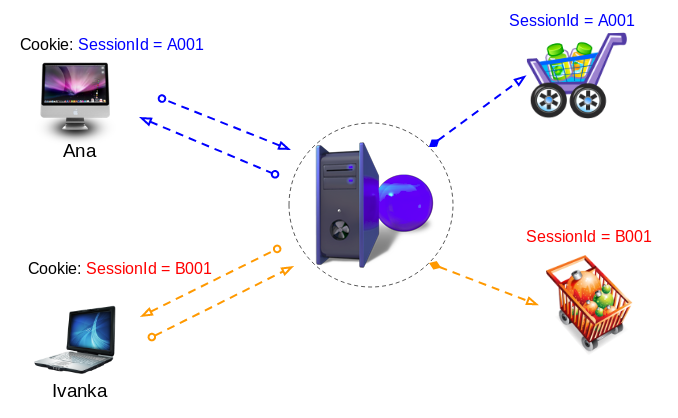
Cookie techniqueisoften used in e-commerce websites. Users buy goods on this website. The commodities bought by users are stored on a Cart. In the first access, the buyer (Browser) will receive a unique SessionId (not coinciding with other users). Each SesionId is attached to a cart managed on Web Server. The SessionId/Value information piece is a Cookie stored on the user's computer. Each time a user creates a new request, this Cookie is always attached to the request, which helps the Web Server distinguish different users.
What does a Cookie include?
A Cookie means a simple data record transmitted from the browser to the Web Server and vice versa, it has the structure as follows:
SSID=Ap4P…GTEq; Domain=foo.com; Path=/; Expires=Wed, 13 Jan 2021 22:23:01 GMT; Secure;
HSID=AYQEVn…DKrdst; Domain=.foo.com; Path=/; Expires=Wed, 13 Jan 2021 22:23:01 GMT; HttpOnly
HSID=AYQEVn…DKrdst; Domain=foo.com; Path=/; Max-Age=300; HttpOnly
Attribute | Meaning |
Name=Value | Name of Cookie and its value |
Domain | Domain Name of website. |
Expires | Specify date and time when this Cookie expires. If Cookie cannot set Expires & Max-Age, it will expire immediately after the user exits the browser. |
Max-Age | The time is calculated in seconds when this Cookie will exist since it is created. If Cookie has both Expires & Max-Age information then Max-Age will be given priority. |
Path | This is the path to a directory or a page that set this Cookie. It can be blank if you want to access this Cookie at every directory or every page. |
Secure | If this attribute is available, i.e. only secured servers can access this Cookie |
HttpOnly | If this attribute is available, i.e. this Cookie can be only manipulated at the Server side, but it is impossible to manipulate with this Cookie by Javascript on the browser. |
- Domain=foo.com: domain name of foo.com manipulated with this Cookie.
- Domain=.foo.com: Domain name of foo.com and all its subdomains (for example: abc.foo.com) which can manipulate with this Cookie.
2. Where is Cookie stored?
Cookies can be stored on memory or on files. In this section, I mention only Cookies stored in files on the user's computer.
IE (Internet Explorer)
With the IE browser, Cookies of the samewebsite are stored in the same file. and you can find them in the directory like below:
- C:\Users\{CurrentUser}\AppData\Roaming\Microsoft\Windows\Cookies
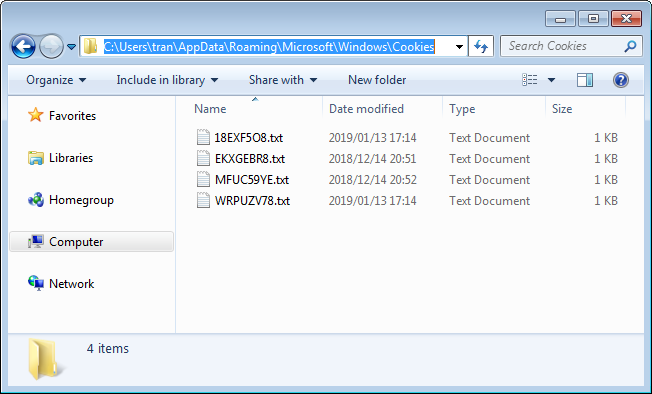
The contents of this file are quite confusing, but you don't need to care about it:
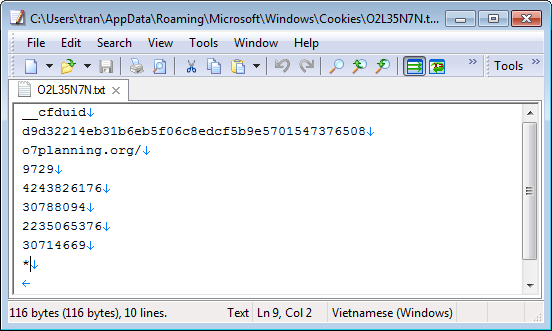
Chrome
Chrome uses the SQLite database to store Cookie records, specifically, they are stored in the file named Cookies, which you can see in the directory as follows:
- Windows: C:\Users\{CurrentUser}\AppData\Local\Google\Chrome\User Data\Default
- Mac OS: ~/Library/Application Support/Google/Chrome/Default
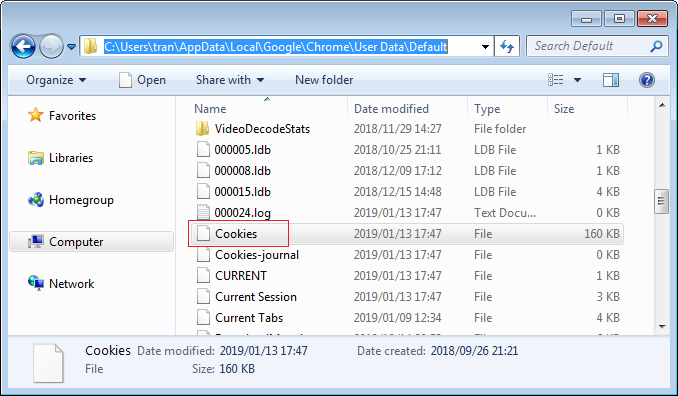
3. See Cookie on browser
Seeing the Cookie on the browser is very simple, for example, with the Chrome browser:
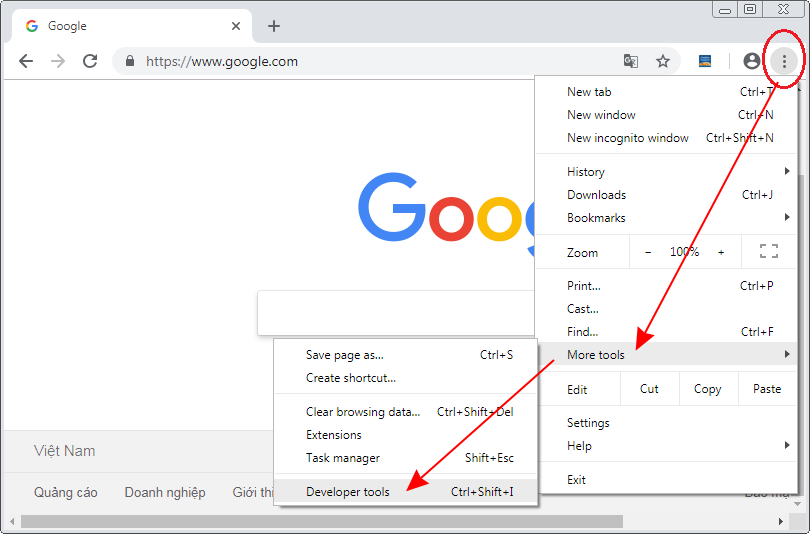
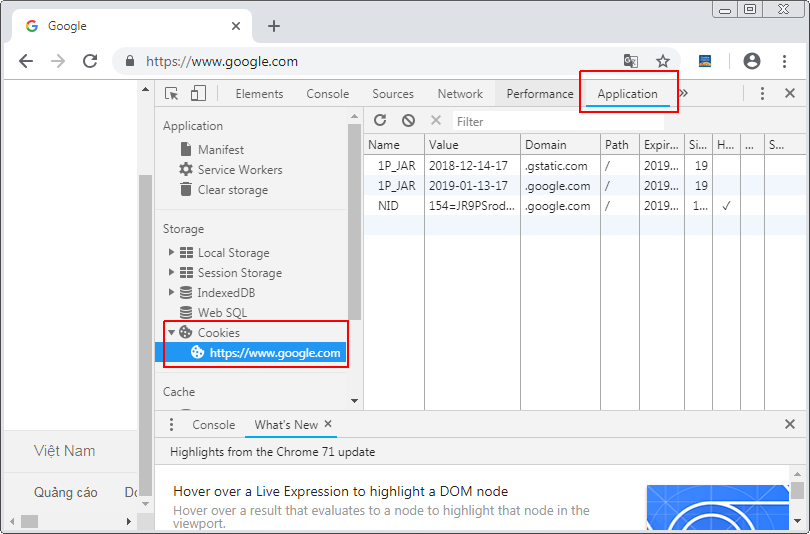
4. ECMAScript Cookie
Basically, there are 2 ways for you to work with Cookies:
- Manipulate Cookies at the Server.
- Manipulate Cookies by Javascript running on user's browser
SSID=Ap4P…GTEq; Domain=foo.com; Path=/; Expires=Wed, 13 Jan 2021 22:23:01 GMT; Secure;
HSID=AYQEVn…DKrdst; Domain=.foo.com; Path=/; Expires=Wed, 13 Jan 2021 22:23:01 GMT; HttpOnly
HSID=AYQEVn…DKrdst; Domain=foo.com; Path=/; Max-Age=300; HttpOnly
In the user's browser, using Javascript (ECMAScript) , you can manipulate with the Cookies without HttpOnly attribute.
Below is an example handling Cookie using the Javascript.
cookie-example.html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Cookie example</title>
<script type="text/javascript" src="cookie-example.js"></script>
</head>
<body onload="readCookie()">
Your Name: <input type="text" name="fullName" id="fullName" />
<br/>
Fovorite Color:
<select name="favoriteColor" id="favoriteColor">
<option value="red">Red</option>
<option value="blue">Blue</option>
<option value="green">Green</option>
</select>
<br>
<button onclick="writeCookies()">Write Cookies</button>
<button onclick="killCookies()">Kill Cookies</button>
<br/><br/>
<!-- Read Cookie Results: -->
<hr />
Read Cookie Results:
<div id="logArea" style="border:1px solid #ccc;padding: 5px;height:150px;">
</div>
<button value = "Get Cookie" onclick = "readCookie()">Read cookies</button>
</body>
</html>
cookie-example.js
// maxAge: Seconds
function writeCookies(maxAge) {
let expires = new Date();
expires.setSeconds(expires.getSeconds() + maxAge);
let selectedColor = document.getElementById("favoriteColor").value;
let cookie1 = "favoriteColor=" + selectedColor + ";";
cookie1 += "Expires=" + expires.toUTCString() + ";";
cookie1 += "Path=/"; // Path: Required in Chrome!!
// Write Cookie 1
document.cookie = cookie1;
let fullName = document.getElementById("fullName").value;
let cookie2 = "fullName=" + fullName + ";";
cookie2 += "Max-Age=" + maxAge + ";"; // Seconds
cookie2 += "Path=/"; // Path: Required in Chrome!!
// Write Cookie 2
document.cookie = cookie2;
alert("Write Successful!");
}
function killCookies() {
writeCookies(0);
}
function readCookie() {
var allCookies = document.cookie;
let logText = "All Cookies : " + allCookies + "<br/>";
// Get all the cookies pairs in an array
let cookieArray = allCookies.split(';');
// Now take key value pair out of this array
for (var i = 0; i < cookieArray.length; i++) {
let kvArray = cookieArray[i].split('=');
logText += "Cookie name: " + kvArray[0] + "<br/>";
logText += "Cookie value: " + kvArray[1] + "<br/>";
}
document.getElementById("logArea").innerHTML = logText;
}
You can test this example by running cookie-example.html directly on Firefox. With Chrome browser, you have to run this file on a HTTP Server.
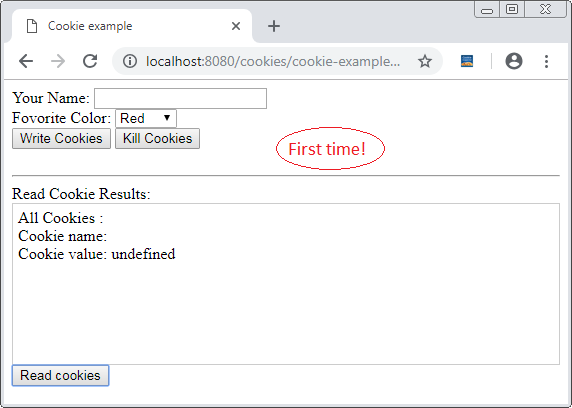
Enter a name and select your favorite color on the Form, then press the "Write Cookies" button. Cookies will be generated and saved into the user's computer.
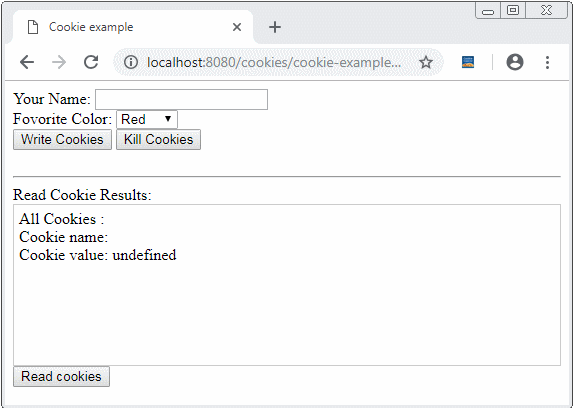
ECMAScript, Javascript Tutorials
- Introduction to Javascript and ECMAScript
- Quickstart with Javascript
- Alert, Confirm, Prompt Dialog Box in Javascript
- Quickstart with JavaScript
- JavaScript Variables Tutorial with Examples
- Bitwise Operations
- JavaScript Arrays Tutorial with Examples
- JavaScript Loops Tutorial with Examples
- JavaScript Functions Tutorial with Examples
- JavaScript Number Tutorial with Examples
- JavaScript Boolean Tutorial with Examples
- JavaScript Strings Tutorial with Examples
- JavaScript if else Statement Tutorial with Examples
- JavaScript Switch Statement
- JavaScript Error Handling Tutorial with Examples
- JavaScript Date Tutorial with Examples
- JavaScript Modules Tutorial with Examples
- The History of Modules in JavaScript
- JavaScript setTimeout and setInterval Function
- Javascript Form Validation Tutorial with Examples
- JavaScript Web Cookies Tutorial with Examples
- JavaScript void Keyword Tutorial with Examples
- Classes and Objects in JavaScript
- Class and inheritance simulation techniques in JavaScript
- Inheritance and polymorphism in JavaScript
- Undertanding Duck Typing in JavaScript
- JavaScript Symbols Tutorial with Examples
- JavaScript Set Collection Tutorial with Examples
- JavaScript Map Collection Tutorial with Examples
- Undertanding JavaScript Iterables and Iterators
- JavaScript Regular Expressions Tutorial with Examples
- JavaScript Promise, Async/Await Tutorial with Examples
- Javascript Window Tutorial with Examples
- Javascript Console Tutorial with Examples
- Javascript Screen Tutorial with Examples
- Javascript Navigator Tutorial with Examples
- Javascript Geolocation API Tutorial with Examples
- Javascript Location Tutorial with Examples
- Javascript History API Tutorial with Examples
- Javascript Statusbar Tutorial with Examples
- Javascript Locationbar Tutorial with Examples
- Javascript Scrollbars Tutorial with Examples
- Javascript Menubar Tutorial with Examples
- JavaScript JSON Tutorial with Examples
- JavaScript Event Handling Tutorial with Examples
- Javascript MouseEvent Tutorial with Examples
- Javascript WheelEvent Tutorial with Examples
- Javascript KeyboardEvent Tutorial with Examples
- Javascript FocusEvent Tutorial with Examples
- Javascript InputEvent Tutorial with Examples
- Javascript ChangeEvent Tutorial with Examples
- Javascript DragEvent Tutorial with Examples
- Javascript HashChangeEvent Tutorial with Examples
- Javascript URL Encoding Tutorial with Examples
- Javascript FileReader Tutorial with Examples
- Javascript XMLHttpRequest Tutorial with Examples
- Javascript Fetch API Tutorial with Examples
- Parsing XML in Javascript with DOMParser
- Introduction to Javascript HTML5 Canvas API
- Highlighting code with SyntaxHighlighter Javascript library
- What are polyfills in programming science?
Show More