Javascript Fetch API Tutorial with Examples
1. An overview of Fetch API
Regularly, in Javascript programming, you need to retrieve data from a URL. If you think of the XMLHttpRequest API, it will definitely be an API that helps you do what you want, but it will not friendly and effective because you have to write lengthy codes to achieve your goals. Instead, using jQuery seems to be a good idea due to the short and understandable jQuery.ajax(..) syntax, but there is one thing that you have to add the jQuery library to your application.
ES6 was introduced in June 2015 with many new features including Promise. Moreover, Fetch API is a new standard for making a request to the server and receiving a data, whose purpose is similar to the XMLHttpRequest. However, there is one difference that the Fetch API is written based on the Promise concept.
It will be easier if you grasp the concept of Promise before you continue with this lesson. Beside that, I kindly recommend that you read through the below article because I ensure that it is completely understandable for everyone.
The Fetch API builds a fetch(url, options) function to retrieve data from a URL, which returns a Promise. Itlooks like this:
function fetch(url, options) {
// A Promise
var aPromise = new Promise (
function (resolve, reject) {
if (allthingOK) {
// ...
var respone = ...;
resolve(response);
} else {
var error = new Error('Error Cause');
reject(error);
}
}
);
return aPromise;
}
Besides, the Fetch API isquite simple to use. What you really do is just call the fetch(url, options) function and get a promise to resolve the response object.
var url = "http://example.com/file";
var options = { method : 'GET', .... };
var aPromise = fetch(url, options);
aPromise
.then(function(response) {
})
.catch(function(error) {
});
var aPromise = fetch(url, {
method: "GET", // POST, PUT, DELETE, etc.
headers: {
"Content-Type": "text/plain;charset=UTF-8" // for a string body, depends on body
},
body: undefined // string, FormData, Blob, BufferSource, or URLSearchParams
referrer: "about:client", // "" for no-referrer, or an url from the current origin
referrerPolicy: "no-referrer-when-downgrade", // no-referrer, origin, same-origin...
mode: "cors", // same-origin, no-cors
credentials: "same-origin", // omit, include
cache: "default", // no-store, reload, no-cache, force-cache, or only-if-cached
redirect: "follow", // manual, error
integrity: "", // a hash, like "sha256-abcdef1234567890"
keepalive: false, // true
signal: undefined, // AbortController to abort request
window: window // null
});
2. Get Text - response.text()
Using the Fetch API to send a request and receive text is the simplest and most understandable task.
This is a simple text data.
The fetch(..) function returns a promise to resolve the response object. Therefore, to send a request to retrieve a textual data, you need to perform the first step as follows:
get-text-example.js (Step 1)
// A URL returns TEXT data.
var url = "https://ex1.o7planning.com/_testdatas_/simple-text-data.txt";
function doGetTEXT() {
// Call fetch(url) with default options.
// It returns a Promise object (Resolve response object)
var aPromise = fetch(url);
// Work with Promise object:
aPromise
.then(function(response) {
console.log("OK! Server returns a response object:");
console.log(response);
})
.catch(function(error) {
console.log("Noooooo! Something error:");
// Network Error!
console.log(error);
});
}
The response.text() method returns a promise to resolve a text object, so you are able to write the next codes for this example as follows:
get-text-example-way1.js
// A URL returns TEXT data.
var url = "https://ex1.o7planning.com/_testdatas_/simple-text-data.txt";
function doGetTEXT() {
// Call fetch(url) with default options.
// It returns a Promise object (Resolve response object)
var aPromise = fetch(url);
// Work with Promise object:
aPromise
.then(function(response) {
console.log("OK! Server returns a response object:");
console.log(response);
if(!response.ok) {
throw new Error("HTTP error, status = " + response.status);
}
response.text()
.then(function(myText) {
console.log("Text:");
console.log(myText);
})
.catch(function(error) {
// Never happened.
});
})
.catch(function(error) {
console.log("Noooooo! Something error:");
// Network Error!
console.log(error);
});
}
The Fetch API is designed to perform tasks in a chain, which may be because the promise.then(..), promise.catch(..) methodis designed to return a promise as well.
No matter what the function(response) function you write returns a normal value or a Promise object, the then() method always returns a Promise.
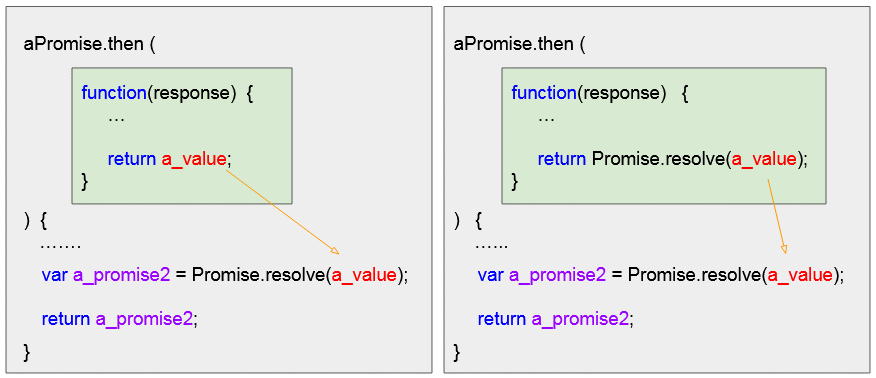
get-text-example-way2.js
// A URL returns TEXT data.
var url = "https://ex1.o7planning.com/_testdatas_/simple-text-data.txt";
function doGetTEXT() {
// Call fetch(url) with default options.
// It returns a Promise object (Resolve response object)
var aPromise = fetch(url);
// Work with Promise object:
aPromise
.then(function(response) {
console.log("OK! Server returns a response object:");
console.log(response);
if(!response.ok) {
throw new Error("HTTP error, status = " + response.status);
}
return response.text();
})
.then(function(myText) {
console.log("Text:");
console.log(myText);
})
.catch(function(error) {
console.log("Noooooo! Something error:");
// Network Error!
console.log(error);
});
}
get-text-example.html
<!DOCTYPE html>
<html>
<head>
<title>fetch get text</title>
<meta charset="UTF-8">
<script src="get-text-example-way2.js"></script>
</head>
<body>
<h3>fetch get text</h3>
<p style="color:red">
Click the button and see the results in the Console.
</p>
<button onclick="doGetJSON()">doGetJSON</button>
</body>
</html>
3. Get JSON - response.json()
Using the Fetch API to send a request and receive a JSON is a bit more complicated than those of text because you need to handle the errors when the JSON has an invalid format or NULL data.
json-simple-data.json
{
"fullName" : "Tom Cat",
"gender" : "Male",
"address" : "Walt Disney"
}
The fetch(..) function returns a promise to resolve a response object.
Step 1: Write a simple code like this:
get-json-example.js (Step 1)
// A URL returns JSON data.
var url = "https://ex1.o7planning.com/_testdatas_/json-simple-data.json";
function doGetJSON() {
// Call fetch(url) with default options.
// It returns a Promise object:
var aPromise = fetch(url);
// Work with Promise object:
aPromise
.then(function(response) {
console.log("OK! Server returns a response object:");
console.log(response);
})
.catch(function(error) {
console.log("Noooooo! Something error:");
console.log(error);
});
}
Way 1:
The response.json() method returns a promise to resolve a JSON object, so you can use the response.json().then(..) method.
get-json-example_way1.js
// A URL returns JSON data.
var url = "https://ex1.o7planning.com/_testdatas_/json-simple-data.json";
function doGetJSON() {
// Call fetch(url) with default options.
// It returns a Promise object:
var aPromise = fetch(url);
// Work with Promise object:
aPromise
.then(function(response) {
console.log("OK! Server returns a response object:");
console.log(response);
if(!response.ok) {
throw new Error("HTTP error, status = " + response.status);
}
// Get JSON Promise from response object:
var myJSON_promise = response.json();
// Work with Promise object:
myJSON_promise.then(function(myJSON)) {
console.log("OK! JSON:");
console.log(myJSON);
}
})
.catch(function(error) {
console.log("Noooooo! Something error:");
console.log(error);
});
}
Way 2:
get-json-example-way2.js
// A URL returns JSON data.
var url = "https://ex1.o7planning.com/_testdatas_/json-simple-data.json";
function doGetJSON() {
// Call fetch(url) with default options.
// It returns a Promise object:
var aPromise = fetch(url);
// Work with Promise object:
aPromise
.then(function(response) {
console.log("OK! Server returns a response object:");
console.log(response);
if(!response.ok) {
throw new Error("HTTP error, status = " + response.status);
}
// Get JSON Promise from response object:
var myJSON_promise = response.json();
// Returns a Promise object.
return myJSON_promise;
})
.then(function(myJSON) {
console.log("OK! JSON:");
console.log(myJSON);
})
.catch(function(error) {
console.log("Noooooo! Something error:");
console.log(error);
});
}
get-json-example.html
<!DOCTYPE html>
<html>
<head>
<title>fetch get json</title>
<meta charset="UTF-8">
<script src="get-json-example-way2.js"></script>
</head>
<body>
<h3>fetch get json</h3>
<p style="color:red">
Click the button and see the results in the Console.
</p>
<button onclick="doGetJSON()">doGetJSON</button>
</body>
</html>
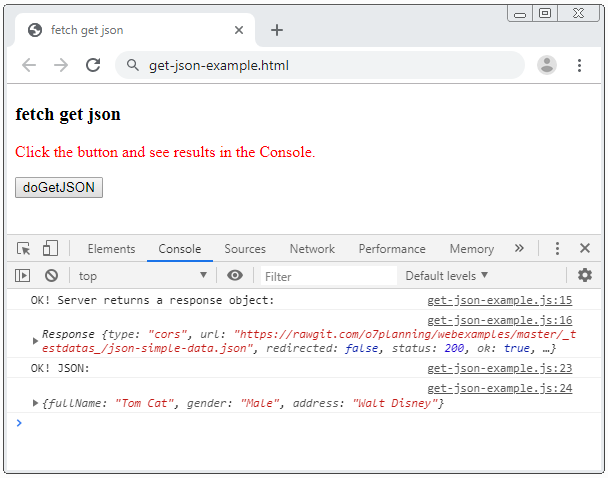
Null JSON Data?
Note: The response.json() method will throw an error, if your URL returns a non-JSON, an invalid JSON, or empty data.
Supposing that you send a request to get the information of an employee by ID. If the employee exists, you will receive a JSON data type. But if the employee does not exist, you will receive a NULL data. However, the NULL data causes an error in the place that call the response.json(). Take it as an example:
- http://example.com/employee?id=123
// Get information of Employee 123:
fetch("http://example.com/employee?id=123")
.then(function(response) {
console.log("OK! Server returns a response object:");
console.log(response);
if(!response.ok) {
throw new Error("HTTP error, status = " + response.status);
}
// Error if Employee 123 does not exist.
// (*** URL returns null).
var myJSON_promise = response.json(); // =====> Error
return myJSON_promise;
})
.then(function(myJSON) {
console.log("OK! JSON:");
console.log(myJSON);
})
.catch(function(error) {
console.log("Noooooo! Something error:");
console.log(error);
});
(Error)
SyntaxError: Unexpected end of JSON input
Use the response.text() instead of the response.json():
get-json-null-example.js
// A URL returns null JSON data.
var url = "https://ex1.o7planning.com/_testdatas_/json-null-data.json";
function doGetJSON() {
// Call fetch(url) with default options.
// It returns a Promise object:
var aPromise = fetch(url);
// Work with Promise object:
aPromise
.then(function(response) {
console.log("OK! Server returns a response object:");
console.log(response);
if(!response.ok) {
throw new Error("HTTP error, status = " + response.status);
}
// Get TEXT Promise object from response object:
var myText_promise = response.text();
return myText_promise;
})
.then(function(myText) {
console.log("OK! TEXT:");
console.log(myText);
var myJSON = null;
if(myText != null && myText.trim().length > 0) {
myJSON = JSON.parse(myText);
}
console.log("OK! JSON:");
console.log(myJSON);
})
.catch(function(error) {
console.log("Noooooo! Something error:");
console.log(error);
});
}
get-json-null-example.html
<!DOCTYPE html>
<html>
<head>
<title>fetch get json</title>
<meta charset="UTF-8">
<script src="get-json-null-example.js"></script>
</head>
<body>
<h3>fetch get null json</h3>
<p style="color:red">
Click the button and see the results in the Console.
</p>
<button onclick="doGetJSON()">doGetJSON</button>
</body>
</html>
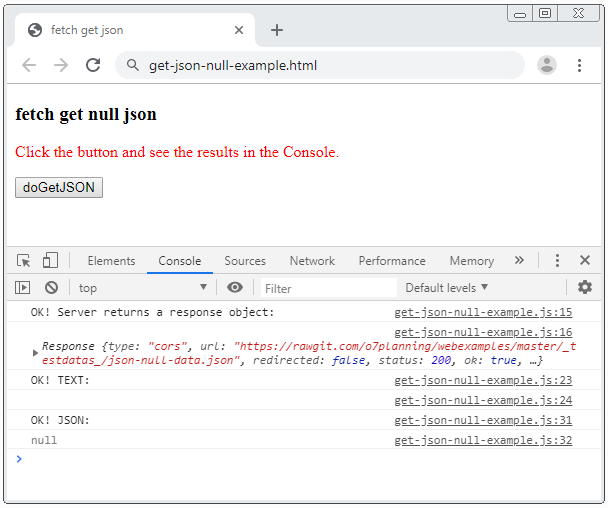
4. Get Blob - response.blob()
The response.blob() method returns a promise to resolve a Blob object.
In the following example, I use the Fetch API to download an image given by a URL and display it on the page.
get-blob-example.js
// A URL returns Image data (Blob).
var url = "https://ex1.o7planning.com/_testdatas_/triceratops.png";
function doGetBlob() {
// Call fetch(url) with default options.
// It returns a Promise object:
var aPromise = fetch(url);
// Work with Promise object:
aPromise
.then(function(response) {
console.log("OK! Server returns a response object:");
console.log(response);
if(!response.ok) {
throw new Error("HTTP error, status = " + response.status);
}
// Get Blob Promise from response object:
var myBlob_promise = response.blob();
return myBlob_promise;
})
.then(function(myBlob) {
console.log("OK! Blob:");
console.log(myBlob);
var objectURL = URL.createObjectURL(myBlob);
var myImage = document.getElementById("my-img");
myImage.src = objectURL;
})
.catch(function(error) {
console.log("Noooooo! Something error:");
console.log(error);
});
}
get-blob-example.html
<!DOCTYPE html>
<html>
<head>
<title>fetch get blob</title>
<meta charset="UTF-8">
<script src="get-blob-example.js"></script>
</head>
<body>
<h3>fetch get Blob</h3>
<p style="color:red">
Click the button to load Image.
</p>
<button onclick="doGetBlob()">doGetBlob</button>
<br/>
<img id="my-img" src="" height = 150 />
</body>
</html>
5. Get ArrayBuffer - response.arrayBuffer()
The response.arrayBuffer() method returns a promise to resolve an ArrayBuffer object.
Data downloaded as a buffer helps you work immediately with it without waiting for the entire data to be downloaded. Take a large Video as an example, you can watch the Video while its data is still being downloaded to your browser.
The example below uses the Fetch API to play a music file:
get-arraybuffer-example.js
// A URL returns Audio data.
var url = "https://ex1.o7planning.com/_testdatas_/yodel.mp3";
// AudioContext
const audioContext = new AudioContext();
var bufferSource;
// Get ArrayBuffer (Audio data) and play it.
function doGetArrayBuffer() {
bufferSource = audioContext.createBufferSource();
bufferSource.onended = function () {
document.getElementById("play").disabled=false;
};
// Call fetch(url) with default options.
// It returns a Promise object:
var aPromise = fetch(url);
// Work with Promise object:
var retPromise =
aPromise
.then(function(response) {
if(!response.ok) {
throw new Error("HTTP error, status = " + response.status);
}
// Get ArrayBuffer Promise object from response object:
var myArrayBuffer_promise = response.arrayBuffer();
return myArrayBuffer_promise;
})
.then(function(myArrayBuffer) {
audioContext.decodeAudioData(myArrayBuffer, function(decodedData) {
bufferSource.buffer = decodedData;
bufferSource.connect(audioContext.destination);
});
});
return retPromise;
}
function playIt() {
console.clear();
doGetArrayBuffer()
.then(function() {
document.getElementById("play").disabled=true;
bufferSource.start(0);
})
.catch(function(error){
alert("Something Error: " + error.message);
});
}
function stopIt() {
bufferSource.stop(0);
document.getElementById("play").disabled=false;
}
get-arraybuffer-example.html
<!DOCTYPE html>
<html>
<head>
<title>fetch get ArrayBuffer</title>
<meta charset="UTF-8">
<script src="get-arraybuffer-example.js"></script>
</head>
<body>
<h3>fetch get ArrayBuffer</h3>
<p style="color:red">
Click the button to play Audio.
</p>
<button onclick="playIt()" id="play">Play Audio</button>
<button onclick="stopIt()" id="play">Stop Audio</button>
</body>
</html>
Web Audio API helps you work with Audio in the web environment, it is a large API and is related to Fetch API, If you are interested in this topic, you should read the following article:
- Javascript Web Audio API
6. Get FormData - response.formData()
The response.formData() method returns a promise to resolve a FormData object. This method is usually used at Service Workers. When users send Form data to the server, those data will go through the Service Workers before going to the server. At Service Workers, you can call response.formData() method as well as modify the values in FormData if you want.
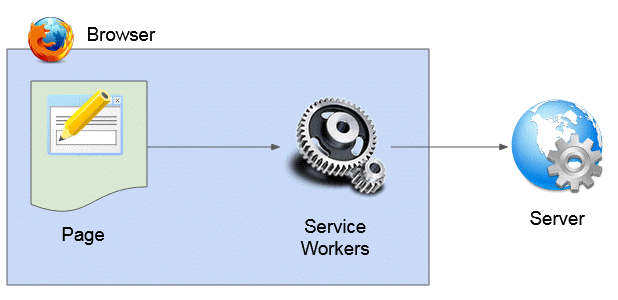
- Javascript Service Worker
ECMAScript, Javascript Tutorials
- Introduction to Javascript and ECMAScript
- Quickstart with Javascript
- Alert, Confirm, Prompt Dialog Box in Javascript
- Quickstart with JavaScript
- JavaScript Variables Tutorial with Examples
- Bitwise Operations
- JavaScript Arrays Tutorial with Examples
- JavaScript Loops Tutorial with Examples
- JavaScript Functions Tutorial with Examples
- JavaScript Number Tutorial with Examples
- JavaScript Boolean Tutorial with Examples
- JavaScript Strings Tutorial with Examples
- JavaScript if else Statement Tutorial with Examples
- JavaScript Switch Statement
- JavaScript Error Handling Tutorial with Examples
- JavaScript Date Tutorial with Examples
- JavaScript Modules Tutorial with Examples
- The History of Modules in JavaScript
- JavaScript setTimeout and setInterval Function
- Javascript Form Validation Tutorial with Examples
- JavaScript Web Cookies Tutorial with Examples
- JavaScript void Keyword Tutorial with Examples
- Classes and Objects in JavaScript
- Class and inheritance simulation techniques in JavaScript
- Inheritance and polymorphism in JavaScript
- Undertanding Duck Typing in JavaScript
- JavaScript Symbols Tutorial with Examples
- JavaScript Set Collection Tutorial with Examples
- JavaScript Map Collection Tutorial with Examples
- Undertanding JavaScript Iterables and Iterators
- JavaScript Regular Expressions Tutorial with Examples
- JavaScript Promise, Async/Await Tutorial with Examples
- Javascript Window Tutorial with Examples
- Javascript Console Tutorial with Examples
- Javascript Screen Tutorial with Examples
- Javascript Navigator Tutorial with Examples
- Javascript Geolocation API Tutorial with Examples
- Javascript Location Tutorial with Examples
- Javascript History API Tutorial with Examples
- Javascript Statusbar Tutorial with Examples
- Javascript Locationbar Tutorial with Examples
- Javascript Scrollbars Tutorial with Examples
- Javascript Menubar Tutorial with Examples
- JavaScript JSON Tutorial with Examples
- JavaScript Event Handling Tutorial with Examples
- Javascript MouseEvent Tutorial with Examples
- Javascript WheelEvent Tutorial with Examples
- Javascript KeyboardEvent Tutorial with Examples
- Javascript FocusEvent Tutorial with Examples
- Javascript InputEvent Tutorial with Examples
- Javascript ChangeEvent Tutorial with Examples
- Javascript DragEvent Tutorial with Examples
- Javascript HashChangeEvent Tutorial with Examples
- Javascript URL Encoding Tutorial with Examples
- Javascript FileReader Tutorial with Examples
- Javascript XMLHttpRequest Tutorial with Examples
- Javascript Fetch API Tutorial with Examples
- Parsing XML in Javascript with DOMParser
- Introduction to Javascript HTML5 Canvas API
- Highlighting code with SyntaxHighlighter Javascript library
- What are polyfills in programming science?
Show More