NodeJS Modules Tutorial with Examples
1. What is NodeJS Module?
Speaking simply, NodeJS Module is a Javascript library. It is a set of functions, objects and variables, which you can put into your applications to use. Using Module helps simplify writing code and manage it in your application. Normally, each module will be written in a separate file.
The NodeJS builds in quite many Modules. It has standard libraries for you to develop applications. Below is the list:
Module | Description |
assert | Provides a set of assertion tests |
buffer | To handle binary data |
child_process | To run a child process |
cluster | To split a single Node process into multiple processes |
crypto | To handle OpenSSL cryptographic functions |
dgram | Provides implementation of UDP datagram sockets |
dns | To do DNS lookups and name resolution functions |
events | To handle events |
fs | To handle the file system |
http | To make Node.js act as an HTTP server |
https | To make Node.js act as an HTTPS server. |
net | To create servers and clients |
os | Provides information about the operation system |
path | To handle file paths |
querystring | To handle URL query strings |
readline | To handle readable streams one line at the time |
stream | To handle streaming data |
string_decoder | To decode buffer objects into strings |
timers | To execute a function after a given number of milliseconds |
tls | To implement TLS and SSL protocols |
tty | Provides classes used by a text terminal |
url | To parse URL strings |
util | To access utility functions |
v8 | To access information about V8 JavaScript engine |
vm | To compile JavaScript code in a virtual machine |
zlib | To compress or decompress files |
To use a module, use require(moduleName) function. For example, http is a module that makes the NodeJS work like a HTTP Server.
Make sure that you have created a NodeJS project successfully. If not, please refer to the following lesson:
On your NodeJS project, create a module-examples directory to contain the Javascript files which you practise in this lesson.
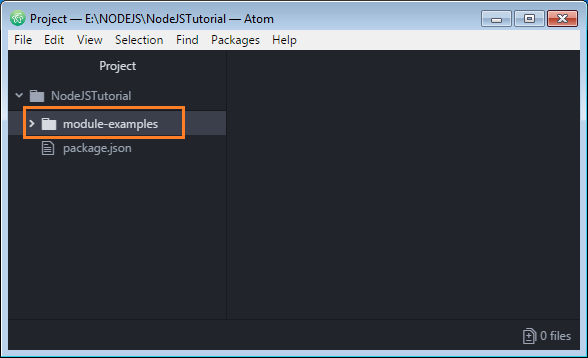
Create a file named http-example.js in the module-examples directory.
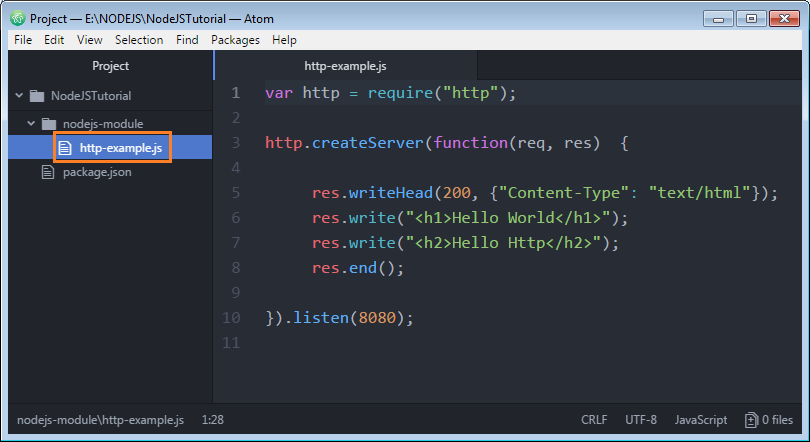
http-example.js
var http = require("http");
http.createServer(function(req, res) {
res.writeHead(200, {"Content-Type": "text/html"});
res.write("<h1>Hello World</h1>");
res.write("<h2>Hello Http</h2>");
res.end();
}).listen(8080);
Open CMD and CD windows to go to your project directory, use the NodeJS to run the http-example.js file:
node ./module-examples/http-example.js
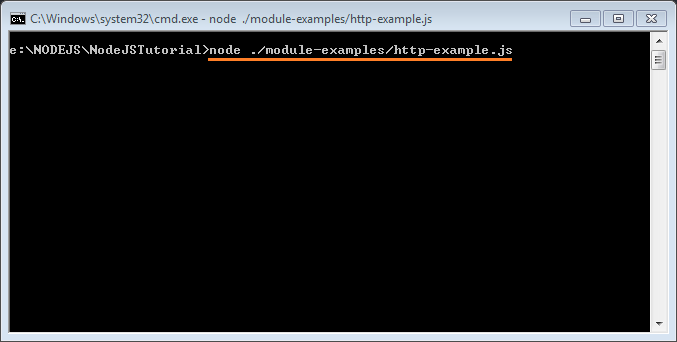
Open the browser and visit the path:
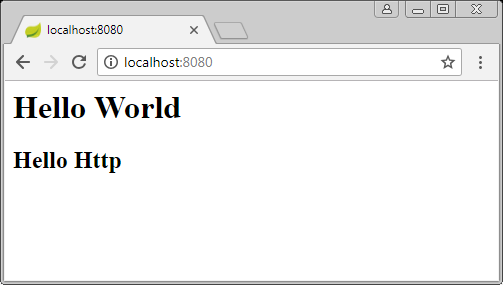
2. Create your Module
In the NodeJS, you can create a customized module. The Module is a Javascript file. please note to the position of this file because it affect the way for you to use it . You have 2 ways of choosing position of this file:
- The Module file is put in the directory named node_modules (subdirectory of the project). This is encouraged position.
- The Module file is put in one of your directories, not node_modules.
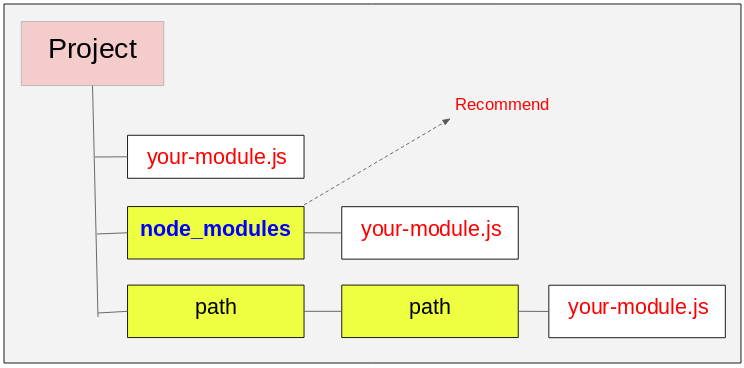
The NodeJS advises you to place your Module files into the node_modules directory of the project. Wait for a minute, I am going to explain the reason why your module should be put in this directory.
The syntax for exporting something in your Javascript file.
exports = something;
// or:
module.exports = something;
my-first-module.js
First of all, create a my-first-module.js file in the node_modules directory and the test-my-first-module.js file in another directory.
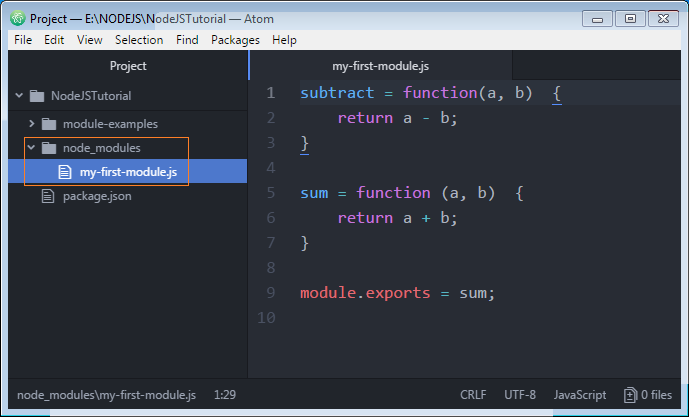
In this example, the my-first-module.js file has a lot of functions but I only export"sum" function.
node_modules/my-first-module.js
subtract = function(a, b) {
return a - b;
}
sum = function (a, b) {
return a + b;
}
module.exports = sum;
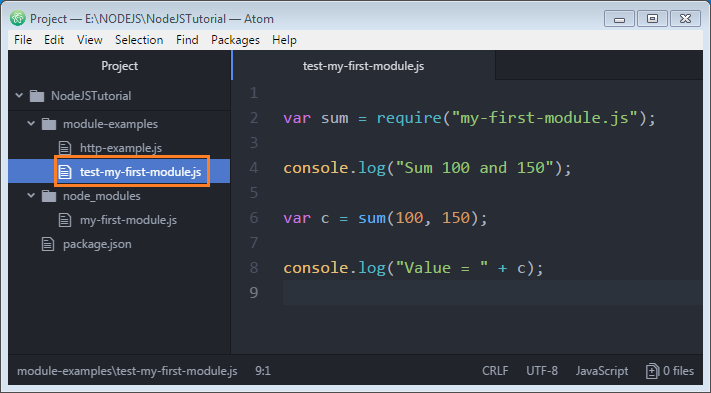
module-examples/test-my-first-module.js
var sum = require("my-first-module.js");
console.log("Sum 100 and 150");
var c = sum(100, 150);
console.log("Value = " + c);
Run the above example:
node ./module-examples/test-my-first-module.js
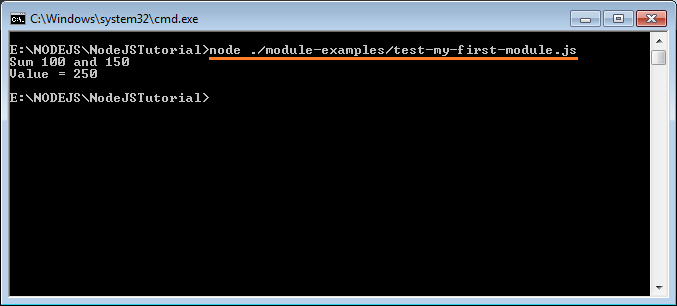
For the module files placed in the node_modules directory, the way of using it is very simple. You don't need to be interest in the relative path or absolute path.var myVar = require("module-file-name.js"); // or: var myVar = require("module-file-name");
my-second-module.js
Next, we create a my-second-module.js file located in a directory, for example, node_modules_2.
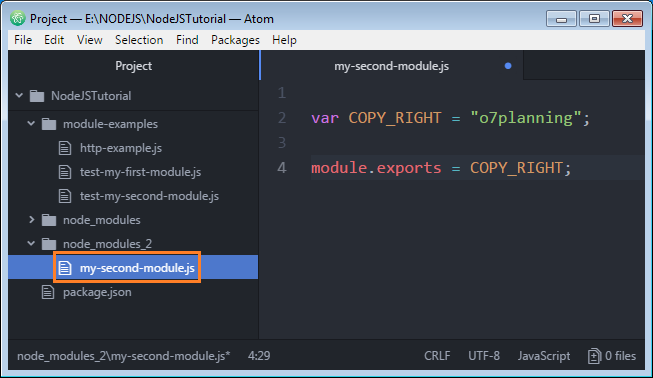
node_modules_2/my-second-module.js
var COPY_RIGHT = "o7planning";
module.exports = COPY_RIGHT;
To use the modules located in other directory with node_modules, you have to use the relative path.
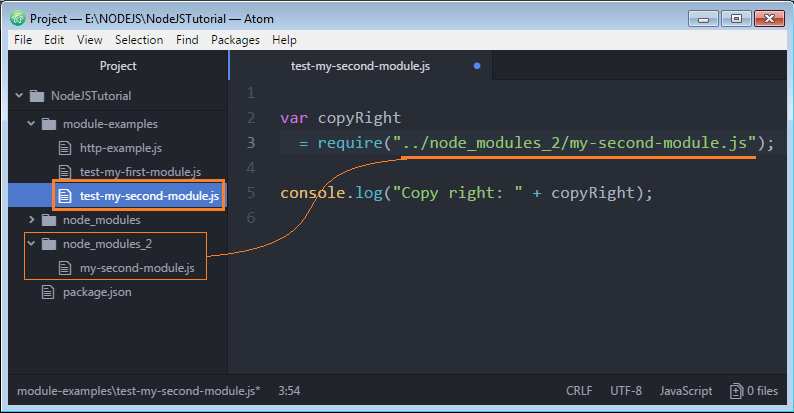
module-examples/test-my-second-module.js
var copyRight
= require("../node_modules_2/my-second-module.js");
console.log("Copy right: " + copyRight);
node ./module-examples/test-my-second-module.js
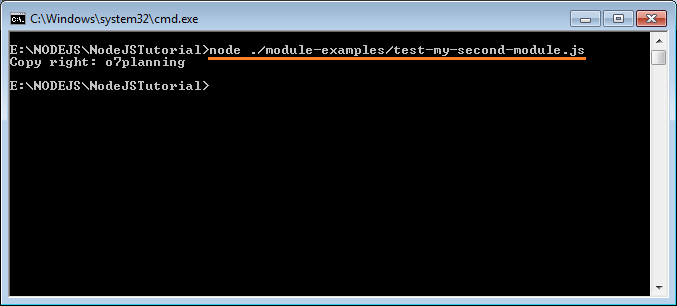
3. Export many things
A module file can consist of a lot of functions, variables, and classes. This example shows you how to export many things in this file.
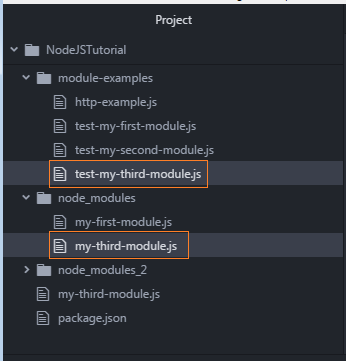
node_modules/my-third-module.js
// A variable!!
var COPY_RIGHT = "o7planning";
// A function!!
var multiple = function(a, b) {
return a * b;
};
// A class!!
var Person = class {
constructor(name, age) {
this.name = name;
this.age = age;
}
showInfo() {
console.log("name="+ this.name+", age="+ this.age);
}
};
// Something to Export!!
var somethingToExport = {
Person: Person,
multiple : multiple,
COPY_RIGHT : COPY_RIGHT
};
// Exprort it!!
module.exports = somethingToExport;
module-examples/test-my-third-module.js
var module3 = require("my-third-module.js");
console.log("Using Person class of module:");
var p = new module3.Person("Smith", 20);
p.showInfo();
console.log(" -- ");
console.log("Using multiple Function of module:");
var c = module3.multiple(20, 5);
console.log("Value= "+ c);
console.log(" -- ");
console.log("Using Variable of module:");
var copyRight = module3.COPY_RIGHT;
console.log("Copy right: " + copyRight);
node ./module-examples/test-my-third-module.js
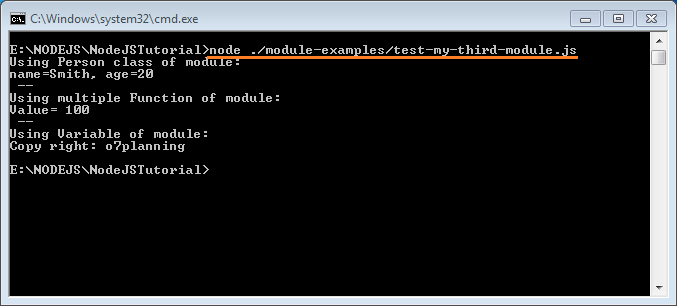
NodeJS Tutorials
- Introduction to NodeJs
- What is NPM?
- NodeJS Tutorial for Beginners
- Install Atom Editor
- Install NodeJS on Windows
- NodeJS Modules Tutorial with Examples
- The concept of Callback in NodeJS
- Create a Simple HTTP Server with NodeJS
- Understanding Event Loop in NodeJS
- NodeJS EventEmitter Tutorial with Examples
- Connect to MySQL database in NodeJS
Show More