Spring Tutorial for Beginners
1. Introduction
This document is written based on:
Spring Framework 4.x
Eclipse 4.6 NEON (ok for Eclipse 4.5 MARS)
In this document, I use Maven to declare the Spring library will use, instead of downloading Spring library and declaring in the normal way.
Maven is a tool that help you manage your library automatically and efficiently, and it has become customary which any Java programmers must know. If you do not know about Maven you can spend 10 minutes to learn how to use it here:
Maven is a tool that help you manage your library automatically and efficiently, and it has become customary which any Java programmers must know. If you do not know about Maven you can spend 10 minutes to learn how to use it here:
In case you want to download Spring and declare the library in the traditional way you can see the appendix at the end of the document.
2. Spring Framework
The illustration below shows the structure of the Spring Framework.
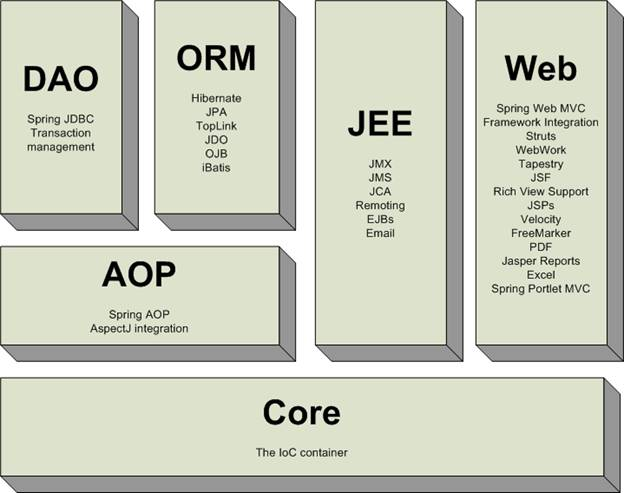
- IoC Container: This is the most important and also the basis, the foundation of Spring. It is the role of configuration and lifecycle management of Java objects. Posts Today we will learn about this part.
- DAO, ORM, AOP, WEB: The module is available tool or framework is integrated into the Spring.
Inversion of Control & Dependency Injection
To understand this issue we take a few classes below:
// Interface HelloWorld
public interface HelloWorld {
public void sayHello();
}
// Class implements HelloWorld
public class SpringHelloWorld implements HelloWorld {
public void sayHello() {
System.out.println("Spring say Hello!");
}
}
// Other class implements HelloWorld
public class StrutsHelloWorld implements HelloWorld {
public void sayHello() {
System.out.println("Struts say Hello!");
}
}
// And Service class
public class HelloWorldService {
// Field type HelloWorld
private HelloWorld helloWorld;
// Constructor HelloWorldService
// It initializes the values for the field 'helloWorld'
public HelloWorldService() {
this.helloWorld = new StrutsHelloWorld();
}
}
It is obvious that class HelloWorldService manages the creation of HelloWorld objects.
- In the above situation, when a HelloWorldService object is created from its constructor, HelloWorld object is also created. It is created from StrutsHelloWorld.
- In the above situation, when a HelloWorldService object is created from its constructor, HelloWorld object is also created. It is created from StrutsHelloWorld.
The question is that you want to create a HelloWorldService object and HelloWorld object is simultaneously created, but it has to be SpringHelloWorld.
So HelloWorldService is controlling "object creation" of HelloWorld. Why don't we transfer the creation of HelloWorld to a third party, instead of making it in HelloWorldService? We have the definition of "inversion of control" (IoC).
So HelloWorldService is controlling "object creation" of HelloWorld. Why don't we transfer the creation of HelloWorld to a third party, instead of making it in HelloWorldService? We have the definition of "inversion of control" (IoC).
And IoC Container will act as manager and creating both HelloWorldService and HelloWorld.
IoC = Inversion of Control
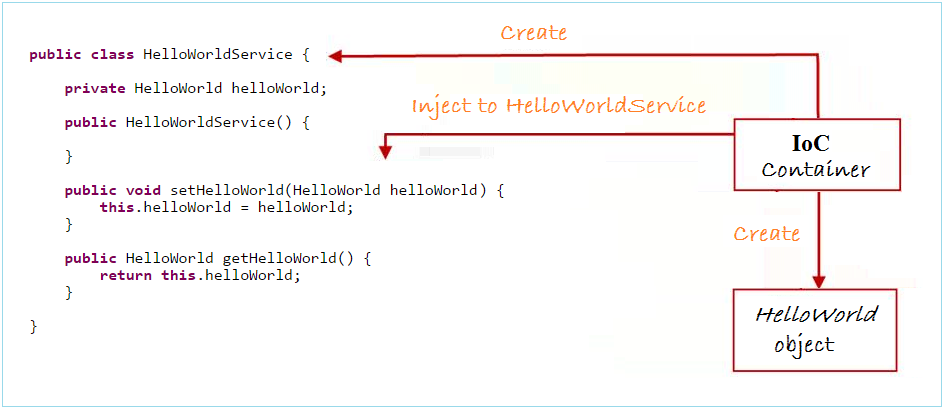
IoC container creates HelloWorldService object and then pass the object HelloWorld into HelloWorldService through setter. The IoC container is doing is "dependency injection" into HelloWorldService. The dependence here means that the dependence between objects: HelloWorldService and HelloWorld.
At this point we have identified clearly what IoC & DI. Let's take an example to better understand.
At this point we have identified clearly what IoC & DI. Let's take an example to better understand.
3. Create Maven Project
- File/New/Other...
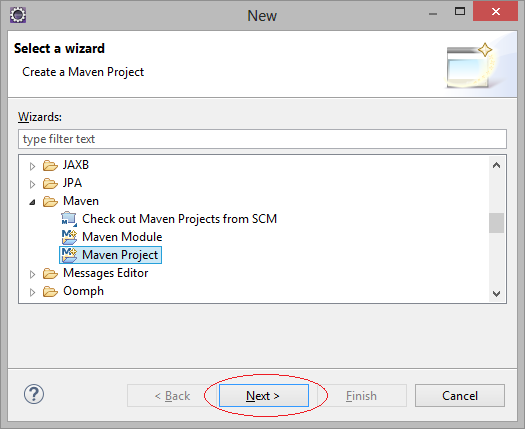
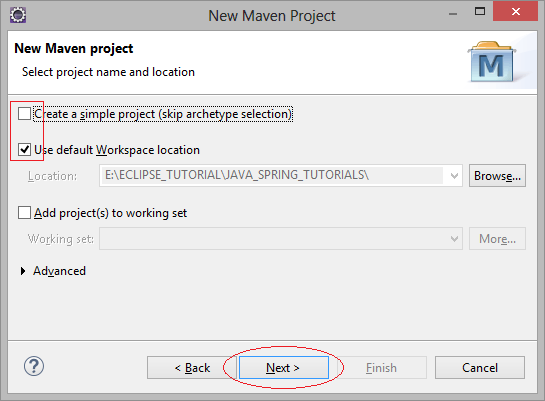
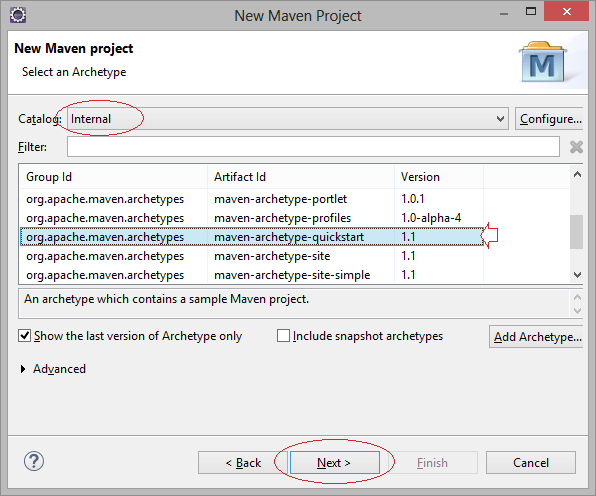
Enter:
- Group Id: org.o7planning
- Artifact Id: HelloSpringAnnotation
- package: org.o7planning.spring
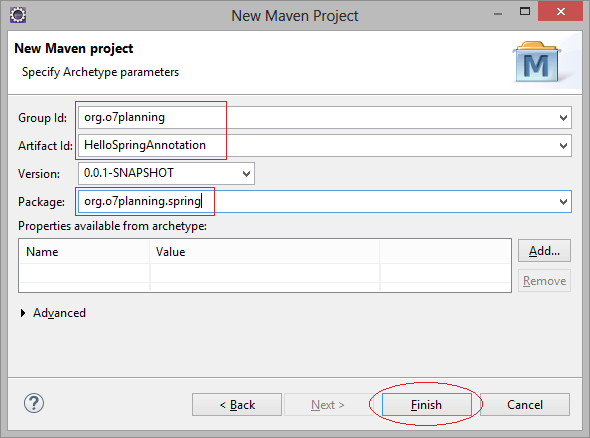
Your project has been created:
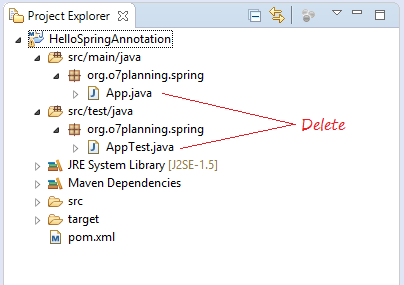
Ensure that your Project is built on Java 7 or newer. Right-click the project and select Properties.
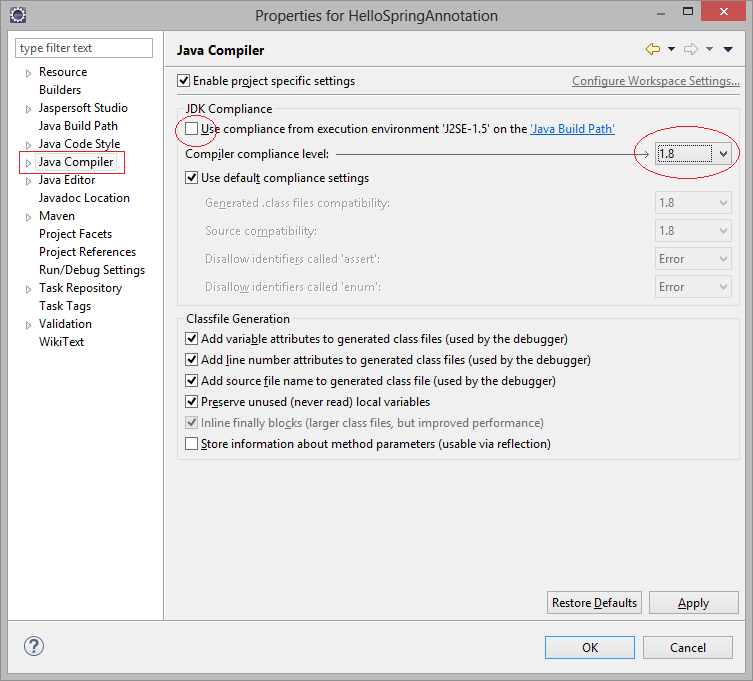
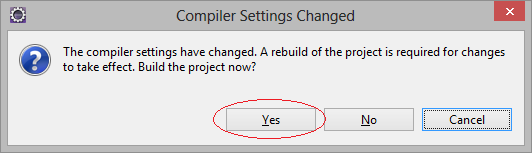
4. Declaring basic library of Spring
This is HelloWorld Spring example, so we just use the basic Spring library (Core). Open the pom.xml file to declare the library will use:
pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0
http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.o7planning</groupId>
<artifactId>HelloSpringAnnotation</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>jar</packaging>
<name>HelloSpringAnnotation</name>
<url>http://maven.apache.org</url>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
</properties>
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>3.8.1</version>
<scope>test</scope>
</dependency>
<!-- Spring Core -->
<!-- http://mvnrepository.com/artifact/org.springframework/spring-core -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-core</artifactId>
<version>4.1.4.RELEASE</version>
</dependency>
<!-- Spring Context -->
<!-- http://mvnrepository.com/artifact/org.springframework/spring-context -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>4.1.4.RELEASE</version>
</dependency>
</dependencies>
</project>
5. Code Project
Below is an illustration of the project structure:
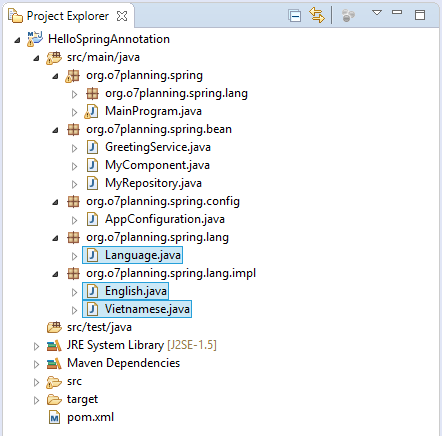
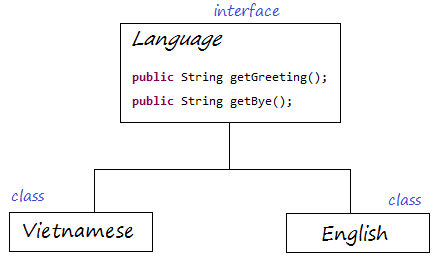
Language.java
package org.o7planning.spring.lang;
// A Language
public interface Language {
// Get a greeting
public String getGreeting();
// Get a bye
public String getBye();
}
English.java
package org.o7planning.spring.lang.impl;
import org.o7planning.spring.lang.Language;
// English
public class English implements Language {
@Override
public String getGreeting() {
return "Hello";
}
@Override
public String getBye() {
return "Bye bye";
}
}
Vietnamese.java
package org.o7planning.spring.lang.impl;
import org.o7planning.spring.lang.Language;
// Vietnamese
public class Vietnamese implements Language {
@Override
public String getGreeting() {
return "Xin Chao";
}
@Override
public String getBye() {
return "Tam Biet";
}
}
@Service is an annotation which is used to annotate on a class to inform Spring that the class is a Spring BEAN.
@Autowired is annotated on a field to inform Spring that let's inject value into the field. Note: The meaning of term "inject" is similar to assigning a value to that field.
@Autowired is annotated on a field to inform Spring that let's inject value into the field. Note: The meaning of term "inject" is similar to assigning a value to that field.
GreetingService.java
package org.o7planning.spring.bean;
import org.o7planning.spring.lang.Language;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
@Service
public class GreetingService {
@Autowired
private Language language;
public GreetingService() {
}
public void sayGreeting() {
String greeting = language.getGreeting();
System.out.println("Greeting: " + greeting);
}
}
@Repository is an annotation which is used to annotate on a class to inform Spring that the class is a Spring BEAN.
MyRepository.java
package org.o7planning.spring.bean;
import java.util.Date;
import org.springframework.stereotype.Repository;
@Repository
public class MyRepository {
public String getAppName() {
return "Hello Spring App";
}
public Date getSystemDateTime() {
return new Date();
}
}
@Component is an annotation which is used to annotate on a class to inform Spring that the class is a Spring BEAN.
@Autowired is annotated on a field to inform Spring that let's inject value into the field. Note: The meaning of term "inject" is similar to assigning a value to that field.
@Autowired is annotated on a field to inform Spring that let's inject value into the field. Note: The meaning of term "inject" is similar to assigning a value to that field.
MyComponent
package org.o7planning.spring.bean;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
@Component
public class MyComponent {
@Autowired
private MyRepository repository;
public void showAppInfo() {
System.out.println("Now is: "+ repository.getSystemDateTime());
System.out.println("App Name: "+ repository.getAppName());
}
}
There is no difference between the usage of @Service, @Component and @Repository, you can use them to annotate on your class that is suitable for the meaning and context of the application.
6. Spring @Configuration & IoC
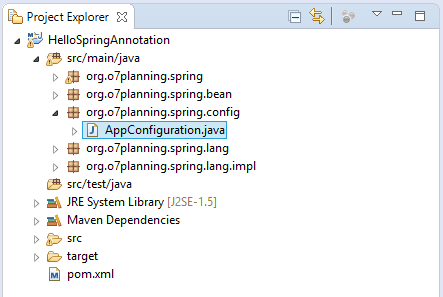
@Configuration is an annotation which is annotated in a class defining Spring BEANs.
@ComponentScan - Deliver report to Spring about packages to search for other Spring BEANs, Spring will scan the packages to search for.
@ComponentScan - Deliver report to Spring about packages to search for other Spring BEANs, Spring will scan the packages to search for.
AppConfiguration.java
package org.o7planning.spring.config;
import org.o7planning.spring.lang.Language;
import org.o7planning.spring.lang.impl.Vietnamese;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.ComponentScan;
import org.springframework.context.annotation.Configuration;
@Configuration
@ComponentScan({"org.o7planning.spring.bean"})
public class AppConfiguration {
@Bean(name ="language")
public Language getLanguage() {
return new Vietnamese();
}
}
Spring BEANs that are created will be managed in Spring IoC Container.
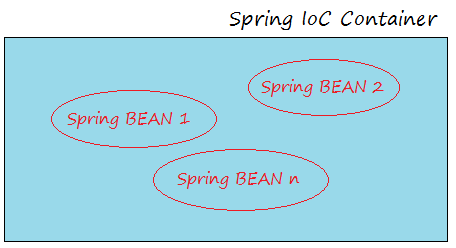
7. Spring ApplicationContext
MainProgram.java
package org.o7planning.spring;
import org.o7planning.spring.bean.GreetingService;
import org.o7planning.spring.bean.MyComponent;
import org.o7planning.spring.config.AppConfiguration;
import org.o7planning.spring.lang.Language;
import org.springframework.context.ApplicationContext;
import org.springframework.context.annotation.AnnotationConfigApplicationContext;
public class MainProgram {
public static void main(String[] args) {
// Creating a Context Application object by reading
// the configuration of the 'AppConfiguration' class.
ApplicationContext context = new AnnotationConfigApplicationContext(AppConfiguration.class);
System.out.println("----------");
Language language = (Language) context.getBean("language");
System.out.println("Bean Language: "+ language);
System.out.println("Call language.sayBye(): "+ language.getBye());
System.out.println("----------");
GreetingService service = (GreetingService) context.getBean("greetingService");
service.sayGreeting();
System.out.println("----------");
MyComponent myComponent = (MyComponent) context.getBean("myComponent");
myComponent.showAppInfo();
}
}
Run MainProgram class

Results:
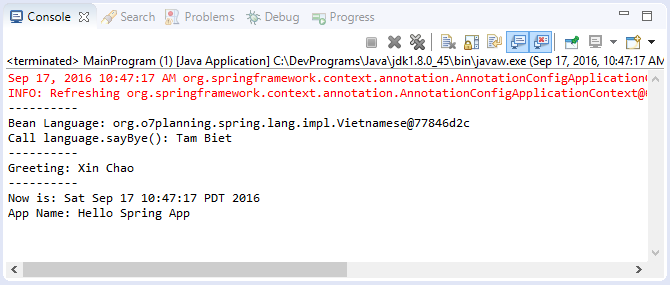
8. Working Principle of Spring
You create an ApplicationContext object by reading configures in class AppConfiguration. For example, see the code below.
ApplicationContext context = new AnnotationConfigApplicationContext(AppConfiguration.class);
Spring will create Spring BEANs according to the definitions in class AppConfiguration (Note: AppConfiguration class must be annotated by @Configuration).
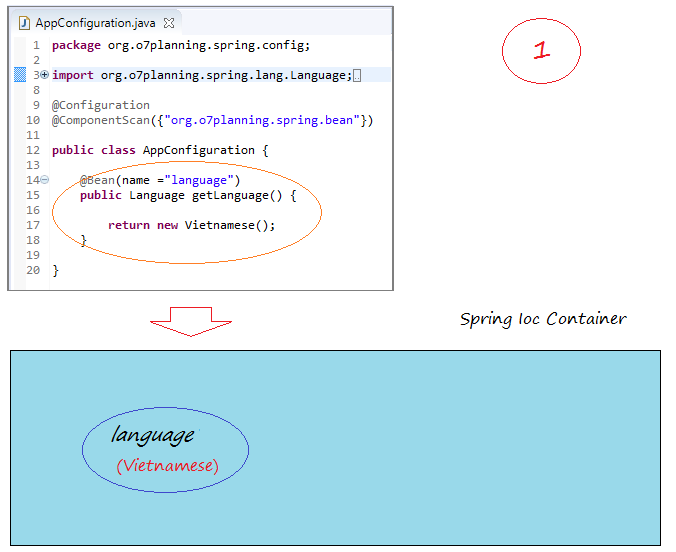
Next, Spring will search in package "org.o7planning.spring.bean" in order to create other Spring BEANs (Create objects from classes annotated by @Service, @Component or @Repository).
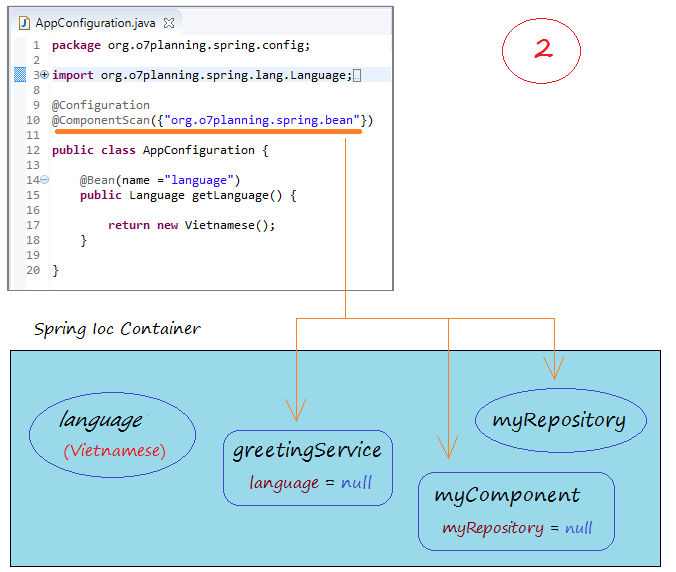
Spring BEAN is now created and is contained in Spring IoC. The fields of Spring BEANs are annotated by @Autowired that will have values injected. Let's see illustration below:
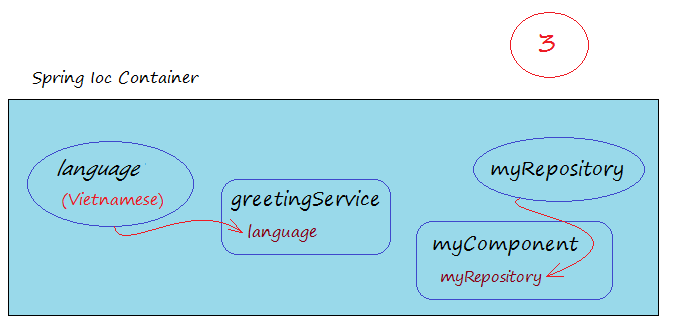
So, what is IoC?In case that an object is created from a class traditionally, its fields have value assigned inside the class. Reversely, for Spring, its objects and fields have value injected from the outside by an object called as IoC.
IoC stands for "Inversion of Control"
IoC Container is a container containing all Spring BEANs used in the application.
9. Programming Web Application using Spring Boot
Next you can learn programming web applications with Spring Boot:
Spring MVC Tutorials
- Spring Tutorial for Beginners
- Install Spring Tool Suite for Eclipse
- Spring MVC Tutorial for Beginners - Hello Spring 4 MVC
- Configure Static Resources in Spring MVC
- Spring MVC Interceptors Tutorial with Examples
- Create a Multiple Languages web application with Spring MVC
- Spring MVC File Upload Tutorial with Examples
- Simple Login Java Web Application using Spring MVC, Spring Security and Spring JDBC
- Spring MVC Security with Hibernate Tutorial with Examples
- Spring MVC Security and Spring JDBC Tutorial (XML Config)
- Social Login in Spring MVC with Spring Social Security
- Spring MVC and Velocity Tutorial with Examples
- Spring MVC and FreeMarker Tutorial with Examples
- Use Template in Spring MVC with Apache Tiles
- Spring MVC and Spring JDBC Transaction Tutorial with Examples
- Use Multiple DataSources in Spring MVC
- Spring MVC and Hibernate Transaction Tutorial with Examples
- Spring MVC Form Handling and Hibernate Tutorial with Examples
- Run background scheduled tasks in Spring
- Create a Java Shopping Cart Web Application using Spring MVC and Hibernate
- Simple CRUD example with Spring MVC RESTful Web Service
- Deploy Spring MVC on Oracle WebLogic Server
Show More
Spring Boot Tutorials
- Install Spring Tool Suite for Eclipse
- Spring Tutorial for Beginners
- Spring Boot Tutorial for Beginners
- Spring Boot Common Properties
- Spring Boot and Thymeleaf Tutorial with Examples
- Spring Boot and FreeMarker Tutorial with Examples
- Spring Boot and Groovy Tutorial with Examples
- Spring Boot and Mustache Tutorial with Examples
- Spring Boot and JSP Tutorial with Examples
- Spring Boot, Apache Tiles, JSP Tutorial with Examples
- Use Logging in Spring Boot
- Application Monitoring with Spring Boot Actuator
- Create a Multi Language web application with Spring Boot
- Use multiple ViewResolvers in Spring Boot
- Use Twitter Bootstrap in Spring Boot
- Spring Boot Interceptors Tutorial with Examples
- Spring Boot, Spring JDBC and Spring Transaction Tutorial with Examples
- Spring JDBC Tutorial with Examples
- Spring Boot, JPA and Spring Transaction Tutorial with Examples
- Spring Boot and Spring Data JPA Tutorial with Examples
- Spring Boot, Hibernate and Spring Transaction Tutorial with Examples
- Integrating Spring Boot, JPA and H2 Database
- Spring Boot and MongoDB Tutorial with Examples
- Use Multiple DataSources with Spring Boot and JPA
- Use Multiple DataSources with Spring Boot and RoutingDataSource
- Create a Login Application with Spring Boot, Spring Security, Spring JDBC
- Create a Login Application with Spring Boot, Spring Security, JPA
- Create a User Registration Application with Spring Boot, Spring Form Validation
- Example of OAuth2 Social Login in Spring Boot
- Run background scheduled tasks in Spring
- CRUD Restful Web Service Example with Spring Boot
- Spring Boot Restful Client with RestTemplate Example
- CRUD Example with Spring Boot, REST and AngularJS
- Secure Spring Boot RESTful Service using Basic Authentication
- Secure Spring Boot RESTful Service using Auth0 JWT
- Spring Boot File Upload Example
- Spring Boot File Download Example
- Spring Boot File Upload with jQuery Ajax Example
- Spring Boot File Upload with AngularJS Example
- Create a Shopping Cart Web Application with Spring Boot, Hibernate
- Spring Email Tutorial with Examples
- Create a simple Chat application with Spring Boot and Websocket
- Deploy Spring Boot Application on Tomcat Server
- Deploy Spring Boot Application on Oracle WebLogic Server
- Install a free Let's Encrypt SSL certificate for Spring Boot
- Configure Spring Boot to redirect HTTP to HTTPS
- Fetch data with Spring Data JPA DTO Projections
Show More