Spring Boot Interceptors Tutorial with Examples
1. What is Spring Interceptor?
When you come to a company and want to meet the company's manager. You need to pass through interceptors which can be a gatekeeper, a receptionist,...
In Spring, when a request is sent to spring controller, it will have to pass through Interceptors (0 or more) before being processed by Controller.
In Spring, when a request is sent to spring controller, it will have to pass through Interceptors (0 or more) before being processed by Controller.
Spring Interceptor is a concept that is rather similar to Servlet Filter.
Spring Interceptor is only applied to requests that are sending to a Controller.
Spring Interceptor is only applied to requests that are sending to a Controller.
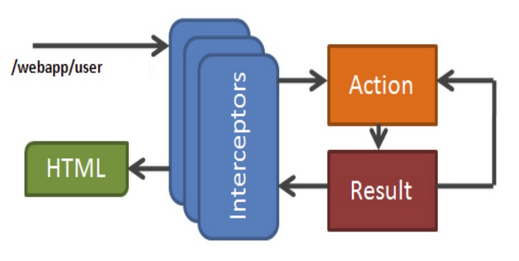
You can use Interceptor to do some tasks such as writing log, adding or updating configurations before request is processed by Controller,...
One of the Spring Boot MVCs using Interceptor as "Multilingual web application". You can see more about the application here:
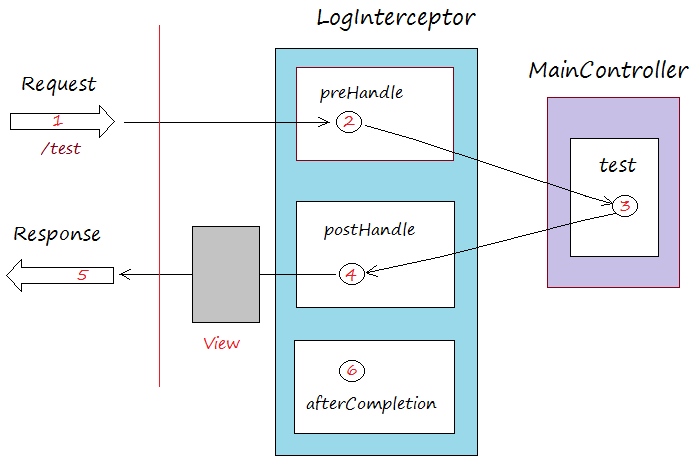
Your Interceptor must implement org.springframework.web.servlet.HandlerInterceptor interface or extended from org.springframework.web.servlet.handler.HandlerInterceptorAdapter class.
You need to implement three abstract methods:
public boolean preHandle(HttpServletRequest request,
HttpServletResponse response,
Object handler)
public void postHandle(HttpServletRequest request,
HttpServletResponse response,
Object handler,
ModelAndView modelAndView)
public void afterCompletion(HttpServletRequest request,
HttpServletResponse response,
Object handler,
Exception ex)
Note: The preHandle method returns true or false. If it returns true, it means request will continue to come to its destination (Is a Controller).
Each request may pass through a lot of Interceptors. The figure below illustrates that.
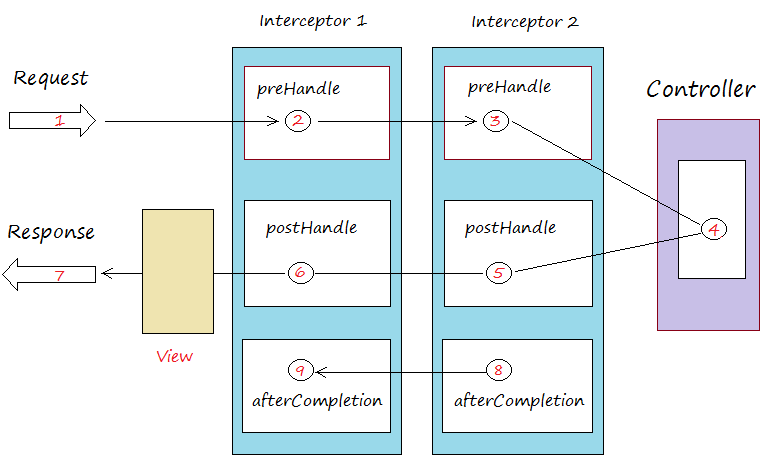
2. Create Spring Boot project
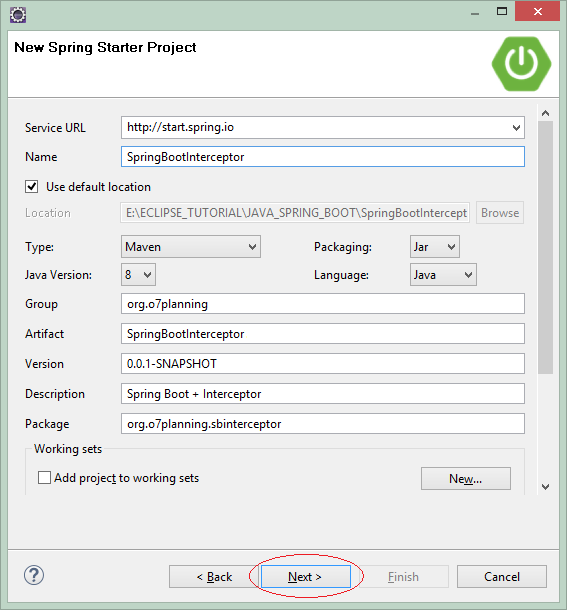
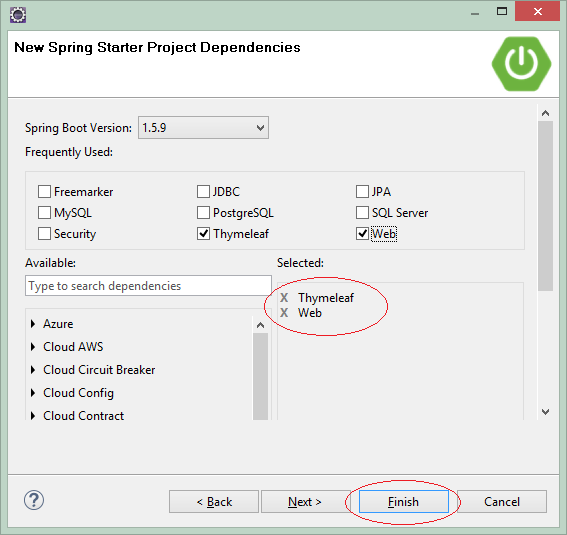
pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.o7planning</groupId>
<artifactId>SpringBootInterceptor</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>jar</packaging>
<name>SpringBootInterceptor</name>
<description>Spring Boot + Interceptor</description>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.0.0.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
SpringBootInterceptorApplication.java
package org.o7planning.sbinterceptor;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class SpringBootInterceptorApplication {
public static void main(String[] args) {
SpringApplication.run(SpringBootInterceptorApplication.class, args);
}
}
3. Interceptor classes
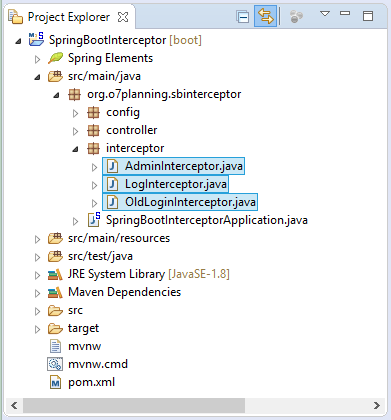
LogInterceptor is applied to all requests coming to a Controller. (See the configure in WebMvcConfig).
LogInterceptor.java
package org.o7planning.sbinterceptor.interceptor;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.springframework.web.servlet.ModelAndView;
import org.springframework.web.servlet.handler.HandlerInterceptorAdapter;
public class LogInterceptor extends HandlerInterceptorAdapter {
@Override
public boolean preHandle(HttpServletRequest request, HttpServletResponse response, Object handler)
throws Exception {
long startTime = System.currentTimeMillis();
System.out.println("\n-------- LogInterception.preHandle --- ");
System.out.println("Request URL: " + request.getRequestURL());
System.out.println("Start Time: " + System.currentTimeMillis());
request.setAttribute("startTime", startTime);
return true;
}
@Override
public void postHandle(HttpServletRequest request, HttpServletResponse response, //
Object handler, ModelAndView modelAndView) throws Exception {
System.out.println("\n-------- LogInterception.postHandle --- ");
System.out.println("Request URL: " + request.getRequestURL());
// You can add attributes in the modelAndView
// and use that in the view page
}
@Override
public void afterCompletion(HttpServletRequest request, HttpServletResponse response, //
Object handler, Exception ex) throws Exception {
System.out.println("\n-------- LogInterception.afterCompletion --- ");
long startTime = (Long) request.getAttribute("startTime");
long endTime = System.currentTimeMillis();
System.out.println("Request URL: " + request.getRequestURL());
System.out.println("End Time: " + endTime);
System.out.println("Time Taken: " + (endTime - startTime));
}
}
OldLoginInterceptor is an interceptor, if user enters the link "/admin/oldLogin", it will redirect to the new one "/admin/login".
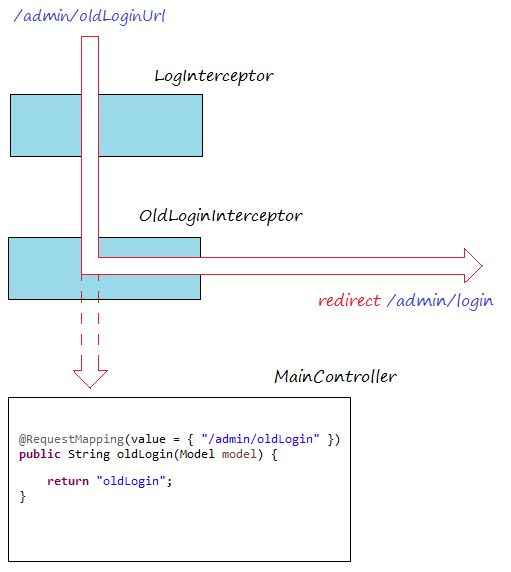
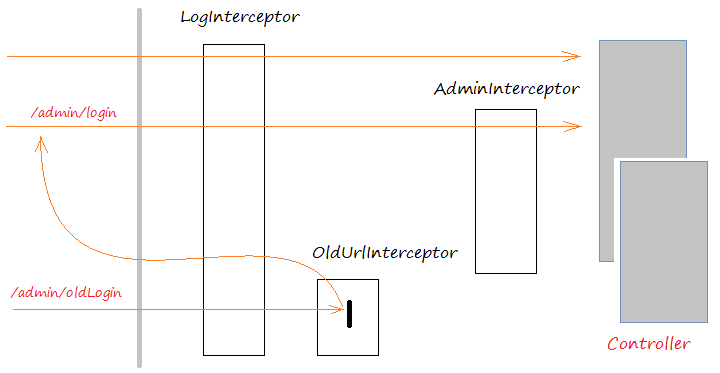
OldLoginInterceptor.java
package org.o7planning.sbinterceptor.interceptor;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.springframework.web.servlet.ModelAndView;
import org.springframework.web.servlet.handler.HandlerInterceptorAdapter;
public class OldLoginInterceptor extends HandlerInterceptorAdapter {
@Override
public boolean preHandle(HttpServletRequest request, HttpServletResponse response, Object handler)
throws Exception {
System.out.println("\n-------- OldLoginInterceptor.preHandle --- ");
System.out.println("Request URL: " + request.getRequestURL());
System.out.println("Sorry! This URL is no longer used, Redirect to /admin/login");
response.sendRedirect(request.getContextPath() + "/admin/login");
return false;
}
@Override
public void postHandle(HttpServletRequest request, HttpServletResponse response, //
Object handler, ModelAndView modelAndView) throws Exception {
// This code will never be run.
System.out.println("\n-------- OldLoginInterceptor.postHandle --- ");
}
@Override
public void afterCompletion(HttpServletRequest request, HttpServletResponse response, //
Object handler, Exception ex) throws Exception {
// This code will never be run.
System.out.println("\n-------- QueryStringInterceptor.afterCompletion --- ");
}
}
AdminInterceptor.java
package org.o7planning.sbinterceptor.interceptor;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.springframework.web.servlet.ModelAndView;
import org.springframework.web.servlet.handler.HandlerInterceptorAdapter;
public class AdminInterceptor extends HandlerInterceptorAdapter {
@Override
public boolean preHandle(HttpServletRequest request, HttpServletResponse response, Object handler)
throws Exception {
System.out.println("\n-------- AdminInterceptor.preHandle --- ");
return true;
}
@Override
public void postHandle(HttpServletRequest request, HttpServletResponse response, //
Object handler, ModelAndView modelAndView) throws Exception {
System.out.println("\n-------- AdminInterceptor.postHandle --- ");
}
@Override
public void afterCompletion(HttpServletRequest request, HttpServletResponse response, //
Object handler, Exception ex) throws Exception {
System.out.println("\n-------- AdminInterceptor.afterCompletion --- ");
}
}
4. Configure Interceptor
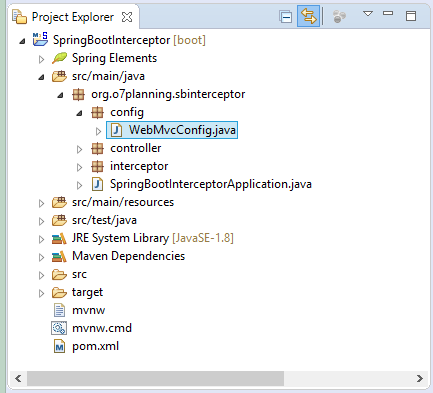
WebMvcConfig.java
package org.o7planning.sbinterceptor.config;
import org.o7planning.sbinterceptor.interceptor.AdminInterceptor;
import org.o7planning.sbinterceptor.interceptor.LogInterceptor;
import org.o7planning.sbinterceptor.interceptor.OldLoginInterceptor;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.config.annotation.InterceptorRegistry;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurerAdapter;
@Configuration
public class WebMvcConfig extends WebMvcConfigurerAdapter {
//
@Override
public void addInterceptors(InterceptorRegistry registry) {
// LogInterceptor apply to all URLs.
registry.addInterceptor(new LogInterceptor());
// Old Login url, no longer use.
// Use OldURLInterceptor to redirect to a new URL.
registry.addInterceptor(new OldLoginInterceptor())//
.addPathPatterns("/admin/oldLogin");
// This interceptor apply to URL like /admin/*
// Exclude /admin/oldLogin
registry.addInterceptor(new AdminInterceptor())//
.addPathPatterns("/admin/*")//
.excludePathPatterns("/admin/oldLogin");
}
}
5. Controllers & Views
MainController.java
package org.o7planning.sbinterceptor.controller;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
@Controller
public class MainController {
@RequestMapping(value = { "/", "/test" })
public String test(Model model) {
System.out.println("\n-------- MainController.test --- ");
System.out.println(" ** You are in Controller ** ");
return "test";
}
// This path is no longer used.
// It will be redirected by OldLoginInterceptor
@Deprecated
@RequestMapping(value = { "/admin/oldLogin" })
public String oldLogin(Model model) {
// Code here never run.
return "oldLogin";
}
@RequestMapping(value = { "/admin/login" })
public String login(Model model) {
System.out.println("\n-------- MainController.login --- ");
System.out.println(" ** You are in Controller ** ");
return "login";
}
}
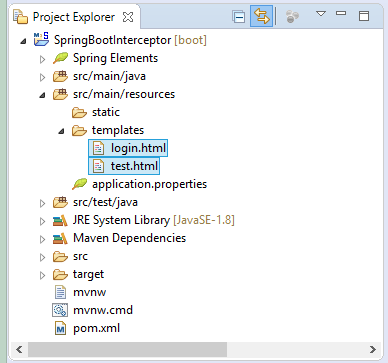
test.html
<!DOCTYPE HTML>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8" />
<title>Spring Boot Mvc Interceptor example</title>
</head>
<body>
<div style="border: 1px solid #ccc;padding: 5px;margin-bottom:10px;">
<a th:href="@{/}">Home</a>
|
<a th:href="@{/admin/oldLogin}">/admin/oldLogin (OLD URL)</a>
</div>
<h3>Spring Boot Mvc Interceptor</h3>
<span style="color:blue;">Testing LogInterceptor</span>
<br/><br/>
See Log in Console..
</body>
</html>
login.html
<!DOCTYPE HTML>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8" />
<title>Spring Boot Mvc Interceptor example</title>
</head>
<body>
<div style="border: 1px solid #ccc;padding: 5px;margin-bottom:10px;">
<a th:href="@{/}">Home</a>
|
<a th:href="@{/admin/oldLogin}">/admin/oldLogin (OLD URL)</a>
</div>
<h3>This is Login Page</h3>
<span style="color:blue">Testing OldLoginInterceptor & AdminInterceptor</span>
<br/><br/>
See more info in the Console.
</body>
</html>
6. Running Apps
Test the case when a user visits a page, LogInterceptor logs the relevant information (page address, access time), and calculates the time that the Web Server served the request.
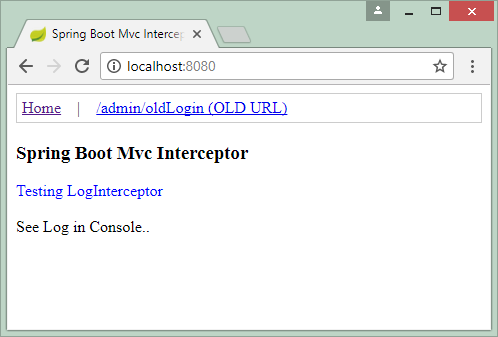
-------- LogInterception.preHandle ---
Request URL: http://localhost:8080/
Start Time: 1512231713663
-------- MainController.test ---
** You are in Controller **
-------- LogInterception.postHandle ---
Request URL: http://localhost:8080/
-------- LogInterception.afterCompletion ---
Request URL: http://localhost:8080/
End Time: 1512231713665
Time Taken: 2
Test case when a user visits an old login page (No longer used), the OldLoginInterceptor redirects the request to the new login page.
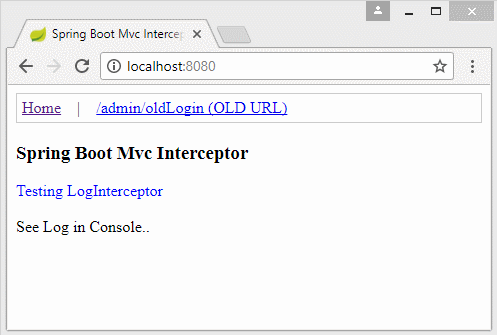
-------- LogInterception.preHandle ---
Request URL: http://localhost:8080/admin/oldLogin
Start Time: 1512231812219
-------- OldLoginInterceptor.preHandle ---
Request URL: http://localhost:8080/admin/oldLogin
Sorry! This URL is no longer used, Redirect to /admin/login
-------- LogInterception.afterCompletion ---
Request URL: http://localhost:8080/admin/oldLogin
End Time: 1512231812219
Time Taken: 1
-------- LogInterception.preHandle ---
Request URL: http://localhost:8080/admin/login
Start Time: 1512231812222
-------- AdminInterceptor.preHandle ---
-------- MainController.login ---
** You are in Controller **
-------- AdminInterceptor.postHandle ---
-------- LogInterception.postHandle ---
Request URL: http://localhost:8080/admin/login
-------- AdminInterceptor.afterCompletion ---
-------- LogInterception.afterCompletion ---
Request URL: http://localhost:8080/admin/login
End Time: 1512231812227
Time Taken: 5
Spring Boot Tutorials
- Install Spring Tool Suite for Eclipse
- Spring Tutorial for Beginners
- Spring Boot Tutorial for Beginners
- Spring Boot Common Properties
- Spring Boot and Thymeleaf Tutorial with Examples
- Spring Boot and FreeMarker Tutorial with Examples
- Spring Boot and Groovy Tutorial with Examples
- Spring Boot and Mustache Tutorial with Examples
- Spring Boot and JSP Tutorial with Examples
- Spring Boot, Apache Tiles, JSP Tutorial with Examples
- Use Logging in Spring Boot
- Application Monitoring with Spring Boot Actuator
- Create a Multi Language web application with Spring Boot
- Use multiple ViewResolvers in Spring Boot
- Use Twitter Bootstrap in Spring Boot
- Spring Boot Interceptors Tutorial with Examples
- Spring Boot, Spring JDBC and Spring Transaction Tutorial with Examples
- Spring JDBC Tutorial with Examples
- Spring Boot, JPA and Spring Transaction Tutorial with Examples
- Spring Boot and Spring Data JPA Tutorial with Examples
- Spring Boot, Hibernate and Spring Transaction Tutorial with Examples
- Integrating Spring Boot, JPA and H2 Database
- Spring Boot and MongoDB Tutorial with Examples
- Use Multiple DataSources with Spring Boot and JPA
- Use Multiple DataSources with Spring Boot and RoutingDataSource
- Create a Login Application with Spring Boot, Spring Security, Spring JDBC
- Create a Login Application with Spring Boot, Spring Security, JPA
- Create a User Registration Application with Spring Boot, Spring Form Validation
- Example of OAuth2 Social Login in Spring Boot
- Run background scheduled tasks in Spring
- CRUD Restful Web Service Example with Spring Boot
- Spring Boot Restful Client with RestTemplate Example
- CRUD Example with Spring Boot, REST and AngularJS
- Secure Spring Boot RESTful Service using Basic Authentication
- Secure Spring Boot RESTful Service using Auth0 JWT
- Spring Boot File Upload Example
- Spring Boot File Download Example
- Spring Boot File Upload with jQuery Ajax Example
- Spring Boot File Upload with AngularJS Example
- Create a Shopping Cart Web Application with Spring Boot, Hibernate
- Spring Email Tutorial with Examples
- Create a simple Chat application with Spring Boot and Websocket
- Deploy Spring Boot Application on Tomcat Server
- Deploy Spring Boot Application on Oracle WebLogic Server
- Install a free Let's Encrypt SSL certificate for Spring Boot
- Configure Spring Boot to redirect HTTP to HTTPS
- Fetch data with Spring Data JPA DTO Projections
Show More