Spring Email Tutorial with Examples
1. Overview of Spring Mail
Spring Framework provides you with a API to send an email. It includes some interfaces and classes, all of which are located in the two packages such as org.springframework.mail & org.springframework.mail.javamail.
To use the Spring Mail in the Spring Boot application, let's add the following dependencies to the pom.xml:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-mail</artifactId>
</dependency>
pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0
http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.o7planning</groupId>
<artifactId>SpringBootMail</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>jar</packaging>
<name>SpringBootMail</name>
<description>Spring Boot + Mail</description>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.0.0.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-mail</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
The Spring Mail also has many classes or interfaces. Basically, below is the list of main classes and interfaces:
- org.springframework.mail.MailSender
- org.springframework.mail.SimpleMailMessage
- org.springframework.mail.MailMessage
- org.springframework.mail.javamail.JavaMailSender
- org.springframework.mail.javamail.JavaMailSenderImpl
- org.springframework.mail.javamail.MimeMessagePreparator
- org.springframework.mail.javamail.MimeMessageHelper
- org.springframework.mail.javamail.MimeMailMessage
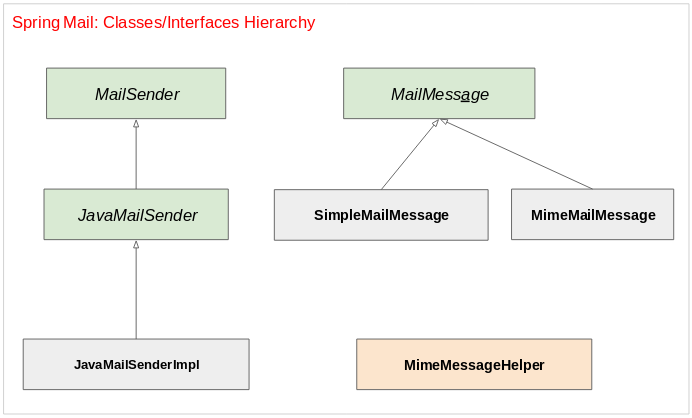
MIME (Multi-Purpose Internet Mail Extensions):MIME (Multi-Purpose Internet Mail Extensions): an extension of initial Internet Email. It allows sending the emails attached with different kinds of data in the Internet such as audio, video, image,.., and supports emails in HTML format.
Class/Interface | Description |
MailSender | This is a top-level interface, which provides functions to send a simple email. |
JavaMailSender | This is the subinterface of MailSender, which supports MIME -typed messages, It is usualy used with MimeMessageHelper class to create MimeMessage. An advice is to use the MimeMessagePreparator interface with this interface. |
JavaMailSenderImpl | means a class implementing the JavaMailSender interface. It supports to send MimeMessage and SimpleMailMessage messages. |
MailMessage | Means an interface representing for a simple message. It includes basic information of an email such as sender, recipient, subject and content of message. |
SimpleMailMessage | This is a class implementing the MailMessage interface, used to create a simple message. |
MimeMailMessage | This is a class implementing the MailMessage interface, used to create a message supporting MIME. |
MimeMessagePreparator | This interface provides callback method called when preparing a MIME message. |
MimeMessageHelper | means a supporting class to create a MIME message. It supports image, and attached files and creates HTML-typed messages. |
2. Note for Gmail
In this lesson, I am going to use a Gmail account to send a message because Gmail is a common Mail-Server . However, for your Gmail account to be able to send an email via a Java application, you have to tell the Google to allow it. OK, first of all, on the browser, you log in your Gmail account and then visit the following address:
Activate to allow your Gmail account to be used by Less Secure Apps.
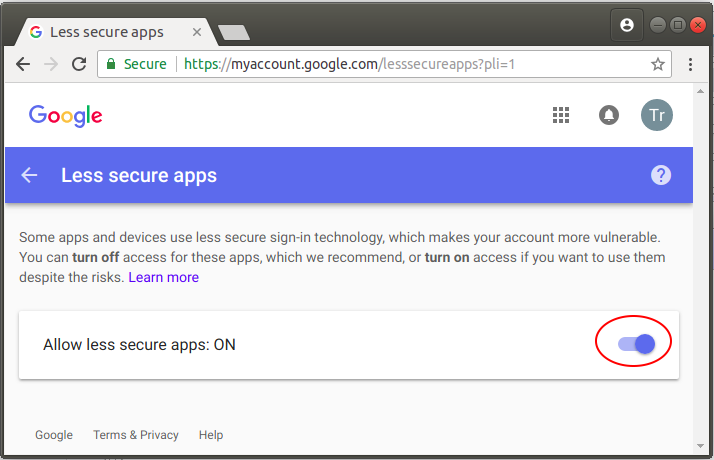
3. Configure Spring Mail
In the Spring application, you should create a Spring-Bean for MailSender. For example, below, I use a Gmail account to send anemail.
MailConfig.java
package org.o7planning.sbmail.config;
import java.util.Properties;
import org.o7planning.sbmail.MyConstants;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.mail.javamail.JavaMailSender;
import org.springframework.mail.javamail.JavaMailSenderImpl;
@Configuration
public class MailConfig {
@Bean
public JavaMailSender getJavaMailSender() {
JavaMailSenderImpl mailSender = new JavaMailSenderImpl();
mailSender.setHost("smtp.gmail.com");
mailSender.setPort(587);
mailSender.setUsername(MyConstants.MY_EMAIL);
mailSender.setPassword(MyConstants.MY_PASSWORD);
Properties props = mailSender.getJavaMailProperties();
props.put("mail.transport.protocol", "smtp");
props.put("mail.smtp.auth", "true");
props.put("mail.smtp.starttls.enable", "true");
props.put("mail.debug", "true");
return mailSender;
}
}
MyConstants.java
package org.o7planning.sbmail;
public class MyConstants {
// Replace with your email here:
public static final String MY_EMAIL = "yourEmail@gmail.com";
// Replace password!!
public static final String MY_PASSWORD = "yourPassword";
// And receiver!
public static final String FRIEND_EMAIL = "yourFriend@gmail.com";
}
4. Example of sending a simple Email
This is a very simple example, sending an email with ordinary text content.
SimpleEmailExampleController.java
package org.o7planning.sbmail.controller;
import org.o7planning.sbmail.MyConstants;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.mail.SimpleMailMessage;
import org.springframework.mail.javamail.JavaMailSender;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.ResponseBody;
@Controller
public class SimpleEmailExampleController {
@Autowired
public JavaMailSender emailSender;
@ResponseBody
@RequestMapping("/sendSimpleEmail")
public String sendSimpleEmail() {
// Create a Simple MailMessage.
SimpleMailMessage message = new SimpleMailMessage();
message.setTo(MyConstants.FRIEND_EMAIL);
message.setSubject("Test Simple Email");
message.setText("Hello, Im testing Simple Email");
// Send Message!
this.emailSender.send(message);
return "Email Sent!";
}
}
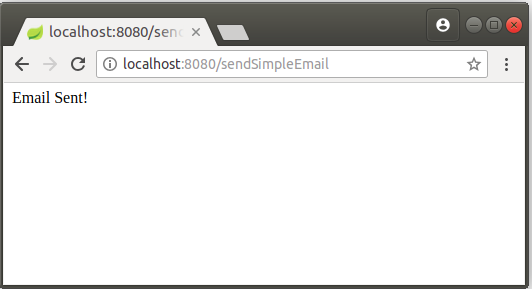
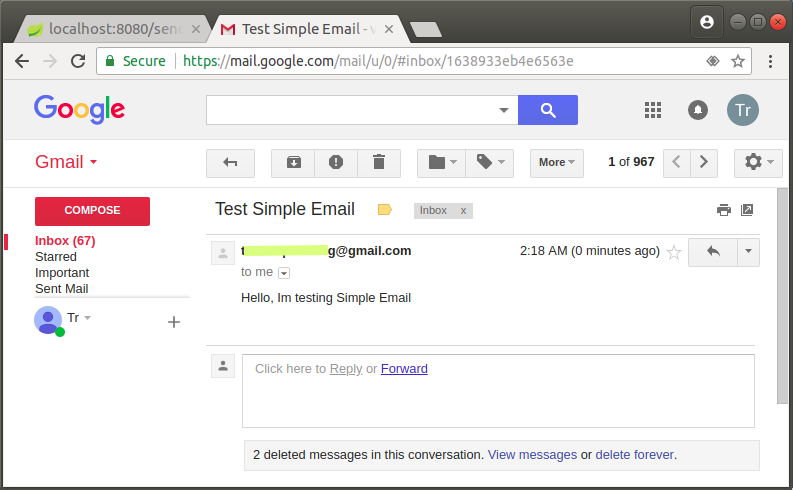
5. Send an Email attached with files
AttachmentEmailExampleController.java
package org.o7planning.sbmail.controller;
import java.io.File;
import javax.mail.MessagingException;
import javax.mail.internet.MimeMessage;
import org.o7planning.sbmail.MyConstants;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.core.io.FileSystemResource;
import org.springframework.mail.javamail.JavaMailSender;
import org.springframework.mail.javamail.MimeMessageHelper;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.ResponseBody;
@Controller
public class AttachmentEmailExampleController {
@Autowired
public JavaMailSender emailSender;
@ResponseBody
@RequestMapping("/sendAttachmentEmail")
public String sendAttachmentEmail() throws MessagingException {
MimeMessage message = emailSender.createMimeMessage();
boolean multipart = true;
MimeMessageHelper helper = new MimeMessageHelper(message, multipart);
helper.setTo(MyConstants.FRIEND_EMAIL);
helper.setSubject("Test email with attachments");
helper.setText("Hello, Im testing email with attachments!");
String path1 = "/home/tran/Downloads/test.txt";
String path2 = "/home/tran/Downloads/readme.zip";
// Attachment 1
FileSystemResource file1 = new FileSystemResource(new File(path1));
helper.addAttachment("Txt file", file1);
// Attachment 2
FileSystemResource file2 = new FileSystemResource(new File(path2));
helper.addAttachment("Readme", file2);
emailSender.send(message);
return "Email Sent!";
}
}
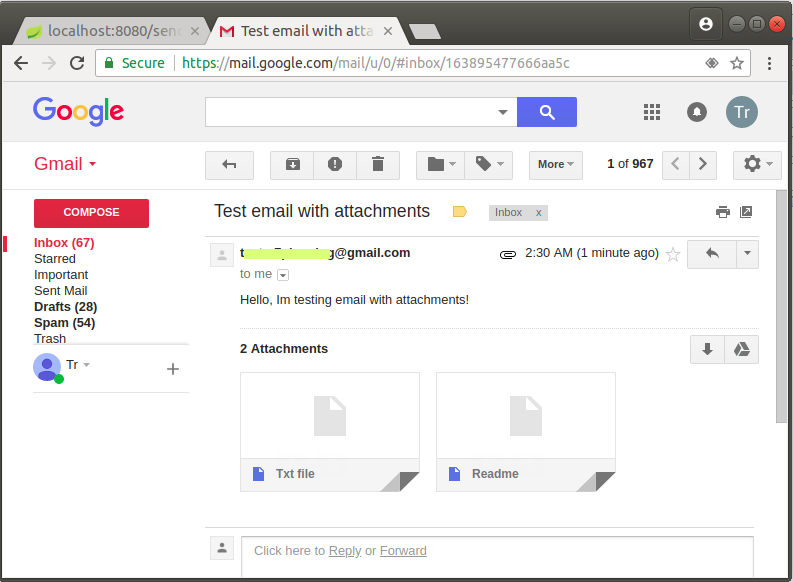
6. Send an email in HTML format
HtmlEmailExampleController.java
package org.o7planning.sbmail.controller;
import javax.mail.MessagingException;
import javax.mail.internet.MimeMessage;
import org.o7planning.sbmail.MyConstants;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.mail.javamail.JavaMailSender;
import org.springframework.mail.javamail.MimeMessageHelper;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.ResponseBody;
@Controller
public class HtmlEmailExampleController {
@Autowired
public JavaMailSender emailSender;
@ResponseBody
@RequestMapping("/sendHtmlEmail")
public String sendHtmlEmail() throws MessagingException {
MimeMessage message = emailSender.createMimeMessage();
boolean multipart = true;
MimeMessageHelper helper = new MimeMessageHelper(message, multipart, "utf-8");
String htmlMsg = "<h3>Im testing send a HTML email</h3>"
+"<img src='http://www.apache.org/images/asf_logo_wide.gif'>";
message.setContent(htmlMsg, "text/html");
helper.setTo(MyConstants.FRIEND_EMAIL);
helper.setSubject("Test send HTML email");
this.emailSender.send(message);
return "Email Sent!";
}
}
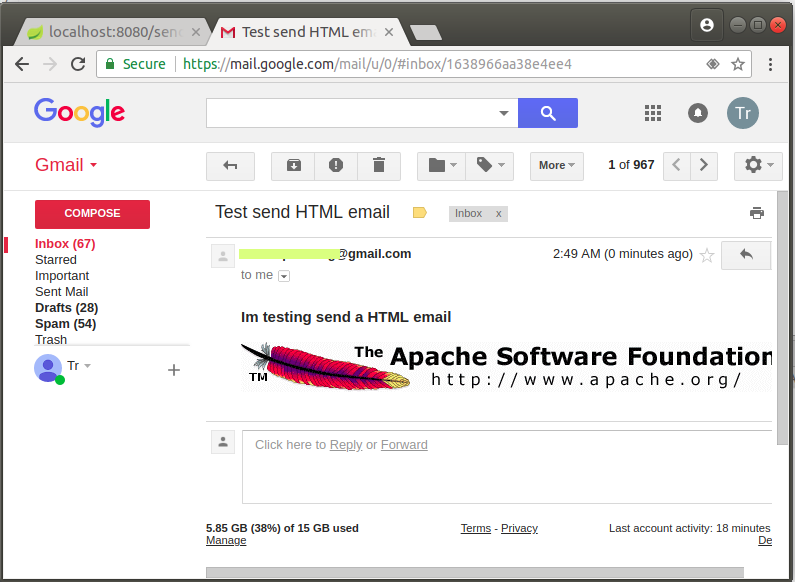
Spring Boot Tutorials
- Fetch data with Spring Data JPA DTO Projections
- Install Spring Tool Suite for Eclipse
- Spring Tutorial for Beginners
- Spring Boot Tutorial for Beginners
- Spring Boot Common Properties
- Spring Boot and Thymeleaf Tutorial with Examples
- Spring Boot and FreeMarker Tutorial with Examples
- Spring Boot and Groovy Tutorial with Examples
- Spring Boot and Mustache Tutorial with Examples
- Spring Boot and JSP Tutorial with Examples
- Spring Boot, Apache Tiles, JSP Tutorial with Examples
- Use Logging in Spring Boot
- Application Monitoring with Spring Boot Actuator
- Create a Multi Language web application with Spring Boot
- Use multiple ViewResolvers in Spring Boot
- Use Twitter Bootstrap in Spring Boot
- Spring Boot Interceptors Tutorial with Examples
- Spring Boot, Spring JDBC and Spring Transaction Tutorial with Examples
- Spring JDBC Tutorial with Examples
- Spring Boot, JPA and Spring Transaction Tutorial with Examples
- Spring Boot and Spring Data JPA Tutorial with Examples
- Spring Boot, Hibernate and Spring Transaction Tutorial with Examples
- Integrating Spring Boot, JPA and H2 Database
- Spring Boot and MongoDB Tutorial with Examples
- Use Multiple DataSources with Spring Boot and JPA
- Use Multiple DataSources with Spring Boot and RoutingDataSource
- Create a Login Application with Spring Boot, Spring Security, Spring JDBC
- Create a Login Application with Spring Boot, Spring Security, JPA
- Create a User Registration Application with Spring Boot, Spring Form Validation
- Example of OAuth2 Social Login in Spring Boot
- Run background scheduled tasks in Spring
- CRUD Restful Web Service Example with Spring Boot
- Spring Boot Restful Client with RestTemplate Example
- CRUD Example with Spring Boot, REST and AngularJS
- Secure Spring Boot RESTful Service using Basic Authentication
- Secure Spring Boot RESTful Service using Auth0 JWT
- Spring Boot File Upload Example
- Spring Boot File Download Example
- Spring Boot File Upload with jQuery Ajax Example
- Spring Boot File Upload with AngularJS Example
- Create a Shopping Cart Web Application with Spring Boot, Hibernate
- Spring Email Tutorial with Examples
- Create a simple Chat application with Spring Boot and Websocket
- Deploy Spring Boot Application on Tomcat Server
- Deploy Spring Boot Application on Oracle WebLogic Server
- Install a free Let's Encrypt SSL certificate for Spring Boot
- Configure Spring Boot to redirect HTTP to HTTPS
Show More