Use Twitter Bootstrap in Spring Boot
1. Objective of post
Before starting the lesson, let's take a few minutes to understand Bootstrap:
The Bootstrap is a HTML, CSS & JavaScript Framework allowing designing and developing the "Responsive Web Mobile" applications. The Bootstrap includes HTML templates, CSS templates & Javascript and basis and available things such as: typography, forms, buttons, tables, navigation, modals, image carousels and others. The Bootstrap also has Javascript Plugins, which helps the designing of your Web Reponsive to be more easier and quicker.
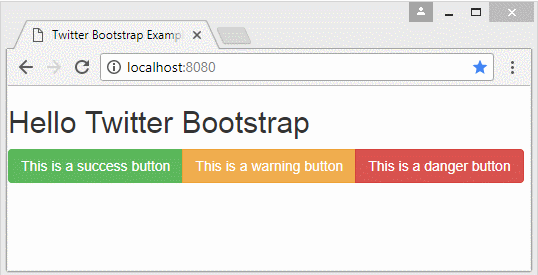
The Bootstrap is developed by the Mark Otto and Jacob Thornton at Twitter. It was published as an open resource code in August 2011 on GitHub.
See more:
In this post, I am going to show you how to create a Spring Boot application using the Bootstrap. And because the Bootstrap is a Html, Css, Javascript Framework , you use any technology for the View layer (JSP, Thymeleaf, Freemarker, ...).
The contents to be mentioned in this lesson:
- Create a Spring Boot application.
- Declare necessary libraries so that you can use the Bootstrap.
- Create a simple page using UI Components provided by the Bootstrap.
3. Declare pom.xml
The Bootstrap has been packaged into a small, beautiful Jar file. To use it, you only need to declare it in the pom.xml, and so you are ready to work with the Bootstrap Framework.
** Bootstrap **
<!-- https://mvnrepository.com/artifact/org.webjars/jquery -->
<dependency>
<groupId>org.webjars</groupId>
<artifactId>jquery</artifactId>
<version>3.3.1-1</version>
</dependency>
<!-- https://mvnrepository.com/artifact/org.webjars/popper.js -->
<dependency>
<groupId>org.webjars</groupId>
<artifactId>popper.js</artifactId>
<version>1.14.1</version>
</dependency>
<!-- https://mvnrepository.com/artifact/org.webjars/bootstrap -->
<dependency>
<groupId>org.webjars</groupId>
<artifactId>bootstrap</artifactId>
<version>4.1.1</version>
</dependency>
The full contents of the pom.xml file:
pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0
http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.o7planning</groupId>
<artifactId>SpringBootBootstrap</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>jar</packaging>
<name>SpringBootBootstrap</name>
<description>Spring Boot + Bootstrap</description>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.0.0.RELEASE</version>
<relativePath /> <!-- lookup parent from repository -->
</parent>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<!-- https://mvnrepository.com/artifact/org.webjars/jquery -->
<dependency>
<groupId>org.webjars</groupId>
<artifactId>jquery</artifactId>
<version>3.3.1-1</version>
</dependency>
<!-- https://mvnrepository.com/artifact/org.webjars/popper.js -->
<dependency>
<groupId>org.webjars</groupId>
<artifactId>popper.js</artifactId>
<version>1.14.1</version>
</dependency>
<!-- https://mvnrepository.com/artifact/org.webjars/bootstrap -->
<dependency>
<groupId>org.webjars</groupId>
<artifactId>bootstrap</artifactId>
<version>4.1.1</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
4. Resource Handler
The data resources of the Bootstrap are packaged in the Jar file. You need to expose them so that they can be accessed by paths, for example:
- http://somedomain/SomeContextPath/jquery/jquery.min.css
- http://somedomain/SomeContextPath/popper/popper.min.js
- http://somedomain/SomeContextPath/bootstrap/css/bootstrap.min.css
- http://somedomain/SomeContextPath/bootstrap/js/bootstrap.min.js
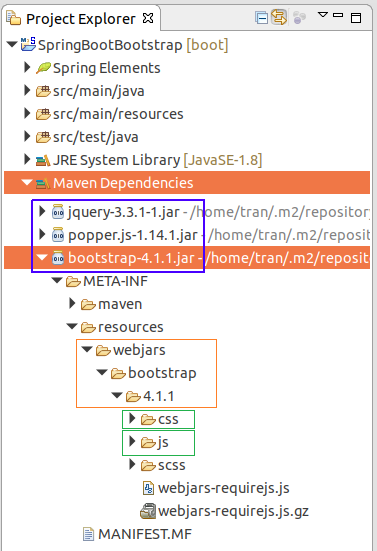
WebMvcConfig.java
package org.o7planning.sbbootstrap.config;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.config.annotation.ResourceHandlerRegistry;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurer;
@Configuration
public class WebMvcConfig implements WebMvcConfigurer {
@Override
public void addResourceHandlers(ResourceHandlerRegistry registry) {
//
// Access Bootstrap static resource:
//
//
// http://somedomain/SomeContextPath/jquery/jquery.min.css
//
registry.addResourceHandler("/jquery/**") //
.addResourceLocations("classpath:/META-INF/resources/webjars/jquery/3.3.1-1/");
//
// http://somedomain/SomeContextPath/popper/popper.min.js
//
registry.addResourceHandler("/popper/**") //
.addResourceLocations("classpath:/META-INF/resources/webjars/popper.js/1.14.1/umd/");
// http://somedomain/SomeContextPath/bootstrap/css/bootstrap.min.css
// http://somedomain/SomeContextPath/bootstrap/js/bootstrap.min.js
//
registry.addResourceHandler("/bootstrap/**") //
.addResourceLocations("classpath:/META-INF/resources/webjars/bootstrap/4.1.1/");
}
}
5. Controller
HelloWorldController.java
package org.o7planning.sbbootstrap.controller;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
@Controller
public class HelloWorldController {
@RequestMapping("/")
public String helloWorld(Model model) {
return "index";
}
}
6. Views
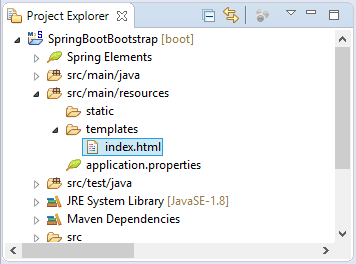
index.html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8"/>
<title>Twitter Bootstrap Example</title>
<meta name="viewport" content="width=device-width, initial-scale=1.0"/>
<!-- See configuration in WebMvConfig.java -->
<link th:href="@{/bootstrap/css/bootstrap.min.css}"
rel="stylesheet" media="screen"/>
<script th:src="@{/jquery/jquery.min.js}"></script>
<script th:src="@{/popper/popper.min.js}"></script>
<script th:src="@{/bootstrap/js/bootstrap.min.js}"></script>
</head>
<body>
<h2>Hello Twitter Bootstrap</h2>
<div class="btn-group">
<button type="button" class="btn btn-success">This is a success button</button>
<button type="button" class="btn btn-warning">This is a warning button</button>
<button type="button" class="btn btn-danger">This is a danger button</button>
</div>
</body>
</html>
And this is the image of website with the UI Components of the Bootstrap:
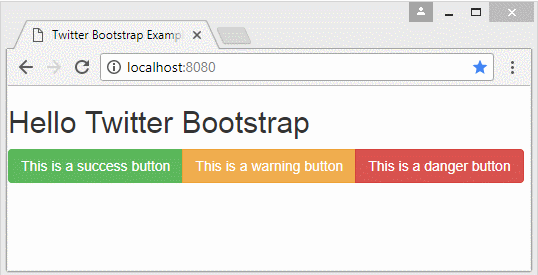
Spring Boot Tutorials
- Install Spring Tool Suite for Eclipse
- Spring Tutorial for Beginners
- Spring Boot Tutorial for Beginners
- Spring Boot Common Properties
- Spring Boot and Thymeleaf Tutorial with Examples
- Spring Boot and FreeMarker Tutorial with Examples
- Spring Boot and Groovy Tutorial with Examples
- Spring Boot and Mustache Tutorial with Examples
- Spring Boot and JSP Tutorial with Examples
- Spring Boot, Apache Tiles, JSP Tutorial with Examples
- Use Logging in Spring Boot
- Application Monitoring with Spring Boot Actuator
- Create a Multi Language web application with Spring Boot
- Use multiple ViewResolvers in Spring Boot
- Use Twitter Bootstrap in Spring Boot
- Spring Boot Interceptors Tutorial with Examples
- Spring Boot, Spring JDBC and Spring Transaction Tutorial with Examples
- Spring JDBC Tutorial with Examples
- Spring Boot, JPA and Spring Transaction Tutorial with Examples
- Spring Boot and Spring Data JPA Tutorial with Examples
- Spring Boot, Hibernate and Spring Transaction Tutorial with Examples
- Integrating Spring Boot, JPA and H2 Database
- Spring Boot and MongoDB Tutorial with Examples
- Use Multiple DataSources with Spring Boot and JPA
- Use Multiple DataSources with Spring Boot and RoutingDataSource
- Create a Login Application with Spring Boot, Spring Security, Spring JDBC
- Create a Login Application with Spring Boot, Spring Security, JPA
- Create a User Registration Application with Spring Boot, Spring Form Validation
- Example of OAuth2 Social Login in Spring Boot
- Run background scheduled tasks in Spring
- CRUD Restful Web Service Example with Spring Boot
- Spring Boot Restful Client with RestTemplate Example
- CRUD Example with Spring Boot, REST and AngularJS
- Secure Spring Boot RESTful Service using Basic Authentication
- Secure Spring Boot RESTful Service using Auth0 JWT
- Spring Boot File Upload Example
- Spring Boot File Download Example
- Spring Boot File Upload with jQuery Ajax Example
- Spring Boot File Upload with AngularJS Example
- Create a Shopping Cart Web Application with Spring Boot, Hibernate
- Spring Email Tutorial with Examples
- Create a simple Chat application with Spring Boot and Websocket
- Deploy Spring Boot Application on Tomcat Server
- Deploy Spring Boot Application on Oracle WebLogic Server
- Install a free Let's Encrypt SSL certificate for Spring Boot
- Configure Spring Boot to redirect HTTP to HTTPS
- Fetch data with Spring Data JPA DTO Projections
Show More