Spring MVC Security with Hibernate Tutorial with Examples
1. Introduction
The document is based on:
- Eclipse 4.6 (NEON)
- Spring MVC 4.x
- Spring Security 4.x
- Hibernate 4.x
- (Annotation Config)
3. Preparing the database
ORACLE:
-- Create table
create table USERS
(
USERNAME VARCHAR2(36) not null,
PASSWORD VARCHAR2(36) not null,
ENABLED NUMBER(1) not null
) ;
alter table USERS
add constraint USER_PK primary key (USERNAME);
---------------------
-- Create table
create table USER_ROLES
(
ROLE_ID VARCHAR2(50) not null,
USERNAME VARCHAR2(36) not null,
USER_ROLE VARCHAR2(30) not null
) ;
alter table USER_ROLES
add constraint USER_ROLE_PK primary key (ROLE_ID);
alter table USER_ROLES
add constraint USER_ROLE_UK unique (USERNAME, USER_ROLE);
-------------------------------
insert into users (USERNAME, PASSWORD, ENABLED)
values ('dbuser1', '12345', 1);
insert into users (USERNAME, PASSWORD, ENABLED)
values ('dbadmin1', '12345', 1);
insert into User_Roles (ROLE_ID, USERNAME, USER_ROLE)
values ('1', 'dbuser1', 'USER');
insert into User_Roles (ROLE_ID, USERNAME, USER_ROLE)
values ('2', 'dbadmin1', 'ADMIN');
insert into User_Roles (ROLE_ID, USERNAME, USER_ROLE)
values ('3', 'dbadmin1', 'USER');
-------------------------------
Commit;
MySQL & SQL Server:
-- Create table
create table USERS
(
USERNAME VARCHAR(36) not null,
PASSWORD VARCHAR(36) not null,
ENABLED smallint not null
) ;
alter table USERS
add constraint USER_PK primary key (USERNAME);
---------------------
-- Create table
create table USER_ROLES
(
ROLE_ID VARCHAR(50) not null,
USERNAME VARCHAR(36) not null,
USER_ROLE VARCHAR(30) not null
) ;
alter table USER_ROLES
add constraint USER_ROLE_PK primary key (ROLE_ID);
alter table USER_ROLES
add constraint USER_ROLE_UK unique (USERNAME, USER_ROLE);
-------------------------------
insert into users (USERNAME, PASSWORD, ENABLED)
values ('dbuser1', '12345', 1);
insert into users (USERNAME, PASSWORD, ENABLED)
values ('dbadmin1', '12345', 1);
insert into User_Roles (ROLE_ID, USERNAME, USER_ROLE)
values ('1', 'dbuser1', 'USER');
insert into User_Roles (ROLE_ID, USERNAME, USER_ROLE)
values ('2', 'dbadmin1', 'ADMIN');
insert into User_Roles (ROLE_ID, USERNAME, USER_ROLE)
values ('3', 'dbadmin1', 'USER');
5. Configuring Maven & web.xml
Configure to use Servlet 3.x:
web.xml
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns="http://java.sun.com/xml/ns/javaee"
xsi:schemaLocation="http://java.sun.com/xml/ns/javaee
http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd"
id="WebApp_ID" version="3.0">
<display-name>Spring MVC Security</display-name>
</web-app>
Maven:
pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0
http://maven.apache.org/maven-v4_0_0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.o7planning</groupId>
<artifactId>SpringMVCHibernateSecurity</artifactId>
<packaging>war</packaging>
<version>0.0.1-SNAPSHOT</version>
<name>SpringMVCHibernateSecurity Maven Webapp</name>
<url>http://maven.apache.org</url>
<properties>
<java-version>1.7</java-version>
</properties>
<repositories>
<!-- Repository for ORACLE JDBC Driver -->
<repository>
<id>codelds</id>
<url>https://code.lds.org/nexus/content/groups/main-repo</url>
</repository>
</repositories>
<dependencies>
<!-- Servlet API -->
<!-- http://mvnrepository.com/artifact/javax.servlet/javax.servlet-api -->
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
<version>3.1.0</version>
<scope>provided</scope>
</dependency>
<!-- Jstl for jsp page -->
<!-- http://mvnrepository.com/artifact/javax.servlet/jstl -->
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>jstl</artifactId>
<version>1.2</version>
</dependency>
<!-- JSP API -->
<!-- http://mvnrepository.com/artifact/javax.servlet.jsp/jsp-api -->
<dependency>
<groupId>javax.servlet.jsp</groupId>
<artifactId>jsp-api</artifactId>
<version>2.2</version>
<scope>provided</scope>
</dependency>
<!-- Spring dependencies -->
<!-- http://mvnrepository.com/artifact/org.springframework/spring-core -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-core</artifactId>
<version>4.1.4.RELEASE</version>
</dependency>
<!-- http://mvnrepository.com/artifact/org.springframework/spring-web -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-web</artifactId>
<version>4.1.4.RELEASE</version>
</dependency>
<!-- http://mvnrepository.com/artifact/org.springframework/spring-webmvc -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>4.1.4.RELEASE</version>
</dependency>
<!-- http://mvnrepository.com/artifact/org.springframework/spring-orm -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-orm</artifactId>
<version>4.1.4.RELEASE</version>
</dependency>
<!-- Spring Security Artifacts - START -->
<!-- Spring Security Artifacts - START -->
<!-- http://mvnrepository.com/artifact/org.springframework.security/spring-security-web -->
<dependency>
<groupId>org.springframework.security</groupId>
<artifactId>spring-security-web</artifactId>
<version>4.0.4.RELEASE</version>
</dependency>
<!-- http://mvnrepository.com/artifact/org.springframework.security/spring-security-config -->
<dependency>
<groupId>org.springframework.security</groupId>
<artifactId>spring-security-config</artifactId>
<version>4.0.4.RELEASE</version>
</dependency>
<!-- http://mvnrepository.com/artifact/org.springframework.security/spring-security-taglibs -->
<dependency>
<groupId>org.springframework.security</groupId>
<artifactId>spring-security-taglibs</artifactId>
<version>4.0.4.RELEASE</version>
</dependency>
<!-- Hibernate -->
<!-- http://mvnrepository.com/artifact/org.hibernate/hibernate-core -->
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>hibernate-core</artifactId>
<version>4.3.8.Final</version>
</dependency>
<!-- http://mvnrepository.com/artifact/org.hibernate/hibernate-entitymanager -->
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>hibernate-entitymanager</artifactId>
<version>4.3.8.Final</version>
</dependency>
<!-- http://mvnrepository.com/artifact/org.hibernate/hibernate-c3p0 -->
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>hibernate-c3p0</artifactId>
<version>4.3.8.Final</version>
</dependency>
<!-- Commons DataSources -->
<!-- https://mvnrepository.com/artifact/commons-dbcp/commons-dbcp -->
<dependency>
<groupId>commons-dbcp</groupId>
<artifactId>commons-dbcp</artifactId>
<version>1.4</version>
</dependency>
<!-- MySQL JDBC driver -->
<!-- http://mvnrepository.com/artifact/mysql/mysql-connector-java -->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>5.1.34</version>
</dependency>
<!-- Oracle JDBC driver -->
<dependency>
<groupId>com.oracle</groupId>
<artifactId>ojdbc6</artifactId>
<version>11.2.0.3</version>
</dependency>
<!-- SQLServer JDBC driver (JTDS) -->
<!-- http://mvnrepository.com/artifact/net.sourceforge.jtds/jtds -->
<dependency>
<groupId>net.sourceforge.jtds</groupId>
<artifactId>jtds</artifactId>
<version>1.3.1</version>
</dependency>
</dependencies>
<build>
<finalName>SpringMVCHibernateSecurity</finalName>
<plugins>
<!-- Config: Maven Tomcat Plugin -->
<!-- http://mvnrepository.com/artifact/org.apache.tomcat.maven/tomcat7-maven-plugin -->
<plugin>
<groupId>org.apache.tomcat.maven</groupId>
<artifactId>tomcat7-maven-plugin</artifactId>
<version>2.2</version>
<!-- Config: contextPath and Port (Default - /SpringMVCHibernateSecurity : 8080) -->
<!--
<configuration>
<path>/</path>
<port>8899</port>
</configuration>
-->
</plugin>
</plugins>
</build>
</project>
6. Hibernate Entity Classes
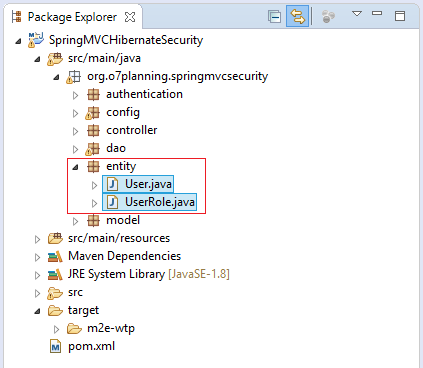
User.java
package org.o7planning.springmvcsecurity.entity;
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.Id;
import javax.persistence.Table;
@Entity
@Table(name = "Users")
public class User {
private String username;
private String password;
private boolean enabled;
@Id
@Column(name = "Username", length = 36, nullable = false)
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
@Column(name = "Password", nullable = false)
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
@Column(name = "enabled", nullable = false)
public boolean isEnabled() {
return enabled;
}
public void setEnabled(boolean enabled) {
this.enabled = enabled;
}
}
UserRole.java
package org.o7planning.springmvcsecurity.entity;
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.FetchType;
import javax.persistence.Id;
import javax.persistence.JoinColumn;
import javax.persistence.ManyToOne;
import javax.persistence.Table;
import javax.persistence.UniqueConstraint;
@Entity
@Table(name = "User_Roles", uniqueConstraints = //
@UniqueConstraint(columnNames = { "Username", "User_Role" }))
public class UserRole {
private String roleId;
private User user;
private String userRole;
@Id
@Column(name = "Role_Id", nullable = false)
public String getRoleId() {
return roleId;
}
public void setRoleId(String roleId) {
this.roleId = roleId;
}
@ManyToOne(fetch = FetchType.LAZY)
@JoinColumn(name = "Username")
public User getUser() {
return user;
}
public void setUser(User user) {
this.user = user;
}
@Column(name = "User_Role", length = 30, nullable = false)
public String getUserRole() {
return userRole;
}
public void setUserRole(String userRole) {
this.userRole = userRole;
}
}
7. Configure Spring MVC & Security
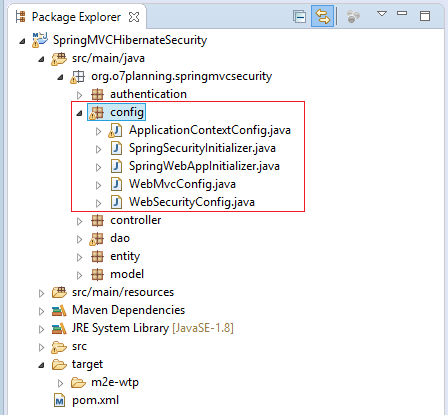
SpringWebAppInitializer.java
package org.o7planning.springmvcsecurity.config;
import javax.servlet.FilterRegistration;
import javax.servlet.ServletContext;
import javax.servlet.ServletException;
import javax.servlet.ServletRegistration;
import org.springframework.web.WebApplicationInitializer;
import org.springframework.web.context.ContextLoaderListener;
import org.springframework.web.context.support.AnnotationConfigWebApplicationContext;
import org.springframework.web.filter.CharacterEncodingFilter;
import org.springframework.web.servlet.DispatcherServlet;
public class SpringWebAppInitializer implements WebApplicationInitializer {
@Override
public void onStartup(ServletContext servletContext) throws ServletException {
AnnotationConfigWebApplicationContext appContext = new AnnotationConfigWebApplicationContext();
appContext.register(ApplicationContextConfig.class);
ServletRegistration.Dynamic dispatcher = servletContext.addServlet("SpringDispatcher",
new DispatcherServlet(appContext));
dispatcher.setLoadOnStartup(1);
dispatcher.addMapping("/");
ContextLoaderListener contextLoaderListener = new ContextLoaderListener(appContext);
servletContext.addListener(contextLoaderListener);
// Filter.
FilterRegistration.Dynamic fr = servletContext.addFilter("encodingFilter", CharacterEncodingFilter.class);
fr.setInitParameter("encoding", "UTF-8");
fr.setInitParameter("forceEncoding", "true");
fr.addMappingForUrlPatterns(null, true, "/*");
}
}
Configure:
- DataSource
- Transaction Manager
- Beans (SessionFactory, ...)
- ...
ApplicationContextConfig.java
package org.o7planning.springmvcsecurity.config;
import java.util.Properties;
import javax.sql.DataSource;
import org.apache.commons.dbcp.BasicDataSource;
import org.hibernate.SessionFactory;
import org.o7planning.springmvcsecurity.dao.UserInfoDAO;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.ComponentScan;
import org.springframework.context.annotation.Configuration;
import org.springframework.context.annotation.PropertySource;
import org.springframework.context.support.ResourceBundleMessageSource;
import org.springframework.core.env.Environment;
import org.springframework.orm.hibernate4.HibernateTransactionManager;
import org.springframework.orm.hibernate4.LocalSessionFactoryBean;
import org.springframework.transaction.annotation.EnableTransactionManagement;
import org.springframework.web.servlet.view.InternalResourceViewResolver;
@Configuration
@ComponentScan("org.o7planning.springmvcsecurity.*")
@EnableTransactionManagement
// Load to Environment.
@PropertySource("classpath:datasource-cfg.properties")
public class ApplicationContextConfig {
// The Environment class serves as the property holder
// and stores all the properties loaded by the @PropertySource
@Autowired
private Environment env;
@Autowired
private UserInfoDAO userInfoDAO;
@Bean
public ResourceBundleMessageSource messageSource() {
ResourceBundleMessageSource rb = new ResourceBundleMessageSource();
// Load property in message/validator.properties
rb.setBasenames(new String[] { "messages/validator" });
return rb;
}
@Bean(name = "viewResolver")
public InternalResourceViewResolver getViewResolver() {
InternalResourceViewResolver viewResolver = new InternalResourceViewResolver();
viewResolver.setPrefix("/WEB-INF/pages/");
viewResolver.setSuffix(".jsp");
return viewResolver;
}
@Bean(name = "dataSource")
public DataSource getDataSource() {
BasicDataSource dataSource = new BasicDataSource();
// See: datasouce-cfg.properties
dataSource.setDriverClassName(env.getProperty("ds.database-driver"));
dataSource.setUrl(env.getProperty("ds.url"));
dataSource.setUsername(env.getProperty("ds.username"));
dataSource.setPassword(env.getProperty("ds.password"));
System.out.println("## getDataSource: " + dataSource);
return dataSource;
}
@Autowired
@Bean(name = "sessionFactory")
public SessionFactory getSessionFactory(DataSource dataSource) throws Exception {
System.out.println("## getSessionFactory .... ");
try {
Properties properties = new Properties();
// See: ds-hibernate-cfg.properties
properties.put("hibernate.dialect", env.getProperty("hibernate.dialect"));
properties.put("hibernate.show_sql", env.getProperty("hibernate.show_sql"));
properties.put("current_session_context_class", env.getProperty("current_session_context_class"));
LocalSessionFactoryBean factoryBean = new LocalSessionFactoryBean();
// Package contain entity classes
factoryBean.setPackagesToScan(new String[] { "org.o7planning.springmvcsecurity.entity" });
factoryBean.setDataSource(dataSource);
factoryBean.setHibernateProperties(properties);
factoryBean.afterPropertiesSet();
//
SessionFactory sf = factoryBean.getObject();
System.out.println("## getSessionFactory: " + sf);
return sf;
} catch (Exception e) {
System.out.println("Error getSessionFactory: " + e);
e.printStackTrace();
throw e;
}
}
// Hibernate Transaction Manager
@Autowired
@Bean(name = "transactionManager")
public HibernateTransactionManager getTransactionManager(SessionFactory sessionFactory) {
HibernateTransactionManager transactionManager = new HibernateTransactionManager(sessionFactory);
return transactionManager;
}
}
ApplicationContextConfig will read datasouce-cfg.xml file:
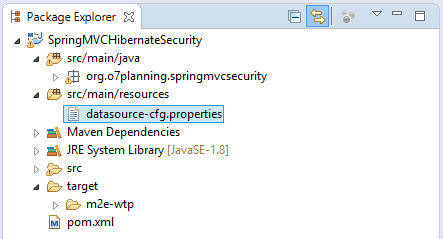
datasouce-cfg.properties (For Oracle)
# DataSource
ds.database-driver=oracle.jdbc.driver.OracleDriver
ds.url=jdbc:oracle:thin:@localhost:1521:db12c
ds.username=test
ds.password=12345
# Hibernate Config
hibernate.dialect=org.hibernate.dialect.Oracle10gDialect
hibernate.show_sql=true
current_session_context_class=thread
hibernate.hbm2ddl.auto=update
datasource-cfg.properties (For SQL Server)
# DataSource
ds.database-driver=net.sourceforge.jtds.jdbc.Driver
ds.url=jdbc:jtds:sqlserver://localhost:1433/simplehr;instance=SQLEXPRESS
ds.username=test
ds.password=12345
# Hibernate Config
hibernate.dialect=org.hibernate.dialect.SQLServerDialect
hibernate.show_sql=true
current_session_context_class=thread
hibernate.hbm2ddl.auto=update
datasource-cfg.properties (For MySQL)
# DataSource
ds.database-driver=com.mysql.jdbc.Driver
ds.url=jdbc:mysql://localhost:3306/mydatabase
ds.username=root
ds.password=12345
# Hibernate Config
hibernate.dialect=org.hibernate.dialect.MySQLDialect
hibernate.show_sql=true
current_session_context_class=thread
hibernate.hbm2ddl.auto=update
WebMvcConfig.java
package org.o7planning.springmvcsecurity.config;
import java.nio.charset.Charset;
import java.util.Arrays;
import java.util.List;
import org.springframework.context.annotation.Configuration;
import org.springframework.http.MediaType;
import org.springframework.http.converter.HttpMessageConverter;
import org.springframework.http.converter.StringHttpMessageConverter;
import org.springframework.web.servlet.config.annotation.DefaultServletHandlerConfigurer;
import org.springframework.web.servlet.config.annotation.EnableWebMvc;
import org.springframework.web.servlet.config.annotation.ResourceHandlerRegistry;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurerAdapter;
@Configuration
@EnableWebMvc
public class WebMvcConfig extends WebMvcConfigurerAdapter {
private static final Charset UTF8 = Charset.forName("UTF-8");
// Config UTF-8 Encoding.
@Override
public void configureMessageConverters(List<HttpMessageConverter<?>> converters) {
StringHttpMessageConverter stringConverter = new StringHttpMessageConverter();
stringConverter.setSupportedMediaTypes(Arrays.asList(new MediaType("text", "plain", UTF8)));
converters.add(stringConverter);
// Add other converters ...
}
@Override
public void addResourceHandlers(ResourceHandlerRegistry registry) {
}
@Override
public void configureDefaultServletHandling(DefaultServletHandlerConfigurer configurer) {
configurer.enable();
}
}
SpringSecurityInitializer.java
package org.o7planning.springmvcsecurity.config;
import org.springframework.security.web.context.AbstractSecurityWebApplicationInitializer;
public class SpringSecurityInitializer extends AbstractSecurityWebApplicationInitializer {
// Do nothing
}
WebSecurityConfig.java
package org.o7planning.springmvcsecurity.config;
import org.o7planning.springmvcsecurity.authentication.MyUserDetailsService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.annotation.Configuration;
import org.springframework.security.config.annotation.authentication.builders.AuthenticationManagerBuilder;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
import org.springframework.transaction.annotation.Transactional;
@Configuration
@EnableWebSecurity
@Transactional
public class WebSecurityConfig extends WebSecurityConfigurerAdapter {
@Autowired
private MyUserDetailsService myUserDetailsService;
@Autowired
public void configureGlobal(AuthenticationManagerBuilder auth) throws Exception {
// Users in memory.
auth.inMemoryAuthentication().withUser("user1").password("12345").roles("USER");
auth.inMemoryAuthentication().withUser("admin1").password("12345").roles("USER, ADMIN");
// For User in database.
auth.userDetailsService(myUserDetailsService);
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http.csrf().disable();
// The pages does not require login
http.authorizeRequests().antMatchers("/", "/welcome", "/login", "/logout").permitAll();
// /userInfo page requires login as USER or ADMIN.
// If no login, it will redirect to /login page.
http.authorizeRequests().antMatchers("/userInfo").access("hasAnyRole('ROLE_USER', 'ROLE_ADMIN')");
// For ADMIN only.
http.authorizeRequests().antMatchers("/admin").access("hasRole('ROLE_ADMIN')");
// When the user has logged in as XX.
// But access a page that requires role YY,
// AccessDeniedException will throw.
http.authorizeRequests().and().exceptionHandling().accessDeniedPage("/403");
// Config for Login Form
http.authorizeRequests().and().formLogin()//
// Submit URL of login page.
.loginProcessingUrl("/j_spring_security_check") // Submit URL
.loginPage("/login")//
.defaultSuccessUrl("/userInfo")//
.failureUrl("/login?error=true")//
.usernameParameter("username")//
.passwordParameter("password")
// Config for Logout Page
.and().logout().logoutUrl("/logout").logoutSuccessUrl("/logoutSuccessful");
}
}
8. Model, DAO, UserDetailService
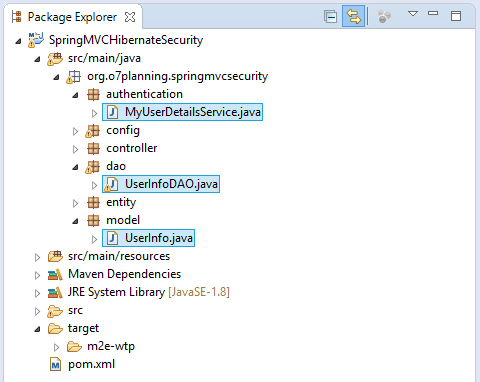
UserInfo class corresponds to a record in the USERS table.
UserInfo.java
package org.o7planning.springmvcsecurity.model;
public class UserInfo {
private String userName;
private String password;
public UserInfo() {
}
// Do not change this constructor, it used in hibernate Query.
public UserInfo(String userName, String password) {
this.userName = userName;
this.password = password;
}
public String getUserName() {
return userName;
}
public void setUserName(String userName) {
this.userName = userName;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
}
UserInfoDAO.java
package org.o7planning.springmvcsecurity.dao;
import java.util.List;
import org.hibernate.Query;
import org.hibernate.Session;
import org.hibernate.SessionFactory;
import org.o7planning.springmvcsecurity.entity.User;
import org.o7planning.springmvcsecurity.entity.UserRole;
import org.o7planning.springmvcsecurity.model.UserInfo;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Repository;
import org.springframework.transaction.annotation.Transactional;
@Repository
@Transactional
public class UserInfoDAO {
@Autowired
private SessionFactory sessionFactory;
public UserInfoDAO() {
}
public UserInfo findUserInfo(String userName) {
String sql = "Select new " + UserInfo.class.getName() + "(u.username,u.password) "//
+ " from " + User.class.getName() + " u where u.username = :username ";
Session session = sessionFactory.getCurrentSession();
Query query = session.createQuery(sql);
query.setParameter("username", userName);
return (UserInfo) query.uniqueResult();
}
public List<String> getUserRoles(String userName) {
String sql = "Select r.userRole "//
+ " from " + UserRole.class.getName() + " r where r.user.username = :username ";
Session session = sessionFactory.getCurrentSession();
Query query = session.createQuery(sql);
query.setParameter("username", userName);
List<String> roles = query.list();
return roles;
}
}
MyUserDetailsService.java
package org.o7planning.springmvcsecurity.authentication;
import java.util.ArrayList;
import java.util.List;
import org.o7planning.springmvcsecurity.dao.UserInfoDAO;
import org.o7planning.springmvcsecurity.model.UserInfo;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.security.core.GrantedAuthority;
import org.springframework.security.core.authority.SimpleGrantedAuthority;
import org.springframework.security.core.userdetails.User;
import org.springframework.security.core.userdetails.UserDetails;
import org.springframework.security.core.userdetails.UserDetailsService;
import org.springframework.security.core.userdetails.UsernameNotFoundException;
import org.springframework.stereotype.Service;
@Service
public class MyUserDetailsService implements UserDetailsService {
@Autowired
private UserInfoDAO userInfoDAO;
@Override
public UserDetails loadUserByUsername(String username) throws UsernameNotFoundException {
UserInfo userInfo = userInfoDAO.findUserInfo(username);
System.out.println("UserInfo= " + userInfo);
if (userInfo == null) {
throw new UsernameNotFoundException("User " + username + " was not found in the database");
}
// [USER,ADMIN,..]
List<String> roles= userInfoDAO.getUserRoles(username);
List<GrantedAuthority> grantList= new ArrayList<GrantedAuthority>();
if(roles!= null) {
for(String role: roles) {
// ROLE_USER, ROLE_ADMIN,..
GrantedAuthority authority = new SimpleGrantedAuthority("ROLE_" + role);
grantList.add(authority);
}
}
UserDetails userDetails = (UserDetails) new User(userInfo.getUserName(), //
userInfo.getPassword(),grantList);
return userDetails;
}
}
9. Spring MVC Controller
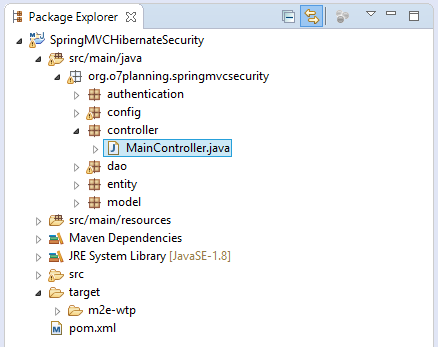
MainController.java
package org.o7planning.springmvcsecurity.controller;
import java.security.Principal;
import org.springframework.stereotype.Controller;
import org.springframework.transaction.annotation.Transactional;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
@Controller
@Transactional
public class MainController {
@RequestMapping(value = { "/", "/welcome" }, method = RequestMethod.GET)
public String welcomePage(Model model) {
model.addAttribute("title", "Welcome");
model.addAttribute("message", "This is welcome page!");
return "welcomePage";
}
@RequestMapping(value = "/admin", method = RequestMethod.GET)
public String adminPage(Model model) {
return "adminPage";
}
@RequestMapping(value = "/login", method = RequestMethod.GET)
public String loginPage(Model model ) {
return "loginPage";
}
@RequestMapping(value = "/logoutSuccessful", method = RequestMethod.GET)
public String logoutSuccessfulPage(Model model) {
model.addAttribute("title", "Logout");
return "logoutSuccessfulPage";
}
@RequestMapping(value = "/userInfo", method = RequestMethod.GET)
public String userInfo(Model model, Principal principal) {
// After user login successfully.
String userName = principal.getName();
System.out.println("User Name: "+ userName);
return "userInfoPage";
}
@RequestMapping(value = "/403", method = RequestMethod.GET)
public String accessDenied(Model model, Principal principal) {
if (principal != null) {
model.addAttribute("message", "Hi " + principal.getName()
+ "<br> You do not have permission to access this page!");
} else {
model.addAttribute("msg",
"You do not have permission to access this page!");
}
return "403Page";
}
}
10. JSP Pages
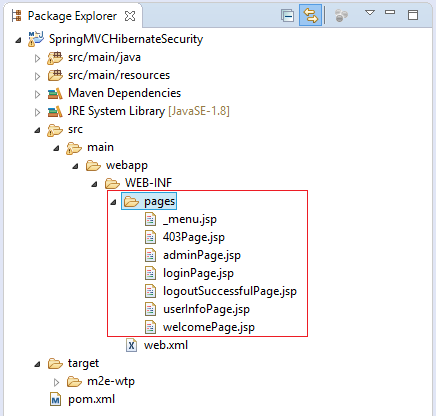
_menu.jsp
<%@taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core"%>
<div style="border: 1px solid #ccc;padding:5px;margin-bottom:20px;">
<a href="${pageContext.request.contextPath}/welcome">Home</a>
|
<a href="${pageContext.request.contextPath}/userInfo">User Info</a>
|
<a href="${pageContext.request.contextPath}/admin">Admin</a>
<c:if test="${pageContext.request.userPrincipal.name != null}">
|
<a href="${pageContext.request.contextPath}/logout">Logout</a>
</c:if>
</div>
403Page.jsp
<%@page session="false"%>
<html>
<head>
<title>Access Denied</title>
</head>
<body>
<jsp:include page="_menu.jsp"/>
<h3 style="color:red;">${message}</h3>
</body>
</html>
adminPage.jsp
<%@taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core"%>
<%@page session="true"%>
<html>
<head>
<title>${title}</title>
</head>
<body>
<jsp:include page="_menu.jsp" />
<h2>Admin Page</h2>
<h3>Welcome : ${pageContext.request.userPrincipal.name}</h3>
<b>This is protected page!</b>
</body>
</html>
loginPage.jsp
<%@taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core"%>
<html>
<head><title>Login</title></head>
<body>
<jsp:include page="_menu.jsp" />
<h1>Login</h1>
<!-- /login?error=true -->
<c:if test="${param.error == 'true'}">
<div style="color:red;margin:10px 0px;">
Login Failed!!!<br />
Reason : ${sessionScope["SPRING_SECURITY_LAST_EXCEPTION"].message}
</div>
</c:if>
<h3>Enter user name and password:</h3>
<form name='f' action="${pageContext.request.contextPath}/j_spring_security_check" method='POST'>
<table>
<tr>
<td>User:</td>
<td><input type='text' name='username' value=''></td>
</tr>
<tr>
<td>Password:</td>
<td><input type='password' name='password' /></td>
</tr>
<tr>
<td><input name="submit" type="submit" value="submit" /></td>
</tr>
</table>
</form>
</body>
</html>
logoutSuccessfulPage.jsp
<html>
<head>
<title>Logout</title>
</head>
<body>
<jsp:include page="_menu.jsp" />
<h1>Logout Successful!</h1>
</body>
</html>
userInfoPage.jsp
<%@taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core"%>
<%@page session="true"%>
<html>
<head>
<title>User Info</title>
</head>
<body>
<jsp:include page="_menu.jsp" />
<h2>User Info Page</h2>
<h3>Welcome : ${pageContext.request.userPrincipal.name}</h3>
<b>This is protected page!</b>
</body>
</html>
welcomePage.jsp
<%@page session="false"%>
<html>
<head>
<title>${title}</title>
</head>
<body>
<jsp:include page="_menu.jsp" />
<h1>Message : ${message}</h1>
</body>
</html>
11. Configure and run the application
In the first, before running the application you need to build the project.
Right-click the project and select:
- Run As/Maven install
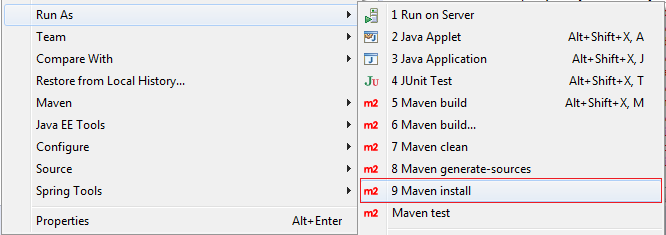
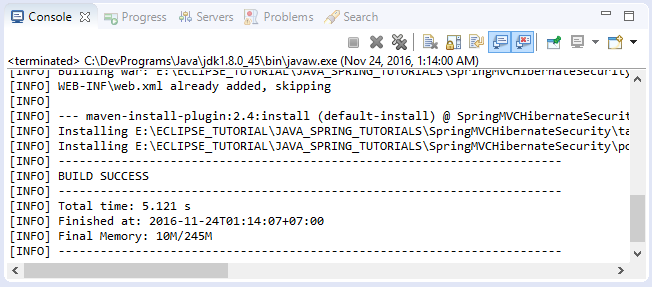
Run configurations:
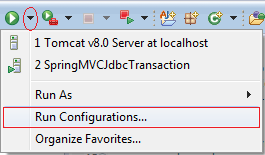
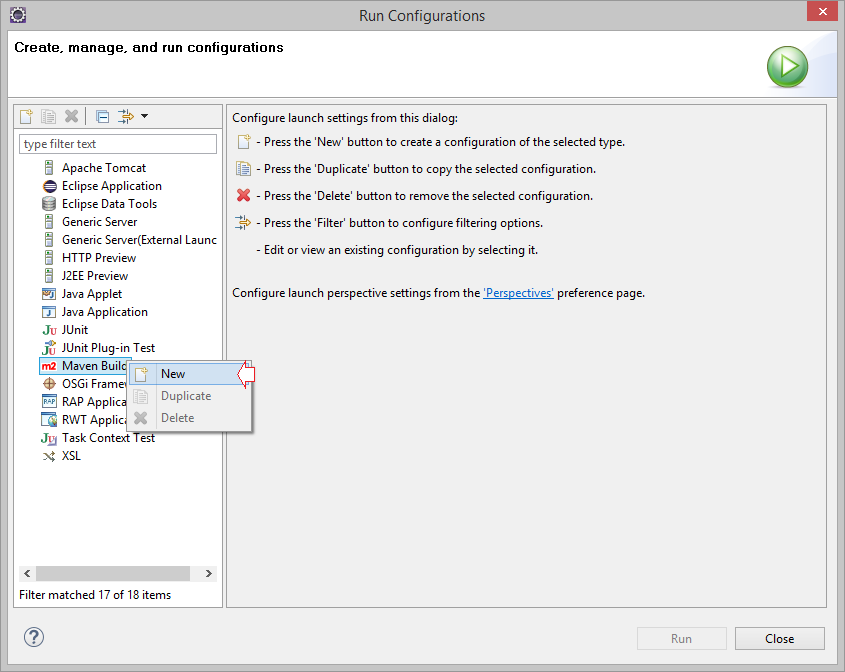
Enter:
- Name: Run SpringMVCHibernateSecurity
- Base directory: ${workspace_loc:/SpringMVCHibernateSecurity}
- Goals: tomcat7:run
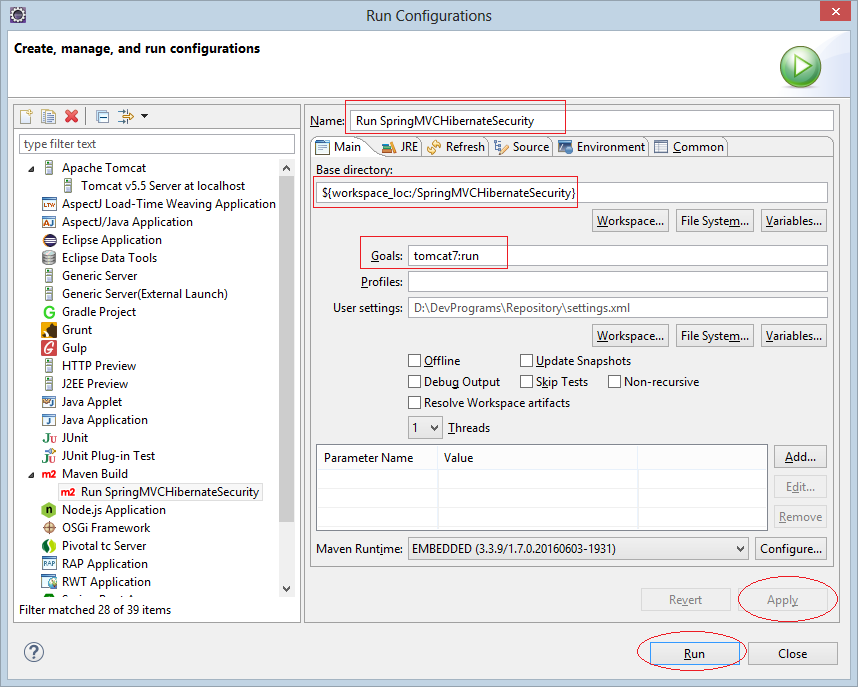
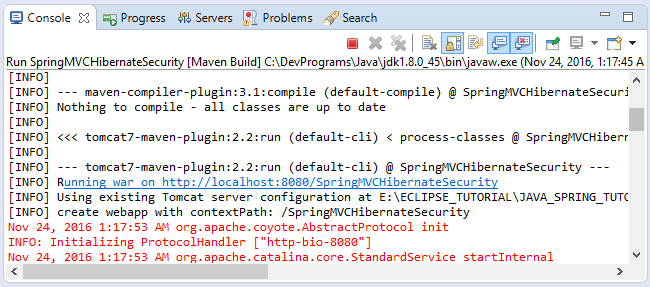
Running URL:
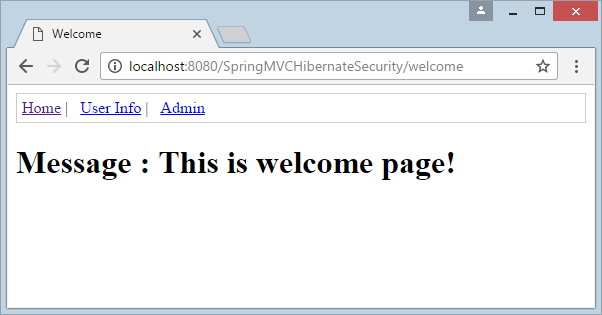
Enter URL to see user information, in case you have not login, the website will be redirected to the login page
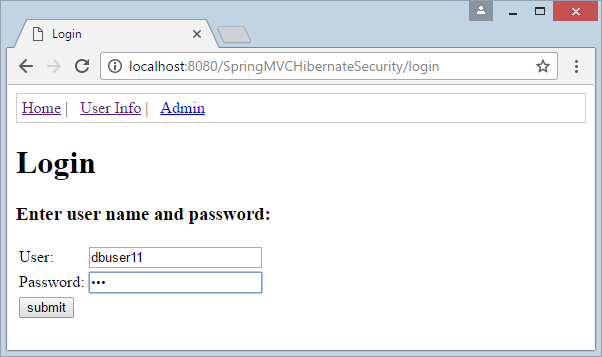
If you enter wrong information and click Submit, website will redirect to the login page.
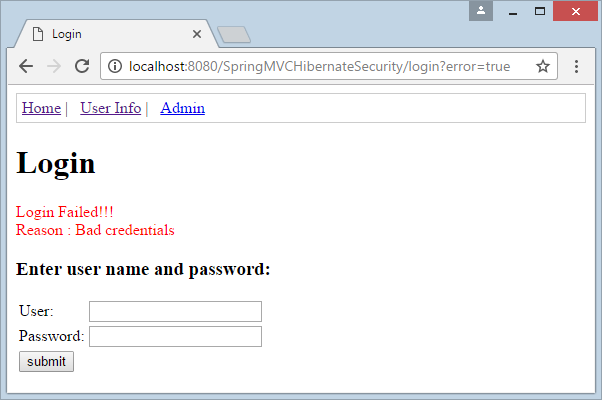
In case of a successful login:
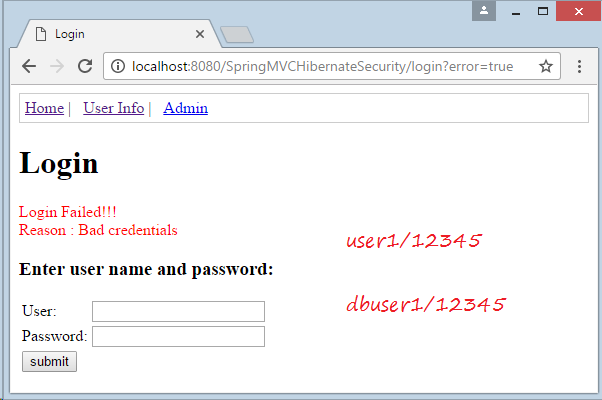
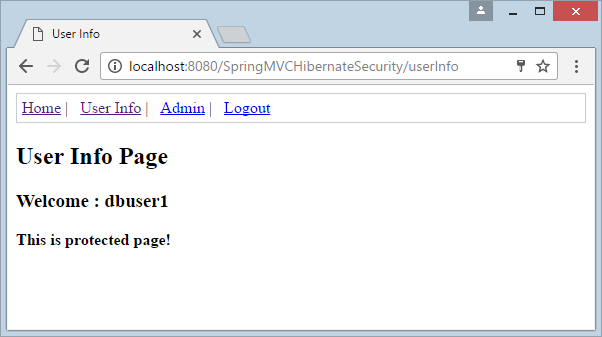
You can try with the URL:
The above URL allows user as an ADMIN (ROLE-ADMIN) to access:
In the case of unlogin, website will redirect to the login page. If you login as a ROLE-USER, you will receive a notice of denying access (Access Denied)
The above URL allows user as an ADMIN (ROLE-ADMIN) to access:
In the case of unlogin, website will redirect to the login page. If you login as a ROLE-USER, you will receive a notice of denying access (Access Denied)
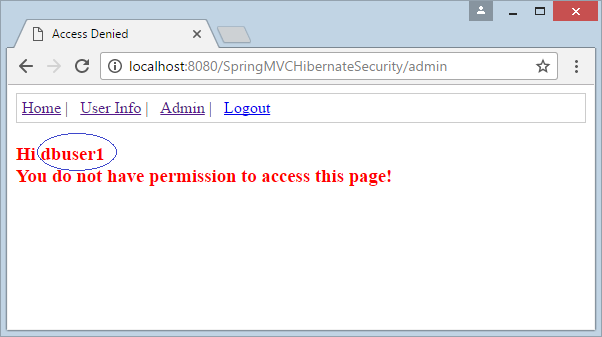
Spring MVC Tutorials
- Spring Tutorial for Beginners
- Install Spring Tool Suite for Eclipse
- Spring MVC Tutorial for Beginners - Hello Spring 4 MVC
- Configure Static Resources in Spring MVC
- Spring MVC Interceptors Tutorial with Examples
- Create a Multiple Languages web application with Spring MVC
- Spring MVC File Upload Tutorial with Examples
- Simple Login Java Web Application using Spring MVC, Spring Security and Spring JDBC
- Spring MVC Security with Hibernate Tutorial with Examples
- Spring MVC Security and Spring JDBC Tutorial (XML Config)
- Social Login in Spring MVC with Spring Social Security
- Spring MVC and Velocity Tutorial with Examples
- Spring MVC and FreeMarker Tutorial with Examples
- Use Template in Spring MVC with Apache Tiles
- Spring MVC and Spring JDBC Transaction Tutorial with Examples
- Use Multiple DataSources in Spring MVC
- Spring MVC and Hibernate Transaction Tutorial with Examples
- Spring MVC Form Handling and Hibernate Tutorial with Examples
- Run background scheduled tasks in Spring
- Create a Java Shopping Cart Web Application using Spring MVC and Hibernate
- Simple CRUD example with Spring MVC RESTful Web Service
- Deploy Spring MVC on Oracle WebLogic Server
Show More