Spring Boot Restful Client with RestTemplate Example
1. Objective of Example
This document is based on:
- Spring Boot 2.x
- RestTemplate
- Eclipse 3.7
In this post, I will guide you for creating a Restful Client application using Spring Boot with the 4 functions:
- Create a request with GET method, and send it to Restful Web Service to receive a list of employees, or an employment's information. The data received is in XML format or JSON format.
- Create a request with PUT method, and send it to Restful Web Service to ask to edit the information of an employment. The data attached to the request is in XML format or JSON format.
- Create a request with POST method and send it to Restful Web Service to create a new employee. The data attached to the request is in XML format or JSON format.
- Create a request with DELETE method, and send it to Restful Web Service to delete an employee.
This post uses the Restful Web Service created from the following example:
The RestTemplate class is the central class in Spring Framework for the synchronous calls by the client to access a REST web-service. This class provides the functionality for consuming the REST Services in a easy manner. When using the said class the user has to only provide the URL, the parameters(if any) and extract the results received. The RestTemplate manages the HTTP connections.
2. Create Spring Boot project
On the Eclipse, create a Spring Boot project.
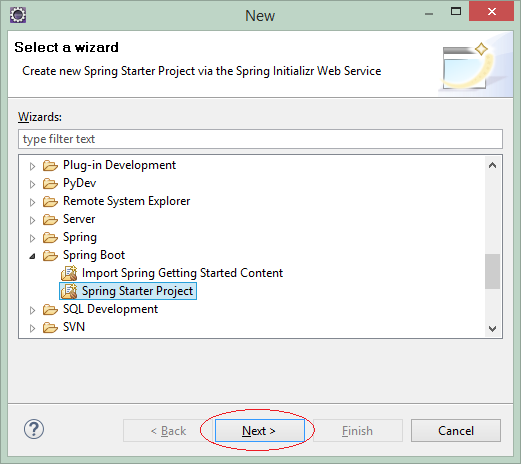
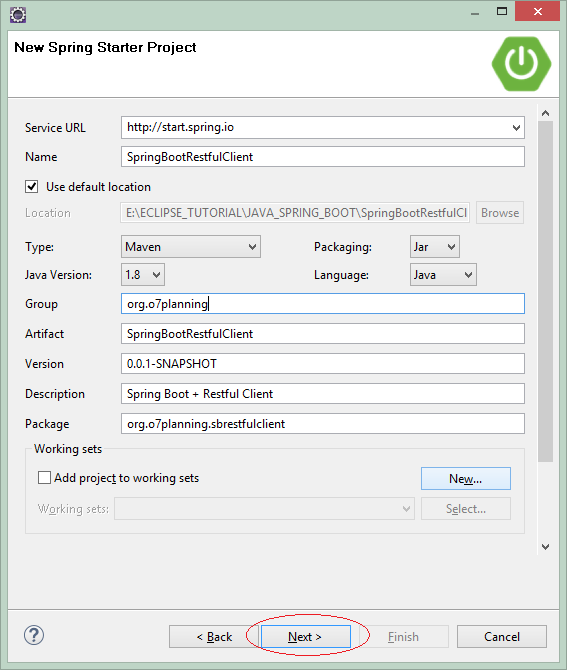
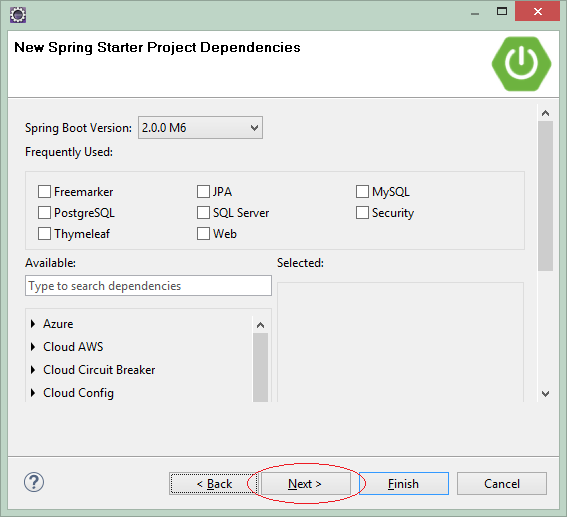
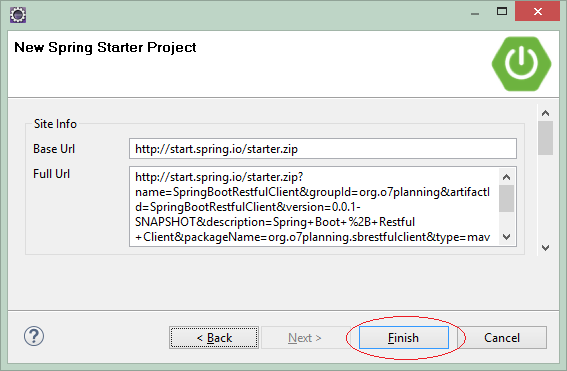
OK, the project has been created.
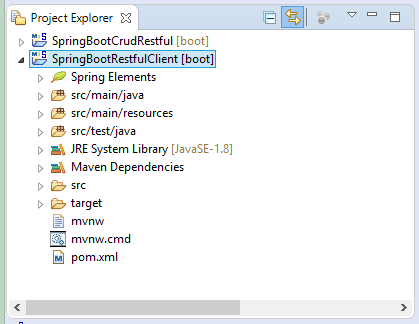
3. Configure pom.xml
This project needs to use Spring Restful Client libraries. Therefore, you have two choices:
- spring-boot-starter-web
- spring-boot-starter-data-rest
spring-boot-starter-web
spring-boot-starter-web consists of libraries to build a web application using Spring MVC, and tomcat as a default embedded Web Container. It includes libraries for RESTful application.
spring-boot-starter-web
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
spring-boot-starter-data-rest
The spring-boot-starter-data-rest includes libraries to work with Spring RESTful.
spring-boot-starter-data-rest
<!-- https://mvnrepository.com/artifact/org.springframework.boot/spring-boot-starter-data-rest -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-rest</artifactId>
</dependency>
Java <==> JSON
Both the above "Starters" contain a jackson-databind library to support conversion of a Java object into JSON and vice versa.
Java <==> XML
Spring uses JAXB (available in JDK) to convert the Java object into XML and vice versa. However, Java classes must be annotated by @XmlRootElement,... Therefore, my advice is that you should use jackson-dataformat-xml as a library to convert XML and Java. To use the jackson-dataformat-xml, you need to declare it inpom.xml file:
jackson-dataformat-xml
<dependency>
<groupId>com.fasterxml.jackson.dataformat</groupId>
<artifactId>jackson-dataformat-xml</artifactId>
</dependency>
OK, you have 2 choices to declare in the pom.xml:
** pom.xml (Option 1) **
<dependencies>
......
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-rest</artifactId>
</dependency>
<dependency>
<groupId>com.fasterxml.jackson.dataformat</groupId>
<artifactId>jackson-dataformat-xml</artifactId>
</dependency>
.....
</dependencies>
** pom.xml (Option 2) **
<dependencies>
......
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>com.fasterxml.jackson.dataformat</groupId>
<artifactId>jackson-dataformat-xml</artifactId>
</dependency>
.....
</dependencies>
The Apache Commons Codec library is necessary for encoding anusername/password in case, you use Rest Client to access data resources secured by Basic Authentication.
<!-- https://mvnrepository.com/artifact/commons-codec/commons-codec -->
<dependency>
<groupId>commons-codec</groupId>
<artifactId>commons-codec</artifactId>
</dependency>
The full content of the pom.xml file:
pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0
http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.o7planning</groupId>
<artifactId>SpringBootRestfulClient</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>jar</packaging>
<name>SpringBootRestfulClient</name>
<description>Spring Boot + Restful Client</description>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.0.0.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-rest</artifactId>
</dependency>
<dependency>
<groupId>com.fasterxml.jackson.dataformat</groupId>
<artifactId>jackson-dataformat-xml</artifactId>
</dependency>
<!-- https://mvnrepository.com/artifact/commons-codec/commons-codec -->
<dependency>
<groupId>commons-codec</groupId>
<artifactId>commons-codec</artifactId>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
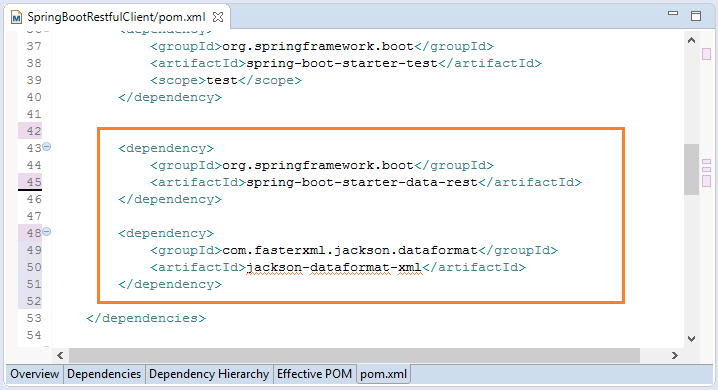
4. GET - getForObject
Use the getForObject method to send a request to the Restful Service, and receive data returned. Below is the simplest example. The data returned is a string.
SimplestGetExample.java
package org.o7planning.sbrestfulclient.get;
import org.springframework.web.client.RestTemplate;
public class SimplestGetExample {
static final String URL_EMPLOYEES = "http://localhost:8080/employees";
static final String URL_EMPLOYEES_XML = "http://localhost:8080/employees.xml";
static final String URL_EMPLOYEES_JSON = "http://localhost:8080/employees.json";
public static void main(String[] args) {
RestTemplate restTemplate = new RestTemplate();
// Send request with GET method and default Headers.
String result = restTemplate.getForObject(URL_EMPLOYEES, String.class);
System.out.println(result);
}
}
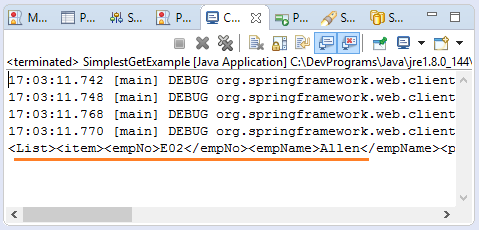
The requests sent to the Restful Service need to customize Headers information to tell the Restful Service about the type of data format you want to get (JSON, XML, ...)
GetWithHeaderExample.java
package org.o7planning.sbrestfulclient.get;
import java.util.Arrays;
import org.springframework.http.HttpEntity;
import org.springframework.http.HttpHeaders;
import org.springframework.http.HttpMethod;
import org.springframework.http.MediaType;
import org.springframework.http.ResponseEntity;
import org.springframework.web.client.RestTemplate;
public class GetWithHeaderExample {
static final String URL_EMPLOYEES = "http://localhost:8080/employees";
public static void main(String[] args) {
// HttpHeaders
HttpHeaders headers = new HttpHeaders();
headers.setAccept(Arrays.asList(new MediaType[] { MediaType.APPLICATION_JSON }));
// Request to return JSON format
headers.setContentType(MediaType.APPLICATION_JSON);
headers.set("my_other_key", "my_other_value");
// HttpEntity<String>: To get result as String.
HttpEntity<String> entity = new HttpEntity<String>(headers);
// RestTemplate
RestTemplate restTemplate = new RestTemplate();
// Send request with GET method, and Headers.
ResponseEntity<String> response = restTemplate.exchange(URL_EMPLOYEES, //
HttpMethod.GET, entity, String.class);
String result = response.getBody();
System.out.println(result);
}
}
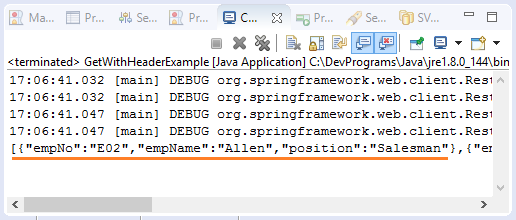
Data returned from RESTful Serivce in XML or JSON format can be automatically converted into a Java object.
Employee.java
package org.o7planning.sbrestfulclient.model;
public class Employee {
private String empNo;
private String empName;
private String position;
public Employee() {
}
public Employee(String empNo, String empName, String position) {
this.empNo = empNo;
this.empName = empName;
this.position = position;
}
public String getEmpNo() {
return empNo;
}
public void setEmpNo(String empNo) {
this.empNo = empNo;
}
public String getEmpName() {
return empName;
}
public void setEmpName(String empName) {
this.empName = empName;
}
public String getPosition() {
return position;
}
public void setPosition(String position) {
this.position = position;
}
}
SimplestGetPOJOExample.java
package org.o7planning.sbrestfulclient.get;
import org.o7planning.sbrestfulclient.model.Employee;
import org.springframework.web.client.RestTemplate;
public class SimplestGetPOJOExample {
static final String URL_EMPLOYEES = "http://localhost:8080/employees";
static final String URL_EMPLOYEES_XML = "http://localhost:8080/employees.xml";
static final String URL_EMPLOYEES_JSON = "http://localhost:8080/employees.json";
public static void main(String[] args) {
RestTemplate restTemplate = new RestTemplate();
// Send request with GET method and default Headers.
Employee[] list = restTemplate.getForObject(URL_EMPLOYEES, Employee[].class);
if (list != null) {
for (Employee e : list) {
System.out.println("Employee: " + e.getEmpNo() + " - " + e.getEmpName());
}
}
}
}
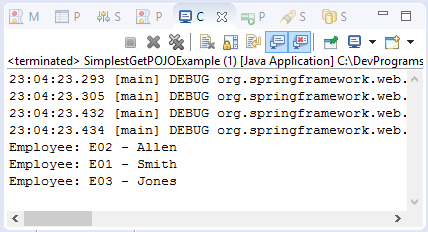
5. GET - exchange
Use exchange method also helps you to be able send a request to the Restful Service. The result returned isResponseEntity object. This object contains a lot of noteworthy information, for example HttpStatus,...
GetPOJOWithHeaderExample.java
package org.o7planning.sbrestfulclient.get;
import java.util.Arrays;
import org.o7planning.sbrestfulclient.model.Employee;
import org.springframework.http.HttpEntity;
import org.springframework.http.HttpHeaders;
import org.springframework.http.HttpMethod;
import org.springframework.http.HttpStatus;
import org.springframework.http.MediaType;
import org.springframework.http.ResponseEntity;
import org.springframework.web.client.RestTemplate;
public class GetPOJOWithHeaderExample {
static final String URL_EMPLOYEES = "http://localhost:8080/employees";
public static void main(String[] args) {
// HttpHeaders
HttpHeaders headers = new HttpHeaders();
headers.setAccept(Arrays.asList(new MediaType[] { MediaType.APPLICATION_XML }));
// Request to return XML format
headers.setContentType(MediaType.APPLICATION_XML);
headers.set("my_other_key", "my_other_value");
// HttpEntity<Employee[]>: To get result as Employee[].
HttpEntity<Employee[]> entity = new HttpEntity<Employee[]>(headers);
// RestTemplate
RestTemplate restTemplate = new RestTemplate();
// Send request with GET method, and Headers.
ResponseEntity<Employee[]> response = restTemplate.exchange(URL_EMPLOYEES, //
HttpMethod.GET, entity, Employee[].class);
HttpStatus statusCode = response.getStatusCode();
System.out.println("Response Satus Code: " + statusCode);
// Status Code: 200
if (statusCode == HttpStatus.OK) {
// Response Body Data
Employee[] list = response.getBody();
if (list != null) {
for (Employee e : list) {
System.out.println("Employee: " + e.getEmpNo() + " - " + e.getEmpName());
}
}
}
}
}
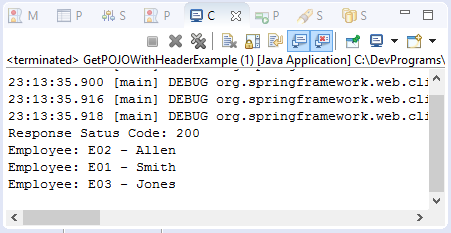
6. GET - Basic Authentication
For the data resources secured by the Basic Authentication, the requests sent to REST Service by you must be attached username/password. The username/password information needs encoding using the Base64 algorithm before being attached with the request.
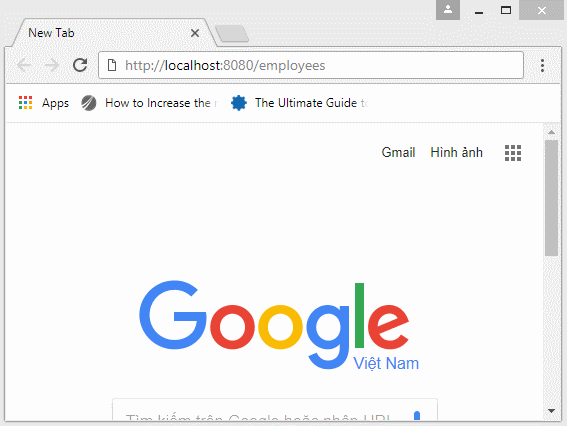
GetWithBasicAuthExample.java
package org.o7planning.sbrestfulclient.get;
import java.nio.charset.Charset;
import java.util.Arrays;
import org.springframework.http.HttpEntity;
import org.springframework.http.HttpHeaders;
import org.springframework.http.HttpMethod;
import org.springframework.http.MediaType;
import org.springframework.http.ResponseEntity;
import org.springframework.web.client.RestTemplate;
import org.apache.commons.codec.binary.Base64;
public class GetWithBasicAuthExample {
public static final String USER_NAME = "tom";
public static final String PASSWORD = "123";
static final String URL_EMPLOYEES = "http://localhost:8080/employees";
public static void main(String[] args) {
// HttpHeaders
HttpHeaders headers = new HttpHeaders();
//
// Authentication
//
String auth = USER_NAME + ":" + PASSWORD;
byte[] encodedAuth = Base64.encodeBase64(auth.getBytes(Charset.forName("US-ASCII")));
String authHeader = "Basic " + new String(encodedAuth);
headers.set("Authorization", authHeader);
//
headers.setAccept(Arrays.asList(new MediaType[] { MediaType.APPLICATION_JSON }));
// Request to return JSON format
headers.setContentType(MediaType.APPLICATION_JSON);
headers.set("my_other_key", "my_other_value");
// HttpEntity<String>: To get result as String.
HttpEntity<String> entity = new HttpEntity<String>(headers);
// RestTemplate
RestTemplate restTemplate = new RestTemplate();
// Send request with GET method, and Headers.
ResponseEntity<String> response = restTemplate.exchange(URL_EMPLOYEES, //
HttpMethod.GET, entity, String.class);
String result = response.getBody();
System.out.println(result);
}
}
7. POST - postForObject
The postForObject method is used to send a request to the Restful Service to create a data resource and return the data resource which has just been created.
Post_postForObject_Example.java
package org.o7planning.sbrestfulclient.post;
import org.o7planning.sbrestfulclient.model.Employee;
import org.springframework.http.HttpEntity;
import org.springframework.http.HttpHeaders;
import org.springframework.http.MediaType;
import org.springframework.web.client.RestTemplate;
public class Post_postForObject_Example {
static final String URL_CREATE_EMPLOYEE = "http://localhost:8080/employee";
public static void main(String[] args) {
String empNo = "E11";
Employee newEmployee = new Employee(empNo, "Tom", "Cleck");
HttpHeaders headers = new HttpHeaders();
headers.add("Accept", MediaType.APPLICATION_XML_VALUE);
headers.setContentType(MediaType.APPLICATION_XML);
RestTemplate restTemplate = new RestTemplate();
// Data attached to the request.
HttpEntity<Employee> requestBody = new HttpEntity<>(newEmployee, headers);
// Send request with POST method.
Employee e = restTemplate.postForObject(URL_CREATE_EMPLOYEE, requestBody, Employee.class);
if (e != null && e.getEmpNo() != null) {
System.out.println("Employee created: " + e.getEmpNo());
} else {
System.out.println("Something error!");
}
}
}
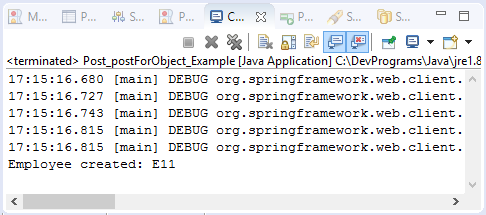
8. POST - postForEntity
The postForEntity method is used to send arequest to the Restful Service to create a data resource. This method returns the ResponseEntity object. This object contains data resouce which has just been created and other noteworthy information, for example, HttpStatus, ...
Post_postForEntity_Example.java
package org.o7planning.sbrestfulclient.post;
import org.o7planning.sbrestfulclient.model.Employee;
import org.springframework.http.HttpEntity;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.client.RestTemplate;
public class Post_postForEntity_Example {
static final String URL_CREATE_EMPLOYEE = "http://localhost:8080/employee";
public static void main(String[] args) {
Employee newEmployee = new Employee("E11", "Tom", "Cleck");
RestTemplate restTemplate = new RestTemplate();
// Data attached to the request.
HttpEntity<Employee> requestBody = new HttpEntity<>(newEmployee);
// Send request with POST method.
ResponseEntity<Employee> result
= restTemplate.postForEntity(URL_CREATE_EMPLOYEE, requestBody, Employee.class);
System.out.println("Status code:" + result.getStatusCode());
// Code = 200.
if (result.getStatusCode() == HttpStatus.OK) {
Employee e = result.getBody();
System.out.println("(Client Side) Employee Created: "+ e.getEmpNo());
}
}
}
9. PUT - Simple Example
The put method of the RestTemplate class is used to send a request to the Restful Service to change a data resource. This method returns nothing.
PutSimpleExample.java
package org.o7planning.sbrestfulclient.put;
import org.o7planning.sbrestfulclient.model.Employee;
import org.springframework.http.HttpEntity;
import org.springframework.http.HttpHeaders;
import org.springframework.http.MediaType;
import org.springframework.web.client.RestTemplate;
public class PutSimpleExample {
static final String URL_UPDATE_EMPLOYEE = "http://localhost:8080/employee";
static final String URL_EMPLOYEE_PREFIX = "http://localhost:8080/employee";
public static void main(String[] args) {
String empNo = "E01";
Employee updateInfo = new Employee(empNo, "Tom", "Cleck");
HttpHeaders headers = new HttpHeaders();
headers.add("Accept", MediaType.APPLICATION_JSON_VALUE);
RestTemplate restTemplate = new RestTemplate();
// Data attached to the request.
HttpEntity<Employee> requestBody = new HttpEntity<>(updateInfo, headers);
// Send request with PUT method.
restTemplate.put(URL_UPDATE_EMPLOYEE, requestBody, new Object[] {});
String resourceUrl = URL_EMPLOYEE_PREFIX + "/" + empNo;
Employee e = restTemplate.getForObject(resourceUrl, Employee.class);
if (e != null) {
System.out.println("(Client side) Employee after update: ");
System.out.println("Employee: " + e.getEmpNo() + " - " + e.getEmpName());
}
}
}
10. PUT - exchange
Example of using the exchange method of the RestTemplate class to send a request to the Restful Service to change a data resource.
PutWithExchangeExample.java
package org.o7planning.sbrestfulclient.put;
import org.o7planning.sbrestfulclient.model.Employee;
import org.springframework.http.HttpEntity;
import org.springframework.http.HttpHeaders;
import org.springframework.http.HttpMethod;
import org.springframework.http.MediaType;
import org.springframework.web.client.RestTemplate;
public class PutWithExchangeExample {
static final String URL_UPDATE_EMPLOYEE = "http://localhost:8080/employee";
static final String URL_EMPLOYEE_PREFIX = "http://localhost:8080/employee";
public static void main(String[] args) {
String empNo = "E01";
Employee updateInfo = new Employee(empNo, "Tom", "Cleck");
HttpHeaders headers = new HttpHeaders();
headers.add("Accept", MediaType.APPLICATION_JSON_VALUE);
RestTemplate restTemplate = new RestTemplate();
// Data attached to the request.
HttpEntity<Employee> requestBody = new HttpEntity<>(updateInfo, headers);
// Send request with PUT method.
restTemplate.exchange(URL_UPDATE_EMPLOYEE, HttpMethod.PUT, requestBody, Void.class);
String resourceUrl = URL_EMPLOYEE_PREFIX + "/" + empNo;
Employee e = restTemplate.getForObject(resourceUrl, Employee.class);
if (e != null) {
System.out.println("(Client side) Employee after update: ");
System.out.println("Employee: " + e.getEmpNo() + " - " + e.getEmpName());
}
}
}
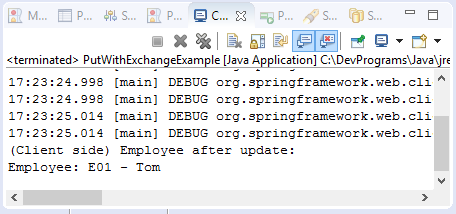
11. DELELE
Use the delete method of the RestTemplate class to send a request to the Restful Service to delete a data resource.
DeleteSimpleExample.java
package org.o7planning.sbrestfulclient.delete;
import org.o7planning.sbrestfulclient.model.Employee;
import org.springframework.web.client.RestTemplate;
public class DeleteSimpleExample {
public static void main(String[] args) {
RestTemplate restTemplate = new RestTemplate();
// empNo="E01"
String resourceUrl = "http://localhost:8080/employee/E01";
// Send request with DELETE method.
restTemplate.delete(resourceUrl);
// Get
Employee e = restTemplate.getForObject(resourceUrl, Employee.class);
if (e != null) {
System.out.println("(Client side) Employee after delete: ");
System.out.println("Employee: " + e.getEmpNo() + " - " + e.getEmpName());
} else {
System.out.println("Employee not found!");
}
}
}
DeleteExample2.java
package org.o7planning.sbrestfulclient.delete;
import org.o7planning.sbrestfulclient.model.Employee;
import org.springframework.web.client.RestTemplate;
public class DeleteExample2 {
public static void main(String[] args) {
RestTemplate restTemplate = new RestTemplate();
// URL with URI-variable
String resourceUrl = "http://localhost:8080/employee/{empNo}";
Object[] uriValues = new Object[] { "E01" };
// Send request with DELETE method.
restTemplate.delete(resourceUrl, uriValues);
Employee e = restTemplate.getForObject(resourceUrl, Employee.class);
if (e != null) {
System.out.println("(Client side) Employee after delete: ");
System.out.println("Employee: " + e.getEmpNo() + " - " + e.getEmpName());
} else {
System.out.println("Employee not found!");
}
}
}
Java Web Services Tutorials
- What are RESTful Web Services?
- Java RESTful Web Services Tutorial for Beginners
- Simple CRUD example with Java RESTful Web Service
- Create Java RESTful Client with Jersey Client
- RESTClient A Debugger for RESTful Web Services
- Simple CRUD example with Spring MVC RESTful Web Service
- CRUD Restful Web Service Example with Spring Boot
- Spring Boot Restful Client with RestTemplate Example
- Secure Spring Boot RESTful Service using Basic Authentication
Show More
Spring Boot Tutorials
- Install Spring Tool Suite for Eclipse
- Spring Tutorial for Beginners
- Spring Boot Tutorial for Beginners
- Spring Boot Common Properties
- Spring Boot and Thymeleaf Tutorial with Examples
- Spring Boot and FreeMarker Tutorial with Examples
- Spring Boot and Groovy Tutorial with Examples
- Spring Boot and Mustache Tutorial with Examples
- Spring Boot and JSP Tutorial with Examples
- Spring Boot, Apache Tiles, JSP Tutorial with Examples
- Use Logging in Spring Boot
- Application Monitoring with Spring Boot Actuator
- Create a Multi Language web application with Spring Boot
- Use multiple ViewResolvers in Spring Boot
- Use Twitter Bootstrap in Spring Boot
- Spring Boot Interceptors Tutorial with Examples
- Spring Boot, Spring JDBC and Spring Transaction Tutorial with Examples
- Spring JDBC Tutorial with Examples
- Spring Boot, JPA and Spring Transaction Tutorial with Examples
- Spring Boot and Spring Data JPA Tutorial with Examples
- Spring Boot, Hibernate and Spring Transaction Tutorial with Examples
- Integrating Spring Boot, JPA and H2 Database
- Spring Boot and MongoDB Tutorial with Examples
- Use Multiple DataSources with Spring Boot and JPA
- Use Multiple DataSources with Spring Boot and RoutingDataSource
- Create a Login Application with Spring Boot, Spring Security, Spring JDBC
- Create a Login Application with Spring Boot, Spring Security, JPA
- Create a User Registration Application with Spring Boot, Spring Form Validation
- Example of OAuth2 Social Login in Spring Boot
- Run background scheduled tasks in Spring
- CRUD Restful Web Service Example with Spring Boot
- Spring Boot Restful Client with RestTemplate Example
- CRUD Example with Spring Boot, REST and AngularJS
- Secure Spring Boot RESTful Service using Basic Authentication
- Secure Spring Boot RESTful Service using Auth0 JWT
- Spring Boot File Upload Example
- Spring Boot File Download Example
- Spring Boot File Upload with jQuery Ajax Example
- Spring Boot File Upload with AngularJS Example
- Create a Shopping Cart Web Application with Spring Boot, Hibernate
- Spring Email Tutorial with Examples
- Create a simple Chat application with Spring Boot and Websocket
- Deploy Spring Boot Application on Tomcat Server
- Deploy Spring Boot Application on Oracle WebLogic Server
- Install a free Let's Encrypt SSL certificate for Spring Boot
- Configure Spring Boot to redirect HTTP to HTTPS
- Fetch data with Spring Data JPA DTO Projections
Show More