Deploy Spring Boot Application on Oracle WebLogic Server
1. Objective of post
In this post, I will show you how to deploy a Spring Boot application on a WebLogic Server. This is a Web Server provided by Oracle, free of charge for downloading, developing, testing, prototyping, and demonstrating your application. The WebLogic is released based on the OTN license.
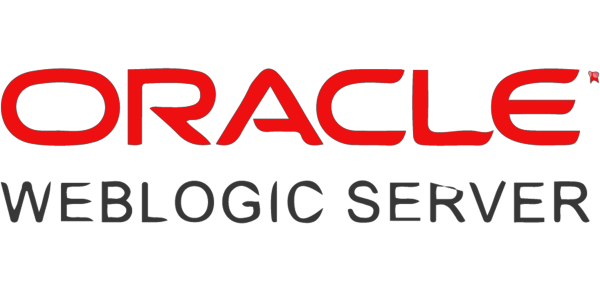
Ensure that you have installed the WebLogic successfully. If not, you can refer to the way of installing WebLogic as in the following post:
In fact, Spring Boot application can be packaged into an Executable JAR file. With this file, you can run the application directly without deploying it on any Web Server. The Executable JAR file can not be compatible with all Web servers, therefore, if you want to deploy it on a Web server, you need to package the Spring Boot application into a WAR file.
2. Edit code
I has a Spring Boot project here, developed with Eclipse IDE. When creating a Spring Boot, you have 2 choices to package this project such as WAR or JAR.
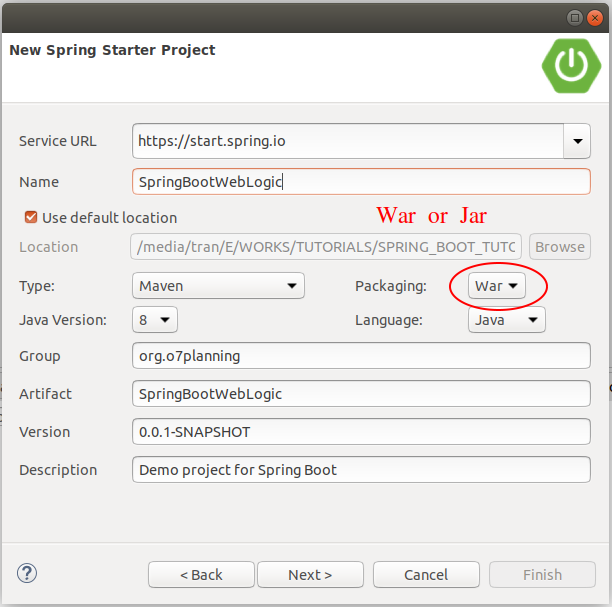
When you create a Spring Boot project with "Packaging = War" option, Eclipse will create 2 classes such as "AbcApplication & ServletInitializer" for you. WAR files are suitable for being deployed on Web Servers.
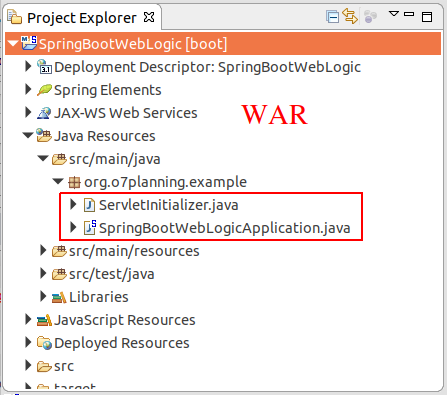
While, if you create a Spring Boot with the "Packaging = Jar" choice, only one AbcApplication class will be created. When the Spring Boot application is packed into the JAR file, it can be independently executabe without being deployed on any Web Server. But the JAR file is not suitable to be deployed on Web Servers.
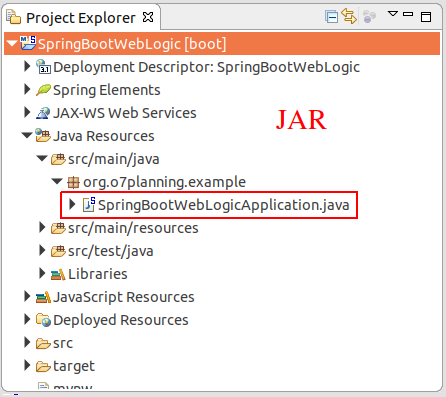
WAR File.
If you have an available Spring Boot, you need to correct packaging method into WAR:
On the Eclipse, open the pom.xml file, and change packaging method into war.
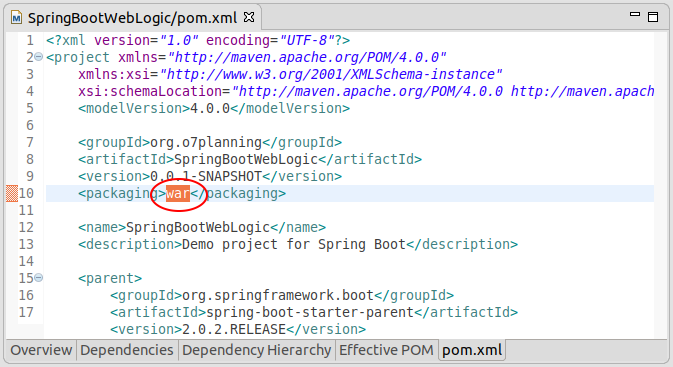
If your project doesn't have a ServletInitializer class, create it.
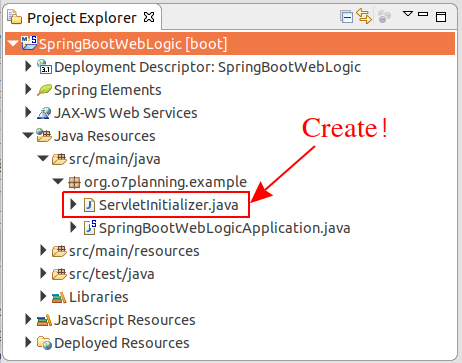
ServletInitializer.java
package org.o7planning.example;
import org.springframework.boot.builder.SpringApplicationBuilder;
import org.springframework.boot.web.servlet.support.SpringBootServletInitializer;
public class ServletInitializer extends SpringBootServletInitializer {
@Override
protected SpringApplicationBuilder configure(SpringApplicationBuilder application) {
return application.sources(SpringBootWebLogicApplication.class);
}
}
Add the following configuration snippet to the pom.xml:
** pom.xml **
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-tomcat</artifactId>
<scope>provided</scope>
</dependency>
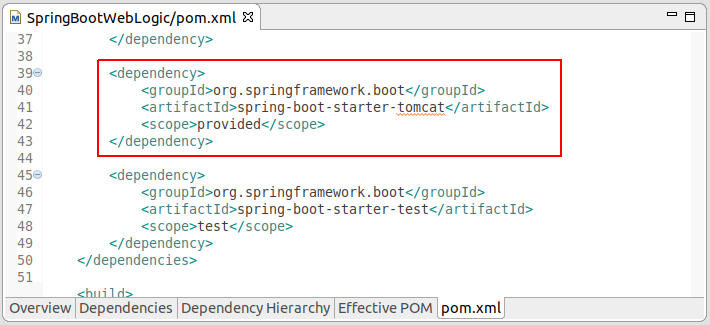
If there are more than one Application class in your project, please tell the Spring Boot which class will be used for your application.
** pom.xml **
<properties>
<start-class>org.o7planning.example.OtherSpringBootWebApplication</start-class>
</properties>
weblogic.xml & dispatcherServlet-servlet.xml
Create the 2 files such as weblogic.xml & dispatcherServlet-servlet.xml in the src/main/webapp/WEB-INF folder. It is noted that if this folder doesn't exist, please create it.
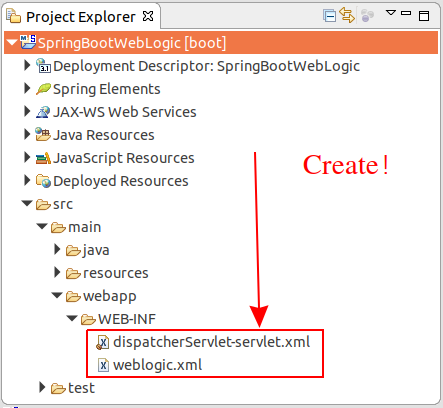
When your application is deployed on the WebLogic, there will be "context-root = /myweb", and you can change it by installing in the weblogic.xml file:
weblogic.xml
<?xml version="1.0" encoding="UTF-8"?>
<wls:weblogic-web-app
xmlns:wls="http://xmlns.oracle.com/weblogic/weblogic-web-app"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://xmlns.oracle.com/weblogic/weblogic-web-app
http://xmlns.oracle.com/weblogic/weblogic-web-app/1.4/weblogic-web-app.xsd">
<wls:context-root>/myweb</wls:context-root>
<wls:container-descriptor>
<wls:prefer-application-packages>
<wls:package-name>org.slf4j.*</wls:package-name>
<wls:package-name>org.springframework.*</wls:package-name>
</wls:prefer-application-packages>
</wls:container-descriptor>
</wls:weblogic-web-app>
dispatcherServlet-servlet.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd">
</beans>
3. Install Spring Boot
In the next step, you need to use Maven to create WAR file. You have to ensure that your Eclipse is using JDK instead of JRE. If not, en error will occur in this process.
Right click on the Project and select:
- Run As/Maven Install
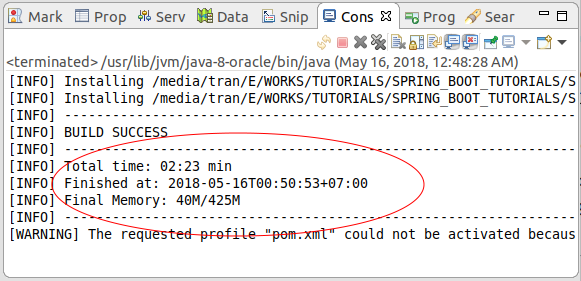
At this moment, you will have a WAR file in the target folder of the project, you can use this file to deploy on the Tomcat Server.
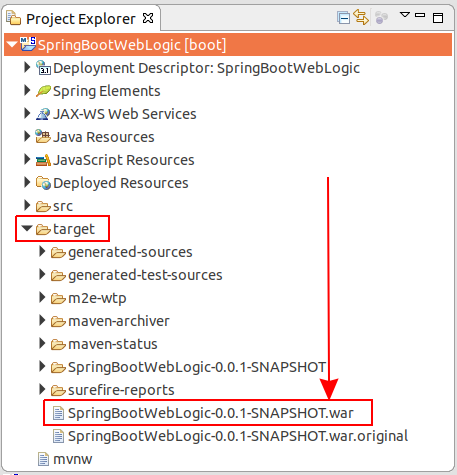
4. Deploy on WebLogic
After packaging the application into a WAR file, you can deploy this file on the WebLogic:
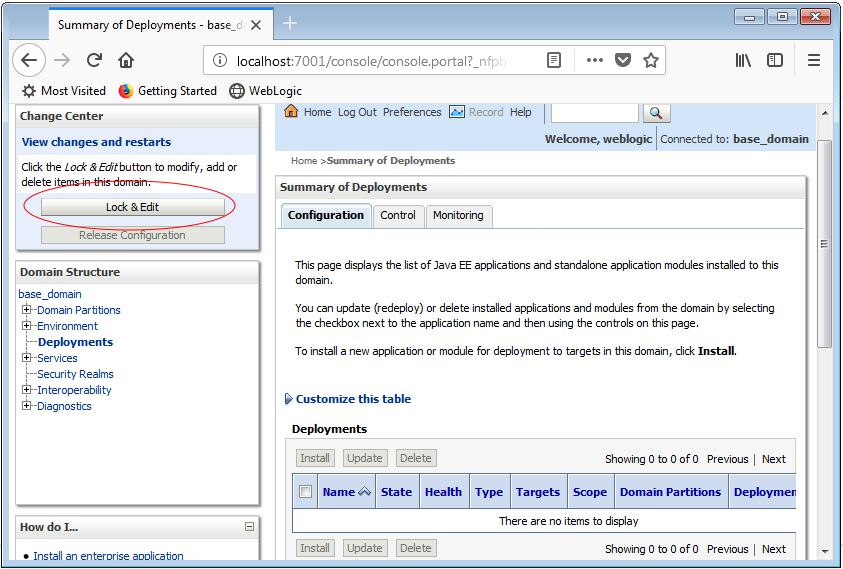
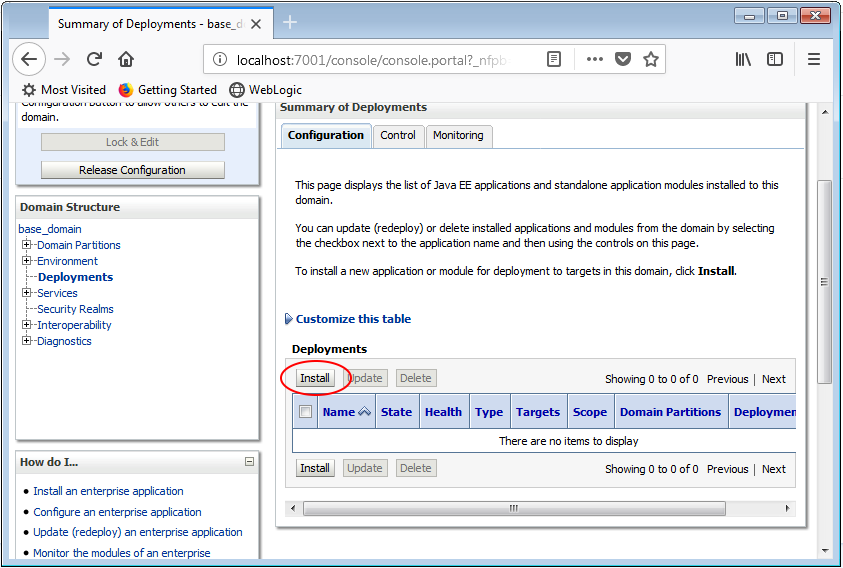
There are two approaches to deploy the application to the WebLogic Server.
- Copy the WAR file to a folder of the server, and then deploy it to the WebLogic. If you want to re-deploy the application, you only need to copy the new WAR file overwriting the old file and notify to the WebLogic to update the application.
- Use Upload function to deploy the WAR file.
OK, here, I have copied the WAR file and install it to a folder in the server.
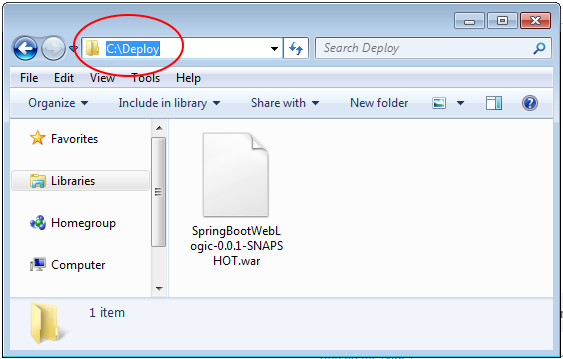
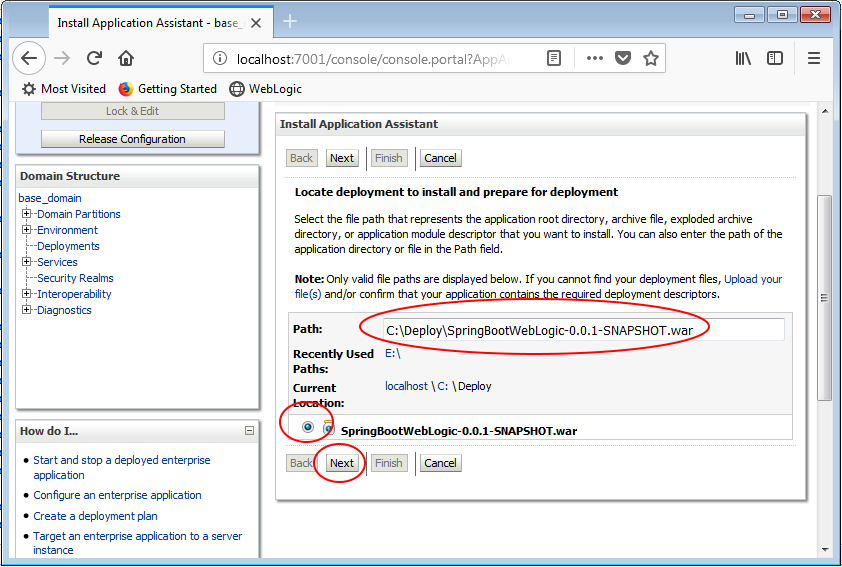
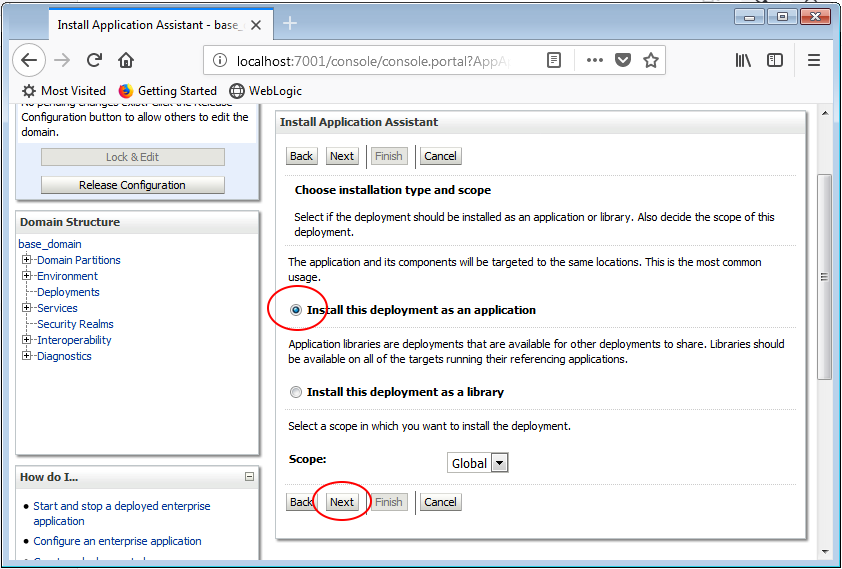
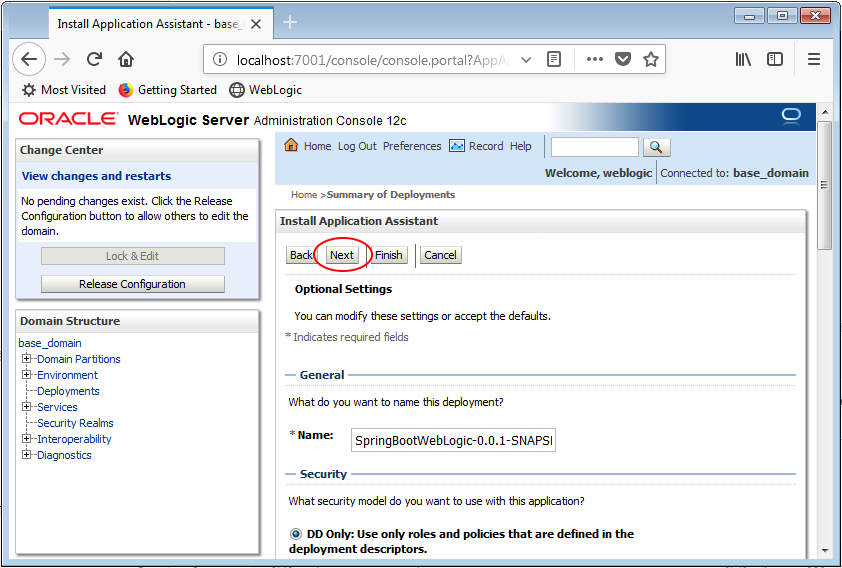
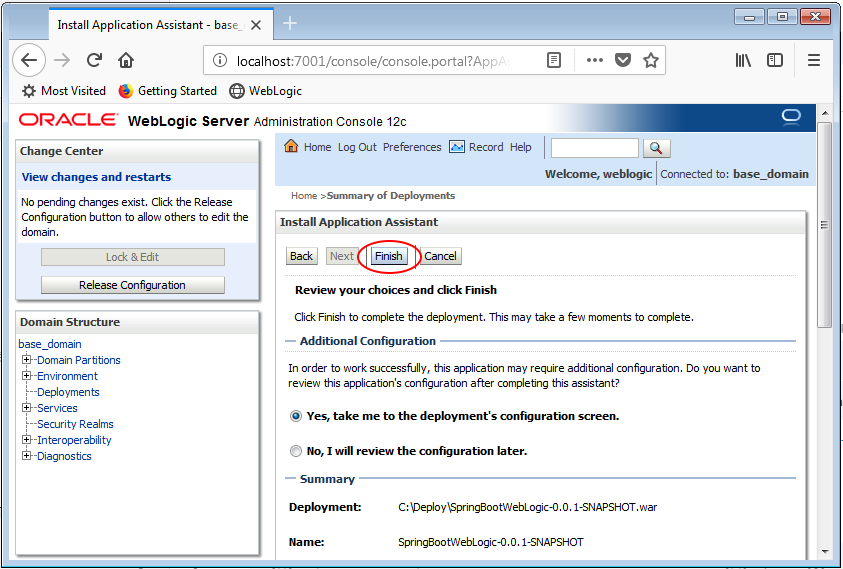
Press "Activate Changes" to activate changes.
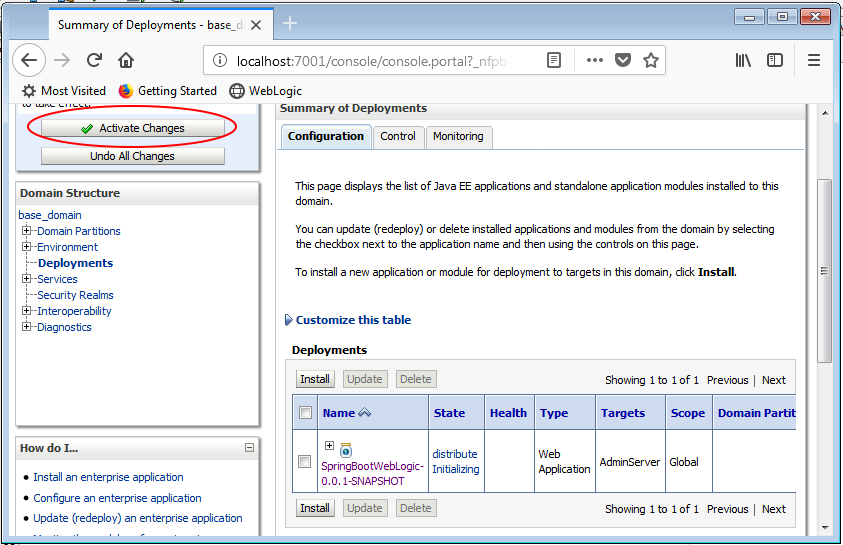
At this moment, your application has "Prepared" status (prepared). It isn't really activated to work.
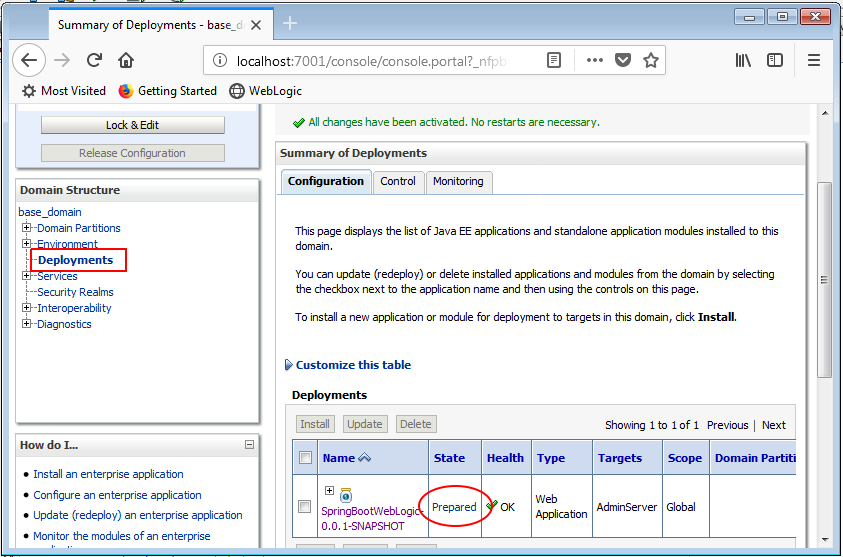
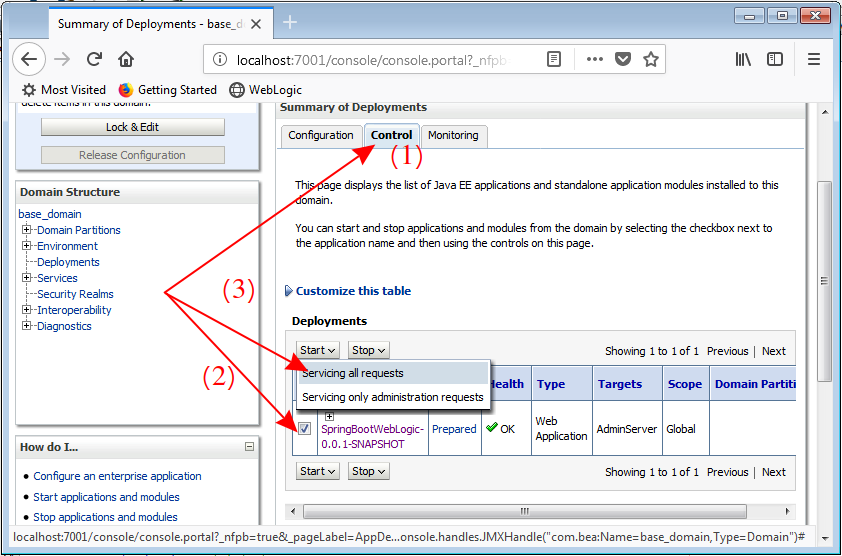
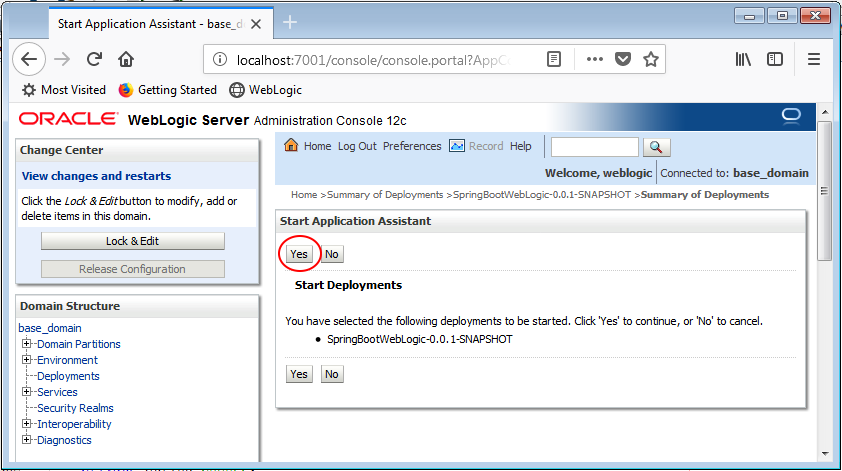
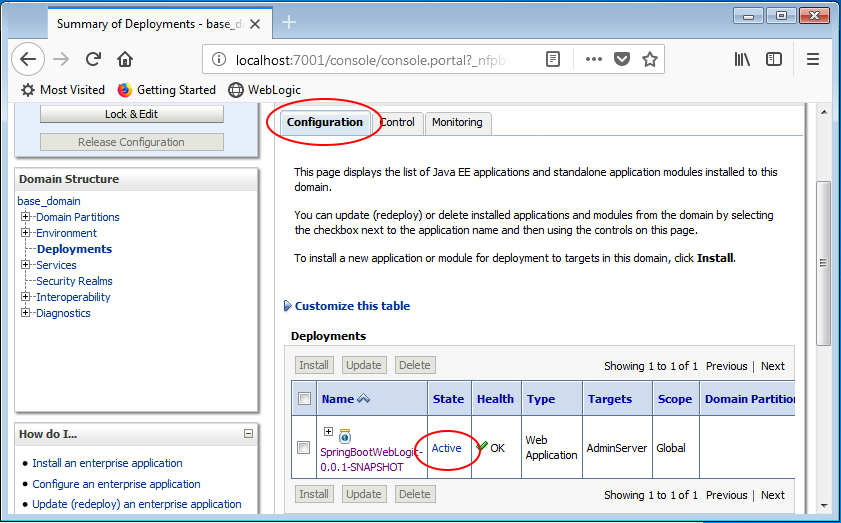
Test
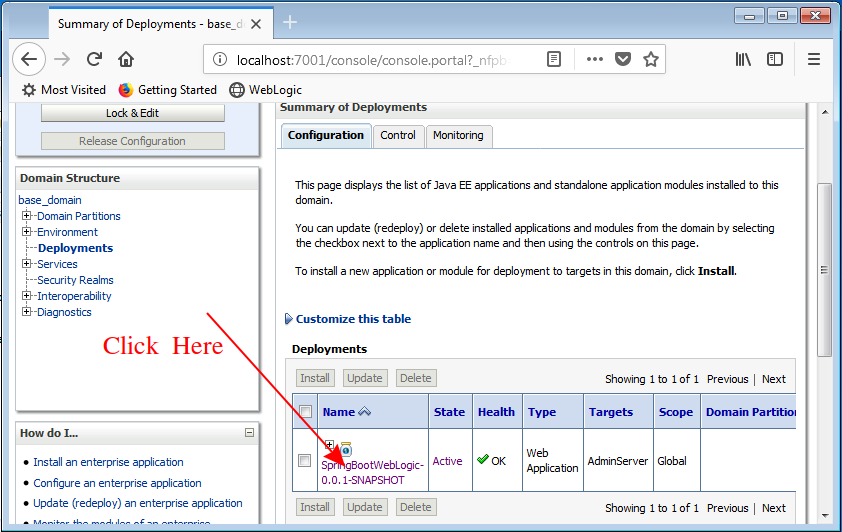
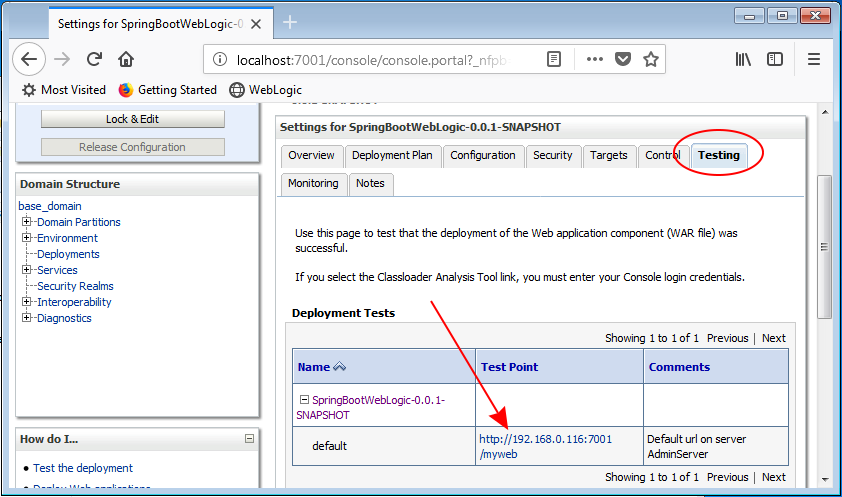
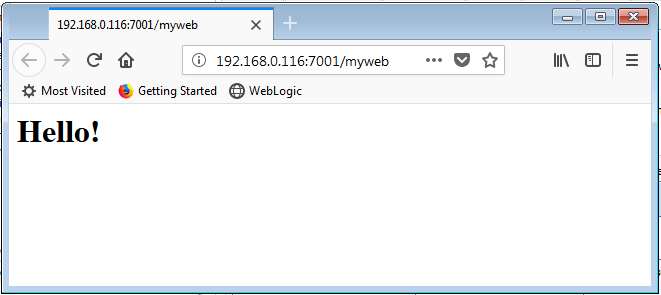
5. Undeploy
Undeploy an application on the WebLogic, you must carry out 2 steps.
- Stop the application.
- Delete the application out of the WebLogic.
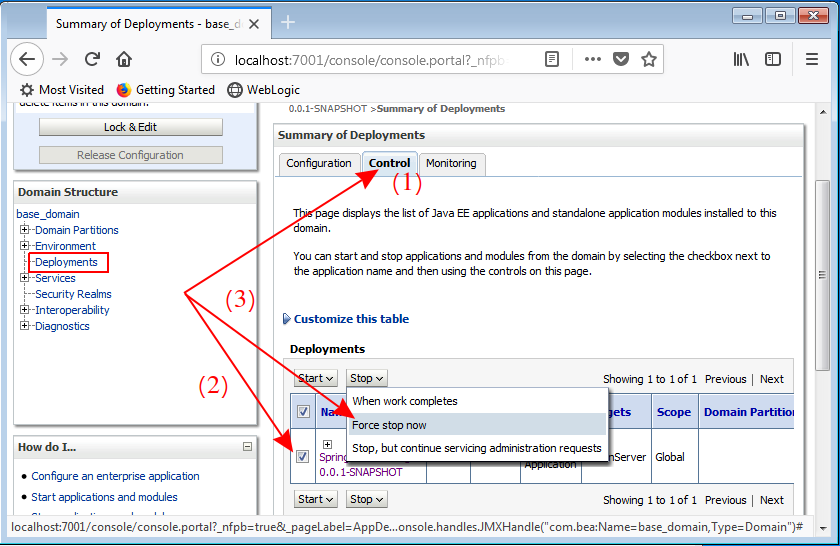
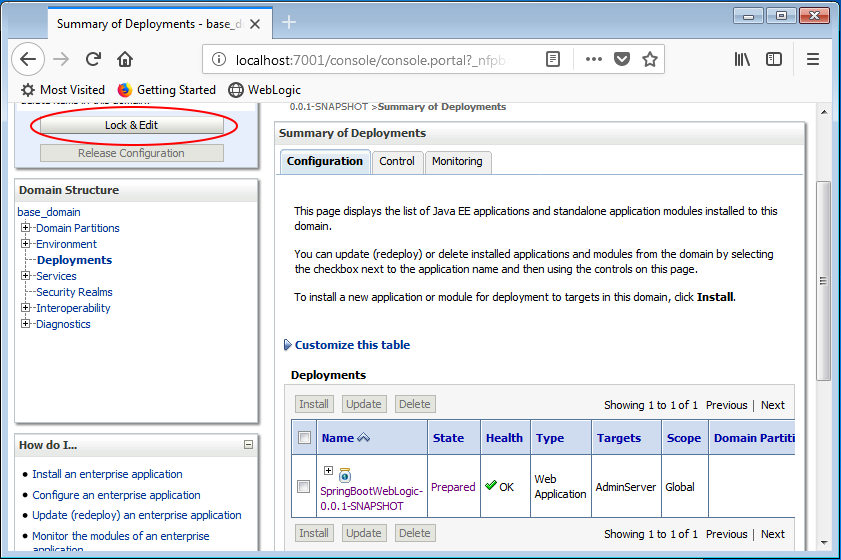
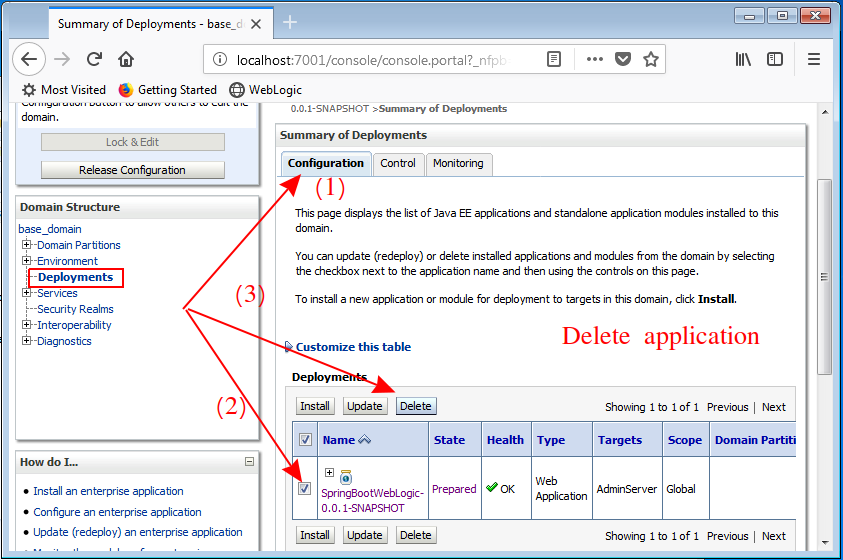
6. Update deployment
If your application is upgraded, you repackage the application and get a new WAR file. To redeploy the application, you have 2 ways to do:
- Undeploy the application and Redeploy.
- Copy a new WAR file overwriting the old WAR file and notify to the WebLogic to update the application again (or restart the WebLogic). Note: This way is used only when your application is deployed directly from the WAR file put on the server.
In the previous step, I have deployed the application directly from the WAR file placed on an folder of the server. Now I will copy the new file to overwrite the old file.
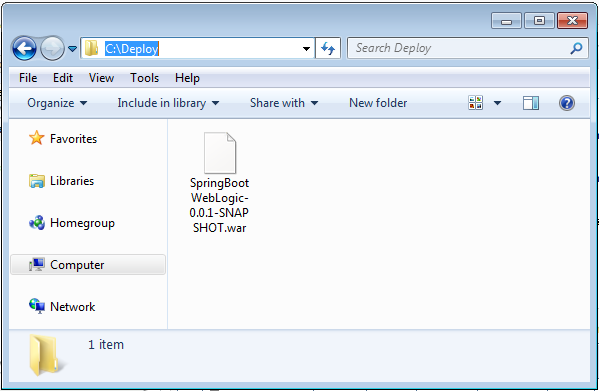
And update the application:
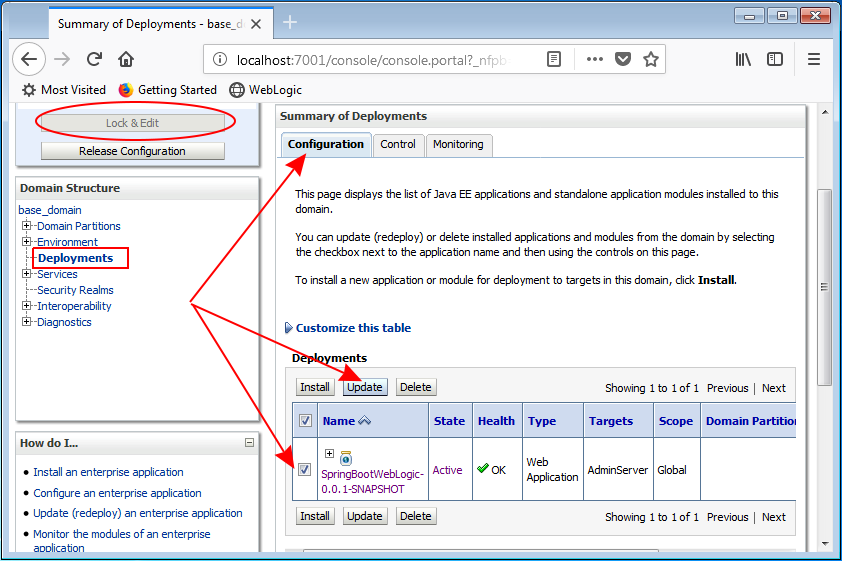
Java Application Servers Tutorials
- Install Tomcat Server for Eclipse
- Install Tomcat Server
- Install Glassfish Web Server on Windows
- Install Oracle WebLogic Server
- How to create Windows Service for Oracle WebLogic Server?
- Deploy Spring Boot Application on Tomcat Server
- Deploy Spring Boot Application on Oracle WebLogic Server
- Deploy Spring MVC on Oracle WebLogic Server
- Install SSL Certificate for Tomcat Server
- Install a free SSL certificate Let's Encrypt for Tomcat Server on Ubuntu
Show More
Spring Boot Tutorials
- Install Spring Tool Suite for Eclipse
- Spring Tutorial for Beginners
- Spring Boot Tutorial for Beginners
- Spring Boot Common Properties
- Spring Boot and Thymeleaf Tutorial with Examples
- Spring Boot and FreeMarker Tutorial with Examples
- Spring Boot and Groovy Tutorial with Examples
- Spring Boot and Mustache Tutorial with Examples
- Spring Boot and JSP Tutorial with Examples
- Spring Boot, Apache Tiles, JSP Tutorial with Examples
- Use Logging in Spring Boot
- Application Monitoring with Spring Boot Actuator
- Create a Multi Language web application with Spring Boot
- Use multiple ViewResolvers in Spring Boot
- Use Twitter Bootstrap in Spring Boot
- Spring Boot Interceptors Tutorial with Examples
- Spring Boot, Spring JDBC and Spring Transaction Tutorial with Examples
- Spring JDBC Tutorial with Examples
- Spring Boot, JPA and Spring Transaction Tutorial with Examples
- Spring Boot and Spring Data JPA Tutorial with Examples
- Spring Boot, Hibernate and Spring Transaction Tutorial with Examples
- Integrating Spring Boot, JPA and H2 Database
- Spring Boot and MongoDB Tutorial with Examples
- Use Multiple DataSources with Spring Boot and JPA
- Use Multiple DataSources with Spring Boot and RoutingDataSource
- Create a Login Application with Spring Boot, Spring Security, Spring JDBC
- Create a Login Application with Spring Boot, Spring Security, JPA
- Create a User Registration Application with Spring Boot, Spring Form Validation
- Example of OAuth2 Social Login in Spring Boot
- Run background scheduled tasks in Spring
- CRUD Restful Web Service Example with Spring Boot
- Spring Boot Restful Client with RestTemplate Example
- CRUD Example with Spring Boot, REST and AngularJS
- Secure Spring Boot RESTful Service using Basic Authentication
- Secure Spring Boot RESTful Service using Auth0 JWT
- Spring Boot File Upload Example
- Spring Boot File Download Example
- Spring Boot File Upload with jQuery Ajax Example
- Spring Boot File Upload with AngularJS Example
- Create a Shopping Cart Web Application with Spring Boot, Hibernate
- Spring Email Tutorial with Examples
- Create a simple Chat application with Spring Boot and Websocket
- Deploy Spring Boot Application on Tomcat Server
- Deploy Spring Boot Application on Oracle WebLogic Server
- Install a free Let's Encrypt SSL certificate for Spring Boot
- Configure Spring Boot to redirect HTTP to HTTPS
- Fetch data with Spring Data JPA DTO Projections
Show More