Spring Boot and Thymeleaf Tutorial with Examples
1. What is Thymeleaf?
Thymeleaf is a Java XML/XHTML/HTML5 Template Engine that can work both in web (Servlet-based) and non-web environments. It is better suited for serving XHTML/HTML5 at the view layer of MVC-based web applications, but it can process any XML file even in offline environments. It provides full Spring Framework integration.
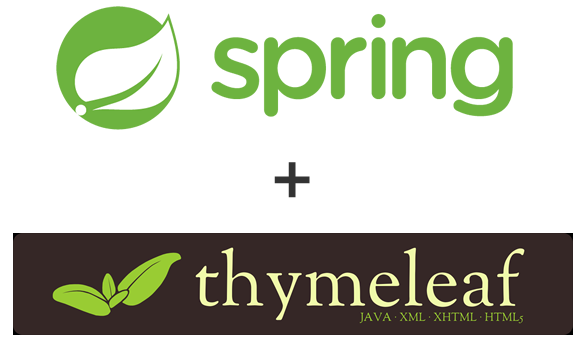
The template file of Thymeleaf in essence are only a ordinary document file with the format of XML/XHTML/HTML5. Thymeleaf Engine will read a template file and combine with Java objects to generate another document.
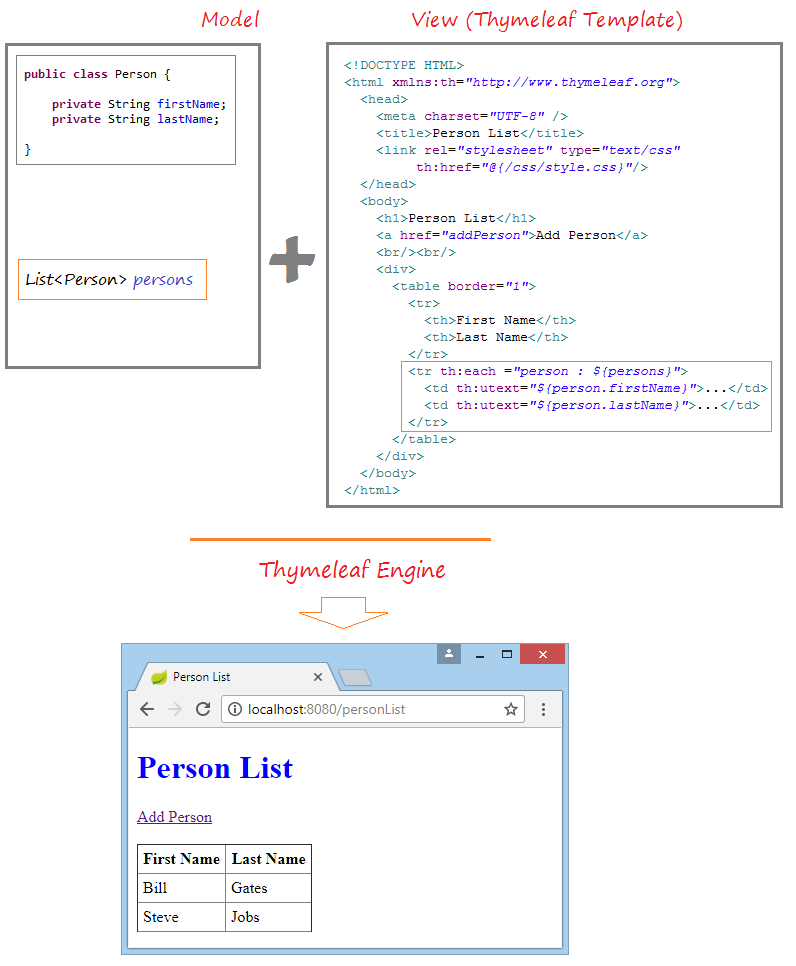
Thymeleaf can be used to replace JSP on the View Layer of Web MVC application. Thymeleaf is open source code software, licensed under theApache 2.0.
Below is the image of the application that we will perform in this lesson:
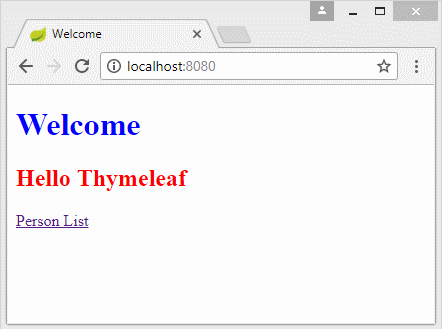
See Also:
2. Create application
On Eclipse, select:
- File/New/Other...
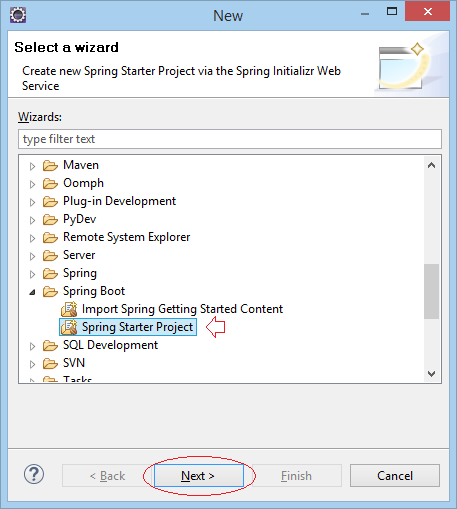
Enter:
- Name: SpringBootThymeleaf
- Group: org.o7planning
- Artifact: SpringBootThymeleaf
- Description: Spring Boot and Thymeleaf
- Package: org.o7planning.thymeleaf
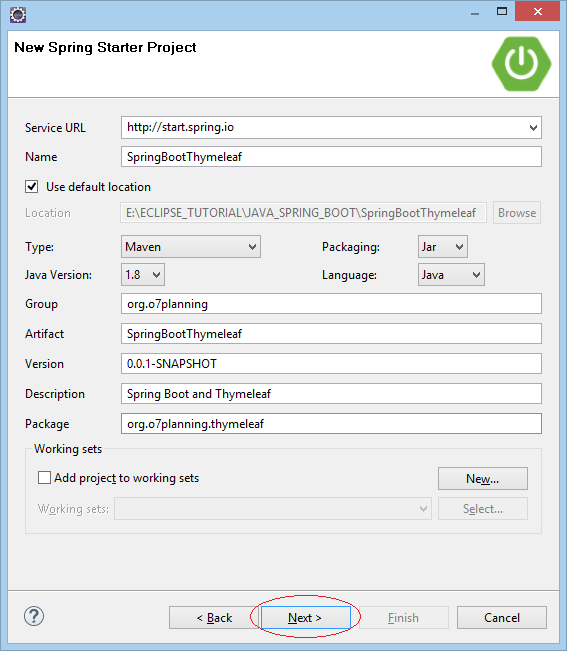
Select 2 Web and Thymeleaf technologies.
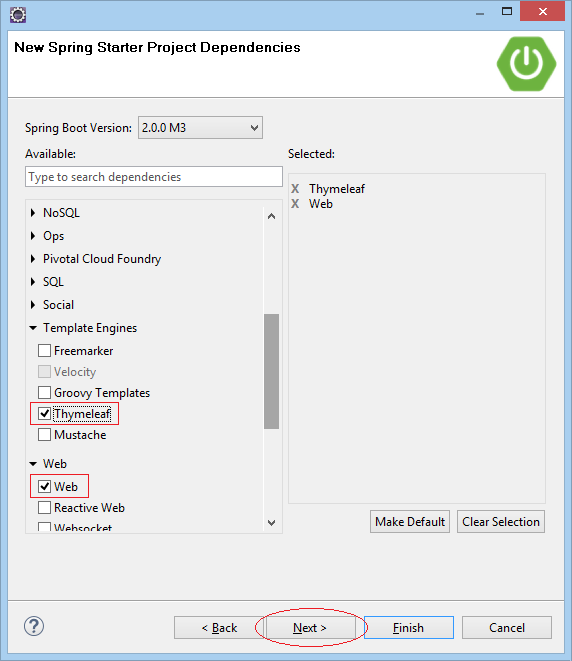
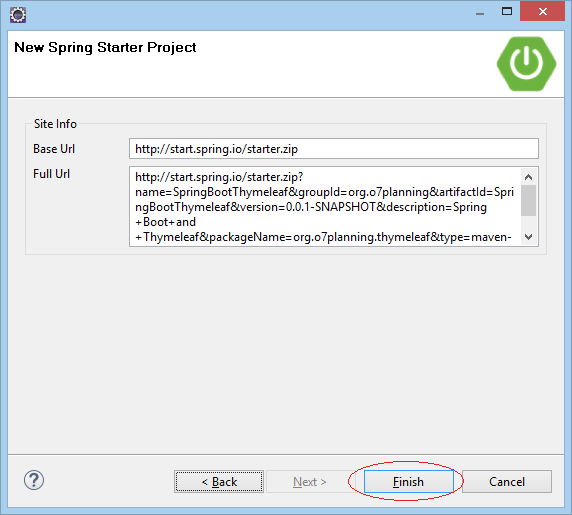
Your Project has been created:
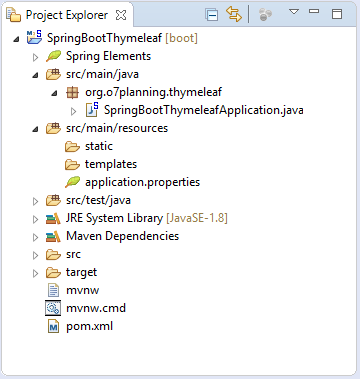
pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0
http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.o7planning</groupId>
<artifactId>SpringBootThymeleaf</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>jar</packaging>
<name>SpringBootThymeleaf</name>
<description>Spring Boot and Thymeleaf</description>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.0.0.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
3. Thymeleaf Template
Thymeleaf Template is a template file. Its contents are in the XML/XHTML/HTML5 format. We will create 3 files and place it in the src/main/resources/templates folder:
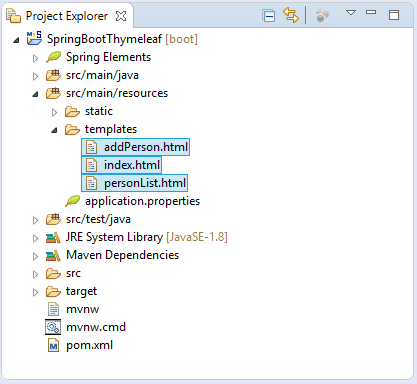
index.html
<!DOCTYPE HTML>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8" />
<title>Welcome</title>
<link rel="stylesheet" type="text/css" th:href="@{/css/style.css}"/>
</head>
<body>
<h1>Welcome</h1>
<h2 th:utext="${message}">..!..</h2>
<!--
In Thymeleaf the equivalent of
JSP's ${pageContext.request.contextPath}/edit.html
would be @{/edit.html}
-->
<a th:href="@{/personList}">Person List</a>
</body>
</html>
personList.html
<!DOCTYPE HTML>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8" />
<title>Person List</title>
<link rel="stylesheet" type="text/css" th:href="@{/css/style.css}"/>
</head>
<body>
<h1>Person List</h1>
<a href="addPerson">Add Person</a>
<br/><br/>
<div>
<table border="1">
<tr>
<th>First Name</th>
<th>Last Name</th>
</tr>
<tr th:each ="person : ${persons}">
<td th:utext="${person.firstName}">...</td>
<td th:utext="${person.lastName}">...</td>
</tr>
</table>
</div>
</body>
</html>
addPerson.html
<!DOCTYPE HTML>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8" />
<title>Add Person</title>
<link rel="stylesheet" type="text/css" th:href="@{/css/style.css}"/>
</head>
<body>
<h1>Create a Person:</h1>
<!--
In Thymeleaf the equivalent of
JSP's ${pageContext.request.contextPath}/edit.html
would be @{/edit.html}
-->
<form th:action="@{/addPerson}"
th:object="${personForm}" method="POST">
First Name:
<input type="text" th:field="*{firstName}" />
<br/>
Last Name:
<input type="text" th:field="*{lastName}" />
<br/>
<input type="submit" value="Create" />
</form>
<br/>
<!-- Check if errorMessage is not null and not empty -->
<div th:if="${errorMessage}" th:utext="${errorMessage}"
style="color:red;font-style:italic;">
...
</div>
</body>
</html>
I have created 3 HTML files above. The above HTML files need to be appropriate to the standards of XML. All tags must have open and close, for example:
<div>A div tag</div>
<br />
<meta charset="UTF-8" />
All HTML files need declaring use of Thymeleaf Namespace:
<!-- Thymeleaf Namespace -->
<html xmlns:th="http://www.thymeleaf.org">
In template files, there are Thymeleaf Markers (markers of Thymeleaf) which are instructions helping Thymeleaf Engine process data.
Thymeleaf Engine analyses template files and combines them with Java data to generate a new document.
Thymeleaf Engine analyses template files and combines them with Java data to generate a new document.
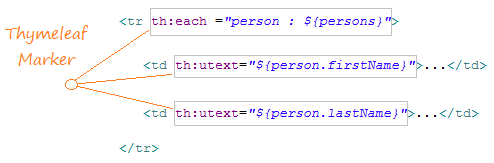
Below are examples to use the Context-Path in the Thymeleaf:<!-- Example 1: --> <a th:href="@{/mypath/abc.html}">A Link</a> Output: ==> <a href="/my-context-path/mypath/abc.html">A Link</a> <!-- Example 2: --> <form th:action="@{/mypath/abc.html}" th:object="${personForm}" method="POST"> Output: ==> <form action="/my-context-path/mypath/abc.html" method="POST">
4. Static Resource & Properties File
For Static Resources, for example, css, javascript, image files,.. you need to put them into src/main/resources/static folder or its subfolders.
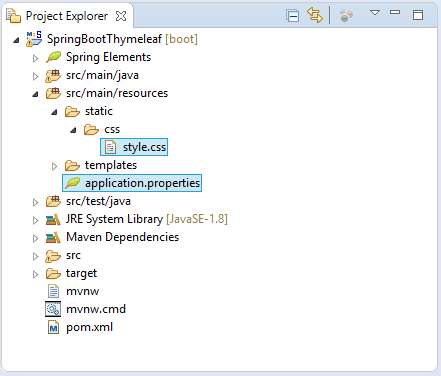
style.css
h1 {
color:#0000FF;
}
h2 {
color:#FF0000;
}
table {
border-collapse: collapse;
}
table th, table td {
padding: 5px;
}
application.properties
spring.thymeleaf.cache=false
welcome.message=Hello Thymeleaf
error.message=First Name & Last Name is required!
5. Model, Form, Controller classes
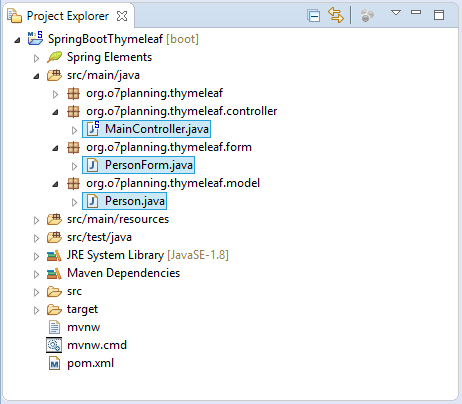
Person.java
package org.o7planning.thymeleaf.model;
public class Person {
private String firstName;
private String lastName;
public Person() {
}
public Person(String firstName, String lastName) {
this.firstName = firstName;
this.lastName = lastName;
}
public String getFirstName() {
return firstName;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public String getLastName() {
return lastName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
}
PersonForm classrepresents for the data ofFORM when you create a new Person on addPerson page..
PersonForm.java
package org.o7planning.thymeleaf.form;
public class PersonForm {
private String firstName;
private String lastName;
public String getFirstName() {
return firstName;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public String getLastName() {
return lastName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
}
MainController is a controller class. It processes a user's requests and controls the flow of the application.
MainController.java
package org.o7planning.thymeleaf.controller;
import java.util.ArrayList;
import java.util.List;
import org.o7planning.thymeleaf.form.PersonForm;
import org.o7planning.thymeleaf.model.Person;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.ModelAttribute;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
@Controller
public class MainController {
private static List<Person> persons = new ArrayList<Person>();
static {
persons.add(new Person("Bill", "Gates"));
persons.add(new Person("Steve", "Jobs"));
}
// Inject via application.properties
@Value("${welcome.message}")
private String message;
@Value("${error.message}")
private String errorMessage;
@RequestMapping(value = { "/", "/index" }, method = RequestMethod.GET)
public String index(Model model) {
model.addAttribute("message", message);
return "index";
}
@RequestMapping(value = { "/personList" }, method = RequestMethod.GET)
public String personList(Model model) {
model.addAttribute("persons", persons);
return "personList";
}
@RequestMapping(value = { "/addPerson" }, method = RequestMethod.GET)
public String showAddPersonPage(Model model) {
PersonForm personForm = new PersonForm();
model.addAttribute("personForm", personForm);
return "addPerson";
}
@RequestMapping(value = { "/addPerson" }, method = RequestMethod.POST)
public String savePerson(Model model, //
@ModelAttribute("personForm") PersonForm personForm) {
String firstName = personForm.getFirstName();
String lastName = personForm.getLastName();
if (firstName != null && firstName.length() > 0 //
&& lastName != null && lastName.length() > 0) {
Person newPerson = new Person(firstName, lastName);
persons.add(newPerson);
return "redirect:/personList";
}
model.addAttribute("errorMessage", errorMessage);
return "addPerson";
}
}
Spring Boot Tutorials
- Install Spring Tool Suite for Eclipse
- Spring Tutorial for Beginners
- Spring Boot Tutorial for Beginners
- Spring Boot Common Properties
- Spring Boot and Thymeleaf Tutorial with Examples
- Spring Boot and FreeMarker Tutorial with Examples
- Spring Boot and Groovy Tutorial with Examples
- Spring Boot and Mustache Tutorial with Examples
- Spring Boot and JSP Tutorial with Examples
- Spring Boot, Apache Tiles, JSP Tutorial with Examples
- Use Logging in Spring Boot
- Application Monitoring with Spring Boot Actuator
- Create a Multi Language web application with Spring Boot
- Use multiple ViewResolvers in Spring Boot
- Use Twitter Bootstrap in Spring Boot
- Spring Boot Interceptors Tutorial with Examples
- Spring Boot, Spring JDBC and Spring Transaction Tutorial with Examples
- Spring JDBC Tutorial with Examples
- Spring Boot, JPA and Spring Transaction Tutorial with Examples
- Spring Boot and Spring Data JPA Tutorial with Examples
- Spring Boot, Hibernate and Spring Transaction Tutorial with Examples
- Integrating Spring Boot, JPA and H2 Database
- Spring Boot and MongoDB Tutorial with Examples
- Use Multiple DataSources with Spring Boot and JPA
- Use Multiple DataSources with Spring Boot and RoutingDataSource
- Create a Login Application with Spring Boot, Spring Security, Spring JDBC
- Create a Login Application with Spring Boot, Spring Security, JPA
- Create a User Registration Application with Spring Boot, Spring Form Validation
- Example of OAuth2 Social Login in Spring Boot
- Run background scheduled tasks in Spring
- CRUD Restful Web Service Example with Spring Boot
- Spring Boot Restful Client with RestTemplate Example
- CRUD Example with Spring Boot, REST and AngularJS
- Secure Spring Boot RESTful Service using Basic Authentication
- Secure Spring Boot RESTful Service using Auth0 JWT
- Spring Boot File Upload Example
- Spring Boot File Download Example
- Spring Boot File Upload with jQuery Ajax Example
- Spring Boot File Upload with AngularJS Example
- Create a Shopping Cart Web Application with Spring Boot, Hibernate
- Spring Email Tutorial with Examples
- Create a simple Chat application with Spring Boot and Websocket
- Deploy Spring Boot Application on Tomcat Server
- Deploy Spring Boot Application on Oracle WebLogic Server
- Install a free Let's Encrypt SSL certificate for Spring Boot
- Configure Spring Boot to redirect HTTP to HTTPS
- Fetch data with Spring Data JPA DTO Projections
Show More