Maven Tutorial for Beginners
1. Introduction
We must first ensure that you have installed Maven into Eclipse. If not, you can see the installation instructions here:
The goal of the tutorial:
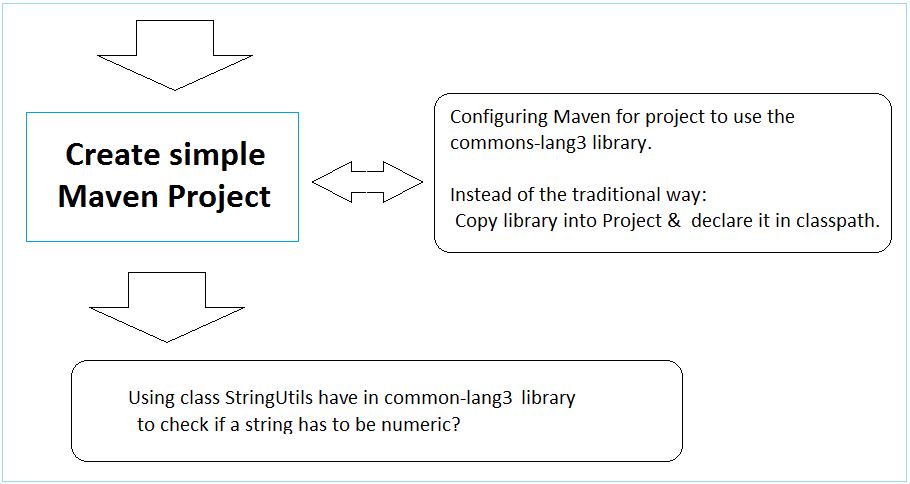
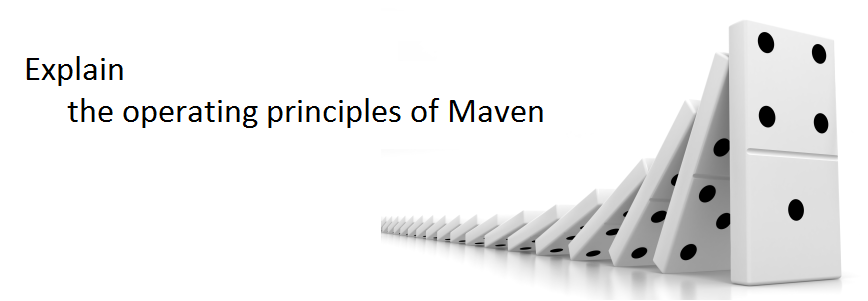
This is the image after Project completion:
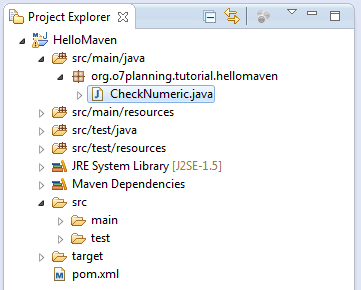
2. Create Maven Project
In Eclipse, Click "New/Other"
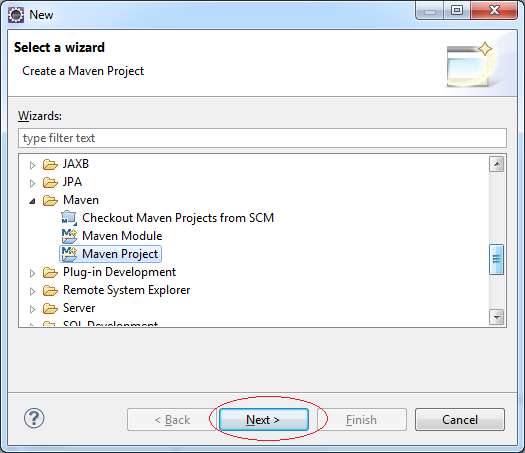
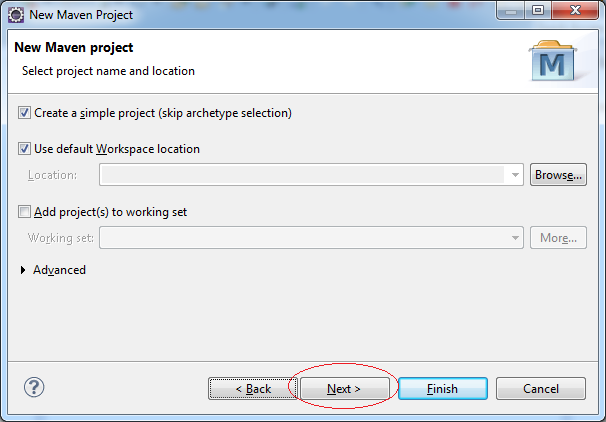
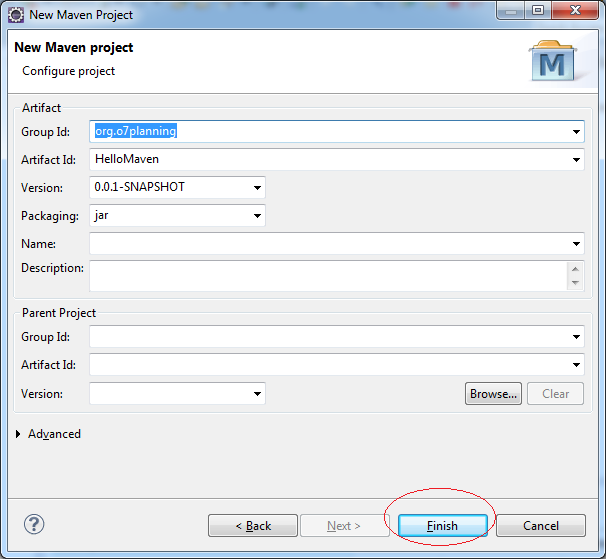
Project is created.
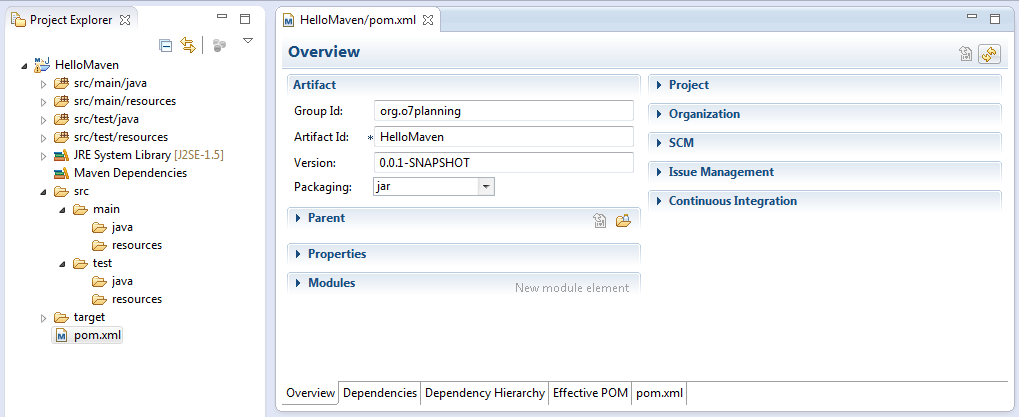
pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.o7planning</groupId>
<artifactId>HelloMaven</artifactId>
<version>0.0.1-SNAPSHOT</version>
</project>
3. Configure Maven
Open pom.xml file to configure the library will use:
pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.o7planning</groupId>
<artifactId>HelloMaven</artifactId>
<version>0.0.1-SNAPSHOT</version>
<dependencies>
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-lang3</artifactId>
<version>3.3.2</version>
</dependency>
</dependencies>
</project>
Create class CheckNumeric.java
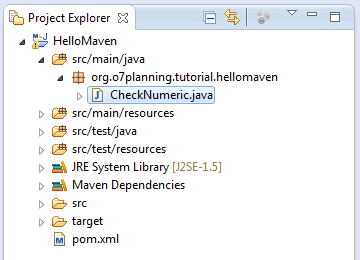
CheckNumeric.java
package org.o7planning.tutorial.hellomaven;
import org.apache.commons.lang3.StringUtils;
public class CheckNumeric {
public static void main(String[] args) {
String text1 = "0123a4";
String text2 = "01234";
boolean result1 = StringUtils.isNumeric(text1);
boolean result2 = StringUtils.isNumeric(text2);
System.out.println(text1 + " is a numeric? " + result1);
System.out.println(text2 + " is a numeric? " + result2);
}
}
Running CheckNumeric class and get results:
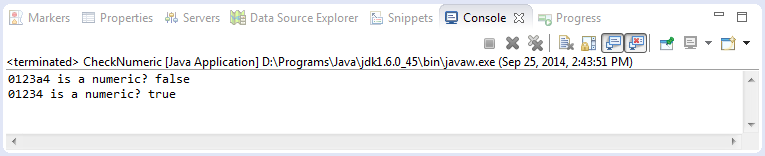
You can clearly see your project using the library. Where is its location on the hard drive.
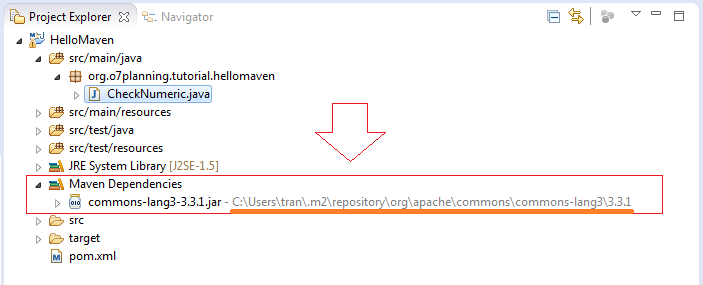
5. Explain the operating principles of Maven
Above you have created the project and ran flawlessly. The Project using StringUtils class, which to be a class of Apache, and not in the standard library of JDK. Traditionally you have to copy this library to project and declare the classpath.
However, the guidelines do not have to copy and declare classpath as the traditional way. The library was managed by Maven management. Now we will explain how Maven works:
However, the guidelines do not have to copy and declare classpath as the traditional way. The library was managed by Maven management. Now we will explain how Maven works:
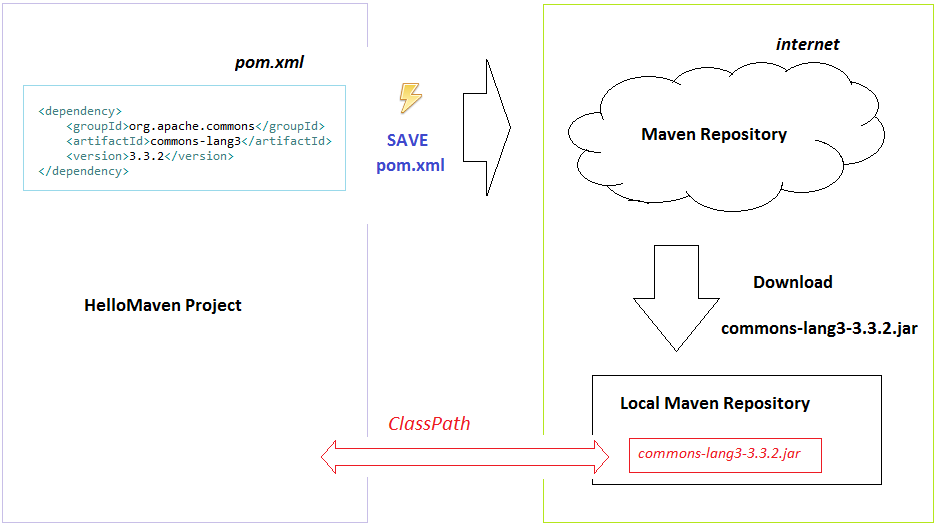
The illustration above shows how the Maven done.
- You declare in pom.xml, that project depend on common-lang3 library version 3.3.2.
- As soon as you SAVE pom.xml file, Maven will check if this library has local repository on your computer yet. If has no, Maven will download it from the repository in the internet.
- Finally, Maven will automatically declare Classpath.
So you only need to declare all libraries want to use in pom.xml. The libraries was managed by Maven.
6. View Local repository
Your question is where local repository located?
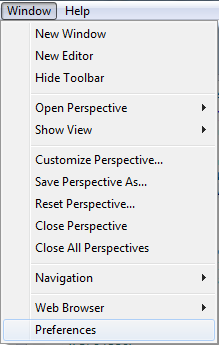
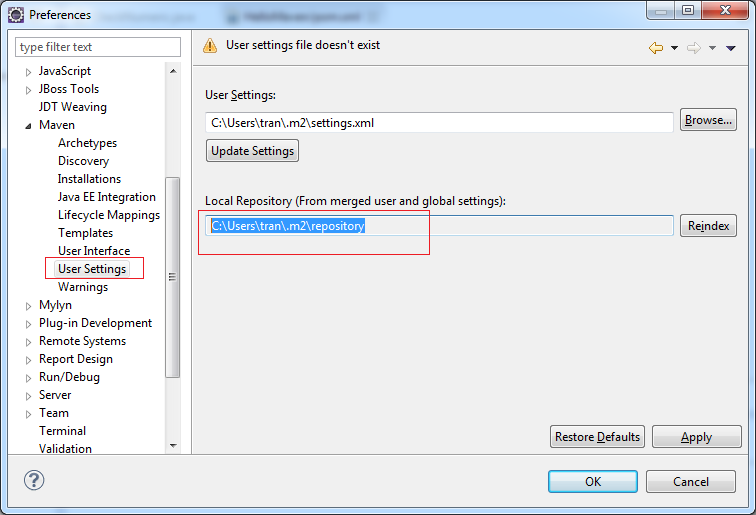
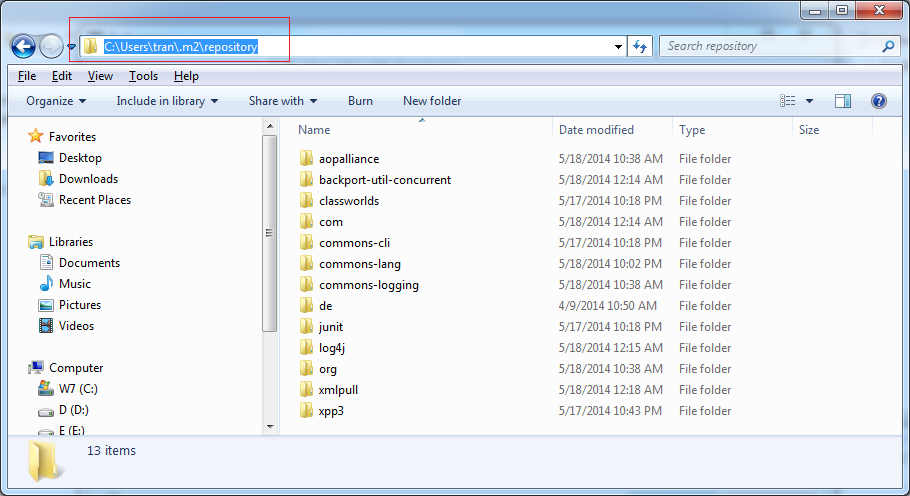
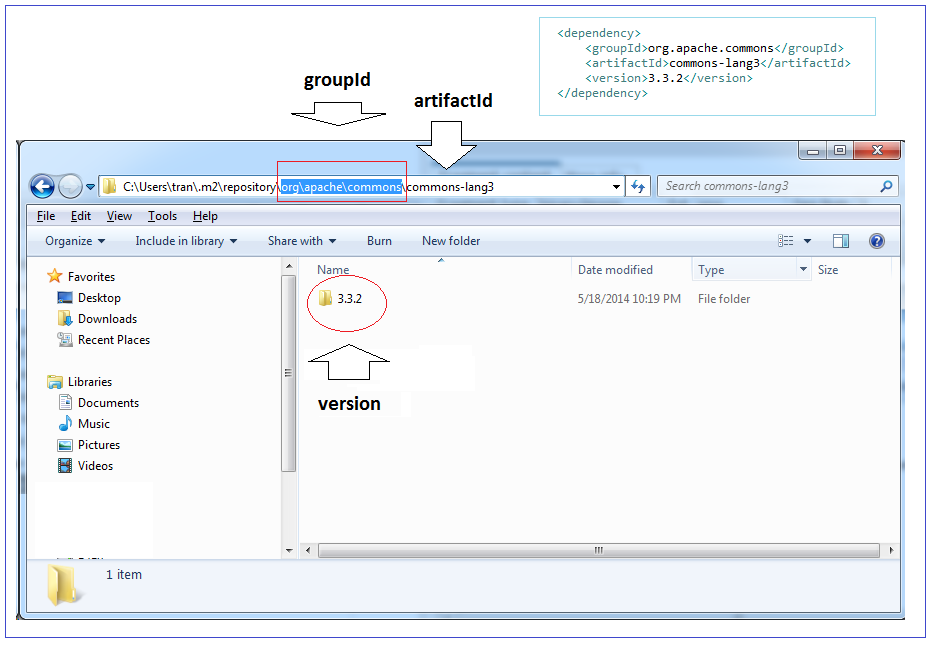
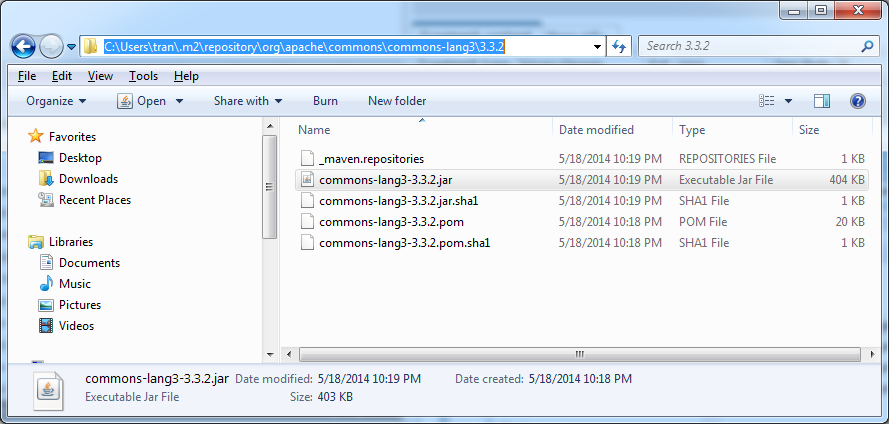
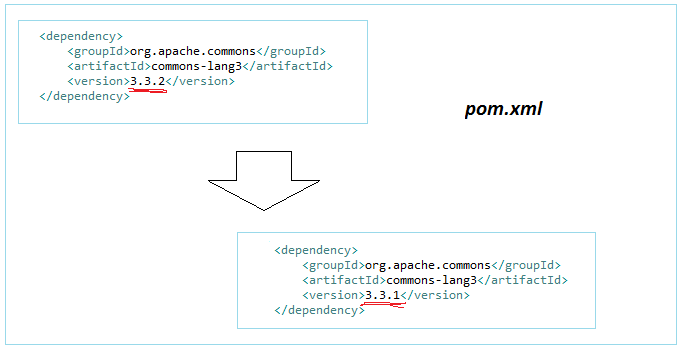
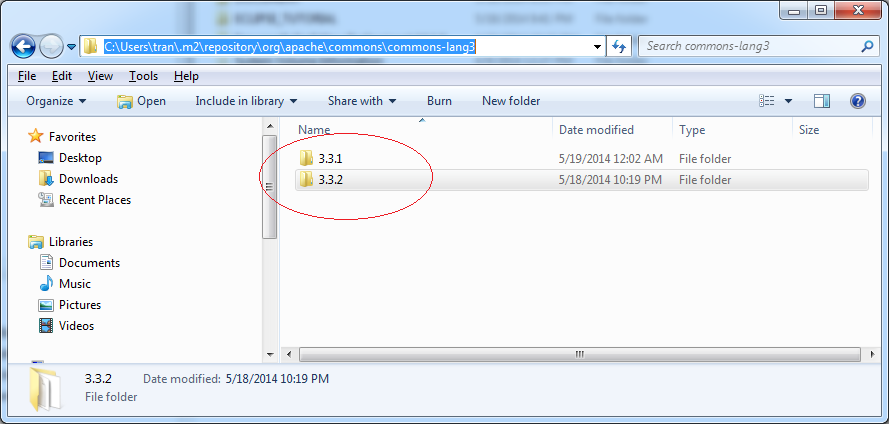
View your project is packed to the Local Repository:
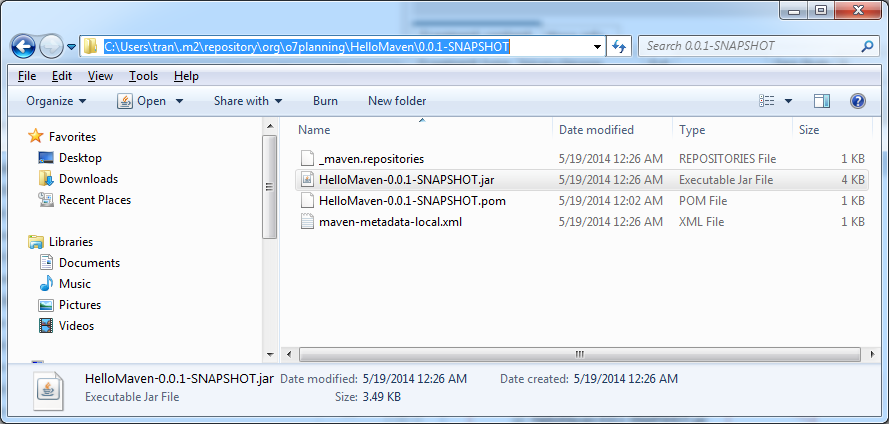
7. View Maven Repository on Internet
The question is where to lookup the information groupId, artifactId and version.
You can go to one of these sites:
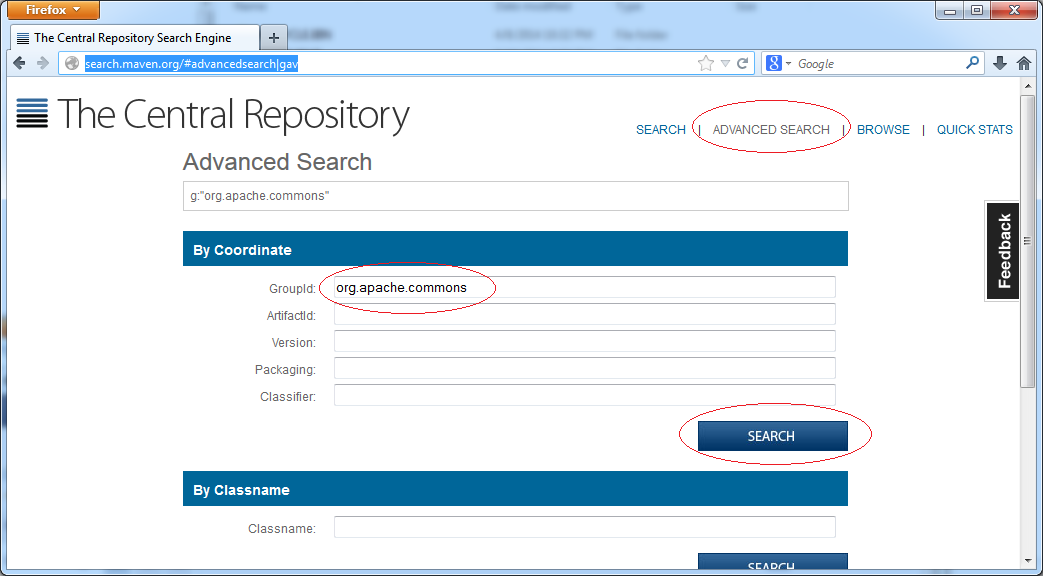
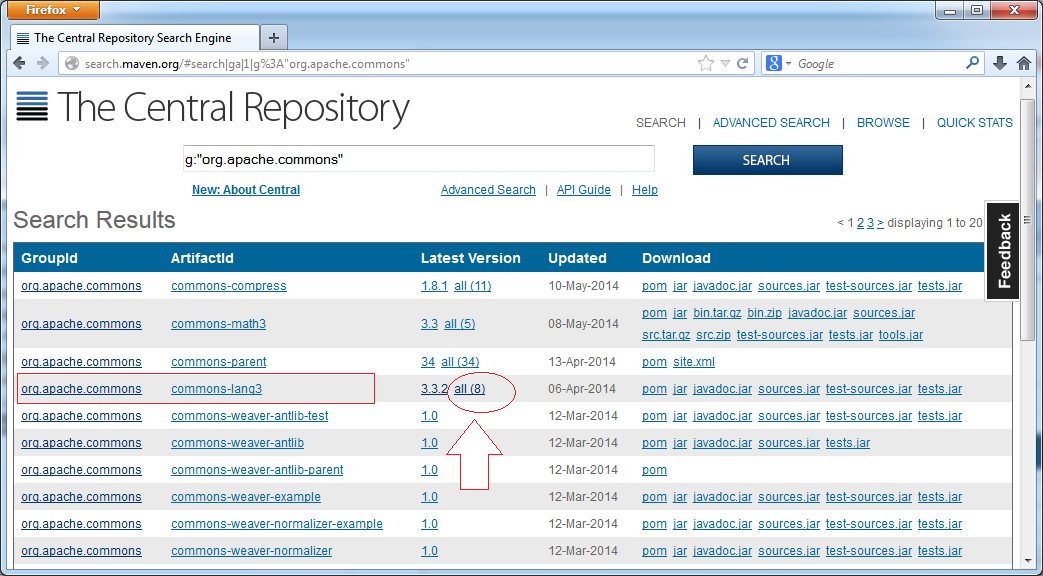
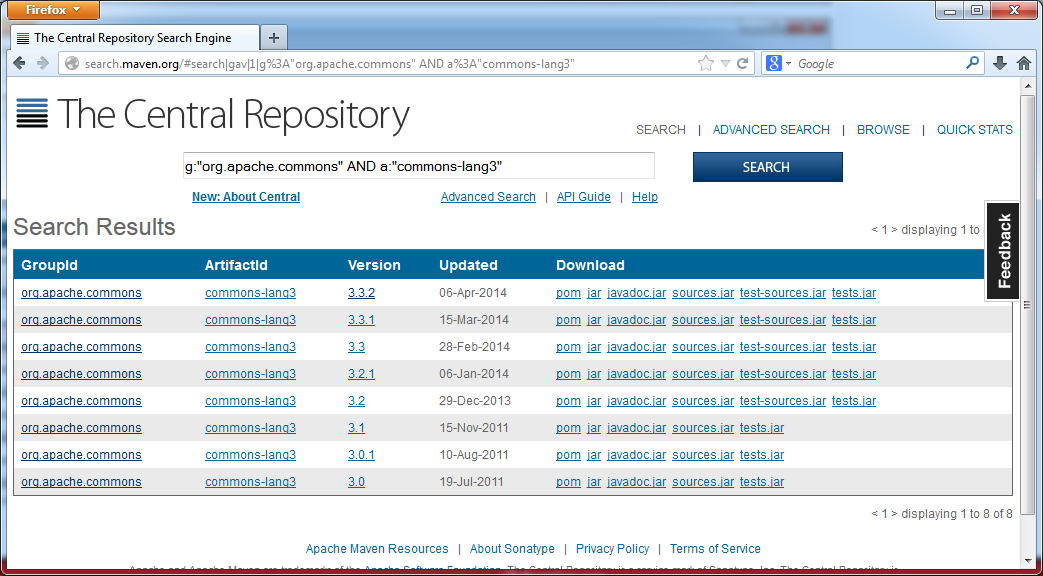
8. Configuring Maven download source and javadoc
Normally Maven only download binary files. To Maven download the source and javadoc, you need to configure on Eclipse.
- Windows/Preferences
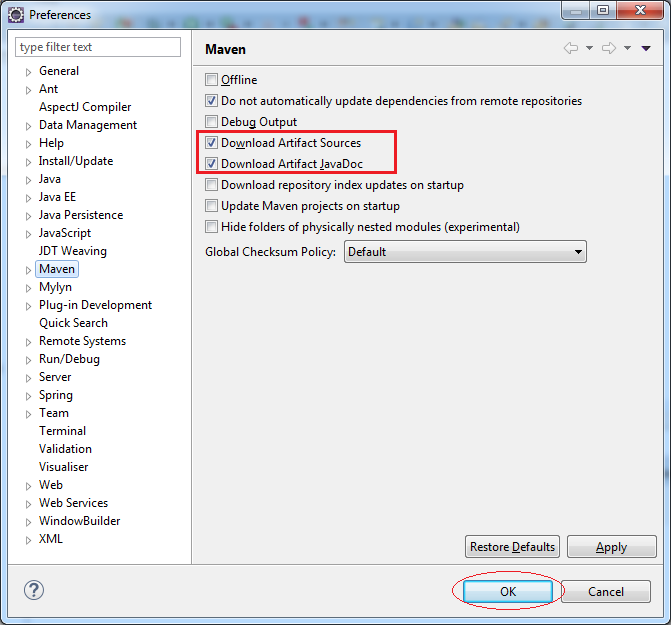
Change something in the pom.xml file and save (Or build project), Maven will download the source and javadoc.
The results you see on the Local Repository:
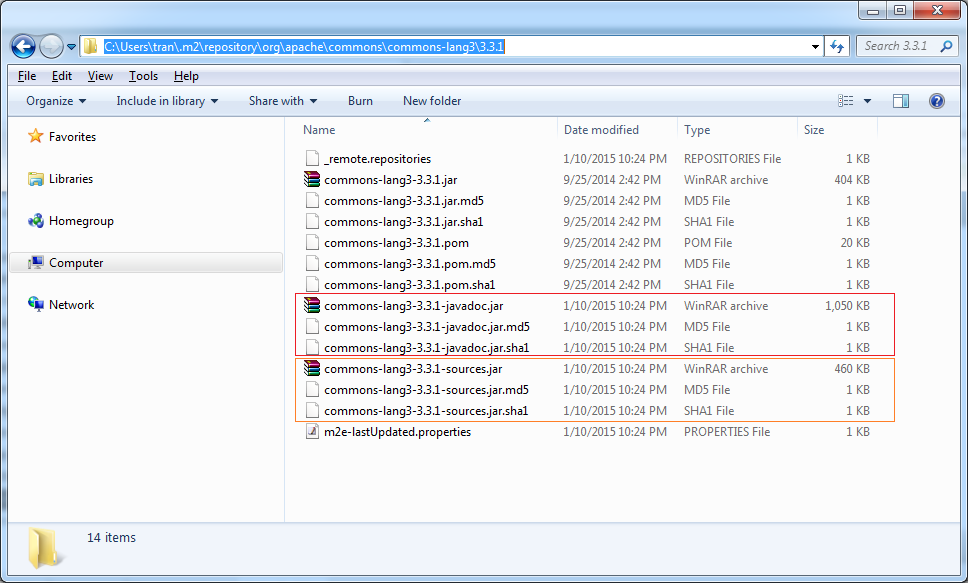
Maven Tutorials
- Install Maven for Eclipse
- Maven Tutorial for Beginners
- Maven Manage Dependencies
- Build a Multiple Module Project with Maven
- Run Maven Java Web Application in Tomcat Maven Plugin
- Run Maven Java Web Application in Jetty Maven Plugin
- Install Tycho for Eclipse
- Create Java OSGi project with Maven and Tycho
- Create an Empty Maven Web App project in Eclipse
- OSGi and AspectJ integration
Show More