Spring Boot and Mustache Tutorial with Examples
1. What is Mustache?
Mustache is aWeb Template system, which is used in combination with the data of Model layer to create HTML files. It is supported by many languages such as Java, ActionScript, C++, Clojure, CoffeeScript, ColdFusion, PHP, ....

Mustache is described as a logic-less system, because it does not has statements controlling the flow of the program clearly, for example, it has no if/else statement and loop statement, it uses lambda expression and mustache tags instead.
Assume that "employees" is a list. In this context, open & close tag pairs: {{#employees}} & {{/employees}} will be equivalent to a loop in the "employees" list.
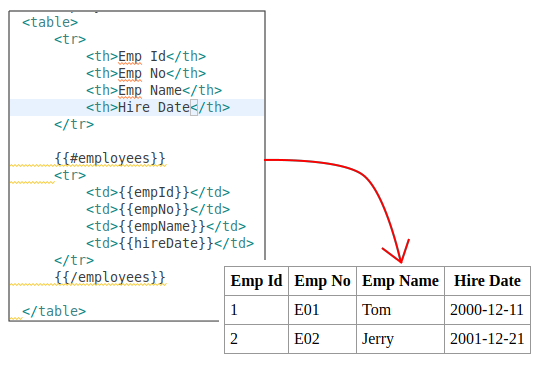
The reason it is named"Mustache" is that the syntax of the Mustache usually uses curly brackets {}, like a pair of mustaches.
2. Some examples of Mustache
You will probably meet with some trouble and confusion with the Mustache for its first time of working with it. Below are some examples, which show the oddity of this system.
if/else?
Mustache doesn't simply haveif/else condition statement, therefore, you have to create logic for it.
Java Code:
boolean kiss = true;
boolean hug = true;
boolean slap = false;
Mustache Template:
{{#kiss}}
Kiss ...
{{/kiss}}
{{#hug}}
Hug ...
{{/hug}}
{{#slap}}
Hic Hic ...
{{/slap}}
Result:
Kiss ...
Hug ...
{{.}}
In the Mustache, {{.}} is a special tag. Please look at an example:
If you have the data as follows:
int[] numbers = new int[] {1, 2, 3, 4, 5};
String string = "Wheee!";
Mustache Template:
{{# numbers }}
* {{ . }}
{{/ numbers }}
-----
{{# string }}
{{ . }}
{{/ string }}
The result received:
* 1
* 2
* 3
* 4
* 5
----
Wheee!
3. Create a Spring Boot project
On the Eclipse, create a Spring Boot project:
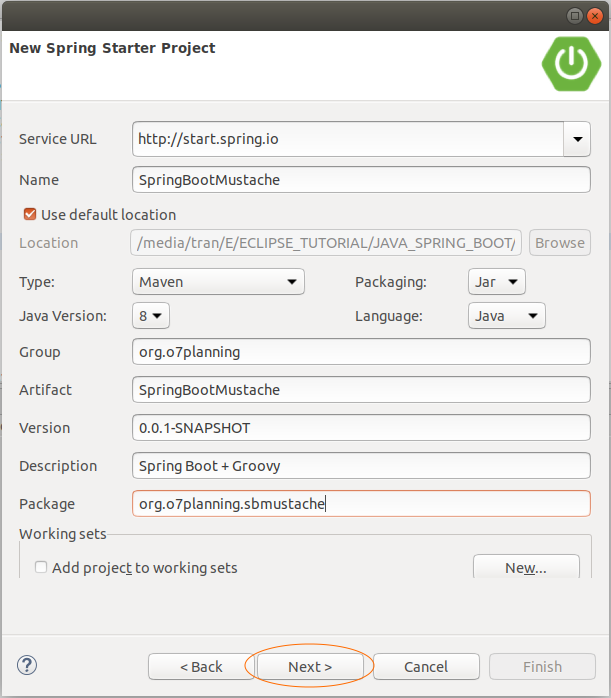
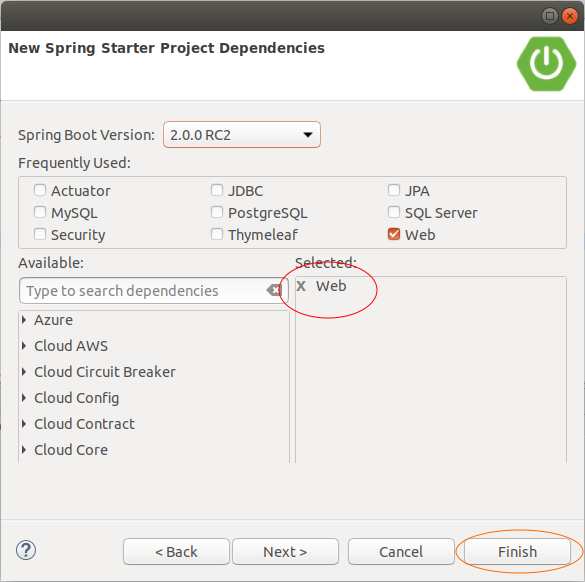
To be able to use the Mustache for View layer, you need to declare spring-boot-starter-mustache dependency in thepom.xml file:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-mustache</artifactId>
</dependency>
The full content of the pom.xml file:
pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0
http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.o7planning</groupId>
<artifactId>SpringBootMustache</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>jar</packaging>
<name>SpringBootMustache</name>
<description>Spring Boot + Groovy</description>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.0.0.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-mustache</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
SpringBootMustacheApplication.java
package org.o7planning.sbmustache;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class SpringBootMustacheApplication {
public static void main(String[] args) {
SpringApplication.run(SpringBootMustacheApplication.class, args);
}
}
4. Model, DAO
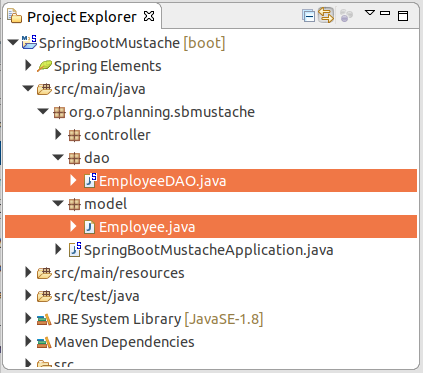
Employee.java
package org.o7planning.sbmustache.model;
import java.util.Date;
public class Employee {
private Long empId;
private String empNo;
private String empName;
private Date hireDate;
public Employee() {
}
public Employee(Long empId, String empNo,
String empName, Date hireDate) {
this.empId = empId;
this.empNo = empNo;
this.empName = empName;
this.hireDate = hireDate;
}
public Long getEmpId() {
return empId;
}
public void setEmpId(Long empId) {
this.empId = empId;
}
public String getEmpNo() {
return empNo;
}
public void setEmpNo(String empNo) {
this.empNo = empNo;
}
public String getEmpName() {
return empName;
}
public void setEmpName(String empName) {
this.empName = empName;
}
public Date getHireDate() {
return hireDate;
}
public void setHireDate(Date hireDate) {
this.hireDate = hireDate;
}
}
EmployeeDAO.java
package org.o7planning.sbmustache.dao;
import java.sql.Date;
import java.time.LocalDate;
import java.util.ArrayList;
import java.util.List;
import org.o7planning.sbmustache.model.Employee;
import org.springframework.stereotype.Repository;
@Repository
public class EmployeeDAO {
public List<Employee> getEmployees() {
Date hireDate1= Date.valueOf(LocalDate.parse("2000-12-11"));
Employee e1= new Employee(1L, "E01", "Tom", hireDate1);
Date hireDate2= Date.valueOf(LocalDate.parse("2001-12-21"));
Employee e2= new Employee(2L, "E02", "Jerry", hireDate2);
List<Employee> list= new ArrayList<Employee>();
list.add(e1);
list.add(e2);
return list;
}
}
5. Controller, Mustache Template
MainController.java
package org.o7planning.sbmustache.controller;
import java.util.List;
import org.o7planning.sbmustache.dao.EmployeeDAO;
import org.o7planning.sbmustache.model.Employee;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
@Controller
public class MainController {
@Autowired
private EmployeeDAO employeeDAO;
@RequestMapping("/")
public String handleRequest(Model model) {
List<Employee> employees = employeeDAO.getEmployees();
model.addAttribute("employees", employees);
return "employee";
}
}
Create an employee.mustache file located in the resources/templates folder of the project.
Note: If you use Spring Boot < 2.x , then Mustache Template files must have the ending in html. Thus, you need to create anemployee.html file.
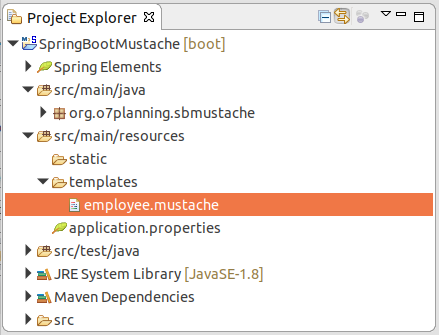
employee.mustache
<html>
<head>
<title>Spring Boot Mustache</title>
<style>
table {
border-collapse: collapse;
}
table, td, th {
border: 1px solid #999;
padding: 5px;
}
</style>
</head>
<body>
<h2>Employees</h2>
<table>
<tr>
<th>Emp Id</th>
<th>Emp No</th>
<th>Emp Name</th>
<th>Hire Date</th>
</tr>
{{#employees}}
<tr>
<td>{{empId}}</td>
<td>{{empNo}}</td>
<td>{{empName}}</td>
<td>{{hireDate}}</td>
</tr>
{{/employees}}
</table>
</body>
</html>
The Spring Boot 2.x automatically configures the Mustache with the following default properties:
application.properties (Default by Spring Boot)
spring.mustache.prefix=classpath:/templates/
spring.mustache.suffix=.mustache
The drawing describes the flow of the application and the relationship between the Controller and Mustache View:
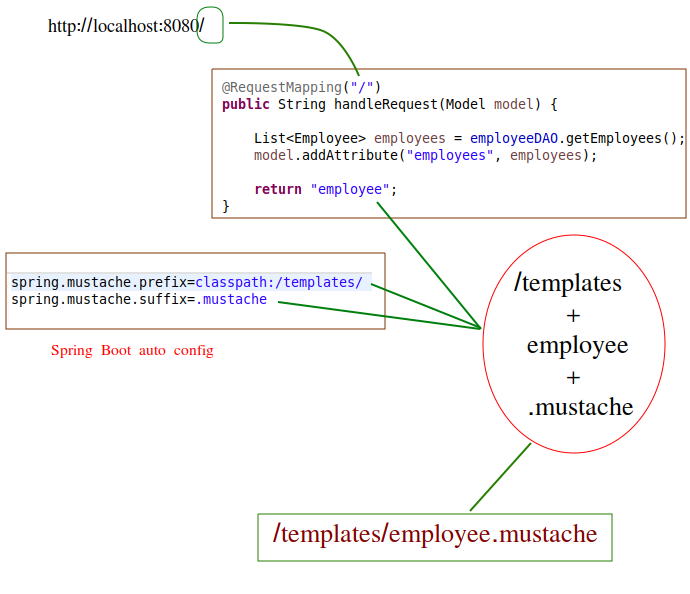
Spring Boot Tutorials
- Install Spring Tool Suite for Eclipse
- Spring Tutorial for Beginners
- Spring Boot Tutorial for Beginners
- Spring Boot Common Properties
- Spring Boot and Thymeleaf Tutorial with Examples
- Spring Boot and FreeMarker Tutorial with Examples
- Spring Boot and Groovy Tutorial with Examples
- Spring Boot and Mustache Tutorial with Examples
- Spring Boot and JSP Tutorial with Examples
- Spring Boot, Apache Tiles, JSP Tutorial with Examples
- Use Logging in Spring Boot
- Application Monitoring with Spring Boot Actuator
- Create a Multi Language web application with Spring Boot
- Use multiple ViewResolvers in Spring Boot
- Use Twitter Bootstrap in Spring Boot
- Spring Boot Interceptors Tutorial with Examples
- Spring Boot, Spring JDBC and Spring Transaction Tutorial with Examples
- Spring JDBC Tutorial with Examples
- Spring Boot, JPA and Spring Transaction Tutorial with Examples
- Spring Boot and Spring Data JPA Tutorial with Examples
- Spring Boot, Hibernate and Spring Transaction Tutorial with Examples
- Integrating Spring Boot, JPA and H2 Database
- Spring Boot and MongoDB Tutorial with Examples
- Use Multiple DataSources with Spring Boot and JPA
- Use Multiple DataSources with Spring Boot and RoutingDataSource
- Create a Login Application with Spring Boot, Spring Security, Spring JDBC
- Create a Login Application with Spring Boot, Spring Security, JPA
- Create a User Registration Application with Spring Boot, Spring Form Validation
- Example of OAuth2 Social Login in Spring Boot
- Run background scheduled tasks in Spring
- CRUD Restful Web Service Example with Spring Boot
- Spring Boot Restful Client with RestTemplate Example
- CRUD Example with Spring Boot, REST and AngularJS
- Secure Spring Boot RESTful Service using Basic Authentication
- Secure Spring Boot RESTful Service using Auth0 JWT
- Spring Boot File Upload Example
- Spring Boot File Download Example
- Spring Boot File Upload with jQuery Ajax Example
- Spring Boot File Upload with AngularJS Example
- Create a Shopping Cart Web Application with Spring Boot, Hibernate
- Spring Email Tutorial with Examples
- Create a simple Chat application with Spring Boot and Websocket
- Deploy Spring Boot Application on Tomcat Server
- Deploy Spring Boot Application on Oracle WebLogic Server
- Install a free Let's Encrypt SSL certificate for Spring Boot
- Configure Spring Boot to redirect HTTP to HTTPS
- Fetch data with Spring Data JPA DTO Projections
Show More