Integrating Spring Boot, JPA and H2 Database
1. Objective of Lesson
H2 is a open source, compact relational database, written in Java language. H2 installer has very little capacity of about 8MB.
One of the interesting features of the H2 is that you can create an In-Memory Database instead of being stored in a computer hard drive. This makes query speed and manipulation with data very fast. However, if you select the "In-Memory Database" feature, data exists only when the application works, when the application is shut down, the data is also deleted from the memory.
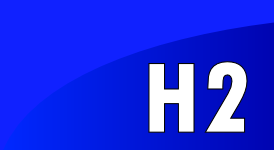
The H2 provides you with an administration tool called H2 Console, and you work with it through browser.
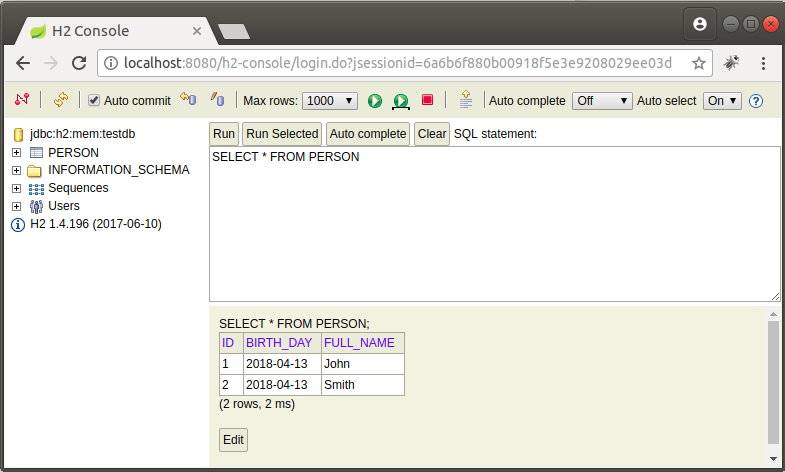
In this lesson, I will show you how to create a Spring Boot project to integrate with the H2 database and use the "In Memory Database" feature, and configure to use the H2Console administration tool.
There is no many difference if you want to connect to the H2 database, installed on computer (See the appendix at the end of the this post)
2. Create a Spring Boot project
On the Eclipse, create the Spring Boot project:
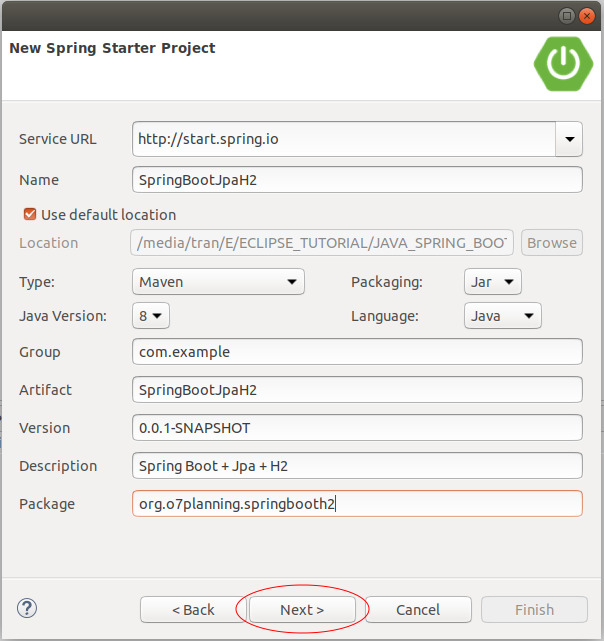
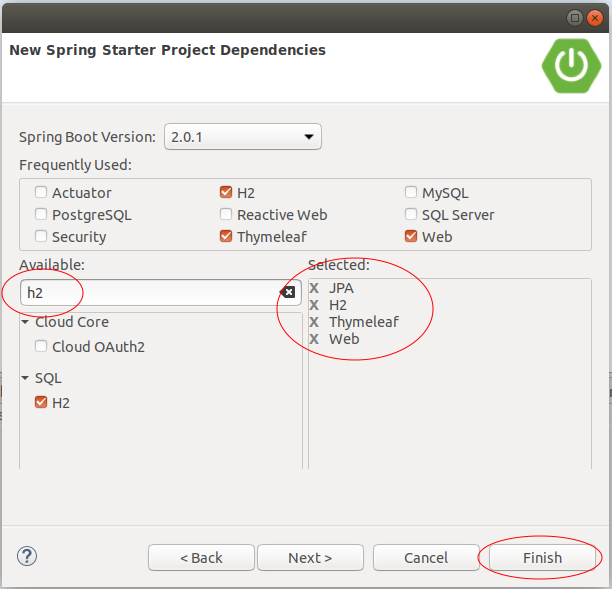
OK, the project has been created.
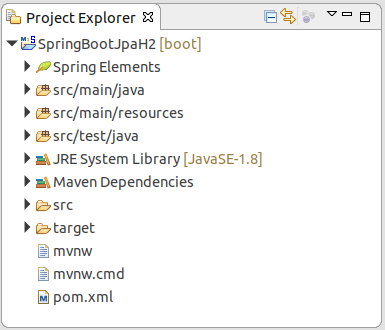
pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>SpringBootJpaH2</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>jar</packaging>
<name>SpringBootJpaH2</name>
<description>Spring Boot + Jpa + H2</description>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.0.1.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>com.h2database</groupId>
<artifactId>h2</artifactId>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
SpringBootJpaH2Application.java
package org.o7planning.springbooth2;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class SpringBootJpaH2Application {
public static void main(String[] args) {
SpringApplication.run(SpringBootJpaH2Application.class, args);
}
}
3. Entity Class, DAO, DataInit, Controller
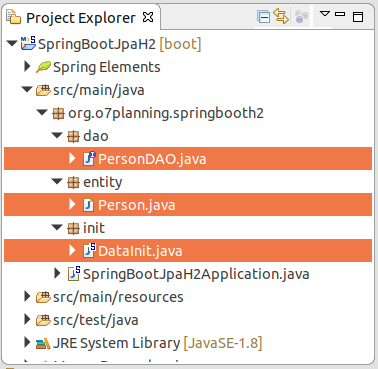
Person.java
package org.o7planning.springbooth2.entity;
import java.util.Date;
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.Id;
import javax.persistence.Table;
import javax.persistence.Temporal;
import javax.persistence.TemporalType;
@Entity
@Table(name = "PERSON")
public class Person {
@Id
@GeneratedValue
@Column(name = "Id", nullable = false)
private Long id;
@Column(name = "Full_Name", length = 64, nullable = false)
private String fullName;
@Temporal(TemporalType.DATE)
@Column(name = "Date_Of_Birth", nullable = false)
private Date dateOfBirth;
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public String getFullName() {
return fullName;
}
public void setFullName(String fullName) {
this.fullName = fullName;
}
public Date getDateOfBirth() {
return dateOfBirth;
}
public void setDateOfBirth(Date dateOfBirth) {
this.dateOfBirth = dateOfBirth;
}
}
PersonDAO is an interface extended from CrudRepository<Person, Long>. Spring Data JPA , which will itself create you a class to implement this interface at the time of starting the application.
See also:
PersonDAO.java
package org.o7planning.springbooth2.dao;
import java.util.Date;
import java.util.List;
import org.o7planning.springbooth2.entity.Person;
import org.springframework.data.repository.CrudRepository;
import org.springframework.stereotype.Repository;
@Repository
public interface PersonDAO extends CrudRepository<Person, Long> {
public List<Person> findByFullNameLike(String name);
public List<Person> findByDateOfBirthGreaterThan(Date date);
}
The DataInit implements ApplicationRunner interface. It will automatically run at the time when the application starts. In this class, we will insert some records to the PERSON table.
DataInit.java
package org.o7planning.springbooth2.init;
import java.text.DateFormat;
import java.text.SimpleDateFormat;
import java.util.Date;
import org.o7planning.springbooth2.dao.PersonDAO;
import org.o7planning.springbooth2.entity.Person;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.ApplicationArguments;
import org.springframework.boot.ApplicationRunner;
import org.springframework.stereotype.Component;
@Component
public class DataInit implements ApplicationRunner {
private PersonDAO personDAO;
private static final DateFormat df = new SimpleDateFormat("yyyy-MM-dd");
@Autowired
public DataInit(PersonDAO personDAO) {
this.personDAO = personDAO;
}
@Override
public void run(ApplicationArguments args) throws Exception {
long count = personDAO.count();
if (count == 0) {
Person p1 = new Person();
p1.setFullName("John");
Date d1 = df.parse("1980-12-20");
p1.setDateOfBirth(d1);
//
Person p2 = new Person();
p2.setFullName("Smith");
Date d2 = df.parse("1985-11-11");
p2.setDateOfBirth(d2);
personDAO.save(p1);
personDAO.save(p2);
}
}
}
MainController.java
package org.o7planning.springbooth2.controller;
import org.o7planning.springbooth2.dao.PersonDAO;
import org.o7planning.springbooth2.entity.Person;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.ResponseBody;
@Controller
public class MainController {
@Autowired
private PersonDAO personDAO;
@ResponseBody
@RequestMapping("/")
public String index() {
Iterable<Person> all = personDAO.findAll();
StringBuilder sb = new StringBuilder();
all.forEach(p -> sb.append(p.getFullName() + "<br>"));
return sb.toString();
}
}
4. Configure Spring Boot & H2
In this example, I will configure the Spring Boot to use the H2 as an In-memory Database, which means we don't need to install the H2 database. It will automatically be created and stored in computer memory.
In another case, if you have installed a H2 database on your computer, and want to interact Spring Boot application with this database, you can also see the appendix at the bottom of this post
application.properties
# To See H2 Console in Browser:
# http://localhost:8080/h2-console
# Enabling H2 Console
spring.h2.console.enabled=true
# ===============================
# DB
# ===============================
spring.datasource.url=jdbc:h2:mem:testdb
spring.datasource.driverClassName=org.h2.Driver
spring.datasource.username=sa
spring.datasource.password=
# ===============================
# JPA / HIBERNATE
# ===============================
spring.jpa.show-sql=true
spring.jpa.hibernate.ddl-auto=update
spring.jpa.properties.hibernate.dialect=org.hibernate.dialect.H2Dialect
spring.h2.console.enabled=true
This configuration tells the Spring to start the administration tool of the H2 database and you can access this tool on the browser:
spring.datasource.url=jdbc:h2:mem:testdb
This configuration tells Spring that you want to use the In-Memory H2 Database.
spring.jpa.hibernate.ddl-auto=update
This configuration tells the Spring to create (or update) the structure of structure-based table of Entity classes. Thus, the PERSON table will automatically be created by the structure of the Person class.
5. Run the application
On the Eclipse, run your application:
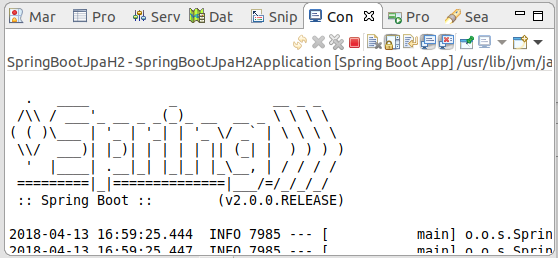
At this moment, the H2 Console is also started with the application and you can access this administration tool:
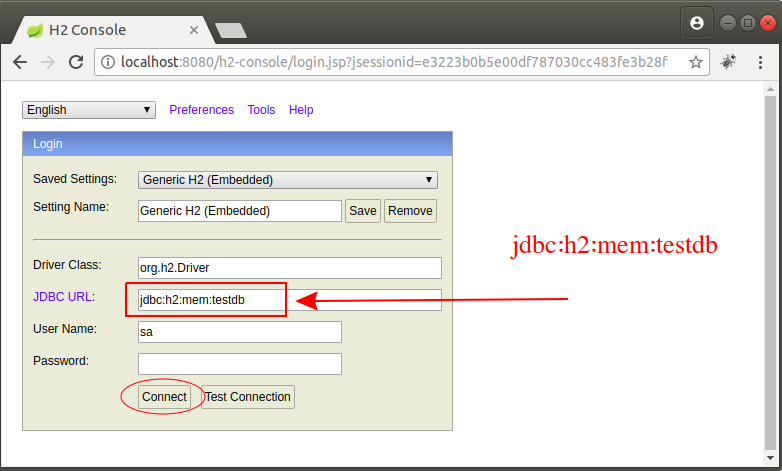
The PERSON table has automatically been created based on the structure of the Person class.
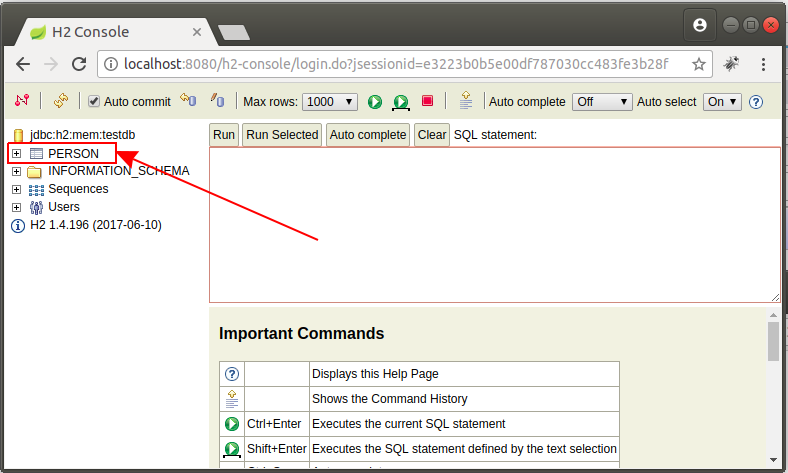
Query the PERSON table:
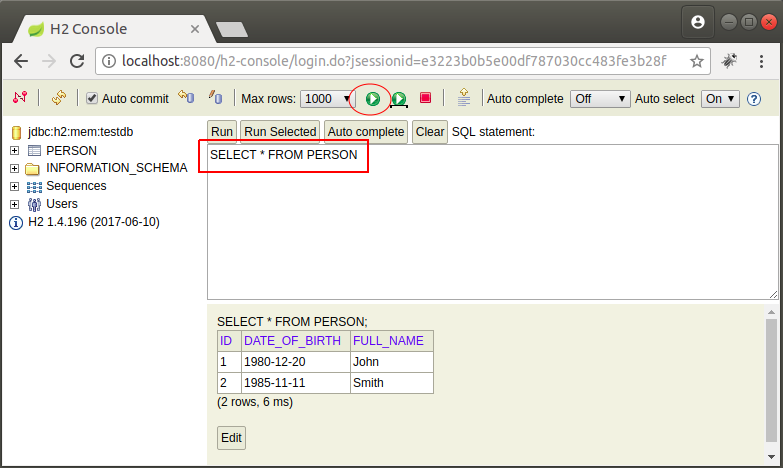
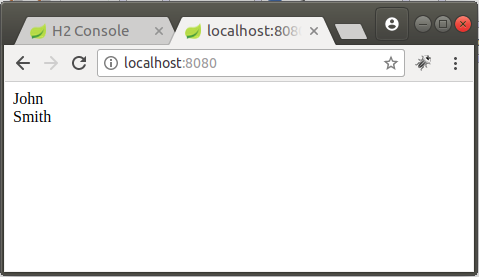
6. Appendix H2
In case you use H2 (Server):
application.properties (H2 Server)
# To See H2 Console in Browser:
# http://localhost:8080/h2-console
# Enabling H2 Console
spring.h2.console.enabled=true
# ===============================
# DB
# ===============================
spring.datasource.url=jdbc:h2:tcp://localhost/~/testdb
spring.datasource.driverClassName=org.h2.Driver
spring.datasource.username=sa
spring.datasource.password=
# ===============================
# JPA / HIBERNATE
# ===============================
spring.jpa.show-sql=true
spring.jpa.hibernate.ddl-auto=update
spring.jpa.properties.hibernate.dialect=org.hibernate.dialect.H2Dialect
Spring Boot Tutorials
- Install Spring Tool Suite for Eclipse
- Spring Tutorial for Beginners
- Spring Boot Tutorial for Beginners
- Spring Boot Common Properties
- Spring Boot and Thymeleaf Tutorial with Examples
- Spring Boot and FreeMarker Tutorial with Examples
- Spring Boot and Groovy Tutorial with Examples
- Spring Boot and Mustache Tutorial with Examples
- Spring Boot and JSP Tutorial with Examples
- Spring Boot, Apache Tiles, JSP Tutorial with Examples
- Use Logging in Spring Boot
- Application Monitoring with Spring Boot Actuator
- Create a Multi Language web application with Spring Boot
- Use multiple ViewResolvers in Spring Boot
- Use Twitter Bootstrap in Spring Boot
- Spring Boot Interceptors Tutorial with Examples
- Spring Boot, Spring JDBC and Spring Transaction Tutorial with Examples
- Spring JDBC Tutorial with Examples
- Spring Boot, JPA and Spring Transaction Tutorial with Examples
- Spring Boot and Spring Data JPA Tutorial with Examples
- Spring Boot, Hibernate and Spring Transaction Tutorial with Examples
- Integrating Spring Boot, JPA and H2 Database
- Spring Boot and MongoDB Tutorial with Examples
- Use Multiple DataSources with Spring Boot and JPA
- Use Multiple DataSources with Spring Boot and RoutingDataSource
- Create a Login Application with Spring Boot, Spring Security, Spring JDBC
- Create a Login Application with Spring Boot, Spring Security, JPA
- Create a User Registration Application with Spring Boot, Spring Form Validation
- Example of OAuth2 Social Login in Spring Boot
- Run background scheduled tasks in Spring
- CRUD Restful Web Service Example with Spring Boot
- Spring Boot Restful Client with RestTemplate Example
- CRUD Example with Spring Boot, REST and AngularJS
- Secure Spring Boot RESTful Service using Basic Authentication
- Secure Spring Boot RESTful Service using Auth0 JWT
- Spring Boot File Upload Example
- Spring Boot File Download Example
- Spring Boot File Upload with jQuery Ajax Example
- Spring Boot File Upload with AngularJS Example
- Create a Shopping Cart Web Application with Spring Boot, Hibernate
- Spring Email Tutorial with Examples
- Create a simple Chat application with Spring Boot and Websocket
- Deploy Spring Boot Application on Tomcat Server
- Deploy Spring Boot Application on Oracle WebLogic Server
- Install a free Let's Encrypt SSL certificate for Spring Boot
- Configure Spring Boot to redirect HTTP to HTTPS
- Fetch data with Spring Data JPA DTO Projections
Show More