Spring Boot File Upload with AngularJS Example
1. Objective of lesson
In this lesson, I am going to guide you for creating a File Upload application using Spring Boot and AngularJS. Below is the preview image of the application to be performed by us:
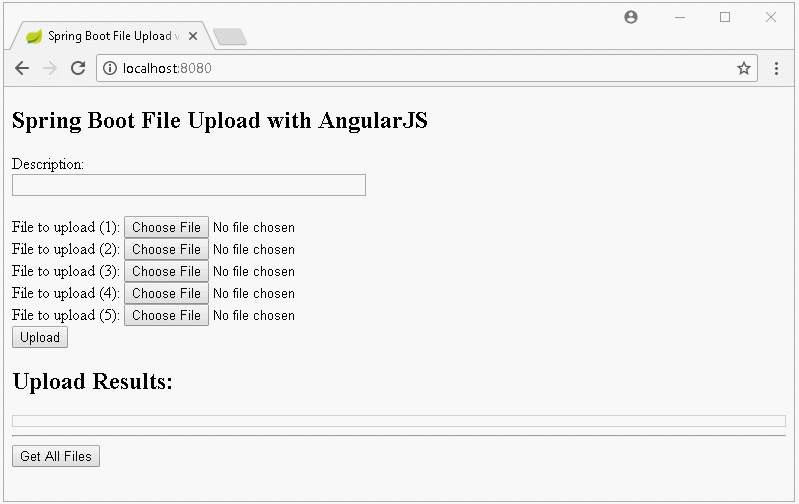
Notify on the interface when uploading fails:
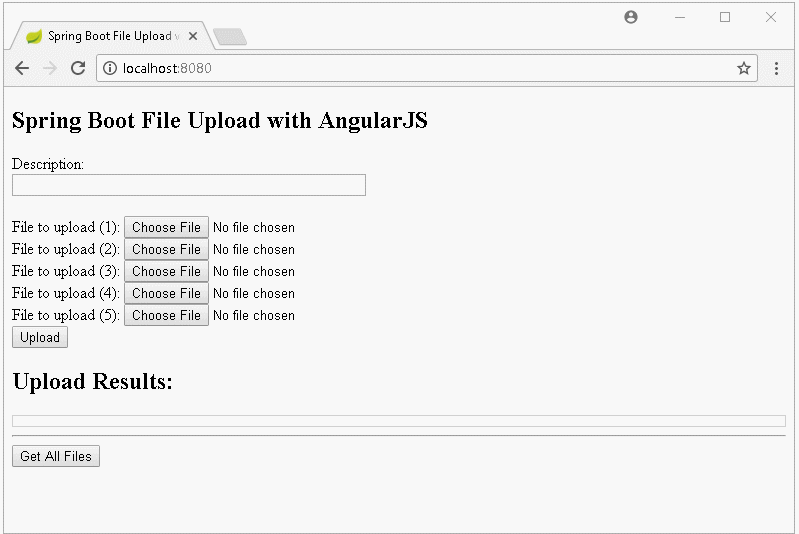
Display the list of uploaded files and handle downloading when an user clicks a link.
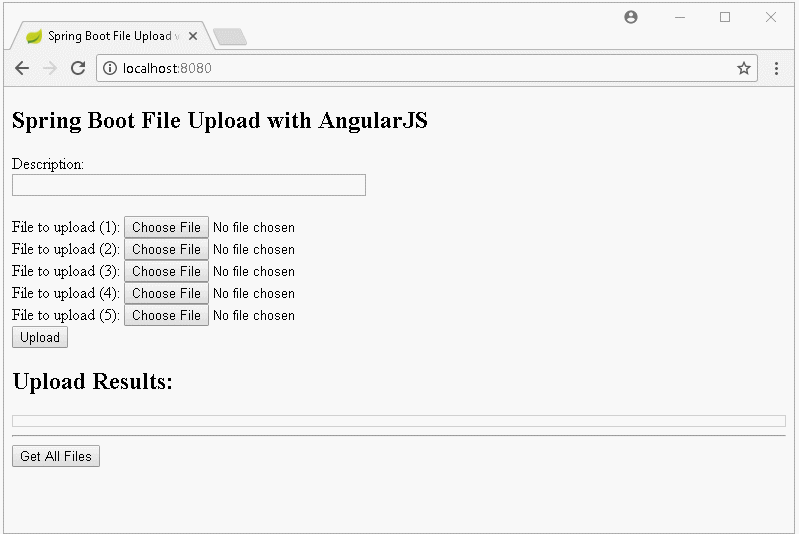
2. Create a Spring Boot Project
On the Eclipse, create a Spring Boot project:
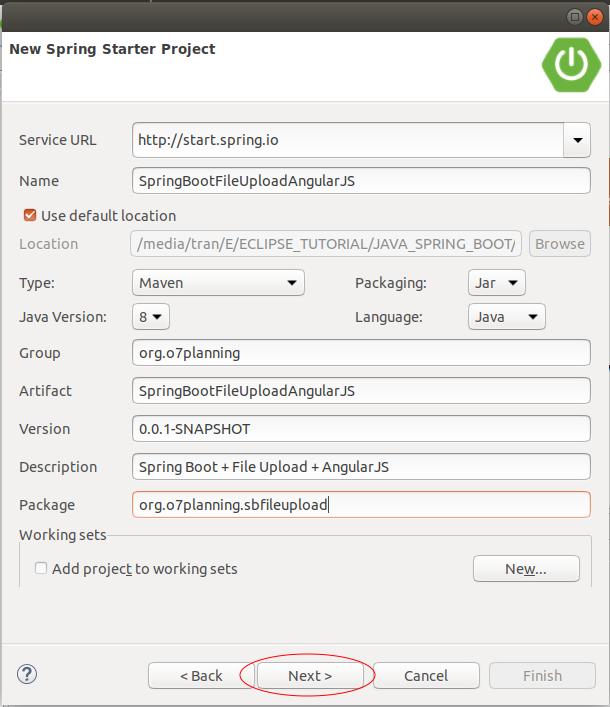
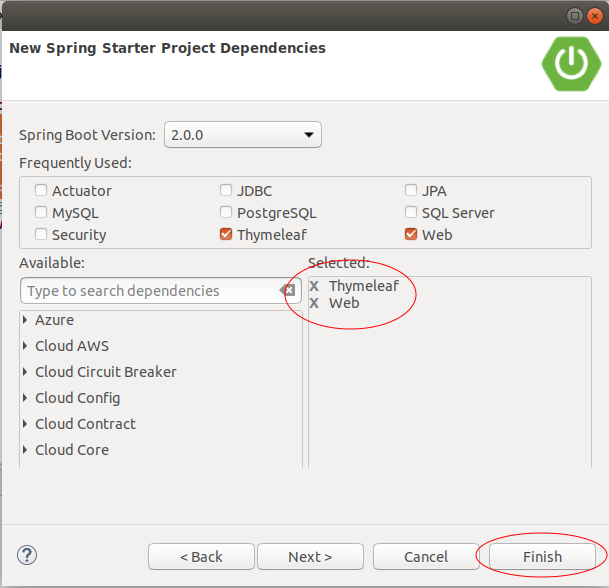
pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0
http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.o7planning</groupId>
<artifactId>SpringBootFileUploadAngularJS</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>jar</packaging>
<name>SpringBootFileUploadAngularJS</name>
<description>Spring Boot + File Upload + AngularJS</description>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.0.0.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
SpringBootFileUploadAngularJsApplication.java
package org.o7planning.sbfileupload;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class SpringBootFileUploadAngularJsApplication {
public static void main(String[] args) {
SpringApplication.run(SpringBootFileUploadAngularJsApplication.class, args);
}
}
3. Form, Controller, Exception Handler
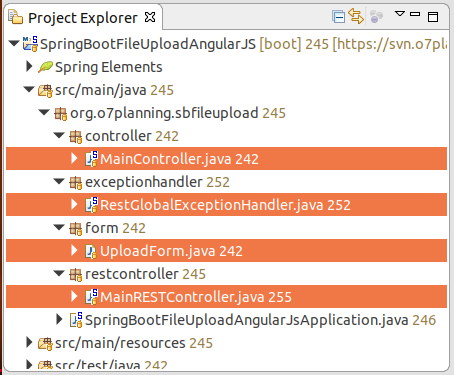
The UploadForm class represents for the data of HTML form.
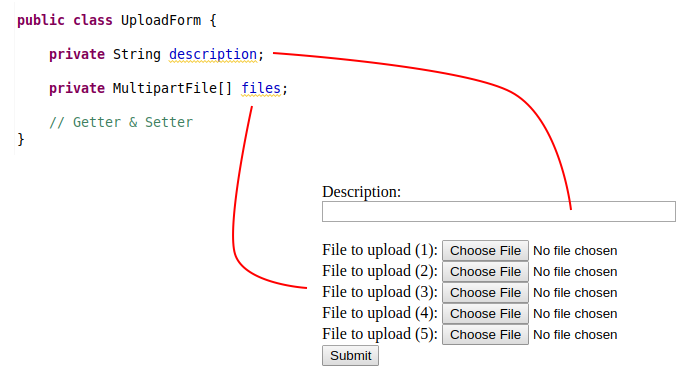
UploadForm.java
package org.o7planning.sbfileupload.form;
import org.springframework.web.multipart.MultipartFile;
public class UploadForm {
private String description;
private MultipartFile[] files;
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public MultipartFile[] getFiles() {
return files;
}
public void setFiles(MultipartFile[] files) {
this.files = files;
}
}
MainController.java
package org.o7planning.sbfileupload.controller;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
@Controller
public class MainController {
@GetMapping("/")
public String index() {
return "upload";
}
}
The MainRESTController class defines REST APIs to process the data of the files uploaded by users. These REST APIs will be called by the AngularJS (See in the UploadFileCtrl.js).
MainRESTController.java
package org.o7planning.sbfileupload.restcontroller;
import java.io.File;
import java.io.IOException;
import java.net.MalformedURLException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.ArrayList;
import java.util.List;
import org.o7planning.sbfileupload.form.UploadForm;
import org.springframework.core.io.Resource;
import org.springframework.core.io.UrlResource;
import org.springframework.http.HttpHeaders;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.ModelAttribute;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.web.multipart.MultipartFile;
@RestController
public class MainRESTController {
// Linux: /home/{user}/test
// Windows: C:/Users/{user}/test
private static String UPLOAD_DIR = System.getProperty("user.home") + "/test";
@PostMapping("/rest/uploadMultiFiles")
public ResponseEntity<?> uploadFileMulti(@ModelAttribute UploadForm form) throws Exception {
System.out.println("Description:" + form.getDescription());
String result = null;
try {
result = this.saveUploadedFiles(form.getFiles());
}
// Here Catch IOException only.
// Other Exceptions catch by RestGlobalExceptionHandler class.
catch (IOException e) {
e.printStackTrace();
return new ResponseEntity<>("Error: " + e.getMessage(), HttpStatus.BAD_REQUEST);
}
return new ResponseEntity<String>("Uploaded to: " + result, HttpStatus.OK);
}
// Save Files
private String saveUploadedFiles(MultipartFile[] files) throws IOException {
// Make sure directory exists!
File uploadDir = new File(UPLOAD_DIR);
uploadDir.mkdirs();
StringBuilder sb = new StringBuilder();
for (MultipartFile file : files) {
if (file.isEmpty()) {
continue;
}
String uploadFilePath = UPLOAD_DIR + "/" + file.getOriginalFilename();
byte[] bytes = file.getBytes();
Path path = Paths.get(uploadFilePath);
Files.write(path, bytes);
sb.append(uploadFilePath).append(", ");
}
return sb.toString();
}
@GetMapping("/rest/getAllFiles")
public List<String> getListFiles() {
File uploadDir = new File(UPLOAD_DIR);
File[] files = uploadDir.listFiles();
List<String> list = new ArrayList<String>();
for (File file : files) {
list.add(file.getName());
}
return list;
}
// @filename: abc.zip,..
@GetMapping("/rest/files/{filename:.+}")
public ResponseEntity<Resource> getFile(@PathVariable String filename) throws MalformedURLException {
File file = new File(UPLOAD_DIR + "/" + filename);
if (!file.exists()) {
throw new RuntimeException("File not found");
}
Resource resource = new UrlResource(file.toURI());
return ResponseEntity.ok()
.header(HttpHeaders.CONTENT_DISPOSITION, "attachment; filename=\"" + file.getName() + "\"")
.body(resource);
}
}
The default size of the file uploaded to the Server doesn't exceed 1MB. And if an user uploads a lot of files at the same time, total size of the files doesn't also exceed 1MB. However, you can configure to change these parameters.
application.properties
spring.servlet.multipart.max-file-size=1MB
spring.servlet.multipart.max-request-size=5MB
spring.thymeleaf.cache=false
RestGlobalExceptionHandler is a custom class, extended from the ResponseEntityExceptionHandler class. In this class, you can handle exceptions thrown from REST methods. This will help you handle the exceptions centralized at a location instead of handling an exception at each REST method.
RestGlobalExceptionHandler.java
package org.o7planning.sbfileupload.exceptionhandler;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.ControllerAdvice;
import org.springframework.web.bind.annotation.ExceptionHandler;
import org.springframework.web.bind.annotation.ResponseBody;
import org.springframework.web.multipart.MultipartException;
import org.springframework.web.servlet.mvc.method.annotation.ResponseEntityExceptionHandler;
import javax.servlet.http.HttpServletRequest;
@ControllerAdvice
public class RestGlobalExceptionHandler extends ResponseEntityExceptionHandler {
// Catch max file size Exception.
@ExceptionHandler(MultipartException.class)
@ResponseBody
public ResponseEntity<?> handleControllerException(HttpServletRequest request, Throwable ex) {
HttpStatus status = this.getStatus(request);
return new ResponseEntity<String>("(Message in RestGlobalExceptionHandler *): " + ex.getMessage(), status);
}
// Catch Other Exception
@ExceptionHandler(Exception.class)
@ResponseBody
public ResponseEntity<?> handleControllerRootException(HttpServletRequest request, Throwable ex) {
HttpStatus status = this.getStatus(request);
return new ResponseEntity<String>("(Message in RestGlobalExceptionHandler **): " + ex.getMessage(), status);
}
private HttpStatus getStatus(HttpServletRequest request) {
Integer statusCode = (Integer) request.getAttribute("javax.servlet.error.status_code");
if (statusCode == null) {
return HttpStatus.INTERNAL_SERVER_ERROR;
}
return HttpStatus.valueOf(statusCode);
}
}
4. Javascript & View (Thymeleaf)
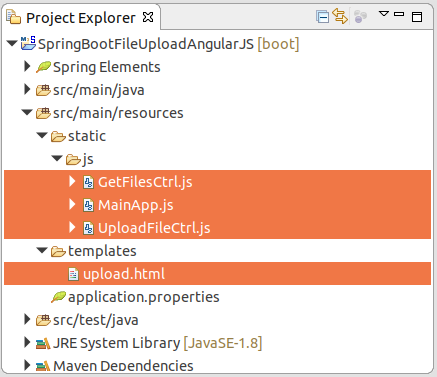
upload.html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<title>Spring Boot File Upload with AngularJS</title>
<meta charset="utf-8" />
<!-- Check other AngularJS version at: -->
<!-- https://code.angularjs.org/1.6.9/docs/misc/downloading -->
<script
src="https://ajax.googleapis.com/ajax/libs/angularjs/1.6.9/angular.min.js"></script>
<script src="/js/MainApp.js"></script>
<script src="/js/UploadFileCtrl.js"></script>
<script src="/js/GetFilesCtrl.js"></script>
</head>
<body ng-app="MainApp">
<h2>Spring Boot File Upload with AngularJS</h2>
<div ng-controller="UploadFileController">
<form>
Description: <br/>
<input type="text" name="description" ng-model="myForm.description" style="width:350px;"/>
<br/><br/>
File to upload (1): <input type="file" file-model="myForm.files[0]"/><br />
File to upload (2): <input type="file" file-model="myForm.files[1]"/><br />
File to upload (3): <input type="file" file-model="myForm.files[2]"/><br />
File to upload (4): <input type="file" file-model="myForm.files[3]"/><br />
File to upload (5): <input type="file" file-model="myForm.files[4]"/><br />
<button type="button" ng-click="doUploadFile()">Upload</button>
</form>
<h2>Upload Results:</h2>
<div style="border:1px solid #ccc;padding: 5px;">
<span ng-bind="uploadResult"></span>
</div>
</div>
<!-- Get Files -->
<hr>
<div ng-controller="GetFilesController">
<button type="button" ng-click="getAllFiles()">Get All Files</button>
<ul>
<li ng-repeat="file in allFiles">
<a href='/rest/files/{{file}}'>{{file}}</a>
</li>
</ul>
</div>
</body>
</html>
In the AngularJS, use the ng-model attribute to help you with 2-way binding between Input element of Form and Model, which means that if the data of the Model changes, the interface of Input element will be updated and vice versa, if an user changes the interface of (Input element), the Model will be updated.
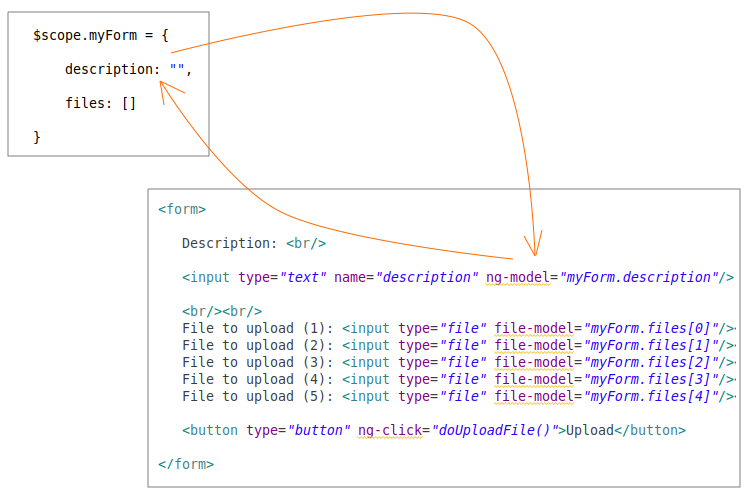
Unfortunately, the ng-model attribute does not support the two-way binding between Model and Input[file], therefore, you need to define an directive called "fileModel" to build the two-way binding between the Model and the Input[file]. This directive is defined in the MainApp.js:
js/MainApp.js
// main app.
var mainApp = angular.module('MainApp', []);
// DIRECTIVE - FILE MODEL
mainApp.directive('fileModel', ['$parse', function ($parse) {
return {
restrict: 'A',
link: function(scope, element, attrs) {
var model = $parse(attrs.fileModel);
var modelSetter = model.assign;
element.bind('change', function(){
scope.$apply(function(){
modelSetter(scope, element[0].files[0]);
});
});
}
};
}]);
The UploadFileCtrl.js file contains AngularJS functions to control uploading files to the Server.
js/UploadFileCtrl.js
// CONTROLLER UPLOAD FILE
mainApp.controller('UploadFileController', function($scope, $http) {
$scope.uploadResult ="";
$scope.myForm = {
description: "",
files: []
}
$scope.doUploadFile = function() {
var url = "/rest/uploadMultiFiles";
var data = new FormData();
data.append("description", $scope.myForm.description);
for (i = 0; i < $scope.myForm.files.length; i++) {
data.append("files", $scope.myForm.files[i]);
}
var config = {
transformRequest: angular.identity,
transformResponse: angular.identity,
headers: {
'Content-Type': undefined
}
}
$http.post(url, data, config).then(
// Success
function(response) {
$scope.uploadResult = response.data;
},
// Error
function(response) {
$scope.uploadResult = response.data;
});
};
});
The GetFilesCtrl.js file contains the AngularJS functions to control obtaining the list of files uploaded to the Server.
js/GetFilesCtrl.js
mainApp.controller('GetFilesController', function($scope, $http) {
$scope.allFiles = [];
$scope.getAllFiles = function() {
// REST URL:
var url = "/rest/getAllFiles";
$http.get(url).then(
// Success
function(response) { alert("OK");
$scope.allFiles = response.data;
},
// Error
function(response) {
alert("Error: " + response.data);
}
);
};
});
Spring Boot Tutorials
- Install Spring Tool Suite for Eclipse
- Spring Tutorial for Beginners
- Spring Boot Tutorial for Beginners
- Spring Boot Common Properties
- Spring Boot and Thymeleaf Tutorial with Examples
- Spring Boot and FreeMarker Tutorial with Examples
- Spring Boot and Groovy Tutorial with Examples
- Spring Boot and Mustache Tutorial with Examples
- Spring Boot and JSP Tutorial with Examples
- Spring Boot, Apache Tiles, JSP Tutorial with Examples
- Use Logging in Spring Boot
- Application Monitoring with Spring Boot Actuator
- Create a Multi Language web application with Spring Boot
- Use multiple ViewResolvers in Spring Boot
- Use Twitter Bootstrap in Spring Boot
- Spring Boot Interceptors Tutorial with Examples
- Spring Boot, Spring JDBC and Spring Transaction Tutorial with Examples
- Spring JDBC Tutorial with Examples
- Spring Boot, JPA and Spring Transaction Tutorial with Examples
- Spring Boot and Spring Data JPA Tutorial with Examples
- Spring Boot, Hibernate and Spring Transaction Tutorial with Examples
- Integrating Spring Boot, JPA and H2 Database
- Spring Boot and MongoDB Tutorial with Examples
- Use Multiple DataSources with Spring Boot and JPA
- Use Multiple DataSources with Spring Boot and RoutingDataSource
- Create a Login Application with Spring Boot, Spring Security, Spring JDBC
- Create a Login Application with Spring Boot, Spring Security, JPA
- Create a User Registration Application with Spring Boot, Spring Form Validation
- Example of OAuth2 Social Login in Spring Boot
- Run background scheduled tasks in Spring
- CRUD Restful Web Service Example with Spring Boot
- Spring Boot Restful Client with RestTemplate Example
- CRUD Example with Spring Boot, REST and AngularJS
- Secure Spring Boot RESTful Service using Basic Authentication
- Secure Spring Boot RESTful Service using Auth0 JWT
- Spring Boot File Upload Example
- Spring Boot File Download Example
- Spring Boot File Upload with jQuery Ajax Example
- Spring Boot File Upload with AngularJS Example
- Create a Shopping Cart Web Application with Spring Boot, Hibernate
- Spring Email Tutorial with Examples
- Create a simple Chat application with Spring Boot and Websocket
- Deploy Spring Boot Application on Tomcat Server
- Deploy Spring Boot Application on Oracle WebLogic Server
- Install a free Let's Encrypt SSL certificate for Spring Boot
- Configure Spring Boot to redirect HTTP to HTTPS
- Fetch data with Spring Data JPA DTO Projections
Show More