Spring MVC and Velocity Tutorial with Examples
1. Introduction
The document is based on:
Spring MVC 4.x
Eclipse 4.6 (NEON)
Apache Velocity is a Java-based template engine that provides a template language to reference objects defined in Java code. It aims to ensure clean separation between the presentation tier and business tiers in a Web application (the model–view–controller design pattern).
Velocity is an open source software project hosted by the Apache Software Foundation. It is released under the Apache License.
Velocity is an open source software project hosted by the Apache Software Foundation. It is released under the Apache License.
Thus, in essence, Velocity can be used on View for displaying data. Velocity is a language of template.
Note: Starting from version 4.3, Spring does not support Velocity, and the Velocity will be be removed from Spring from version 5 (In the future).Here is a conversation or quarrel between Velocity (Apache) developers and Spring ones revolving around the reason why Velocity is not supported on the Spring framework.
2. Create Maven Project
- File/New/Other...
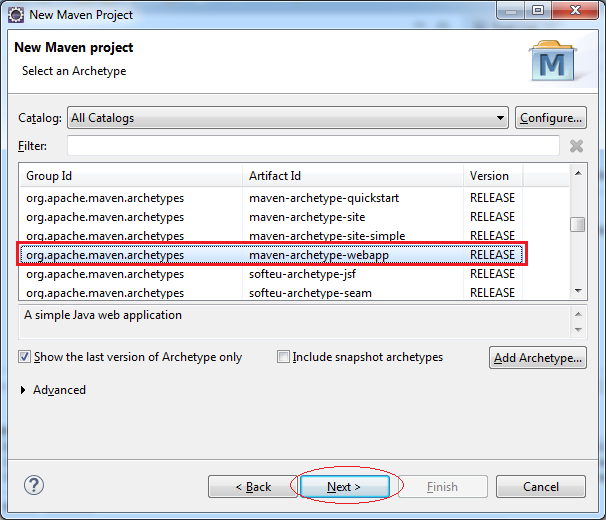
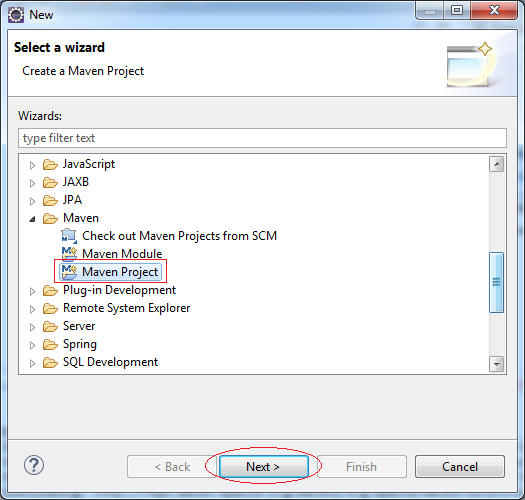
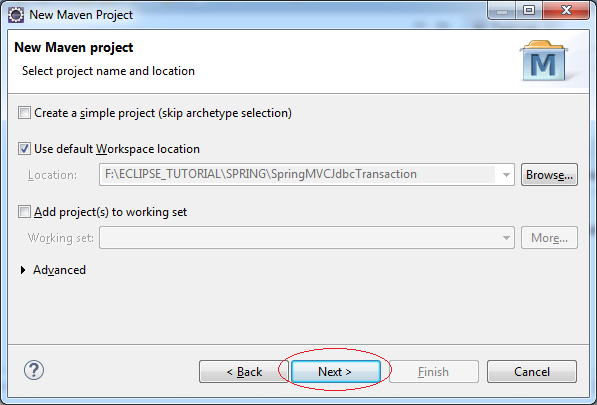
Enter:
- Group Id: org.o7planning
- Artifact Id: SpringMVCVelocity
- Package: org.o7planning.tutorial.springmvcvelocity
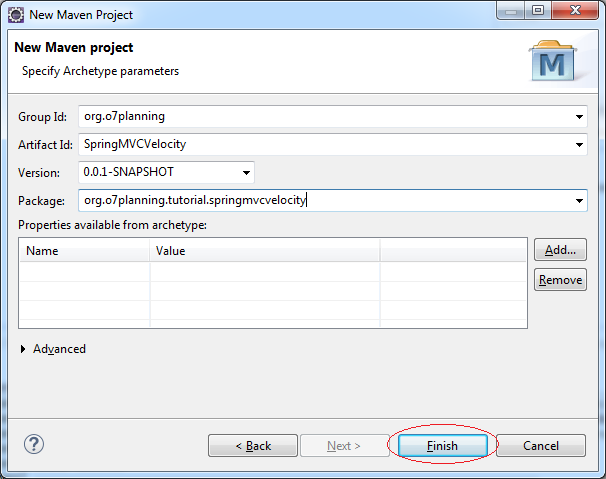
Project was created:
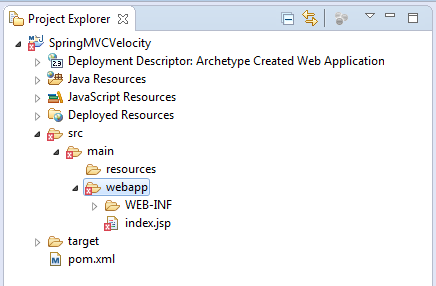
Do not worry with the error message when Project has been created. The reason is that you have not declared Servlet library.
Note:
Eclipse 4.4 (Luna) create Maven project structure may be wrong. You need to check.
Eclipse 4.4 (Luna) create Maven project structure may be wrong. You need to check.
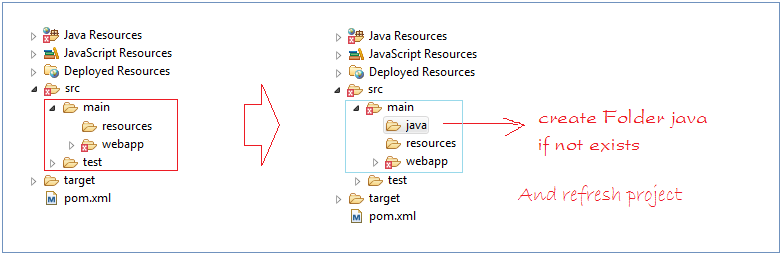
3. Configure maven
pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0
http://maven.apache.org/maven-v4_0_0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.o7planning</groupId>
<artifactId>SpringMVCVelocity</artifactId>
<packaging>war</packaging>
<version>0.0.1-SNAPSHOT</version>
<name>SpringMVCVelocity Maven Webapp</name>
<url>http://maven.apache.org</url>
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>3.8.1</version>
<scope>test</scope>
</dependency>
<!-- Spring framework START -->
<!-- http://mvnrepository.com/artifact/org.springframework/spring-core -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-core</artifactId>
<version>4.1.4.RELEASE</version>
</dependency>
<!-- http://mvnrepository.com/artifact/org.springframework/spring-web -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-web</artifactId>
<version>4.1.4.RELEASE</version>
</dependency>
<!-- http://mvnrepository.com/artifact/org.springframework/spring-webmvc -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>4.1.4.RELEASE</version>
</dependency>
<!-- contains: VelocityEngineFactory -->
<!-- http://mvnrepository.com/artifact/org.springframework/spring-context-support -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context-support</artifactId>
<version>4.1.4.RELEASE</version>
</dependency>
<!-- Spring framework END -->
<!-- Servlet API -->
<!-- http://mvnrepository.com/artifact/javax.servlet/javax.servlet-api -->
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
<version>3.1.0</version>
<scope>provided</scope>
</dependency>
<!-- http://mvnrepository.com/artifact/org.apache.velocity/velocity -->
<dependency>
<groupId>org.apache.velocity</groupId>
<artifactId>velocity</artifactId>
<version>1.7</version>
</dependency>
<!-- http://mvnrepository.com/artifact/org.apache.velocity/velocity-tools -->
<dependency>
<groupId>org.apache.velocity</groupId>
<artifactId>velocity-tools</artifactId>
<version>2.0</version>
</dependency>
</dependencies>
<build>
<finalName>SpringMVCVelocity</finalName>
<plugins>
<!-- Config: Maven Tomcat Plugin -->
<!-- http://mvnrepository.com/artifact/org.apache.tomcat.maven/tomcat7-maven-plugin -->
<plugin>
<groupId>org.apache.tomcat.maven</groupId>
<artifactId>tomcat7-maven-plugin</artifactId>
<version>2.2</version>
<!-- Config: contextPath and Port (Default - /SpringMVCVelocity : 8080) -->
<!--
<configuration>
<path>/</path>
<port>8899</port>
</configuration>
-->
</plugin>
</plugins>
</build>
</project>
4. Configure Spring
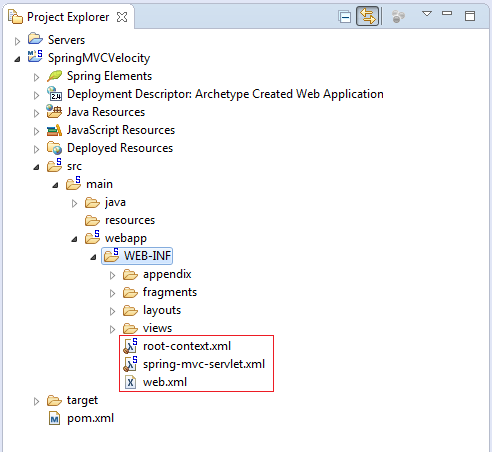
web.xml
<web-app id="WebApp_ID" version="2.4"
xmlns="http://java.sun.com/xml/ns/j2ee" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://java.sun.com/xml/ns/j2ee
http://java.sun.com/xml/ns/j2ee/web-app_2_4.xsd">
<display-name>Archetype Created Web Application</display-name>
<servlet>
<servlet-name>spring-mvc</servlet-name>
<servlet-class>
org.springframework.web.servlet.DispatcherServlet
</servlet-class>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>spring-mvc</servlet-name>
<url-pattern>/</url-pattern>
</servlet-mapping>
<!-- Other XML Configuration -->
<!-- Load by Spring ContextLoaderListener -->
<context-param>
<param-name>contextConfigLocation</param-name>
<param-value>
/WEB-INF/root-context.xml,
/WEB-INF/spring-mvc-servlet.xml
</param-value>
</context-param>
<!-- Spring ContextLoaderListener -->
<listener>
<listener-class>org.springframework.web.context.ContextLoaderListener</listener-class>
</listener>
</web-app>
spring-mvc-servlet.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:mvc="http://www.springframework.org/schema/mvc"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context-4.1.xsd
http://www.springframework.org/schema/mvc
http://www.springframework.org/schema/mvc/spring-mvc-4.1.xsd">
<!-- Register the annotated components in the container eg : annotated controllers -->
<context:component-scan base-package="org.o7planning.tutorial.springmvcvelocity.*" />
<!-- Tell the container that we are going to use annotations -->
<context:annotation-config />
<bean id="velocityConfig"
class="org.springframework.web.servlet.view.velocity.VelocityConfigurer">
<property name="resourceLoaderPath">
<value>/</value>
</property>
</bean>
<bean id="viewResolver"
class="org.springframework.web.servlet.view.velocity.VelocityLayoutViewResolver">
<property name="cache" value="true" />
<property name="prefix" value="/WEB-INF/views/" />
<property name="layoutUrl" value="/WEB-INF/layouts/layout.vm" />
<property name="suffix" value=".vm" />
</bean>
</beans>
root-context.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd">
<!-- Empty -->
</beans>
5. Views
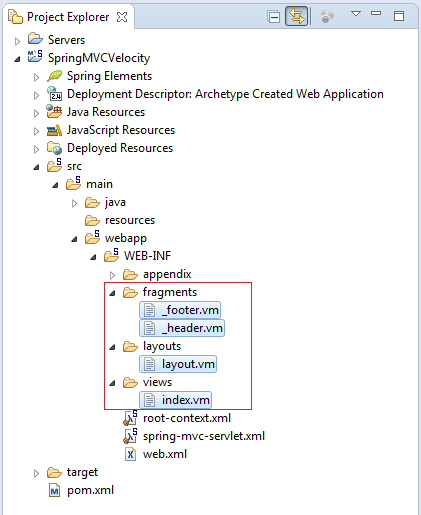
/WEB-INF/fragments/_header.vm
<div style="background: #E0E0E0; height: 80px; padding: 5px;">
<div style="float: left">
<h1>My Site</h1>
</div>
<div style="float: right; padding: 10px;">
Search <input name="search">
</div>
</div>
/WEB-INF/fragments/_footer.vm
<div
style="background: #E0E0E0; text-align: center; padding: 5px; margin-top: 10px;">
@Copyright mysite.com
</div>
/WEB-INF/layouts/layout.vm
<html>
<head>
<title>Spring & Velocity</title>
</head>
<body>
<div>
#parse("/WEB-INF/fragments/_header.vm")
</div>
<div>
<!-- View abc.vm is inserted here -->
<!-- Noi dung View abc.vm se duoc tren tai day -->
$screen_content
</div>
<div>
#parse("/WEB-INF/fragments/_footer.vm")
</div>
</body>
</html>
The $screen_content variable is used by the VelocityLayoutViewResolver to include the result of an other VM in the layout
/WEB-INF/views/index.vm
<h1>Index</h1>
<h2>Department list</h2>
<table border="1">
<tr>
<th>Dept No</th>
<th>Dept Name</th>
</tr>
#foreach($dept in $departments)
<tr>
<td>$dept.deptNo</td>
<td>$dept.deptName</td>
</tr>
#end
</table>
6. Java Classes
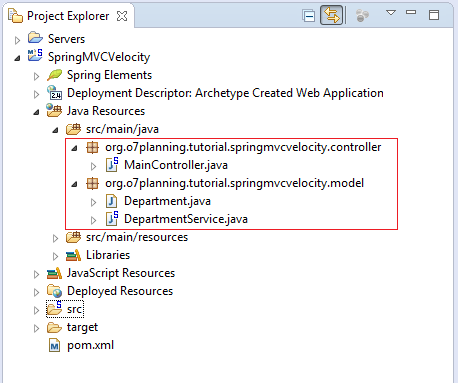
Department.java
package org.o7planning.tutorial.springmvcvelocity.model;
public class Department {
private Integer deptId;
private String deptNo;
private String deptName;
private String location;
public Department() {
}
public Department(Integer deptId, String deptName, String location) {
this.deptId = deptId;
this.deptNo = "D" + deptId;
this.deptName = deptName;
this.location = location;
}
public Integer getDeptId() {
return deptId;
}
public void setDeptId(Integer deptId) {
this.deptId = deptId;
}
public String getDeptNo() {
return deptNo;
}
public void setDeptNo(String deptNo) {
this.deptNo = deptNo;
}
public String getDeptName() {
return deptName;
}
public void setDeptName(String deptName) {
this.deptName = deptName;
}
public String getLocation() {
return location;
}
public void setLocation(String location) {
this.location = location;
}
}
DepartmentService.java
package org.o7planning.tutorial.springmvcvelocity.model;
import java.util.ArrayList;
import java.util.List;
import org.springframework.stereotype.Service;
@Service
public class DepartmentService {
public List<Department> listDepartment() {
List<Department> list = new ArrayList<Department>();
list.add(new Department(1, "Operations", "Chicago"));
list.add(new Department(2, "HR", "Hanoi"));
return list;
}
}
The classes annotated by @Controller or @Service be regarded as the Bean, and Spring will automatically inject dependencies in the Field annotated by @Autowired annotation.
MainController.java
package org.o7planning.tutorial.springmvcvelocity.controller;
import java.util.List;
import org.o7planning.tutorial.springmvcvelocity.model.Department;
import org.o7planning.tutorial.springmvcvelocity.model.DepartmentService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
@Controller
public class MainController {
@Autowired
private DepartmentService deptService;
@RequestMapping(value = { "/", "/welcome" }, method = RequestMethod.GET)
public String welcomePage(Model model) {
List<Department> list = deptService.listDepartment();
model.addAttribute("departments", list);
return "index";
}
}
Spring MVC Tutorials
- Spring Tutorial for Beginners
- Install Spring Tool Suite for Eclipse
- Spring MVC Tutorial for Beginners - Hello Spring 4 MVC
- Configure Static Resources in Spring MVC
- Spring MVC Interceptors Tutorial with Examples
- Create a Multiple Languages web application with Spring MVC
- Spring MVC File Upload Tutorial with Examples
- Simple Login Java Web Application using Spring MVC, Spring Security and Spring JDBC
- Spring MVC Security with Hibernate Tutorial with Examples
- Spring MVC Security and Spring JDBC Tutorial (XML Config)
- Social Login in Spring MVC with Spring Social Security
- Spring MVC and Velocity Tutorial with Examples
- Spring MVC and FreeMarker Tutorial with Examples
- Use Template in Spring MVC with Apache Tiles
- Spring MVC and Spring JDBC Transaction Tutorial with Examples
- Use Multiple DataSources in Spring MVC
- Spring MVC and Hibernate Transaction Tutorial with Examples
- Spring MVC Form Handling and Hibernate Tutorial with Examples
- Run background scheduled tasks in Spring
- Create a Java Shopping Cart Web Application using Spring MVC and Hibernate
- Simple CRUD example with Spring MVC RESTful Web Service
- Deploy Spring MVC on Oracle WebLogic Server
Show More