Application Monitoring with Spring Boot Actuator
1. What is Spring Boot Actuator?
The Spring Boot Actuator is a sub-project ofSpring Boot project. It is built to collect and monitor application information. You can embed it in your application and use its features. To monitor the application you need to access the built-in endpoints of the Spring Boot Actuator , and you can also create your own endpoints if you want.
In this post, I am going to guide you for using the Spring Boot Actuator in the Spring Boot application, version 2.0.0.M7 or newer.
It is noted that the Spring Boot Actuator has a wide range of changes in version 2.x compared to version 1.x, therefore, let's ensure that you are working with the Spring Boot, version 2.0.0.M7 or newer.
2. Create Spring Boot project
The Spring Boot Actuator is a sub-project (a complete product) in the Spring ecosystem, and you can embed it into your existing Spring Boot project. All things that you need to do is to declare the Spring Boot Actuator libraries and some configuration information in the application.properties file. In this lesson, I will create a Spring Boot project and embed the Spring Boot Actuator into it. Our goal is to learn about the features provided by the Spring Boot Actuator.
OK!, On the Eclipse, create a Spring Boot project.
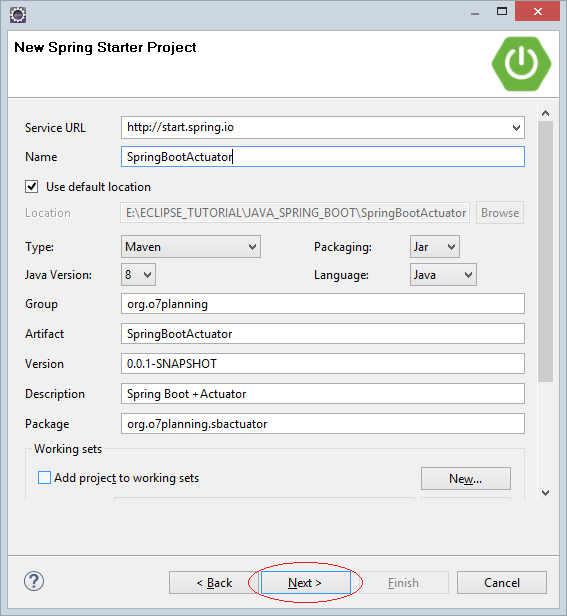
Herein, we will select version 2.0.0.M7 or newer.
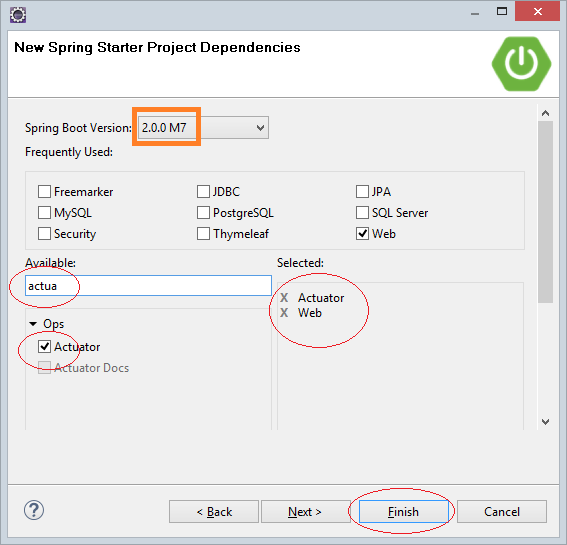
pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0
http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.o7planning</groupId>
<artifactId>SpringBootActuator</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>jar</packaging>
<name>SpringBootActuator</name>
<description>Spring Boot +Actuator</description>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.0.0.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
3. Configurate the Spring Boot Actuator
Add the configuration to the application.properties file:
application.properties
server.port=8080
management.server.port=8090
management.endpoints.web.exposure.include=*
management.endpoint.shutdown.enabled=true
- server.port=8080
Your application will run on port 8080 which is the default port and you can change it if you want.
- management.server.port=8090
The endpoints related to the monitoring of Spring Boot Actuator will be updated by a port other than port 8080 above. Its purpose is to avoid mistake and enhance security. However, this í not mandatory.
- management.endpoints.web.exposure.include=*
By default, not all Endpoints of theSpring Boot Actuator are activated. Use * to activate all these Endpoints.
- management.endpoint.shutdown.enabled=true
shutdown is a special Endpoint ofSpring Boot Actuator. It allows you to shut down applications safely without using commands such as "Kill process", "end task" of the operating system
4. The Endpoints of Spring Boot Actuator
MainController.java
package org.o7planning.sbactuator.controller;
import javax.servlet.http.HttpServletRequest;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.ResponseBody;
@Controller
public class MainController {
@ResponseBody
@RequestMapping(path = "/")
public String home(HttpServletRequest request) {
String contextPath = request.getContextPath();
String host = request.getServerName();
// Spring Boot >= 2.0.0.M7
String endpointBasePath = "/actuator";
StringBuilder sb = new StringBuilder();
sb.append("<h2>Sprig Boot Actuator</h2>");
sb.append("<ul>");
// http://localhost:8090/actuator
String url = "http://" + host + ":8090" + contextPath + endpointBasePath;
sb.append("<li><a href='" + url + "'>" + url + "</a></li>");
sb.append("</ul>");
return sb.toString();
}
}
Run your application adn visit the following link:
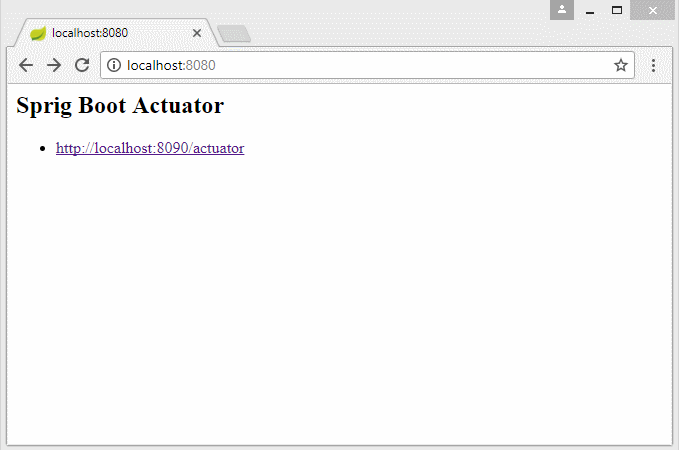
Note: The data provided by the endpoints of the Spring Boot Actuator has JSON format, therefore, for better visibility, you can reformat this data. For example, the following website provides an online tool for formatting the JSON:
/actuator
/actuator is an endpoint, which provides a list of other endpoints of the Spring Boot Actuator that you can access.
/actuator
{
"_links": {
"self": {
"href": "http://localhost:8090/actuator",
"templated": false
},
"auditevents": {
"href": "http://localhost:8090/actuator/auditevents",
"templated": false
},
"beans": {
"href": "http://localhost:8090/actuator/beans",
"templated": false
},
"health": {
"href": "http://localhost:8090/actuator/health",
"templated": false
},
"conditions": {
"href": "http://localhost:8090/actuator/conditions",
"templated": false
},
"shutdown": {
"href": "http://localhost:8090/actuator/shutdown",
"templated": false
},
"configprops": {
"href": "http://localhost:8090/actuator/configprops",
"templated": false
},
"env": {
"href": "http://localhost:8090/actuator/env",
"templated": false
},
"env-toMatch": {
"href": "http://localhost:8090/actuator/env/{toMatch}",
"templated": true
},
"info": {
"href": "http://localhost:8090/actuator/info",
"templated": false
},
"loggers": {
"href": "http://localhost:8090/actuator/loggers",
"templated": false
},
"loggers-name": {
"href": "http://localhost:8090/actuator/loggers/{name}",
"templated": true
},
"heapdump": {
"href": "http://localhost:8090/actuator/heapdump",
"templated": false
},
"threaddump": {
"href": "http://localhost:8090/actuator/threaddump",
"templated": false
},
"metrics-requiredMetricName": {
"href": "http://localhost:8090/actuator/metrics/{requiredMetricName}",
"templated": true
},
"metrics": {
"href": "http://localhost:8090/actuator/metrics",
"templated": false
},
"scheduledtasks": {
"href": "http://localhost:8090/actuator/scheduledtasks",
"templated": false
},
"trace": {
"href": "http://localhost:8090/actuator/trace",
"templated": false
},
"mappings": {
"href": "http://localhost:8090/actuator/mappings",
"templated": false
}
}
}
/actuator/health
/actuator/health provides you with information on the health of the application. The UP or DOWN status and other information on the hard drive such as hard drive size, used and unused capacity.
/actuator/health
{
"status": "UP",
"details": {
"diskSpace": {
"status": "UP",
"details": {
"total": 75812040704,
"free": 8067600384,
"threshold": 10485760
}
}
}
}
actuator/metrics
This Endpoint provides you with a list of metrics (Measurement standard)
actuator/metrics
{
"names": [
"jvm.memory.committed",
"jvm.buffer.memory.used",
"jvm.buffer.count",
"logback.events",
"process.uptime",
"jvm.memory.max",
"jvm.memory.used",
"jvm.buffer.total.capacity",
"system.cpu.count",
"process.start.time"
]
}
And you can view the information of a specific metric.
actuator/metrics/{requiredMetricName}
/actuator/metrics/jvm.memory.used
{
"name": "jvm.memory.used",
"measurements": [
{
"statistic": "Value",
"value": 109937744
}
],
"availableTags": [
{
"tag": "area",
"values": [
"heap",
"nonheap",
"nonheap",
"heap",
"nonheap",
"nonheap",
"heap"
]
},
{
"tag": "id",
"values": [
"G1 Old Gen",
"Compressed Class Space",
"CodeHeap 'non-nmethods'",
"G1 Survivor Space",
"Metaspace",
"CodeHeap 'non-profiled nmethods'",
"G1 Eden Space"
]
}
]
}
/actuator/beans
The /actuator/beans provides you with list of Spring BEAN managed in your application.
/actuator/beans
{
"contextId": "application:8080",
"beans": {
"endpointCachingOperationInvokerAdvisor": {
"aliases": [],
"scope": "singleton",
"type": "org.springframework.boot.actuate.endpoint.cache.CachingOperationInvokerAdvisor",
"resource": "class path resource [org/springframework/boot/actuate/autoconfigure/endpoint/EndpointAutoConfiguration.class]",
"dependencies": [
"environment"
]
},
"defaultServletHandlerMapping": {
"aliases": [],
"scope": "singleton",
"type": "org.springframework.web.servlet.config.annotation.WebMvcConfigurationSupport$EmptyHandlerMapping",
"resource": "class path resource [org/springframework/boot/autoconfigure/web/servlet/WebMvcAutoConfiguration$EnableWebMvcConfiguration.class]",
"dependencies": []
}
....
},
"parent": null
}
/actuator/env
The /actuator/env provides you with all information on environment where your application is running, for example, name of operating system, classpath, Java version,...
/actuator/env
{
"activeProfiles": [],
"propertySources": [
{
"name": "server.ports",
"properties": {
"local.management.port": {
"value": 8090
},
"local.server.port": {
"value": 8080
}
}
},
{
"name": "servletContextInitParams",
"properties": {}
},
{
"name": "systemProperties",
"properties": {
"sun.desktop": {
"value": "windows"
},
"awt.toolkit": {
"value": "sun.awt.windows.WToolkit"
},
"java.specification.version": {
"value": "9"
},
"file.encoding.pkg": {
"value": "sun.io"
},
"sun.cpu.isalist": {
"value": "amd64"
},
"sun.jnu.encoding": {
"value": "Cp1252"
},
"java.class.path": {
"value": "C:\\Users\\tran\\.m2\\repository\\org\\springframework\\spring-jcl\\5.0.2.RELEASE\\spring-jcl-5.0.2.RELEASE.jar;...."
},
"com.sun.management.jmxremote.authenticate": {
"value": "false"
},
"java.vm.vendor": {
"value": "Oracle Corporation"
},
"sun.arch.data.model": {
"value": "64"
},
"user.variant": {
"value": ""
},
"java.vendor.url": {
"value": "http://java.oracle.com/"
},
"catalina.useNaming": {
"value": "false"
},
"user.timezone": {
"value": "Asia/Bangkok"
},
"os.name": {
"value": "Windows 8.1"
},
"java.vm.specification.version": {
"value": "9"
},
"sun.java.launcher": {
"value": "SUN_STANDARD"
},
"user.country": {
"value": "US"
},
"sun.boot.library.path": {
"value": "C:\\DevPrograms\\Java\\jre-9.0.1\\bin"
},
"com.sun.management.jmxremote.ssl": {
"value": "false"
},
"spring.application.admin.enabled": {
"value": "true"
},
"sun.java.command": {
"value": "org.o7planning.sbactuator.SpringBootActuatorApplication"
},
"com.sun.management.jmxremote": {
"value": ""
},
"jdk.debug": {
"value": "release"
},
"sun.cpu.endian": {
"value": "little"
},
"user.home": {
"value": "C:\\Users\\tran"
},
"user.language": {
"value": "en"
},
"java.specification.vendor": {
"value": "Oracle Corporation"
},
"java.home": {
"value": "C:\\DevPrograms\\Java\\jre-9.0.1"
},
"file.separator": {
"value": "\\"
},
"java.vm.compressedOopsMode": {
"value": "Zero based"
},
"line.separator": {
"value": "\r\n"
},
"java.specification.name": {
"value": "Java Platform API Specification"
},
"java.vm.specification.vendor": {
"value": "Oracle Corporation"
},
"java.awt.graphicsenv": {
"value": "sun.awt.Win32GraphicsEnvironment"
},
"java.awt.headless": {
"value": "true"
},
"user.script": {
"value": ""
},
"sun.management.compiler": {
"value": "HotSpot 64-Bit Tiered Compilers"
},
"java.runtime.version": {
"value": "9.0.1+11"
},
"user.name": {
"value": "tran"
},
"path.separator": {
"value": ";"
},
"os.version": {
"value": "6.3"
},
"java.runtime.name": {
"value": "Java(TM) SE Runtime Environment"
},
"file.encoding": {
"value": "UTF-8"
},
"spring.beaninfo.ignore": {
"value": "true"
},
"java.vm.name": {
"value": "Java HotSpot(TM) 64-Bit Server VM"
},
"java.vendor.url.bug": {
"value": "http://bugreport.java.com/bugreport/"
},
"java.io.tmpdir": {
"value": "C:\\Users\\tran\\AppData\\Local\\Temp\\"
},
"catalina.home": {
"value": "C:\\Users\\tran\\AppData\\Local\\Temp\\tomcat.16949807720416048110.8080"
},
"java.version": {
"value": "9.0.1"
},
"com.sun.management.jmxremote.port": {
"value": "54408"
},
"user.dir": {
"value": "E:\\ECLIPSE_TUTORIAL\\JAVA_SPRING_BOOT\\SpringBootActuator"
},
"os.arch": {
"value": "amd64"
},
"java.vm.specification.name": {
"value": "Java Virtual Machine Specification"
},
"PID": {
"value": "5372"
},
"java.awt.printerjob": {
"value": "sun.awt.windows.WPrinterJob"
},
"sun.os.patch.level": {
"value": ""
},
"catalina.base": {
"value": "C:\\Users\\tran\\AppData\\Local\\Temp\\tomcat.8934969984130443549.8090"
},
"java.library.path": {
"value": "C:\\DevPrograms\\Java\\jre-9.0.1\\bin;...;C:\\Windows\\System32\\WindowsPowerShell\\v1.0\\;C:\\Program Files\\EmEditor;."
},
"java.vm.info": {
"value": "mixed mode"
},
"java.vendor": {
"value": "Oracle Corporation"
},
"java.vm.version": {
"value": "9.0.1+11"
},
"java.rmi.server.hostname": {
"value": "localhost"
},
"java.rmi.server.randomIDs": {
"value": "true"
},
"sun.io.unicode.encoding": {
"value": "UnicodeLittle"
},
"java.class.version": {
"value": "53.0"
}
}
},
{
"name": "systemEnvironment",
"properties": {
"USERDOMAIN_ROAMINGPROFILE": {
"value": "tran-pc",
"origin": "System Environment Property \"USERDOMAIN_ROAMINGPROFILE\""
},
"LOCALAPPDATA": {
"value": "C:\\Users\\tran\\AppData\\Local",
"origin": "System Environment Property \"LOCALAPPDATA\""
},
"PROCESSOR_LEVEL": {
"value": "6",
"origin": "System Environment Property \"PROCESSOR_LEVEL\""
},
"FP_NO_HOST_CHECK": {
"value": "NO",
"origin": "System Environment Property \"FP_NO_HOST_CHECK\""
},
"USERDOMAIN": {
"value": "tran-pc",
"origin": "System Environment Property \"USERDOMAIN\""
},
"LOGONSERVER": {
"value": "\\\\TRAN-PC",
"origin": "System Environment Property \"LOGONSERVER\""
},
"SESSIONNAME": {
"value": "Console",
"origin": "System Environment Property \"SESSIONNAME\""
},
"ALLUSERSPROFILE": {
"value": "C:\\ProgramData",
"origin": "System Environment Property \"ALLUSERSPROFILE\""
},
"PROCESSOR_ARCHITECTURE": {
"value": "AMD64",
"origin": "System Environment Property \"PROCESSOR_ARCHITECTURE\""
},
"PSModulePath": {
"value": "C:\\Windows\\system32\\WindowsPowerShell\\v1.0\\Modules\\",
"origin": "System Environment Property \"PSModulePath\""
},
"SystemDrive": {
"value": "C:",
"origin": "System Environment Property \"SystemDrive\""
},
"APPDATA": {
"value": "C:\\Users\\tran\\AppData\\Roaming",
"origin": "System Environment Property \"APPDATA\""
},
"USERNAME": {
"value": "tran",
"origin": "System Environment Property \"USERNAME\""
},
"ProgramFiles(x86)": {
"value": "C:\\Program Files (x86)",
"origin": "System Environment Property \"ProgramFiles(x86)\""
},
"CommonProgramFiles": {
"value": "C:\\Program Files\\Common Files",
"origin": "System Environment Property \"CommonProgramFiles\""
},
"Path": {
"value": "D:\\DEV_PROGRAMS\\Oracle12c\\product\\12.2.0\\dbhome_1\\bin;...;C:\\Program Files\\EmEditor",
"origin": "System Environment Property \"Path\""
},
"PATHEXT": {
"value": ".COM;.EXE;.BAT;.CMD;.VBS;.VBE;.JS;.JSE;.WSF;.WSH;.MSC",
"origin": "System Environment Property \"PATHEXT\""
},
"OS": {
"value": "Windows_NT",
"origin": "System Environment Property \"OS\""
},
"COMPUTERNAME": {
"value": "TRAN-PC",
"origin": "System Environment Property \"COMPUTERNAME\""
},
"PROCESSOR_REVISION": {
"value": "3c03",
"origin": "System Environment Property \"PROCESSOR_REVISION\""
},
"CommonProgramW6432": {
"value": "C:\\Program Files\\Common Files",
"origin": "System Environment Property \"CommonProgramW6432\""
},
"ComSpec": {
"value": "C:\\Windows\\system32\\cmd.exe",
"origin": "System Environment Property \"ComSpec\""
},
"ProgramData": {
"value": "C:\\ProgramData",
"origin": "System Environment Property \"ProgramData\""
},
"ProgramW6432": {
"value": "C:\\Program Files",
"origin": "System Environment Property \"ProgramW6432\""
},
"HOMEPATH": {
"value": "\\Users\\tran",
"origin": "System Environment Property \"HOMEPATH\""
},
"SystemRoot": {
"value": "C:\\Windows",
"origin": "System Environment Property \"SystemRoot\""
},
"TEMP": {
"value": "C:\\Users\\tran\\AppData\\Local\\Temp",
"origin": "System Environment Property \"TEMP\""
},
"HOMEDRIVE": {
"value": "C:",
"origin": "System Environment Property \"HOMEDRIVE\""
},
"PROCESSOR_IDENTIFIER": {
"value": "Intel64 Family 6 Model 60 Stepping 3, GenuineIntel",
"origin": "System Environment Property \"PROCESSOR_IDENTIFIER\""
},
"USERPROFILE": {
"value": "C:\\Users\\tran",
"origin": "System Environment Property \"USERPROFILE\""
},
"TMP": {
"value": "C:\\Users\\tran\\AppData\\Local\\Temp",
"origin": "System Environment Property \"TMP\""
},
"CommonProgramFiles(x86)": {
"value": "C:\\Program Files (x86)\\Common Files",
"origin": "System Environment Property \"CommonProgramFiles(x86)\""
},
"ProgramFiles": {
"value": "C:\\Program Files",
"origin": "System Environment Property \"ProgramFiles\""
},
"PUBLIC": {
"value": "C:\\Users\\Public",
"origin": "System Environment Property \"PUBLIC\""
},
"NUMBER_OF_PROCESSORS": {
"value": "8",
"origin": "System Environment Property \"NUMBER_OF_PROCESSORS\""
},
"windir": {
"value": "C:\\Windows",
"origin": "System Environment Property \"windir\""
}
}
},
{
"name": "applicationConfig: [classpath:/application.properties]",
"properties": {
"server.port": {
"value": "8080",
"origin": "class path resource [application.properties]:1:13"
},
"management.server.port": {
"value": "8090",
"origin": "class path resource [application.properties]:2:24"
},
"management.endpoints.web.expose": {
"value": "*",
"origin": "class path resource [application.properties]:3:33"
},
"management.endpoint.shutdown.enabled": {
"value": "true",
"origin": "class path resource [application.properties]:4:38"
}
}
}
]
}
/actuator/info
The /actuator/info provides your customization information. It is by default a empty information. Therefore, you need to create a Spring BEAN to provide this information.
BuildInfoContributor.java
package org.o7planning.sbactuator.monitoring;
import java.util.HashMap;
import java.util.Map;
import org.springframework.boot.actuate.info.Info;
import org.springframework.boot.actuate.info.InfoContributor;
import org.springframework.stereotype.Component;
@Component
public class BuildInfoContributor implements InfoContributor {
@Override
public void contribute(Info.Builder builder) {
Map<String,String> data= new HashMap<String,String>();
data.put("build.version", "2.0.0.M7");
builder.withDetail("buildInfo", data);
}
}
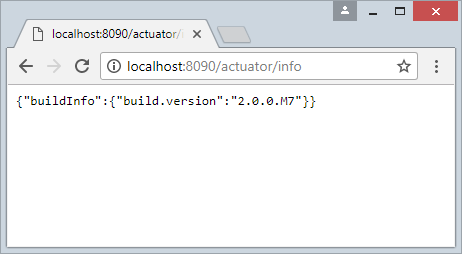
5. /actuator/shutdown
This is an endpoint helping you shut down an application. You have to call it with the POST method. If it is successful, you will get a "Shutting down, bye ..." message
ShutdownController.java
package org.o7planning.sbactuator.controller;
import org.springframework.http.HttpEntity;
import org.springframework.http.HttpHeaders;
import org.springframework.http.MediaType;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.ResponseBody;
import org.springframework.web.client.RestTemplate;
@Controller
public class ShutdownController {
@ResponseBody
@RequestMapping(path = "/shutdown")
public String callActuatorShutdown() {
// Actuator Shutdown Endpoint:
String url = "http://localhost:8090/actuator/shutdown";
// Http Headers
HttpHeaders headers = new HttpHeaders();
headers.add("Accept", MediaType.APPLICATION_JSON_VALUE);
headers.setContentType(MediaType.APPLICATION_JSON);
RestTemplate restTemplate = new RestTemplate();
// Data attached to the request.
HttpEntity<String> requestBody = new HttpEntity<>("", headers);
// Send request with POST method.
String e = restTemplate.postForObject(url, requestBody, String.class);
return "Result: " + e;
}
}
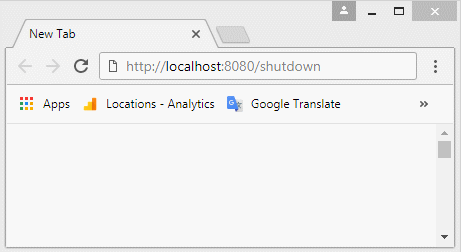
Spring Boot Tutorials
- Install Spring Tool Suite for Eclipse
- Spring Tutorial for Beginners
- Spring Boot Tutorial for Beginners
- Spring Boot Common Properties
- Spring Boot and Thymeleaf Tutorial with Examples
- Spring Boot and FreeMarker Tutorial with Examples
- Spring Boot and Groovy Tutorial with Examples
- Spring Boot and Mustache Tutorial with Examples
- Spring Boot and JSP Tutorial with Examples
- Spring Boot, Apache Tiles, JSP Tutorial with Examples
- Use Logging in Spring Boot
- Application Monitoring with Spring Boot Actuator
- Create a Multi Language web application with Spring Boot
- Use multiple ViewResolvers in Spring Boot
- Use Twitter Bootstrap in Spring Boot
- Spring Boot Interceptors Tutorial with Examples
- Spring Boot, Spring JDBC and Spring Transaction Tutorial with Examples
- Spring JDBC Tutorial with Examples
- Spring Boot, JPA and Spring Transaction Tutorial with Examples
- Spring Boot and Spring Data JPA Tutorial with Examples
- Spring Boot, Hibernate and Spring Transaction Tutorial with Examples
- Integrating Spring Boot, JPA and H2 Database
- Spring Boot and MongoDB Tutorial with Examples
- Use Multiple DataSources with Spring Boot and JPA
- Use Multiple DataSources with Spring Boot and RoutingDataSource
- Create a Login Application with Spring Boot, Spring Security, Spring JDBC
- Create a Login Application with Spring Boot, Spring Security, JPA
- Create a User Registration Application with Spring Boot, Spring Form Validation
- Example of OAuth2 Social Login in Spring Boot
- Run background scheduled tasks in Spring
- CRUD Restful Web Service Example with Spring Boot
- Spring Boot Restful Client with RestTemplate Example
- CRUD Example with Spring Boot, REST and AngularJS
- Secure Spring Boot RESTful Service using Basic Authentication
- Secure Spring Boot RESTful Service using Auth0 JWT
- Spring Boot File Upload Example
- Spring Boot File Download Example
- Spring Boot File Upload with jQuery Ajax Example
- Spring Boot File Upload with AngularJS Example
- Create a Shopping Cart Web Application with Spring Boot, Hibernate
- Spring Email Tutorial with Examples
- Create a simple Chat application with Spring Boot and Websocket
- Deploy Spring Boot Application on Tomcat Server
- Deploy Spring Boot Application on Oracle WebLogic Server
- Install a free Let's Encrypt SSL certificate for Spring Boot
- Configure Spring Boot to redirect HTTP to HTTPS
- Fetch data with Spring Data JPA DTO Projections
Show More