Flutter Border Tutorial with Examples
1. Border
Border creates a border for a box, including 4 edges: top, bottom, left, right.
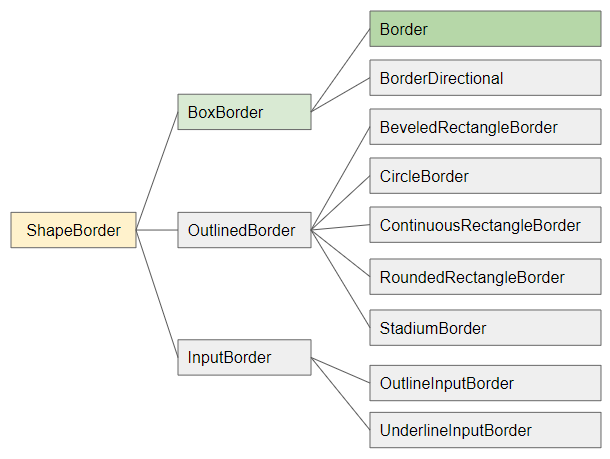
- ShapeBorder
- BoxBorder
- BorderDirectional
- ContinuousRectangleBorder
- CircleBorder
- RoundedRectangleBorder
- StadiumBorder
- BeveledRectangleBorder
- OutlineInputBorder
- UnderlineInputBorder
- InputBorder
- OutlinedBorder
Border constructor
const Border (
{BorderSide top: BorderSide.none,
BorderSide right: BorderSide.none,
BorderSide bottom: BorderSide.none,
BorderSide left: BorderSide.none}
)
- Flutter BorderSide
Example:
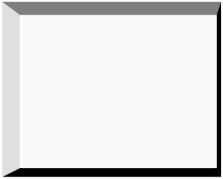
(ex1)
Container(
width: 250,
height: 200,
decoration: BoxDecoration(
border: Border(
top: BorderSide(width: 15.0, color: Color(0xFFFF7F7F7F)),
left: BorderSide(width: 20.0, color: Color(0xFFFFDFDFDF)),
right: BorderSide(width: 5.0, color: Color(0xFFFF000000)),
bottom: BorderSide(width: 10.0, color: Color(0xFFFF000000)),
),
)
)
Example: Use 2 nested Container(s) and Border to simulate a button:
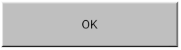
(ex3)
Container(
decoration: BoxDecoration(
border: Border(
top: BorderSide(width: 1.0, color: Color(0xFFFFFFFFFF)),
left: BorderSide(width: 1.0, color: Color(0xFFFFFFFFFF)),
right: BorderSide(width: 1.0, color: Color(0xFFFF000000)),
bottom: BorderSide(width: 1.0, color: Color(0xFFFF000000)),
),
),
child: Container(
width: 200,
height: 50,
padding: EdgeInsets.symmetric(horizontal: 20.0, vertical: 2.0),
alignment: Alignment.center,
decoration: BoxDecoration(
border: Border(
top: BorderSide(width: 1.0, color: Color(0xFFFFDFDFDF)),
left: BorderSide(width: 1.0, color: Color(0xFFFFDFDFDF)),
right: BorderSide(width: 1.0, color: Color(0xFFFF7F7F7F)),
bottom: BorderSide(width: 1.0, color: Color(0xFFFF7F7F7F)),
),
color: Color(0xFFBFBFBF),
),
child: Text(
'OK',
textAlign: TextAlign.center,
style: TextStyle(color: Color(0xFF000000))
),
),
)
2. Border.all constructor
Border.all constructor creates a consistent border (same on all edges) from color, width, and style parameters.
Border.all constructor
Border.all(
{Color color: const Color(0xFF000000),
double width: 1.0,
BorderStyle style: BorderStyle.solid}
)
Example:
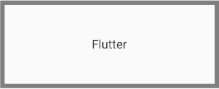
Container(
width: 250,
height: 100,
alignment: Alignment.center,
decoration: BoxDecoration(
border: Border.all(
width: 5.0,
color: Color(0xFFFF7F7F7F)
),
),
child: Text("Flutter")
)
3. Border.fromBorderSide constructor
Border.fromBorderSide constructor creates a consistent border (same for all edges) with parameters given by the BorderSide object.
Border.fromBorderSide constructor
const Border.fromBorderSide(
BorderSide side
)
Example:
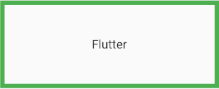
Container(
width: 250,
height: 100,
alignment: Alignment.center,
decoration: BoxDecoration(
border: Border.fromBorderSide(
BorderSide (
width: 5,
color: Colors.green,
style: BorderStyle.solid
)
),
),
child: Text("Flutter")
)
4. Border.symmetric constructor
Border.symmetric constructor creates a vertically and horizontally symmetrical border.
vertical parameter is applied to left and right edges. horizontal parameter is applied to top and bottom edges. The default values for the vertical and horizontal parameters are BorderSide.none and not null.
Border.symmetric constructor
const Border.symmetric(
{BorderSide vertical: BorderSide.none,
BorderSide horizontal: BorderSide.none}
)
Example:
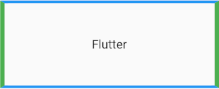
Container(
width: 250,
height: 100,
alignment: Alignment.center,
decoration: BoxDecoration(
border: Border.symmetric (
vertical: BorderSide (
width: 5,
color: Colors.green,
style: BorderStyle.solid
),
horizontal: BorderSide (
width: 3,
color: Colors.blue,
style: BorderStyle.solid
),
),
),
child: Text("Flutter")
)
Flutter Programming Tutorials
- Flutter Column Tutorial with Examples
- Flutter Stack Tutorial with Examples
- Flutter IndexedStack Tutorial with Examples
- Flutter Spacer Tutorial with Examples
- Flutter Expanded Tutorial with Examples
- Flutter SizedBox Tutorial with Examples
- Flutter Tween Tutorial with Examples
- Install Flutter SDK on Windows
- Install Flutter Plugin for Android Studio
- Create your first Flutter app - Hello Flutter
- Flutter Scaffold Tutorial with Examples
- Flutter AppBar Tutorial with Examples
- Flutter BottomAppBar Tutorial with Examples
- Flutter TextButton Tutorial with Examples
- Flutter ElevatedButton Tutorial with Examples
- Flutter EdgeInsetsGeometry Tutorial with Examples
- Flutter EdgeInsets Tutorial with Examples
- Flutter CircularProgressIndicator Tutorial with Examples
- Flutter LinearProgressIndicator Tutorial with Examples
- Flutter Center Tutorial with Examples
- Flutter Align Tutorial with Examples
- Flutter Row Tutorial with Examples
- Flutter SplashScreen Tutorial with Examples
- Flutter Alignment Tutorial with Examples
- Flutter Positioned Tutorial with Examples
- Flutter SimpleDialog Tutorial with Examples
- Flutter AlertDialog Tutorial with Examples
- Flutter Navigation and Routing Tutorial with Examples
- Flutter TabBar Tutorial with Examples
- Flutter Banner Tutorial with Examples
- Flutter BottomNavigationBar Tutorial with Examples
- Flutter FancyBottomNavigation Tutorial with Examples
- Flutter Card Tutorial with Examples
- Flutter Border Tutorial with Examples
- Flutter ContinuousRectangleBorder Tutorial with Examples
- Flutter RoundedRectangleBorder Tutorial with Examples
- Flutter CircleBorder Tutorial with Examples
- Flutter StadiumBorder Tutorial with Examples
- Flutter Container Tutorial with Examples
- Flutter RotatedBox Tutorial with Examples
- Flutter CircleAvatar Tutorial with Examples
- Flutter IconButton Tutorial with Examples
- Flutter FlatButton Tutorial with Examples
- Flutter SnackBar Tutorial with Examples
Show More