Flutter CircleAvatar Tutorial with Examples
1. CircleAvatar
CircleAvatar is simply a circle in which we can add background color, background image, or just some text. It usually represents a user with his image or with his initials. Although we can make a similar widget from the ground up, this widget comes in handy in the fast development of an application.
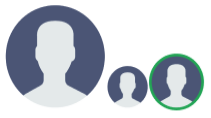
CircleAvatar Constructor
const CircleAvatar(
{Key key,
Widget child,
Color backgroundColor,
ImageProvider<Object> backgroundImage,
void onBackgroundImageError(
dynamic exception,
StackTrace stackTrace
),
Color foregroundColor,
double radius,
double minRadius,
double maxRadius}
)
Let's start with a simple CircleAvatar consisting of a given background image and default background color.
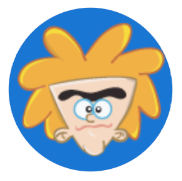
(ex1)
CircleAvatar(
radius: 100,
backgroundImage: NetworkImage("https://s3.o7planning.com/images/boy-128.png"),
)
Basically, CircleAvatar does not provide a property to set borders. However, you can wrap it in a different CircleAvatar with a larger radius and a different background color to create something similar to the border.
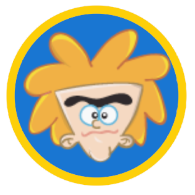
(ex2)
CircleAvatar(
radius: 110,
backgroundColor: Color(0xffFDCF09),
child: CircleAvatar(
radius: 100,
backgroundImage: NetworkImage("https://s3.o7planning.com/images/boy-128.png"),
)
)
Example: A CircleAvatar with user's initials.
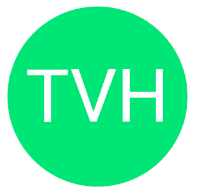
(ex3)
CircleAvatar(
radius: 110,
backgroundColor: Colors.greenAccent[400],
child: Text(
'TVH',
style: TextStyle(
fontSize: 90,
color: Colors.white
),
),
)
3. backgroundColor
backgroundColor - Background color of the CircleAvatar.
The default value of backgroundColor is ThemeData.primaryColorLight if foregroundColor is dark, and is ThemeData.primaryColorDark if foregroundColor is light.
Color backgroundColor
Example:
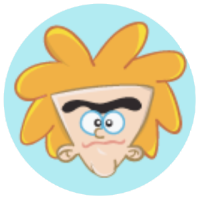
backgroundColor (ex1)
CircleAvatar(
radius: 110,
backgroundImage: NetworkImage("https://s3.o7planning.com/images/boy-128.png"),
backgroundColor: Colors.cyan[100],
)
4. backgroundImage
backgroundImage - Background image of CircleAvatar, which is the user's avatar.
If you want to display user's initials on CircleAvatar, use child property.
ImageProvider<Object> backgroundImage
- Flutter ImageProvider
backgroundImage (ex1)
CircleAvatar(
radius: 110,
backgroundImage: NetworkImage("https://s3.o7planning.com/images/boy-128.png"),
)
5. foregroundColor
foregroundColor - The default color of the text in CircleAvatar.
The default value of foregroundColor is ThemeData.primaryColorLight if backgroundColor is dark, and is ThemeData.primaryColorDark if backgroundColor is light.
Color foregroundColor
Example:
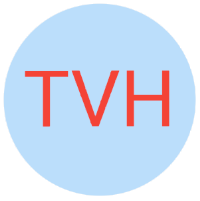
foregroundColor (ex1)
CircleAvatar(
radius: 110,
foregroundColor: Colors.red,
child: Text(
'TVH',
style: TextStyle(
fontSize: 90
),
),
)
6. radius
radius - Radius of the CircleAvatar circle.
If radius is specified then minRadius and maxRadius cannot be specified. Specifying a radius is also equivalent to specifying values for minRadius and maxRadius, they all have the same value.
double radius
7. minRadius
minRadius - Minimum radius of CircleAvatar.
If minRadius is specified then radius will not be specified. Flutter will automatically calculate the appropriate size for the CircleAvatar based on the available space.
double minRadius
8. maxRadius
maxRadius - The maximum radius of the CircleAvatar.
If maxRadius is specified then radius will not be specified. Flutter will automatically calculate the appropriate size for the CircleAvatar based on the available space.
double maxRadius
9. onBackgroundImageError
onBackgroundImageError - an optional callback function - is called when an image-loading error has occurred with backgroundImage.
void onBackgroundImageError(
dynamic exception,
StackTrace stackTrace
)
Example: A CircleAvatar tries to display an user's avatar. If error occurrs while loading the image, it will display an error text.
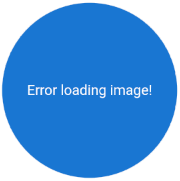
onBackgroundImageError (ex1)
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'o7planning.org',
debugShowCheckedModeBanner: false,
theme: ThemeData(
primarySwatch: Colors.blue,
visualDensity: VisualDensity.adaptivePlatformDensity,
),
home: MyHomePage(),
);
}
}
class MyHomePage extends StatefulWidget {
@override
State<StatefulWidget> createState() {
return _MyHomePageState();
}
}
class _MyHomePageState extends State<MyHomePage> {
// String imageUrl = "https://s3.o7planning.com/images/boy-128.png";
String imageUrl = "https://file-not-found";
bool _loadImageError = false;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text("Flutter CircleAvatar Example")
),
body: Center (
child: CircleAvatar(
radius: 100,
backgroundImage: this._loadImageError? null: NetworkImage(this.imageUrl),
onBackgroundImageError: this._loadImageError? null:
(dynamic exception, StackTrace stackTrace) {
print("Error loading image! " + exception.toString());
this.setState(() {
this._loadImageError = true;
});
},
child: this._loadImageError? Text("Error loading image!") : null
)
)
);
}
}
Flutter Programming Tutorials
- Flutter Column Tutorial with Examples
- Flutter Stack Tutorial with Examples
- Flutter IndexedStack Tutorial with Examples
- Flutter Spacer Tutorial with Examples
- Flutter Expanded Tutorial with Examples
- Flutter SizedBox Tutorial with Examples
- Flutter Tween Tutorial with Examples
- Install Flutter SDK on Windows
- Install Flutter Plugin for Android Studio
- Create your first Flutter app - Hello Flutter
- Flutter Scaffold Tutorial with Examples
- Flutter AppBar Tutorial with Examples
- Flutter BottomAppBar Tutorial with Examples
- Flutter TextButton Tutorial with Examples
- Flutter ElevatedButton Tutorial with Examples
- Flutter EdgeInsetsGeometry Tutorial with Examples
- Flutter EdgeInsets Tutorial with Examples
- Flutter CircularProgressIndicator Tutorial with Examples
- Flutter LinearProgressIndicator Tutorial with Examples
- Flutter Center Tutorial with Examples
- Flutter Align Tutorial with Examples
- Flutter Row Tutorial with Examples
- Flutter SplashScreen Tutorial with Examples
- Flutter Alignment Tutorial with Examples
- Flutter Positioned Tutorial with Examples
- Flutter SimpleDialog Tutorial with Examples
- Flutter AlertDialog Tutorial with Examples
- Flutter Navigation and Routing Tutorial with Examples
- Flutter TabBar Tutorial with Examples
- Flutter Banner Tutorial with Examples
- Flutter BottomNavigationBar Tutorial with Examples
- Flutter FancyBottomNavigation Tutorial with Examples
- Flutter Card Tutorial with Examples
- Flutter Border Tutorial with Examples
- Flutter ContinuousRectangleBorder Tutorial with Examples
- Flutter RoundedRectangleBorder Tutorial with Examples
- Flutter CircleBorder Tutorial with Examples
- Flutter StadiumBorder Tutorial with Examples
- Flutter Container Tutorial with Examples
- Flutter RotatedBox Tutorial with Examples
- Flutter CircleAvatar Tutorial with Examples
- Flutter IconButton Tutorial with Examples
- Flutter FlatButton Tutorial with Examples
- Flutter SnackBar Tutorial with Examples
Show More