Flutter EdgeInsets Tutorial with Examples
1. Flutter EdgeInsets
EdgeInsets helps create the outer padding or the inner padding for a Widget based on the visual parameters, left, top, right, and bottom. It does not depend on the text direction.
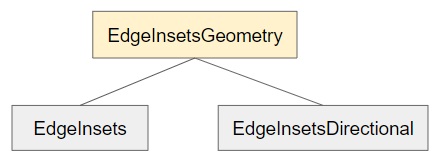
To support both left-to-right and right-to-left layouts, take the EdgeInsetsDirectional into consideration.
- Flutter EdgeInsetsDirectional
2. EdgeInsets.all
EdgeInsets.all constructor is used to create an EdgeInsets object with the same value for all four left, top, right, bottom properties.
const EdgeInsets.all(
double value
)
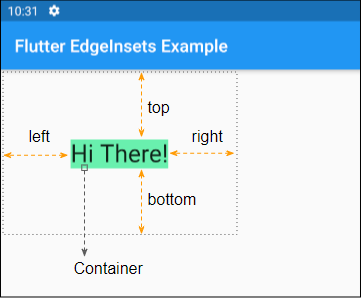
EdgeInsets.all (ex1)
Container (
margin: EdgeInsets.all(80),
color: Colors.greenAccent,
child:Text(
"Hi There!",
style: TextStyle(fontSize: 28)
)
)
3. EdgeInsets.fromLTRB
EdgeInsets.fromLTRB constructor is used to create an EdgeInsets object based on the left, top, right and bottom values.
const EdgeInsets.fromLTRB(
double left,
double top,
double right,
double bottom
)
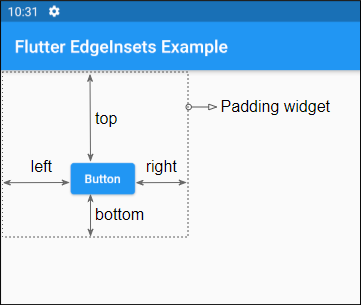
EdgeInsets.fromLTRB (ex1)
Padding (
padding: EdgeInsets.fromLTRB(80, 100, 70, 50),
child: ElevatedButton (
child: Text("Button"),
onPressed: (){}
)
)
4. EdgeInsets.only
EdgeInsets.only constructor creates an EdgeInsets object from the left, top, right and bottom optional parameters. The unspecified parameters will have a value of 0.
const EdgeInsets.only(
{double left: 0.0,
double top: 0.0,
double right: 0.0,
double bottom: 0.0}
)
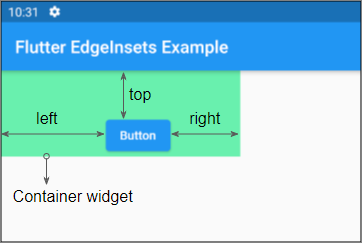
EdgeInsets.only (ex1)
Container (
color: Colors.greenAccent,
padding: EdgeInsets.only(left: 120, top: 50, right: 80),
child: ElevatedButton (
child: Text("Button"),
onPressed: (){}
)
)
5. EdgeInsets.symmetric
EdgeInsets.symmetric constructor creates a symmetric EdgeInsets object from the two horizontal and vertical parameters. It means:
- left = right = horizontal
- top = bottom = vertical
const EdgeInsets.symmetric(
{double vertical: 0.0,
double horizontal: 0.0}
)
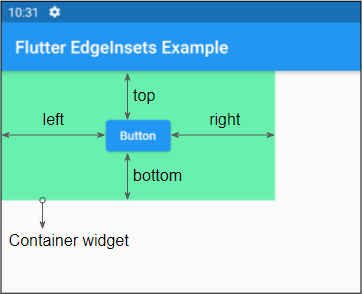
EdgeInsets.symmetric (ex1)
Container (
color: Colors.greenAccent,
padding: EdgeInsets.symmetric(horizontal: 120, vertical: 50),
child: ElevatedButton (
child: Text("Button"),
onPressed: (){}
)
)
Flutter Programming Tutorials
- Flutter Column Tutorial with Examples
- Flutter Stack Tutorial with Examples
- Flutter IndexedStack Tutorial with Examples
- Flutter Spacer Tutorial with Examples
- Flutter Expanded Tutorial with Examples
- Flutter SizedBox Tutorial with Examples
- Flutter Tween Tutorial with Examples
- Install Flutter SDK on Windows
- Install Flutter Plugin for Android Studio
- Create your first Flutter app - Hello Flutter
- Flutter Scaffold Tutorial with Examples
- Flutter AppBar Tutorial with Examples
- Flutter BottomAppBar Tutorial with Examples
- Flutter TextButton Tutorial with Examples
- Flutter ElevatedButton Tutorial with Examples
- Flutter EdgeInsetsGeometry Tutorial with Examples
- Flutter EdgeInsets Tutorial with Examples
- Flutter CircularProgressIndicator Tutorial with Examples
- Flutter LinearProgressIndicator Tutorial with Examples
- Flutter Center Tutorial with Examples
- Flutter Align Tutorial with Examples
- Flutter Row Tutorial with Examples
- Flutter SplashScreen Tutorial with Examples
- Flutter Alignment Tutorial with Examples
- Flutter Positioned Tutorial with Examples
- Flutter SimpleDialog Tutorial with Examples
- Flutter AlertDialog Tutorial with Examples
- Flutter Navigation and Routing Tutorial with Examples
- Flutter TabBar Tutorial with Examples
- Flutter Banner Tutorial with Examples
- Flutter BottomNavigationBar Tutorial with Examples
- Flutter FancyBottomNavigation Tutorial with Examples
- Flutter Card Tutorial with Examples
- Flutter Border Tutorial with Examples
- Flutter ContinuousRectangleBorder Tutorial with Examples
- Flutter RoundedRectangleBorder Tutorial with Examples
- Flutter CircleBorder Tutorial with Examples
- Flutter StadiumBorder Tutorial with Examples
- Flutter Container Tutorial with Examples
- Flutter RotatedBox Tutorial with Examples
- Flutter CircleAvatar Tutorial with Examples
- Flutter IconButton Tutorial with Examples
- Flutter FlatButton Tutorial with Examples
- Flutter SnackBar Tutorial with Examples
Show More