Flutter Banner Tutorial with Examples
1. Flutter Banner
In Flutter, Banner is a diagonal message displays on the surface and at the corner of another Widget. Banner is often used to decorate and highlight a message regarding the Widget.
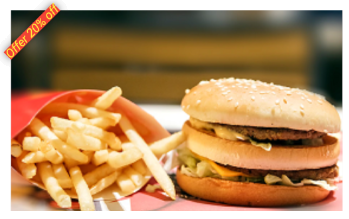
Banner Constructor:
Banner Constructor
const Banner(
{Key key,
Widget child,
@required String message,
TextDirection textDirection,
@required BannerLocation location,
TextDirection layoutDirection,
Color color: _kColor,
TextStyle textStyle: _kTextStyle}
)
2. Banner Example
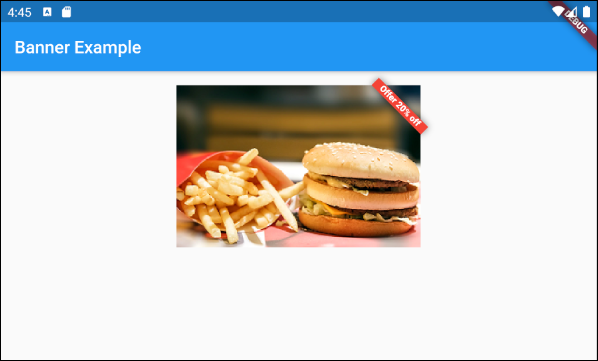
main.dart (ex1)
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'o7planning',
theme: ThemeData(
primarySwatch: Colors.blue,
visualDensity: VisualDensity.adaptivePlatformDensity,
),
home: MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
MyHomePage({Key key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold (
appBar: AppBar(
title: Text("Banner Example"),
),
body: Container(
padding: EdgeInsets.all(16),
child: Align(
alignment: Alignment.topCenter,
child: Banner (
message: 'Offer 20% off',
location: BannerLocation.topEnd,
color: Colors.red,
child: Container(
height: 186,
width: 280,
child: Image.network(
'https://raw.githubusercontent.com/o7planning/rs/master/flutter/fast_food.png',
fit: BoxFit.cover,
),
),
),
),
)
);
}
}
3. child
The child property is used to define the content under the "banner". In most use cases, it is a Widget containing an image.
Widget child
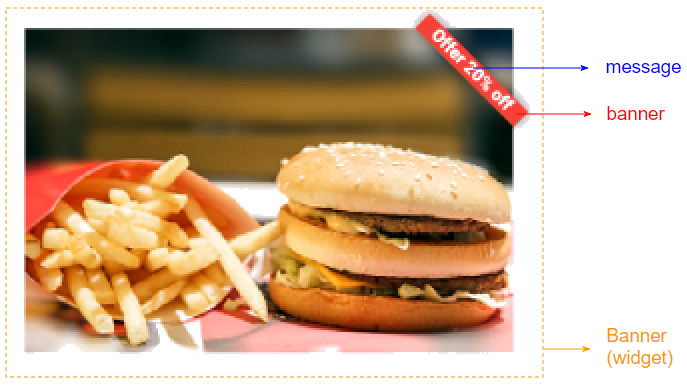
A child can also be a mix of text and images:
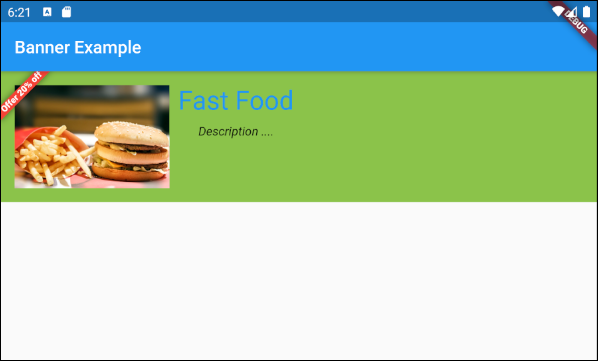
main.dart (child ex2)
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'o7planning',
theme: ThemeData(
primarySwatch: Colors.blue,
visualDensity: VisualDensity.adaptivePlatformDensity,
),
home: MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
MyHomePage({Key key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold (
appBar: AppBar(
title: Text("Banner Example"),
),
body: Banner (
message: 'Offer 20% off',
location: BannerLocation.topStart,
color: Colors.red,
child: Container(
height: 150,
width: double.infinity,
color: Colors.lightGreen,
child: Padding (
padding: EdgeInsets.all(16),
child: Row (
children: [
Image.network (
"https://raw.githubusercontent.com/o7planning/rs/master/flutter/fast_food.png"
),
SizedBox(width: 10),
Column (
children: [
Text("Fast Food",style: TextStyle(fontSize: 30, color: Colors.blue)),
SizedBox(height: 10),
Text("Description ....", style: TextStyle(fontStyle: FontStyle.italic))
],
)
],
),
),
),
)
);
}
}
5. layoutDirection
layoutDirection property is used to specify the direction of the layout. Its default value is TextDirection.ltr (Left to Right).
TextDirection layoutDirection
// Enum values:
TextDirection.ltr // Left to Right (Default)
TextDirection.rtl // Right to Left
The Layout Direction concept helps create applications suitable for different languages and cultures. Specifically, English is written from left to right, while Arabic is written from right to left.
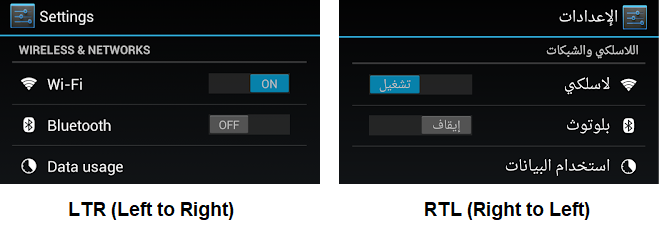
6. location
location property is used to specify the position to display "banner". It can receive one of the four values as follows:
- BannerLocation.topStart
- BannerLocation.topEnd
- BannerLocation.bottomStart
- BannerLocation.bottomEnd
@required BannerLocation location
If the Layout Direction is from Left to Right:
- layoutDirection: TextDirection.ltr (Default)
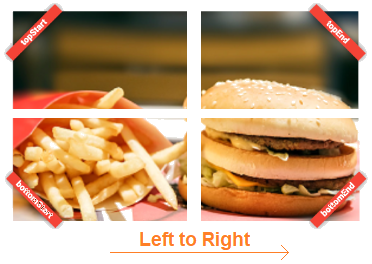
If the Layout Direction is from Right to Left:
- layoutDirection: TextDirection.rtl
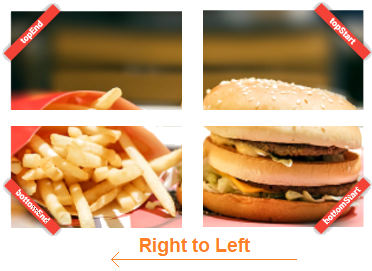
Flutter Programming Tutorials
- Flutter Column Tutorial with Examples
- Flutter Stack Tutorial with Examples
- Flutter IndexedStack Tutorial with Examples
- Flutter Spacer Tutorial with Examples
- Flutter Expanded Tutorial with Examples
- Flutter SizedBox Tutorial with Examples
- Flutter Tween Tutorial with Examples
- Install Flutter SDK on Windows
- Install Flutter Plugin for Android Studio
- Create your first Flutter app - Hello Flutter
- Flutter Scaffold Tutorial with Examples
- Flutter AppBar Tutorial with Examples
- Flutter BottomAppBar Tutorial with Examples
- Flutter TextButton Tutorial with Examples
- Flutter ElevatedButton Tutorial with Examples
- Flutter EdgeInsetsGeometry Tutorial with Examples
- Flutter EdgeInsets Tutorial with Examples
- Flutter CircularProgressIndicator Tutorial with Examples
- Flutter LinearProgressIndicator Tutorial with Examples
- Flutter Center Tutorial with Examples
- Flutter Align Tutorial with Examples
- Flutter Row Tutorial with Examples
- Flutter SplashScreen Tutorial with Examples
- Flutter Alignment Tutorial with Examples
- Flutter Positioned Tutorial with Examples
- Flutter SimpleDialog Tutorial with Examples
- Flutter AlertDialog Tutorial with Examples
- Flutter Navigation and Routing Tutorial with Examples
- Flutter TabBar Tutorial with Examples
- Flutter Banner Tutorial with Examples
- Flutter BottomNavigationBar Tutorial with Examples
- Flutter FancyBottomNavigation Tutorial with Examples
- Flutter Card Tutorial with Examples
- Flutter Border Tutorial with Examples
- Flutter ContinuousRectangleBorder Tutorial with Examples
- Flutter RoundedRectangleBorder Tutorial with Examples
- Flutter CircleBorder Tutorial with Examples
- Flutter StadiumBorder Tutorial with Examples
- Flutter Container Tutorial with Examples
- Flutter RotatedBox Tutorial with Examples
- Flutter CircleAvatar Tutorial with Examples
- Flutter IconButton Tutorial with Examples
- Flutter FlatButton Tutorial with Examples
- Flutter SnackBar Tutorial with Examples
Show More