Flutter Spacer Tutorial with Examples
1. Flutter Spacer
Spacer creates an empty, adjustable space which is used to adjust the spacing between child Widget(s) in a Flex container such as Column, Row, etc.
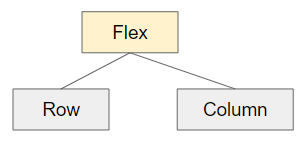
Spacer Constructor
const Spacer(
{Key key,
int flex: 1}
)
For example:
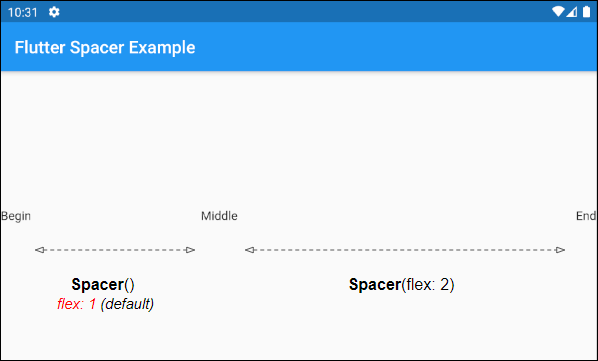
Row (
children: <Widget>[
Text('Begin'),
Spacer(), // Defaults to a flex of one.
Text('Middle'),
// Gives twice the space between Middle and End than Begin and Middle.
Spacer(flex: 2),
Text('End'),
],
)
main.dart (ex1)
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'o7planning.org',
debugShowCheckedModeBanner: false,
theme: ThemeData(
primarySwatch: Colors.blue,
visualDensity: VisualDensity.adaptivePlatformDensity,
),
home: MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
MyHomePage({Key key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold (
appBar: AppBar(
title: Text("Flutter Spacer Example")
),
body: Center (
child: Row(
children: <Widget>[
Text('Begin'),
Spacer(), // Defaults to a flex of one.
Text('Middle'),
// Gives twice the space between Middle and End than Begin and Middle.
Spacer(flex: 2),
Text('End'),
],
)
)
);
}
}
2. flex
flex property is considered as the weight of the Spacer. It determines how much space will be allocated to the Spacer. The allocated space is proportional to the flex value. The default value of flex is 1.
int flex: 1
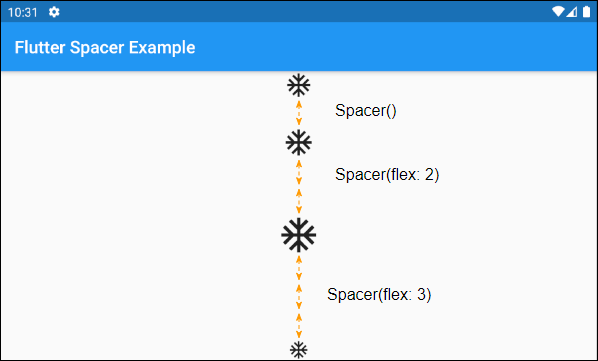
flex (ex1)
Column (
children: <Widget>[
Icon(Icons.ac_unit, size: 32),
Spacer(), // flex : 1 (Default)
Icon(Icons.ac_unit, size: 36),
Spacer(flex: 2),
Icon(Icons.ac_unit, size: 48),
Spacer(flex: 3),
Icon(Icons.ac_unit, size: 24),
],
)
Flutter Programming Tutorials
- Flutter Column Tutorial with Examples
- Flutter Stack Tutorial with Examples
- Flutter IndexedStack Tutorial with Examples
- Flutter Spacer Tutorial with Examples
- Flutter Expanded Tutorial with Examples
- Flutter SizedBox Tutorial with Examples
- Flutter Tween Tutorial with Examples
- Install Flutter SDK on Windows
- Install Flutter Plugin for Android Studio
- Create your first Flutter app - Hello Flutter
- Flutter Scaffold Tutorial with Examples
- Flutter AppBar Tutorial with Examples
- Flutter BottomAppBar Tutorial with Examples
- Flutter TextButton Tutorial with Examples
- Flutter ElevatedButton Tutorial with Examples
- Flutter EdgeInsetsGeometry Tutorial with Examples
- Flutter EdgeInsets Tutorial with Examples
- Flutter CircularProgressIndicator Tutorial with Examples
- Flutter LinearProgressIndicator Tutorial with Examples
- Flutter Center Tutorial with Examples
- Flutter Align Tutorial with Examples
- Flutter Row Tutorial with Examples
- Flutter SplashScreen Tutorial with Examples
- Flutter Alignment Tutorial with Examples
- Flutter Positioned Tutorial with Examples
- Flutter SimpleDialog Tutorial with Examples
- Flutter AlertDialog Tutorial with Examples
- Flutter Navigation and Routing Tutorial with Examples
- Flutter TabBar Tutorial with Examples
- Flutter Banner Tutorial with Examples
- Flutter BottomNavigationBar Tutorial with Examples
- Flutter FancyBottomNavigation Tutorial with Examples
- Flutter Card Tutorial with Examples
- Flutter Border Tutorial with Examples
- Flutter ContinuousRectangleBorder Tutorial with Examples
- Flutter RoundedRectangleBorder Tutorial with Examples
- Flutter CircleBorder Tutorial with Examples
- Flutter StadiumBorder Tutorial with Examples
- Flutter Container Tutorial with Examples
- Flutter RotatedBox Tutorial with Examples
- Flutter CircleAvatar Tutorial with Examples
- Flutter IconButton Tutorial with Examples
- Flutter FlatButton Tutorial with Examples
- Flutter SnackBar Tutorial with Examples
Show More