Flutter FancyBottomNavigation Tutorial with Examples
1. Flutter FancyBottomNavigation
There are plenty of libraries provided by the Flutter community that help you create a navigation bar similar to the BottomNavigationBar, and one of them is FacyBottomNavigation.

FancyBottomNavigation Constructor
FancyBottomNavigation(
{Key key,
@required List<TabData> tabs,
@required Function(int position) onTabChangedListener,
int initialSelection = 0,
Color circleColor,
Color activeIconColor,
Color inactiveIconColor,
Color textColor,
Color barBackgroundColor}
)
FacyBottomNavigation only allows the number of Tab(s) to be greater than 1 and less than 5. That means if you want an application bar with 5 or more than 5 Tab(s), you'd better find another library.
In order to use the FacyBottomNavigation, you need to declare this library with the project. Specifically, open the pubspect.yaml file and add the following content:
pubspect.yaml
dependencies:
...
fancy_bottom_navigation: ^0.3.2
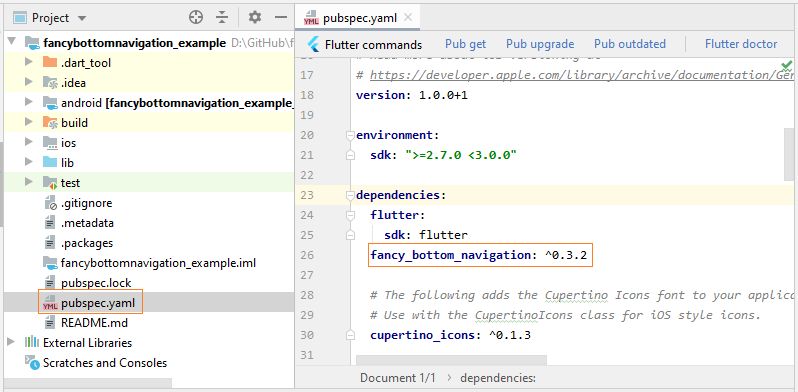
You can visit the home page of the library on GitHub to get more information about the latest version:
2. FancyBottomNavigation Example
Here is a simple example of the FancyBottomNavigation:
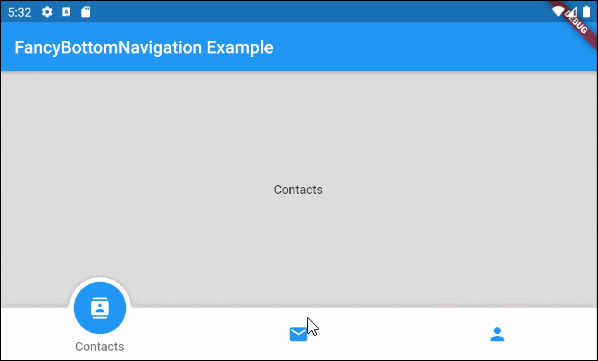
main.dart (ex1)
import 'package:fancy_bottom_navigation/fancy_bottom_navigation.dart';
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Title of Application',
theme: ThemeData(
primarySwatch: Colors.blue,
visualDensity: VisualDensity.adaptivePlatformDensity,
),
home: MyHomePage(),
);
}
}
class MyHomePage extends StatefulWidget {
MyHomePage({Key key}) : super(key: key);
@override
State<StatefulWidget> createState() {
return MyHomePageState();
}
}
class MyHomePageState extends State<MyHomePage> {
int currentPage = 0;
Widget _myContacts = MyContacts();
Widget _myEmails = MyEmails();
Widget _myProfile = MyProfile();
@override
Widget build(BuildContext context) {
FancyBottomNavigation a;
return Scaffold(
appBar: AppBar(
title: Text("FancyBottomNavigation Example"),
),
body: Container(
color: Colors.black12,
child: getPage(),
constraints: BoxConstraints.expand(),
),
bottomNavigationBar: FancyBottomNavigation(
tabs: [
TabData(iconData: Icons.contacts, title: "Contacts"),
TabData(iconData: Icons.mail, title: "Emails"),
TabData(iconData: Icons.person, title: "Profile")
],
onTabChangedListener: (position) {
setState(() {
currentPage = position;
});
},
)
);
}
Widget getPage() {
if(this.currentPage == 0) {
return this._myContacts;
} else if(this.currentPage==1) {
return this._myEmails;
} else {
return this._myProfile;
}
}
}
class MyContacts extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Center(child: Text("Contacts"));
}
}
class MyEmails extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Center(child: Text("Emails"));
}
}
class MyProfile extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Center(child: Text("Profile"));
}
}
Flutter Programming Tutorials
- Flutter Column Tutorial with Examples
- Flutter Stack Tutorial with Examples
- Flutter IndexedStack Tutorial with Examples
- Flutter Spacer Tutorial with Examples
- Flutter Expanded Tutorial with Examples
- Flutter SizedBox Tutorial with Examples
- Flutter Tween Tutorial with Examples
- Install Flutter SDK on Windows
- Install Flutter Plugin for Android Studio
- Create your first Flutter app - Hello Flutter
- Flutter Scaffold Tutorial with Examples
- Flutter AppBar Tutorial with Examples
- Flutter BottomAppBar Tutorial with Examples
- Flutter TextButton Tutorial with Examples
- Flutter ElevatedButton Tutorial with Examples
- Flutter EdgeInsetsGeometry Tutorial with Examples
- Flutter EdgeInsets Tutorial with Examples
- Flutter CircularProgressIndicator Tutorial with Examples
- Flutter LinearProgressIndicator Tutorial with Examples
- Flutter Center Tutorial with Examples
- Flutter Align Tutorial with Examples
- Flutter Row Tutorial with Examples
- Flutter SplashScreen Tutorial with Examples
- Flutter Alignment Tutorial with Examples
- Flutter Positioned Tutorial with Examples
- Flutter SimpleDialog Tutorial with Examples
- Flutter AlertDialog Tutorial with Examples
- Flutter Navigation and Routing Tutorial with Examples
- Flutter TabBar Tutorial with Examples
- Flutter Banner Tutorial with Examples
- Flutter BottomNavigationBar Tutorial with Examples
- Flutter FancyBottomNavigation Tutorial with Examples
- Flutter Card Tutorial with Examples
- Flutter Border Tutorial with Examples
- Flutter ContinuousRectangleBorder Tutorial with Examples
- Flutter RoundedRectangleBorder Tutorial with Examples
- Flutter CircleBorder Tutorial with Examples
- Flutter StadiumBorder Tutorial with Examples
- Flutter Container Tutorial with Examples
- Flutter RotatedBox Tutorial with Examples
- Flutter CircleAvatar Tutorial with Examples
- Flutter IconButton Tutorial with Examples
- Flutter FlatButton Tutorial with Examples
- Flutter SnackBar Tutorial with Examples
Show More