Flutter ContinuousRectangleBorder Tutorial with Examples
1. ContinuousRectangleBorder
ContinuousRectangleBorder creates a rectangular border with smooth continuous transitions between the straight sides and the rounded corners.
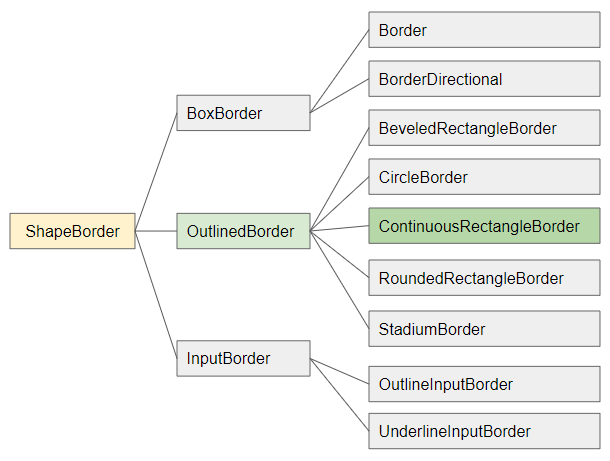
- ShapeBorder
- OutlinedBorder
- RoundedRectangleBorder
- StadiumBorder
- CircleBorder
- Border
- BoxBorder
- InputBorder
- BorderDirectional
- BeveledRectangleBorder
- StadiumBorder
- OutlineInputBorder
- UnderlineInputBorder
ContinuousRectangleBorder constructor
const ContinuousRectangleBorder(
{BorderSide side: BorderSide.none,
BorderRadiusGeometry borderRadius: BorderRadius.zero}
)
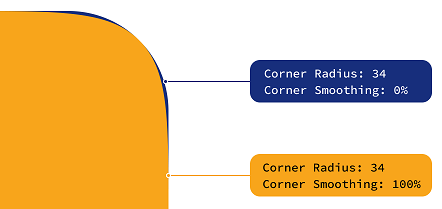
Basically, ContinuousRectangleBorder's usage is the same as RoundedRectangleBorder. They all create a rectangular border with rounded corners. However, the rounded corners created by ContinuousRectangleBorder are smoother.
2. Examples
For example: Use ContinuousRectangleBorder for a Container:
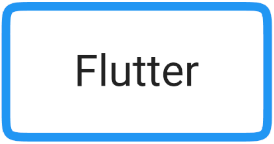
(ex1)
Container(
width: 300,
height: 150,
decoration: ShapeDecoration(
color: Colors.white,
shape: ContinuousRectangleBorder (
borderRadius: BorderRadius.circular(32.0),
side: BorderSide(
width: 10,
color: Colors.blue
)
)
),
child: Center(
child: Text(
"Flutter",
style: TextStyle(fontSize: 50)
)
),
)
Use addition operator (+) to add 2 ShapeBorder in order to create an associative border:
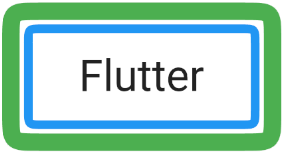
(ex2)
Container(
width: 300,
height: 150,
decoration: ShapeDecoration(
color: Colors.white,
shape: ContinuousRectangleBorder (
borderRadius: BorderRadius.circular(16.0),
side: BorderSide(
width: 10,
color: Colors.blue
)
) + ContinuousRectangleBorder (
borderRadius: BorderRadius.circular(32.0),
side: BorderSide(
width: 20,
color: Colors.green
)
)
),
child: Center(
child: Text(
"Flutter",
style: TextStyle(fontSize: 50)
)
),
)
3. side
side - Provides parameters related to border such as color, width, style.
BorderSide side: BorderSide.none
BorderSide constructor
const BorderSide (
{Color color: const Color(0xFF000000),
double width: 1.0,
BorderStyle style: BorderStyle.solid}
)
- Flutter BorderSide
Note: ContinuousRectangleBorder.side property does not work with ElevatedButton, TextButton and OutlinedButton. It is overridden by ButtonStyle.side.
4. borderRadius
borderRadius - Provides 4 corner radius value of the rectangle.
BorderRadiusGeometry borderRadius: BorderRadius.zero
- Flutter BorderRadiusGeometry
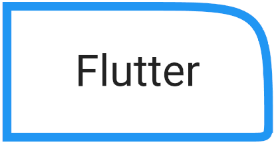
Container(
width: 300,
height: 150,
decoration: ShapeDecoration(
color: Colors.white,
shape: ContinuousRectangleBorder (
borderRadius: BorderRadius.only(
bottomLeft: Radius.zero,
topLeft: Radius.zero,
bottomRight: Radius.circular(20),
topRight: Radius.circular(45)
),
side: BorderSide(
width: 10,
color: Colors.blue
)
)
),
child: Center(
child: Text(
"Flutter",
style: TextStyle(fontSize: 50)
)
),
)
Flutter Programming Tutorials
- Flutter Column Tutorial with Examples
- Flutter Stack Tutorial with Examples
- Flutter IndexedStack Tutorial with Examples
- Flutter Spacer Tutorial with Examples
- Flutter Expanded Tutorial with Examples
- Flutter SizedBox Tutorial with Examples
- Flutter Tween Tutorial with Examples
- Install Flutter SDK on Windows
- Install Flutter Plugin for Android Studio
- Create your first Flutter app - Hello Flutter
- Flutter Scaffold Tutorial with Examples
- Flutter AppBar Tutorial with Examples
- Flutter BottomAppBar Tutorial with Examples
- Flutter TextButton Tutorial with Examples
- Flutter ElevatedButton Tutorial with Examples
- Flutter EdgeInsetsGeometry Tutorial with Examples
- Flutter EdgeInsets Tutorial with Examples
- Flutter CircularProgressIndicator Tutorial with Examples
- Flutter LinearProgressIndicator Tutorial with Examples
- Flutter Center Tutorial with Examples
- Flutter Align Tutorial with Examples
- Flutter Row Tutorial with Examples
- Flutter SplashScreen Tutorial with Examples
- Flutter Alignment Tutorial with Examples
- Flutter Positioned Tutorial with Examples
- Flutter SimpleDialog Tutorial with Examples
- Flutter AlertDialog Tutorial with Examples
- Flutter Navigation and Routing Tutorial with Examples
- Flutter TabBar Tutorial with Examples
- Flutter Banner Tutorial with Examples
- Flutter BottomNavigationBar Tutorial with Examples
- Flutter FancyBottomNavigation Tutorial with Examples
- Flutter Card Tutorial with Examples
- Flutter Border Tutorial with Examples
- Flutter ContinuousRectangleBorder Tutorial with Examples
- Flutter RoundedRectangleBorder Tutorial with Examples
- Flutter CircleBorder Tutorial with Examples
- Flutter StadiumBorder Tutorial with Examples
- Flutter Container Tutorial with Examples
- Flutter RotatedBox Tutorial with Examples
- Flutter CircleAvatar Tutorial with Examples
- Flutter IconButton Tutorial with Examples
- Flutter FlatButton Tutorial with Examples
- Flutter SnackBar Tutorial with Examples
Show More