Flutter TextButton Tutorial with Examples
1. TextButton
In Flutter, TextButton is used to create a button containing a text with the idea of creating a flat button and an elevation of 0 by default. But in fact, you can customize its style by using style property.
Note: Formerly, to create a flat button, we used the FlatButton class. However, since October 2020, this class has been marked as outdated, and it has been replaced by the TextButton class. This is one of the Flutter development team efforts to simplify and make the Flutter API consistent.
TextButton Constructor:
TextButton Constructor
const TextButton({Key key,
@required Widget child,
@required VoidCallback onPressed,
VoidCallback onLongPress,
ButtonStyle style,
FocusNode focusNode,
bool autofocus: false,
Clip clipBehavior: Clip.none
}
)
TextButton can be used in toolbars, Dialogs, etc. But sometimes you need to customize its style to avoid confusion for the user. Or when you use it as a flat button, you should place it in a appropriate position with the context and not put the TextButton in which it is mixed with other contents, such as in the middle of the list.
TextButton.icon Constructor:
TextButton.icon Constructor
TextButton.icon(
{Key key,
@required Widget icon,
@required Widget label,
@required VoidCallback onPressed,
VoidCallback onLongPress,
ButtonStyle style,
FocusNode focusNode,
bool autofocus,
Clip clipBehavior}
)
If both the callback functions, onPressed and onLongPress are not specified, the TextButton will be disabled and have no response when touched.
2. Examples
Below is an example including two TextButton(s). One is the simplest TextButton (containing only one text label), and the other is the TextButton with the backgroundColor and foregroundColor.
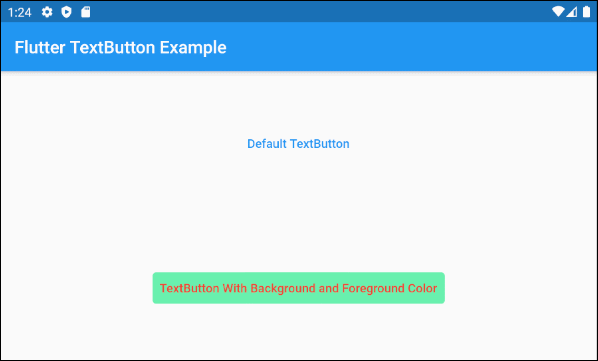
main.dart (ex1)
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'o7planning',
debugShowCheckedModeBanner: false,
theme: ThemeData(
primarySwatch: Colors.blue,
visualDensity: VisualDensity.adaptivePlatformDensity,
),
home: MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
MyHomePage({Key key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text("Flutter TextButton Example")
),
body: Center (
child: Column (
mainAxisAlignment: MainAxisAlignment.spaceAround,
children: [
TextButton (
child: Text("Default TextButton"),
onPressed: () {},
),
TextButton (
child: Text("TextButton With Background and Foreground Color"),
onPressed: () {},
style: ButtonStyle(
backgroundColor: MaterialStateProperty.all<Color>(Colors.greenAccent),
foregroundColor: MaterialStateProperty.all<Color>(Colors.red),
)
)
],
)
)
);
}
}
The most handy way to have a TextButton containing an icon is to use the TextButton.icon constructor.
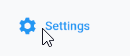
TextButton.icon (
icon: Icon(Icons.settings),
label: Text("Settings"),
onPressed: () {},
)
3. child
child is the most important property of the TextButton. In most use cases, it is a Text object.
@required Widget child
An easy childexample is a Text:
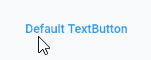
TextButton (
child: Text("Default TextButton"),
onPressed: () {},
)
By assigning Row object to the child property, you can create a more complex TextButton, such as both Icon and Text included.

child (ex1)
// 1 Icon and 1 Text
TextButton (
child: Row (
children: [
Icon(Icons.settings),
SizedBox(width: 5),
Text("Settings")
],
) ,
onPressed: () {},
)
// 2 Icons and 1 Text
TextButton (
child: Row (
children: [
Icon(Icons.directions_bus),
Icon(Icons.train),
SizedBox(width: 5),
Text("Transportation")
],
) ,
onPressed: () {},
)
4. onPressed
onPressed is a callback function. It is called when the user clicks on the Button. Specifically, the onPressed event will happen when the user completes two manipulations including pressing down and releasing the Button.
@required VoidCallback onPressed
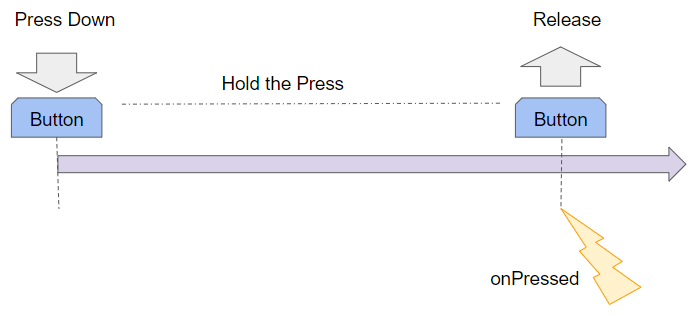
Note: If both onPressed and onLongPress propertiesare not specified, the Button will be disabled and have no response when touched.
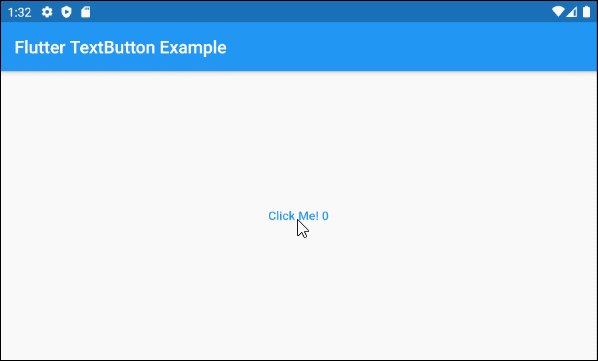
main.dart (onPressed - ex1)
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'o7planning.org',
debugShowCheckedModeBanner: false,
theme: ThemeData(
primarySwatch: Colors.blue,
visualDensity: VisualDensity.adaptivePlatformDensity,
),
home: MyHomePage(),
);
}
}
class MyHomePage extends StatefulWidget {
MyHomePage({Key key}) : super(key: key);
@override
State<StatefulWidget> createState() {
return MyHomePageState();
}
}
class MyHomePageState extends State<MyHomePage> {
int pressCount = 0;
@override
Widget build(BuildContext context) {
return Scaffold (
appBar: AppBar(
title: Text("Flutter TextButton Example"),
),
body: Center (
child: TextButton (
child: Text("Click Me! " + this.pressCount.toString()),
onPressed: onPressHander
),
)
);
}
onPressHander() {
this.setState(() {
this.pressCount++;
});
}
}
5. onLongPress
onLongPress is a callback function. It is called when the user presses down the Button for a longer time than LONG_PRESS_TIMEOUT milliseconds. The Long-Press event will occur at the LONG_PRESS_TIMEOUT th milliseconds when the user presses it down and during this time, (0 -> LONG_PRESS_TIMEOUT), the user does not move the cursor.
VoidCallback onLongPress
If you assign two callback functions, onPressed and onLongPress for a Button, in any situation, there is only at most one function to be called.
LONG_PRESS_TIMEOUT | 500 milliseconds |
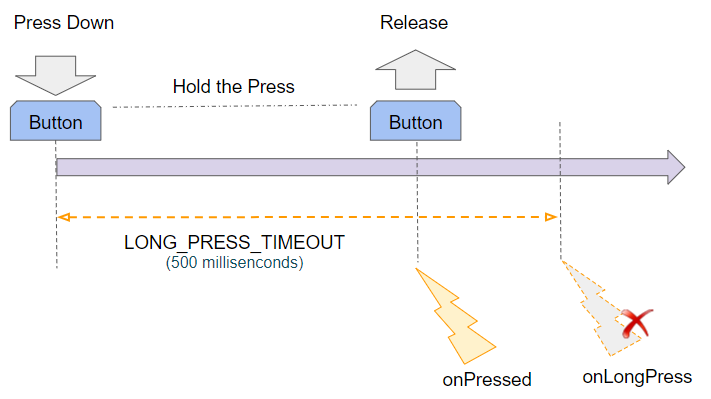
If the user presses down and releases it before the LONG_PRESS_TIMEOUT time, only the onPressed event will occur.
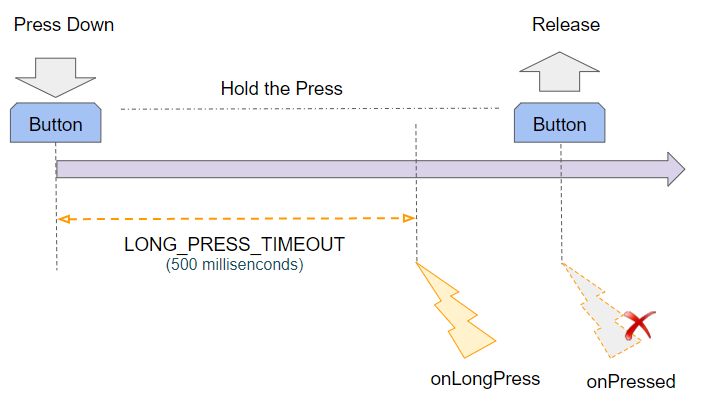
If the user presses it down for a longer time than LONG_PRESS_TIMEOUT milliseconds, the onLongPress event will happen and Flutter will ignore the onPressed event which takes place after that.
For example:
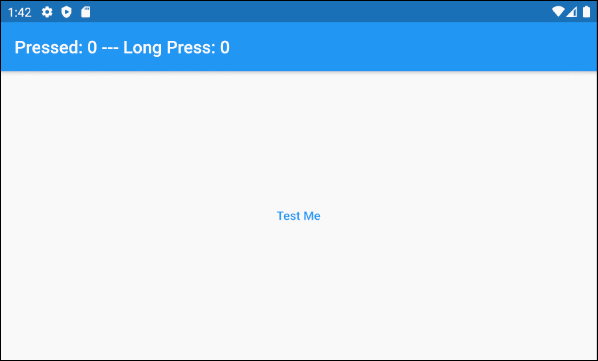
main.dart (onLongPress - ex1)
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'o7planning.org',
debugShowCheckedModeBanner: false,
theme: ThemeData(
primarySwatch: Colors.blue,
visualDensity: VisualDensity.adaptivePlatformDensity,
),
home: MyHomePage(),
);
}
}
class MyHomePage extends StatefulWidget {
MyHomePage({Key key}) : super(key: key);
@override
State<StatefulWidget> createState() {
return MyHomePageState();
}
}
class MyHomePageState extends State<MyHomePage> {
int pressedCount = 0;
int longPressCount = 0;
@override
Widget build(BuildContext context) {
return Scaffold (
appBar: AppBar(
title: Text("Pressed: " + this.pressedCount.toString()
+" --- Long Press: " + this.longPressCount.toString()),
),
body: Center (
child: TextButton (
child: Text("Test Me"),
onPressed: onPressHander,
onLongPress: onLongPressHandler
),
)
);
}
onPressHander() {
this.setState(() {
this.pressedCount++;
});
}
onLongPressHandler() {
this.setState(() {
this.longPressCount++;
});
}
}
6. style
style property is used to customize the style of TextButton.
ButtonStyle style
ButtonStyle constructor
const ButtonStyle(
{MaterialStateProperty<TextStyle> textStyle,
MaterialStateProperty<Color> backgroundColor,
MaterialStateProperty<Color> foregroundColor,
MaterialStateProperty<Color> overlayColor,
MaterialStateProperty<Color> shadowColor,
MaterialStateProperty<double> elevation,
MaterialStateProperty<EdgeInsetsGeometry> padding,
MaterialStateProperty<Size> minimumSize,
MaterialStateProperty<BorderSide> side,
MaterialStateProperty<OutlinedBorder> shape,
MaterialStateProperty<MouseCursor> mouseCursor,
VisualDensity visualDensity,
MaterialTapTargetSize tapTargetSize,
Duration animationDuration,
bool enableFeedback}
)
For example, a TextButton with the changeable background color and foreground color based on its state.
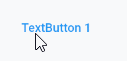
TextButton (
child: Text("TextButton 1"),
onPressed: () {},
style: ButtonStyle (
backgroundColor: MaterialStateProperty.resolveWith<Color>(
(Set<MaterialState> states) {
if (states.contains(MaterialState.pressed)) {
return Colors.red;
}
return null; // Use the component's default.
}
),
foregroundColor: MaterialStateProperty.resolveWith<Color>(
(Set<MaterialState> states) {
if (states.contains(MaterialState.pressed)) {
return Colors.yellow;
}
return null; // Use the component's default.
},
)
)
)
For example: A TextButton with an elevation of 10 in a pressed state will have the elevation of 0.
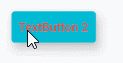
TextButton (
child: Text("TextButton 2"),
onPressed: () {},
style: ButtonStyle(
backgroundColor: MaterialStateProperty.resolveWith<Color>(
(Set<MaterialState> states) {
if (states.contains(MaterialState.disabled)) {
return Colors.black26;
}
return Colors.cyan;
}
),
foregroundColor: MaterialStateProperty.all<Color>(Colors.red),
elevation: MaterialStateProperty.resolveWith<double>(
(Set<MaterialState> states) {
if (states.contains(MaterialState.pressed)
|| states.contains(MaterialState.disabled)) {
return 0;
}
return 10;
},
)
)
)
- ButtonStyle
- MaterialStateProperty
Flutter Programming Tutorials
- Flutter Column Tutorial with Examples
- Flutter Stack Tutorial with Examples
- Flutter IndexedStack Tutorial with Examples
- Flutter Spacer Tutorial with Examples
- Flutter Expanded Tutorial with Examples
- Flutter SizedBox Tutorial with Examples
- Flutter Tween Tutorial with Examples
- Install Flutter SDK on Windows
- Install Flutter Plugin for Android Studio
- Create your first Flutter app - Hello Flutter
- Flutter Scaffold Tutorial with Examples
- Flutter AppBar Tutorial with Examples
- Flutter BottomAppBar Tutorial with Examples
- Flutter TextButton Tutorial with Examples
- Flutter ElevatedButton Tutorial with Examples
- Flutter EdgeInsetsGeometry Tutorial with Examples
- Flutter EdgeInsets Tutorial with Examples
- Flutter CircularProgressIndicator Tutorial with Examples
- Flutter LinearProgressIndicator Tutorial with Examples
- Flutter Center Tutorial with Examples
- Flutter Align Tutorial with Examples
- Flutter Row Tutorial with Examples
- Flutter SplashScreen Tutorial with Examples
- Flutter Alignment Tutorial with Examples
- Flutter Positioned Tutorial with Examples
- Flutter SimpleDialog Tutorial with Examples
- Flutter AlertDialog Tutorial with Examples
- Flutter Navigation and Routing Tutorial with Examples
- Flutter TabBar Tutorial with Examples
- Flutter Banner Tutorial with Examples
- Flutter BottomNavigationBar Tutorial with Examples
- Flutter FancyBottomNavigation Tutorial with Examples
- Flutter Card Tutorial with Examples
- Flutter Border Tutorial with Examples
- Flutter ContinuousRectangleBorder Tutorial with Examples
- Flutter RoundedRectangleBorder Tutorial with Examples
- Flutter CircleBorder Tutorial with Examples
- Flutter StadiumBorder Tutorial with Examples
- Flutter Container Tutorial with Examples
- Flutter RotatedBox Tutorial with Examples
- Flutter CircleAvatar Tutorial with Examples
- Flutter IconButton Tutorial with Examples
- Flutter FlatButton Tutorial with Examples
- Flutter SnackBar Tutorial with Examples
Show More