JavaFX TextInputDialog Tutorial with Examples
1. JavaFX TextInputDialog
TextInputDialog is a subclass of the Dialog class. It is used to display and wait for entering a text content by users.
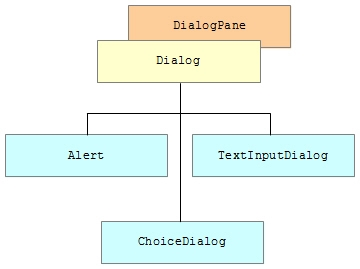
This is image of a standard TextInputDialog:
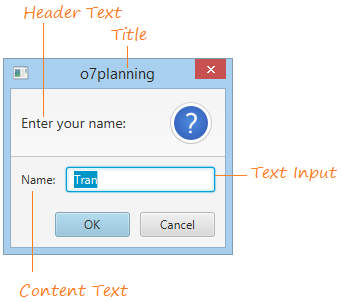
If you don't set Header Text, Title, Content Text, and Text Input, the following image is a default TextInputDialog:
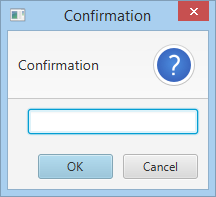
** Code **
TextInputDialog dialog = new TextInputDialog("Tran");
dialog.setTitle("o7planning");
dialog.setHeaderText("Enter your name:");
dialog.setContentText("Name:");
Optional<String> result = dialog.showAndWait();
result.ifPresent(name -> {
this.label.setText(name);
});
Use the following code snippet, if you don't want to display Header region:
dialog.setHeaderText(null)
2. TextInputDialog example
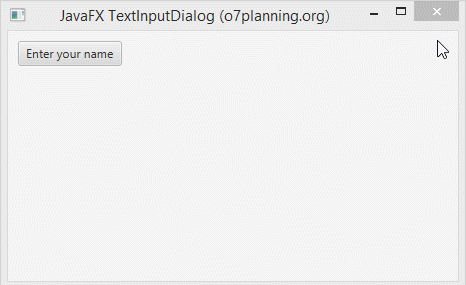
TextInputDialogExample.java
package org.o7planning.javafx.textinputdialog;
import java.util.Optional;
import javafx.application.Application;
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
import javafx.geometry.Insets;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.Label;
import javafx.scene.control.TextInputDialog;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class TextInputDialogExample extends Application {
private Label label;
private void showInputTextDialog() {
TextInputDialog dialog = new TextInputDialog("Tran");
dialog.setTitle("o7planning");
dialog.setHeaderText("Enter your name:");
dialog.setContentText("Name:");
Optional<String> result = dialog.showAndWait();
result.ifPresent(name -> {
this.label.setText(name);
});
}
@Override
public void start(Stage stage) {
VBox root = new VBox();
root.setPadding(new Insets(10));
root.setSpacing(10);
this.label = new Label();
Button button = new Button("Enter your name");
button.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
showInputTextDialog();
}
});
root.getChildren().addAll(button, label);
Scene scene = new Scene(root, 450, 250);
stage.setTitle("JavaFX TextInputDialog (o7planning.org)");
stage.setScene(scene);
stage.show();
}
public static void main(String args[]) {
launch(args);
}
}
JavaFX Tutorials
- Open a new Window in JavaFX
- JavaFX ChoiceDialog Tutorial with Examples
- JavaFX Alert Dialogs Tutorial with Examples
- JavaFX TextInputDialog Tutorial with Examples
- Install e(fx)clipse for Eclipse (JavaFX Tooling)
- Install JavaFX Scene Builder for Eclipse
- JavaFX Tutorial for Beginners - Hello JavaFX
- JavaFX FlowPane Layout Tutorial with Examples
- JavaFX TilePane Layout Tutorial with Examples
- JavaFX HBox, VBox Layout Tutorial with Examples
- JavaFX BorderPane Layout Tutorial with Examples
- JavaFX AnchorPane Layout Tutorial with Examples
- JavaFX TitledPane Tutorial with Examples
- JavaFX Accordion Tutorial with Examples
- JavaFX ListView Tutorial with Examples
- JavaFX Group Tutorial with Examples
- JavaFX ComboBox Tutorial with Examples
- JavaFX Transformations Tutorial with Examples
- JavaFX Effects Tutorial with Examples
- JavaFX GridPane Layout Tutorial with Examples
- JavaFX StackPane Layout Tutorial with Examples
- JavaFX ScrollPane Tutorial with Examples
- JavaFX WebView and WebEngine Tutorial with Examples
- JavaFX HTMLEditor Tutorial with Examples
- JavaFX TableView Tutorial with Examples
- JavaFX TreeView Tutorial with Examples
- JavaFX TreeTableView Tutorial with Examples
- JavaFX Menu Tutorial with Examples
- JavaFX ContextMenu Tutorial with Examples
- JavaFX Image and ImageView Tutorial with Examples
- JavaFX Label Tutorial with Examples
- JavaFX Hyperlink Tutorial with Examples
- JavaFX Button Tutorial with Examples
- JavaFX ToggleButton Tutorial with Examples
- JavaFX RadioButton Tutorial with Examples
- JavaFX MenuButton and SplitMenuButton Tutorial with Examples
- JavaFX TextField Tutorial with Examples
- JavaFX PasswordField Tutorial with Examples
- JavaFX TextArea Tutorial with Examples
- JavaFX Slider Tutorial with Examples
- JavaFX Spinner Tutorial with Examples
- JavaFX ProgressBar and ProgressIndicator Tutorial with Examples
- JavaFX ChoiceBox Tutorial with Examples
- JavaFX Tooltip Tutorial with Examples
- JavaFX DatePicker Tutorial with Examples
- JavaFX ColorPicker Tutorial with Examples
- JavaFX FileChooser and DirectoryChooser Tutorial with Examples
- JavaFX PieChart Tutorial with Examples
- JavaFX AreaChart and StackedAreaChart Tutorial with Examples
- JavaFX BarChart and StackedBarChart Tutorial with Examples
- JavaFX Line Tutorial with Examples
- JavaFX Rectangle and Ellipse Tutorial with Examples
Show More