JavaFX Transformations Tutorial with Examples
1. What is Transformation?
Transformation Is a geometrical concept. It means changing the shape of an object to a different shape. There are some common transformations such as:
- Translation
- Scale
- Rotation
- Shearing
- ....
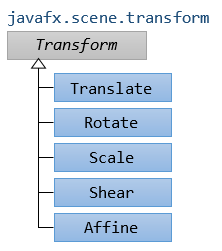
Translation
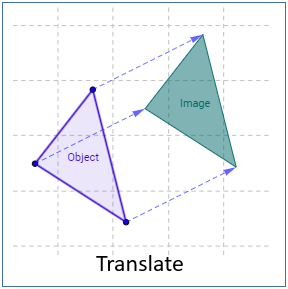
Reflection
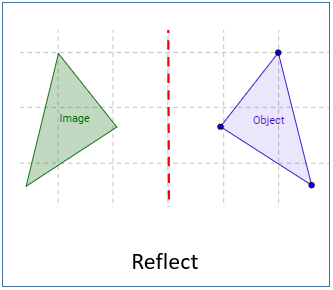
Rotation
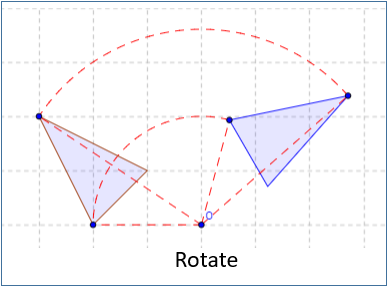
Shearing/Skewing
Shear by x axis:
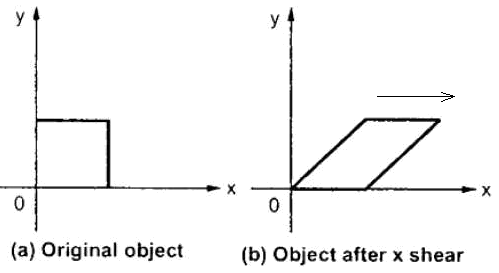
Shear by y axis
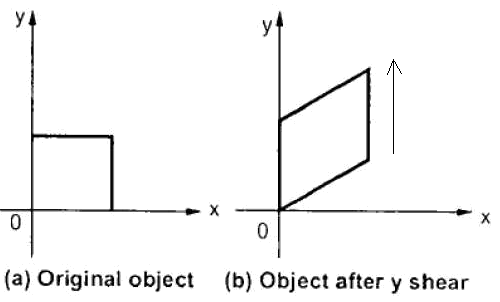
2. Translation Transformation
Translation transformation will move an object to another location in a particular direction. The simplest example is moving one point to another location.
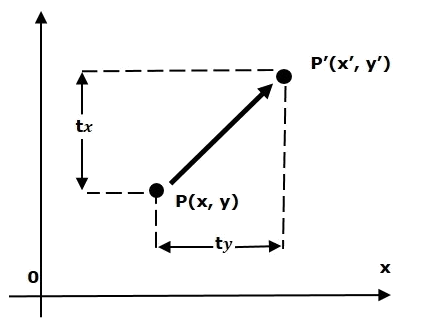
All points on the geometry object move in one direction and move the same distance:
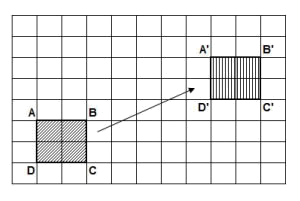
Example:
Example, there are two rectangles in the same position and size, we will use the Translation Transformation to move the second rectangle. And see the result:
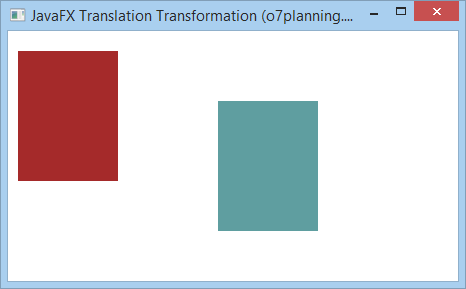
TranslationExample.java
package org.o7planning.javafx.transformation;
import javafx.application.Application;
import javafx.scene.Group;
import javafx.scene.Scene;
import javafx.scene.paint.Color;
import javafx.scene.shape.Rectangle;
import javafx.scene.transform.Translate;
import javafx.stage.Stage;
public class TranslationExample extends Application {
@Override
public void start(Stage stage) {
final int x = 10;
final int y = 20;
final int width = 100;
final int height = 130;
// Rectangle1
Rectangle rectangle1 = new Rectangle(x, y, width, height);
// Rectangle2 (Same position and size to rectangle1)
Rectangle rectangle2 = new Rectangle(x, y, width, height);
rectangle1.setFill(Color.BROWN);
rectangle1.setStrokeWidth(20);
rectangle2.setFill(Color.CADETBLUE);
rectangle2.setStrokeWidth(20);
// Creating the translation transformation
Translate translate = new Translate();
// Set arguments for translation
translate.setX(200);
translate.setY(50);
translate.setZ(100);
// Adding transformation to rectangle2
rectangle2.getTransforms().addAll(translate);
Group root = new Group(rectangle1, rectangle2);
Scene scene = new Scene(root, 450, 250);
stage.setTitle("JavaFX Translation Transformation (o7planning.org)");
stage.setScene(scene);
stage.show();
}
public static void main(String args[]) {
launch(args);
}
}
3. Rotation Transformation
Rotation Transformation rotates an geometric object one angle around a fixed point. This fixed point is called pivot point.
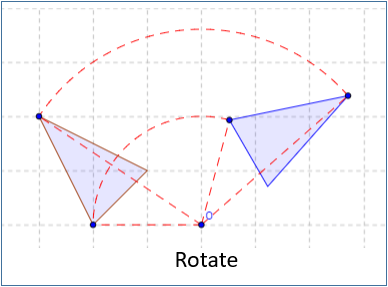
Example:
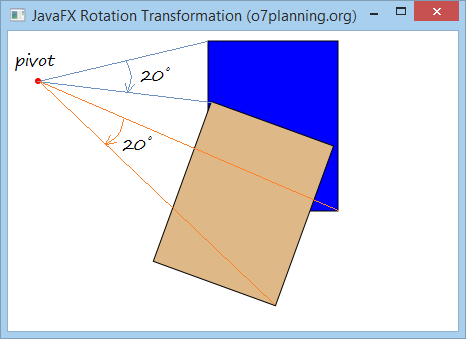
RotationExample.java
package org.o7planning.javafx.transformation;
import javafx.application.Application;
import javafx.scene.Group;
import javafx.scene.Scene;
import javafx.scene.paint.Color;
import javafx.scene.shape.Circle;
import javafx.scene.shape.Rectangle;
import javafx.scene.transform.Rotate;
import javafx.stage.Stage;
public class RotationExample extends Application {
@Override
public void start(Stage stage) {
final int x = 200;
final int y = 10;
final int width = 130;
final int height = 170;
int pivotX = 30;
int pivotY = 50;
// Rectangle1
Rectangle rectangle1 = new Rectangle(x, y, width, height);
rectangle1.setFill(Color.BLUE);
rectangle1.setStroke(Color.BLACK);
// Rectangle2
Rectangle rectangle2 = new Rectangle(x, y, width, height);
rectangle2.setFill(Color.BURLYWOOD);
rectangle2.setStroke(Color.BLACK);
Circle pivot = new Circle(pivotX, pivotY, 3);
pivot.setFill(Color.RED);
// Creating the rotation transformation
Rotate rotate = new Rotate();
// Setting the angle for the rotation (20 degrees)
rotate.setAngle(20);
// Setting pivot points for the rotation
rotate.setPivotX(pivotX);
rotate.setPivotY(pivotY);
// Adding the transformation to rectangle2
rectangle2.getTransforms().addAll(rotate);
Group root = new Group(rectangle1, rectangle2, pivot);
Scene scene = new Scene(root, 450, 300);
stage.setTitle("JavaFX Rotation Transformation (o7planning.org)");
stage.setScene(scene);
stage.show();
}
public static void main(String args[]) {
launch(args);
}
}
4. Scaling Transformation
Scaling Transformation is used to change the size of a geometric object. You can use it to scale the dimensions of the object.
A point will be taken as the origin of the new coordinate system. Scaling can be achieved by multiplying the original coordinates of the object with the scaling factor to get the desired result. See more illustration below.
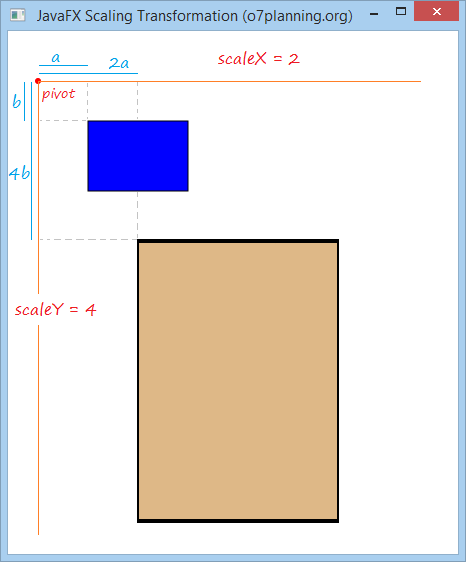
For example:
There are two rectangles at the same location and in the same size. Select a point to act as origin of new coordinate system and use Scaling Transformation to scale coordinates of the object by X axis 2 times and by Y axis 4 times.
ScalingExample.java
package org.o7planning.javafx.transformation;
import javafx.application.Application;
import javafx.scene.Group;
import javafx.scene.Scene;
import javafx.scene.paint.Color;
import javafx.scene.shape.Circle;
import javafx.scene.shape.Rectangle;
import javafx.scene.transform.Scale;
import javafx.stage.Stage;
public class ScalingExample extends Application {
@Override
public void start(Stage stage) {
final int x = 80;
final int y = 90;
final int width = 100;
final int height = 70;
int pivotX = 30;
int pivotY = 50;
double scaleX = 2;
double scaleY = 4;
// Rectangle1
Rectangle rectangle1 = new Rectangle(x, y, width, height);
rectangle1.setFill(Color.BLUE);
rectangle1.setStroke(Color.BLACK);
// Rectangle2
Rectangle rectangle2 = new Rectangle(x, y, width, height);
rectangle2.setFill(Color.BURLYWOOD);
rectangle2.setStroke(Color.BLACK);
Circle pivot = new Circle(pivotX, pivotY, 3);
pivot.setFill(Color.RED);
// Creating the Scale transformation
Scale scale = new Scale();
// Setting the scaliing factor.
scale.setX(scaleX);
scale.setY(scaleY);
// Setting Orgin of new coordinate system
scale.setPivotX(pivotX);
scale.setPivotY(pivotY);
// Adding the transformation to rectangle2
rectangle2.getTransforms().addAll(scale);
Group root = new Group(rectangle1, rectangle2, pivot);
Scene scene = new Scene(root, 450, 500);
stage.setTitle("JavaFX Scaling Transformation (o7planning.org)");
stage.setScene(scene);
stage.show();
}
public static void main(String args[]) {
launch(args);
}
}
5. Shearing Transformation
Shearing - distorting an object by pushing it over in a particular direction
Parhaps you know "shear a sheep", that is an action indicating shearing a sheep. Shearing Transformation is not intended to cut part off the initial geometry. It only distorts the original image.
Shearing uses a point as pivot to transfroms an object.
If the pivot resets the origin of the coordinate system. The shearing factorofthe object by X direction, and Y direction respectively is shearX, shearY, then:
- (x, y) ==> (x + y * shearY, y + x * shearX)
If the pivot point is located at the coordinate (pivotX, pivotY), and the shearing factor in the X direction, Y direction respectively is shearX, shearY, then:
- (x, y) ===> (X", Y")
- X" = pivotX + (x-pivotX) + (y-pivotY) * shearY
- Y" = pivotY + (y-pivotY) + (x-pivotX) * shearX
Example:
Please see the following illustration:
- Assume that the pivot point is at the origin.
- The shearing factor by X axis is shearX = 2.
- The shearing factor by Y axis is shearY = 0.
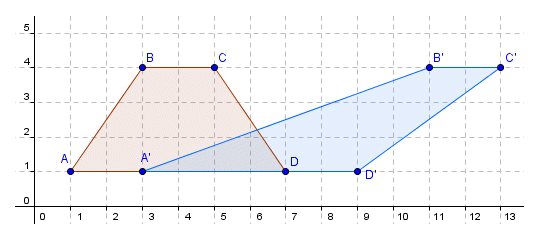
We will obtain the result:
- xA" = xA + shearY * yA = 1 + 2 * 1 = 3
- yA" = yA + shearX * xA = 1 + 0 * 1 = 1
- xB" = xB + shearY * yB = 3 + 2 * 4 = 11
- yB" = yB + shearX + yB = 4 + 0 * 4 = 4
JavaFX Example:
There are two rectangles, at the same location and in the same size. Select a pivot point and use the Shearing Transformation for the second rectangle, and see the result:
ShearingExample.java
package org.o7planning.javafx.transformation;
import javafx.application.Application;
import javafx.scene.Group;
import javafx.scene.Scene;
import javafx.scene.paint.Color;
import javafx.scene.shape.Circle;
import javafx.scene.shape.Rectangle;
import javafx.scene.transform.Shear;
import javafx.stage.Stage;
public class ShearingExample extends Application {
@Override
public void start(Stage stage) {
final int x = 120;
final int y = 80;
final int width = 150;
final int height = 100;
int pivotX = 40;
int pivotY = 50;
double shearX = 0.2;
double shearY = 0.5;
// (all)
// Rectangle1
Rectangle rectangle1 = new Rectangle(x, y, width, height);
rectangle1.setFill(Color.BLUE);
rectangle1.setStroke(Color.BLACK);
// (all)
// Rectangle2
Rectangle rectangle2 = new Rectangle(x, y, width, height);
rectangle2.setFill(Color.BURLYWOOD);
rectangle2.setStroke(Color.BLACK);
Circle pivot = new Circle(pivotX, pivotY, 3);
pivot.setFill(Color.RED);
// Creating the Shearing transformation
Shear shear = new Shear();
// Setting the pivot point
shear.setPivotX(pivotX);
shear.setPivotY(pivotY);
// Setting the shearing factor
shear.setX(shearX);
shear.setY(shearY);
// Adding the transformation to rectangle2
rectangle2.getTransforms().addAll(shear);
Group root = new Group(rectangle1, rectangle2, pivot);
Scene scene = new Scene(root, 450, 300);
stage.setTitle("Shearing Transformation (o7planning.org)");
stage.setScene(scene);
stage.show();
}
public static void main(String args[]) {
launch(args);
}
}
Run the example:
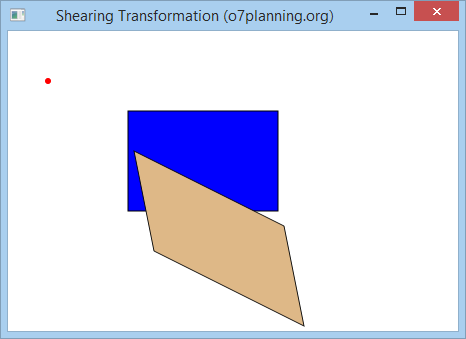
Edit the above example with:
- double shearX = 0;
- double shearY = 0.5;
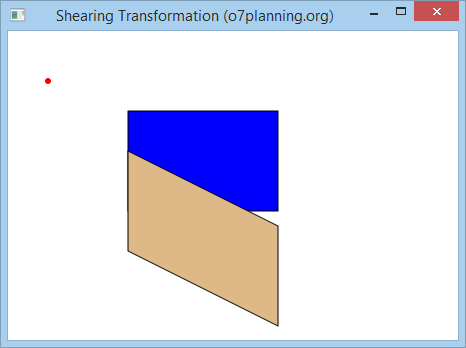
Edit the above example with the values:
- double shearX = 0.2;
- double shearY = 0;
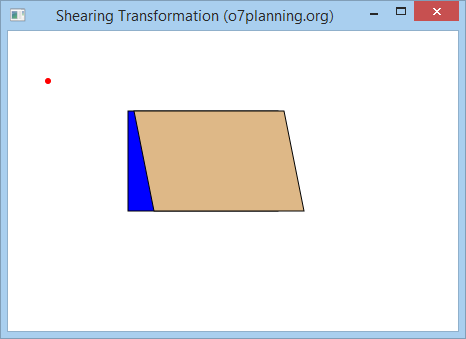
6. Affine Transformation
Affine means a linear transformation that transforms an object from a two or three-dimensional space into another two or three-dimensional space and still keeps the straightness and parallelness of lines unchanged.
One point A with the coordinate (x, y, z) in the three-dimensional space will transfer to position A" with the coordinate (x", y", z") by a matrix multiplication:

The Affine class in the JavaFX has some Constructors. Below are common structructors:
// Constructor to create 2D Affine.
public Affine(double mxx, double mxy, double tx,
double myx, double myy, double ty);
// Constructor to create 3D Affine.
public Affine(double mxx, double mxy, double mxz, double tx,
double myx, double myy, double myz, double ty,
double mzx, double mzy, double mzz, double tz) ;
Example:
AffineExample.java
package org.o7planning.javafx.transformation;
import javafx.application.Application;
import javafx.scene.Group;
import javafx.scene.Scene;
import javafx.scene.paint.Color;
import javafx.scene.shape.Circle;
import javafx.scene.shape.Rectangle;
import javafx.scene.transform.Affine;
import javafx.stage.Stage;
public class AffineExample extends Application {
@Override
public void start(Stage stage) {
final int x = 80;
final int y = 90;
final int width = 100;
final int height = 70;
double mxx = 0.2;
double mxy = 1.5;
double myx = 1.5;
double myy = 0.5;
double tx = 40;
double ty = 50;
// Rectangle1
Rectangle rectangle1 = new Rectangle(x, y, width, height);
rectangle1.setFill(Color.BLUE);
rectangle1.setStroke(Color.BLACK);
// Rectangle2
Rectangle rectangle2 = new Rectangle(x, y, width, height);
rectangle2.setFill(Color.BURLYWOOD);
rectangle2.setStroke(Color.BLACK);
Circle pivot = new Circle(tx, ty, 3);
pivot.setFill(Color.RED);
// Creating the 2D Affine transformation
Affine affine = new Affine(mxx, mxy, tx, myx, myy, ty);
// Adding the transformation to rectangle2
rectangle2.getTransforms().addAll(affine);
Group root = new Group(rectangle1, rectangle2, pivot);
Scene scene = new Scene(root, 450, 500);
stage.setTitle("JavaFX Affine Transformation (o7planning.org)");
stage.setScene(scene);
stage.show();
}
public static void main(String args[]) {
launch(args);
}
}
Run the example:
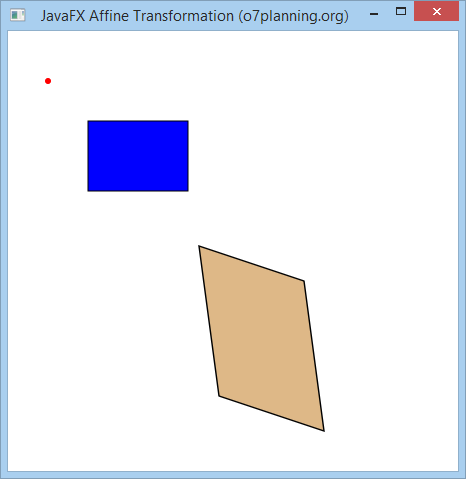
7. Combination of transformations
You can apply many transformations to a geometric object:
Example:
In this example, you have 3 rectangles at the same location and in the same size. Create two transformations such as Rotation and Translation. Add the Rotation to the second rectangle and both above transformations to the third rectangle and see the result.
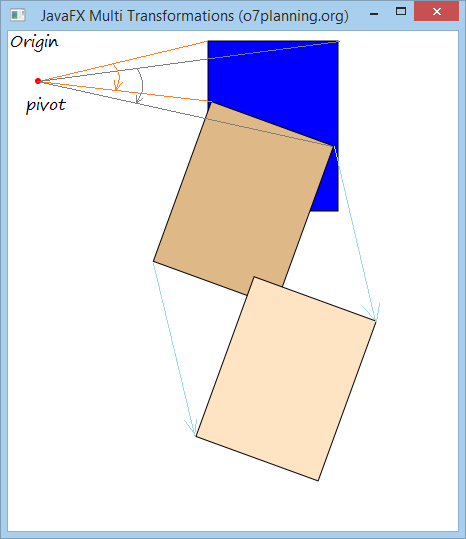
MultipleTransformationsExample.java
package org.o7planning.javafx.transformation;
import javafx.application.Application;
import javafx.scene.Group;
import javafx.scene.Scene;
import javafx.scene.paint.Color;
import javafx.scene.shape.Circle;
import javafx.scene.shape.Rectangle;
import javafx.scene.transform.Rotate;
import javafx.scene.transform.Translate;
import javafx.stage.Stage;
public class MultipleTransformationsExample extends Application {
@Override
public void start(Stage stage) {
final int x = 200;
final int y = 10;
final int width = 130;
final int height = 170;
int pivotX = 30;
int pivotY = 50;
// Rectangle1
Rectangle rectangle1 = new Rectangle(x, y, width, height);
rectangle1.setFill(Color.BLUE);
rectangle1.setStroke(Color.BLACK);
// Rectangle2
Rectangle rectangle2 = new Rectangle(x, y, width, height);
rectangle2.setFill(Color.BURLYWOOD);
rectangle2.setStroke(Color.BLACK);
// Rectangle3
Rectangle rectangle3 = new Rectangle(x, y, width, height);
rectangle3.setFill(Color.BISQUE);
rectangle3.setStroke(Color.BLACK);
Circle pivot = new Circle(pivotX, pivotY, 3);
pivot.setFill(Color.RED);
// Creating the rotation transformation
Rotate rotate = new Rotate();
// Setting the angle for the rotation (20 degrees)
rotate.setAngle(20);
// Setting pivot points for the rotation
rotate.setPivotX(pivotX);
rotate.setPivotY(pivotY);
// Adding the transformation to rectangle2
rectangle2.getTransforms().addAll(rotate);
// Create Translation Transformation.
Translate translate = new Translate(100, 150);
// Adding 2 transformations to rectangle3
rectangle3.getTransforms().addAll(rotate, translate);
Group root = new Group(rectangle1, rectangle2,rectangle3, pivot);
Scene scene = new Scene(root, 450, 500);
stage.setTitle("JavaFX Multi Transformations (o7planning.org)");
stage.setScene(scene);
stage.show();
}
public static void main(String args[]) {
launch(args);
}
}
JavaFX Tutorials
- Open a new Window in JavaFX
- JavaFX ChoiceDialog Tutorial with Examples
- JavaFX Alert Dialogs Tutorial with Examples
- JavaFX TextInputDialog Tutorial with Examples
- Install e(fx)clipse for Eclipse (JavaFX Tooling)
- Install JavaFX Scene Builder for Eclipse
- JavaFX Tutorial for Beginners - Hello JavaFX
- JavaFX FlowPane Layout Tutorial with Examples
- JavaFX TilePane Layout Tutorial with Examples
- JavaFX HBox, VBox Layout Tutorial with Examples
- JavaFX BorderPane Layout Tutorial with Examples
- JavaFX AnchorPane Layout Tutorial with Examples
- JavaFX TitledPane Tutorial with Examples
- JavaFX Accordion Tutorial with Examples
- JavaFX ListView Tutorial with Examples
- JavaFX Group Tutorial with Examples
- JavaFX ComboBox Tutorial with Examples
- JavaFX Transformations Tutorial with Examples
- JavaFX Effects Tutorial with Examples
- JavaFX GridPane Layout Tutorial with Examples
- JavaFX StackPane Layout Tutorial with Examples
- JavaFX ScrollPane Tutorial with Examples
- JavaFX WebView and WebEngine Tutorial with Examples
- JavaFX HTMLEditor Tutorial with Examples
- JavaFX TableView Tutorial with Examples
- JavaFX TreeView Tutorial with Examples
- JavaFX TreeTableView Tutorial with Examples
- JavaFX Menu Tutorial with Examples
- JavaFX ContextMenu Tutorial with Examples
- JavaFX Image and ImageView Tutorial with Examples
- JavaFX Label Tutorial with Examples
- JavaFX Hyperlink Tutorial with Examples
- JavaFX Button Tutorial with Examples
- JavaFX ToggleButton Tutorial with Examples
- JavaFX RadioButton Tutorial with Examples
- JavaFX MenuButton and SplitMenuButton Tutorial with Examples
- JavaFX TextField Tutorial with Examples
- JavaFX PasswordField Tutorial with Examples
- JavaFX TextArea Tutorial with Examples
- JavaFX Slider Tutorial with Examples
- JavaFX Spinner Tutorial with Examples
- JavaFX ProgressBar and ProgressIndicator Tutorial with Examples
- JavaFX ChoiceBox Tutorial with Examples
- JavaFX Tooltip Tutorial with Examples
- JavaFX DatePicker Tutorial with Examples
- JavaFX ColorPicker Tutorial with Examples
- JavaFX FileChooser and DirectoryChooser Tutorial with Examples
- JavaFX PieChart Tutorial with Examples
- JavaFX AreaChart and StackedAreaChart Tutorial with Examples
- JavaFX BarChart and StackedBarChart Tutorial with Examples
- JavaFX Line Tutorial with Examples
- JavaFX Rectangle and Ellipse Tutorial with Examples
Show More