JavaFX Line Tutorial with Examples
1. JavaFX Line
In JavaFX, The Line class is used to draw a straight line. The same as class Rectangle, Circle, ...they all are extended from Shape class.
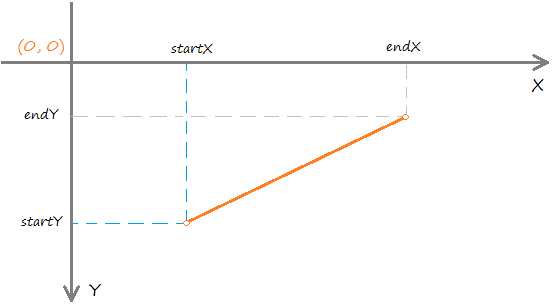
2. Line Example
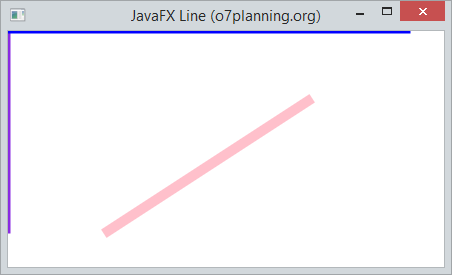
LineDemo.java
package org.o7planning.javafx.line;
import javafx.application.Application;
import javafx.geometry.Insets;
import javafx.scene.Scene;
import javafx.scene.layout.AnchorPane;
import javafx.scene.paint.Color;
import javafx.scene.shape.Line;
import javafx.stage.Stage;
public class LineDemo extends Application {
@Override
public void start(Stage stage) {
// A line in Ox Axis
Line oxLine1 = new Line(0, 0, 400, 0);
// Stroke Width
oxLine1.setStrokeWidth(5);
oxLine1.setStroke(Color.BLUE);
// A line in Oy Axis
Line oyLine = new Line(0, 0, 0, 200);
// Stroke Width
oyLine.setStrokeWidth(5);
oyLine.setStroke(Color.BLUEVIOLET);
// An other Line
Line line = new Line();
line.setStartX(100.0f);
line.setStartY(200.0f);
line.setEndX(300.0f);
line.setEndY(70.0f);
line.setStrokeWidth(10);
line.setStroke(Color.PINK);
AnchorPane root = new AnchorPane();
root.setPadding(new Insets(15));
final Scene scene = new Scene(root, 400, 250);
scene.setFill(null);
root.getChildren().addAll(oyLine, oxLine1, line);
stage.setTitle("JavaFX Line (o7planning.org)");
stage.setScene(scene);
stage.show();
}
public static void main(String[] args) {
launch(args);
}
}
3. The properties of Line
smooth
Value true if you want to turn on anti-aliasing, false to turn off anti-aliasing.
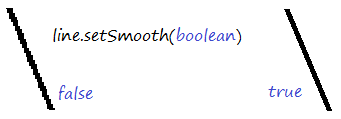
strokeWidth
Set a line's width.

stroke
Using setStroke method to setting color for Line.
// Set Line color.
line.setStroke(Color.RED);
Stroke Dash Array
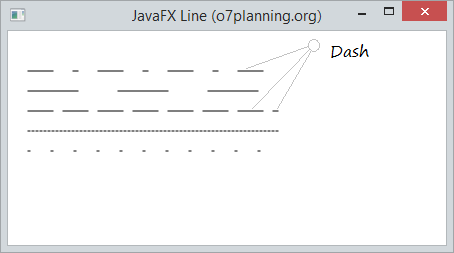
LineStrokeDashArrayDemo.java
package org.o7planning.javafx.line;
import javafx.application.Application;
import javafx.geometry.Insets;
import javafx.scene.Scene;
import javafx.scene.layout.AnchorPane;
import javafx.scene.shape.Line;
import javafx.stage.Stage;
public class LineStrokeDashArrayDemo extends Application {
@Override
public void start(Stage stage) {
Line line1 = new Line(20, 40, 270, 40);
line1.getStrokeDashArray().addAll(25.0, 20.0, 5.0, 20.0);
Line line2 = new Line(20, 60, 270, 60);
line2.getStrokeDashArray().addAll(50.0, 40.0);
Line line3 = new Line(20, 80, 270, 80);
line3.getStrokeDashArray().addAll(25.0, 10.0);
Line line4 = new Line(20, 100, 270, 100);
line4.getStrokeDashArray().addAll(2.0);
Line line5 = new Line(20, 120, 270, 120);
line5.getStrokeDashArray().addAll(2.0, 21.0);
AnchorPane root = new AnchorPane();
root.setPadding(new Insets(5));
final Scene scene = new Scene(root, 400, 250);
root.getChildren().addAll(line1, line2, line3, line4, line5);
stage.setTitle("JavaFX Line (o7planning.org)");
stage.setScene(scene);
stage.show();
}
public static void main(String[] args) {
launch(args);
}
}
strokeDashOffset
In addition to the stroke-dasharray property, there is a possiblity to translate the beginning of the dash. This is controlled by the stroke-dashoffset property.
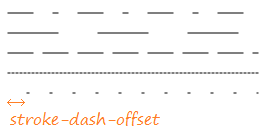
strokeLineCap
strokeLineCap - specifies the shape to be used at the end of open subpaths when they are stroked. There are three styles:
- StrokeLineCap.BUTT
- StrokeLineCap.ROUND
- StrokeLineCap.SQUARE

strokeLineJoin
stroke-linejoin: specifies the shape to be used at the corners of paths or basic shapes when they are stroked. There are three types:
- StrokeLineJoin.MITER
- StrokeLineJoin.BEVEL
- StrokeLineJoin.ROUND
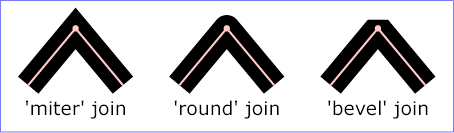
strokeMiterLimit
strokeMiterLimit - Is a value in range [0,1] that is applied in the case of strokeLineJoin = StoreLineJoin.MITER in order to limit the ratio between miterLengh and strokeWidth
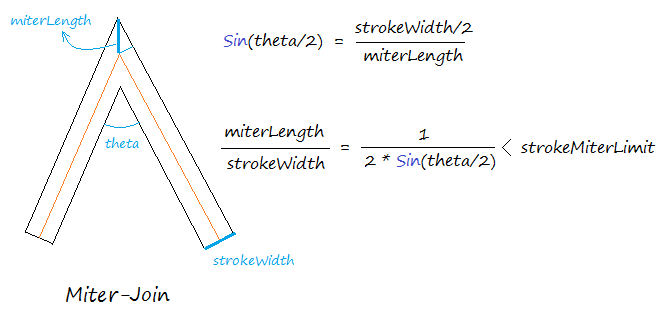
The angle between two straight lines is small (acute angle). The ratio between miterLength and storeWidth can be greater than storeMiterLimit. In this case, the shape of intersection points between two straight lines will be changed from "Miter-Join" to "Bevel-Join".
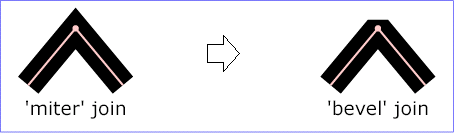
JavaFX Tutorials
- Open a new Window in JavaFX
- JavaFX ChoiceDialog Tutorial with Examples
- JavaFX Alert Dialogs Tutorial with Examples
- JavaFX TextInputDialog Tutorial with Examples
- Install e(fx)clipse for Eclipse (JavaFX Tooling)
- Install JavaFX Scene Builder for Eclipse
- JavaFX Tutorial for Beginners - Hello JavaFX
- JavaFX FlowPane Layout Tutorial with Examples
- JavaFX TilePane Layout Tutorial with Examples
- JavaFX HBox, VBox Layout Tutorial with Examples
- JavaFX BorderPane Layout Tutorial with Examples
- JavaFX AnchorPane Layout Tutorial with Examples
- JavaFX TitledPane Tutorial with Examples
- JavaFX Accordion Tutorial with Examples
- JavaFX ListView Tutorial with Examples
- JavaFX Group Tutorial with Examples
- JavaFX ComboBox Tutorial with Examples
- JavaFX Transformations Tutorial with Examples
- JavaFX Effects Tutorial with Examples
- JavaFX GridPane Layout Tutorial with Examples
- JavaFX StackPane Layout Tutorial with Examples
- JavaFX ScrollPane Tutorial with Examples
- JavaFX WebView and WebEngine Tutorial with Examples
- JavaFX HTMLEditor Tutorial with Examples
- JavaFX TableView Tutorial with Examples
- JavaFX TreeView Tutorial with Examples
- JavaFX TreeTableView Tutorial with Examples
- JavaFX Menu Tutorial with Examples
- JavaFX ContextMenu Tutorial with Examples
- JavaFX Image and ImageView Tutorial with Examples
- JavaFX Label Tutorial with Examples
- JavaFX Hyperlink Tutorial with Examples
- JavaFX Button Tutorial with Examples
- JavaFX ToggleButton Tutorial with Examples
- JavaFX RadioButton Tutorial with Examples
- JavaFX MenuButton and SplitMenuButton Tutorial with Examples
- JavaFX TextField Tutorial with Examples
- JavaFX PasswordField Tutorial with Examples
- JavaFX TextArea Tutorial with Examples
- JavaFX Slider Tutorial with Examples
- JavaFX Spinner Tutorial with Examples
- JavaFX ProgressBar and ProgressIndicator Tutorial with Examples
- JavaFX ChoiceBox Tutorial with Examples
- JavaFX Tooltip Tutorial with Examples
- JavaFX DatePicker Tutorial with Examples
- JavaFX ColorPicker Tutorial with Examples
- JavaFX FileChooser and DirectoryChooser Tutorial with Examples
- JavaFX PieChart Tutorial with Examples
- JavaFX AreaChart and StackedAreaChart Tutorial with Examples
- JavaFX BarChart and StackedBarChart Tutorial with Examples
- JavaFX Line Tutorial with Examples
- JavaFX Rectangle and Ellipse Tutorial with Examples
Show More