JavaFX BorderPane Layout Tutorial with Examples
1. BorderPane Layout
BorderPane is a container, which is divided into 5 separate areas, each of area can contain a subcomponent.
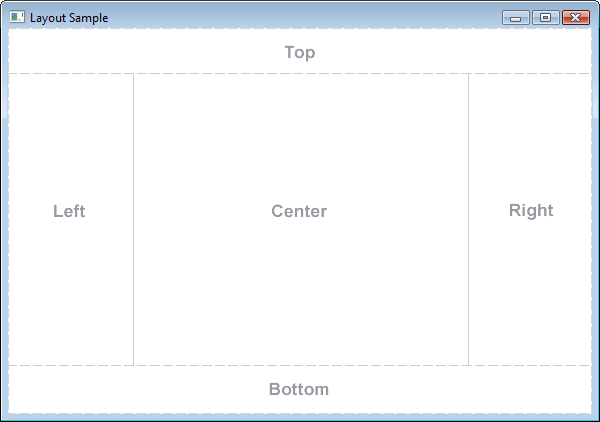
- Top/Bottom area: Can shrink/expand horizontally and keep the height unchanged.
- Left/Right area: Can shrink/expand vertically and keep the length unchanged.
- Center area: Can shrink/expand in both directions.
Features of these above areas are illustrated as below:
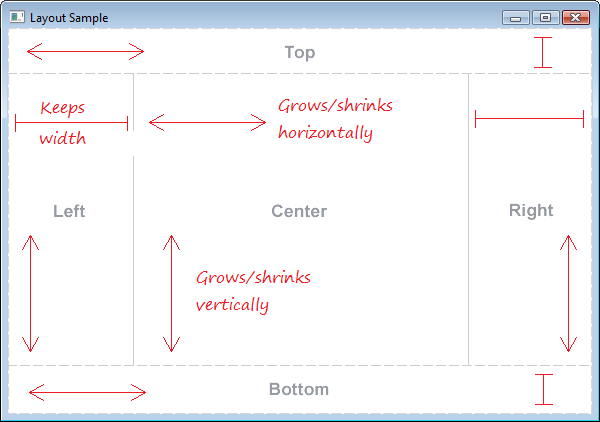
If a certain area does not contain subcomponents, the area will be occupied by other areas.
For example: The area of TOP has no subcomponents, its space will be occupied by other components:
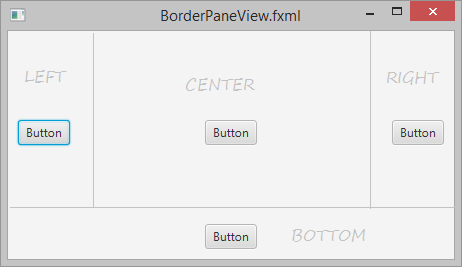
For example: The area of TOP & RIGHT has no subcomponents, its space will be occupied by other components:
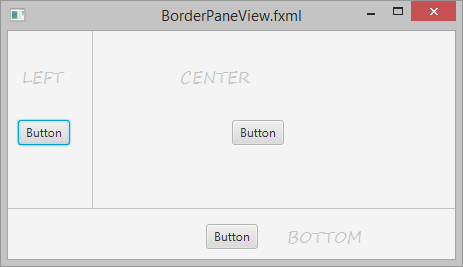
Note: For JavaFX, the subcomponents lying in a certain area of BorderPane maybe not fill the space up, for example if Button lies in an area of BorderPane, by default it will not fill the area up.But if VBox or Hbox lies in an area of BorderPane, by default it will fill the area up.
2. BorderPane example
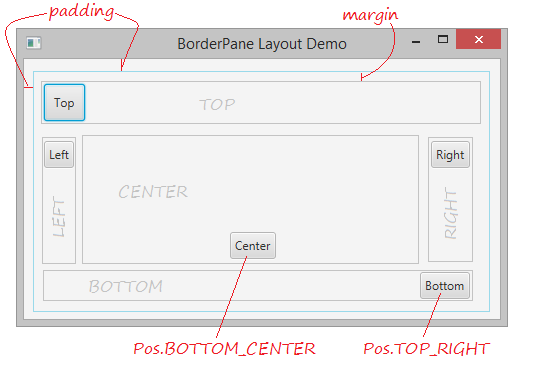
BorderPaneDemo.java
package org.o7planning.javafx.borderpane;
import javafx.application.Application;
import javafx.geometry.Insets;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.BorderPane;
import javafx.stage.Stage;
public class BorderPaneDemo extends Application {
@Override
public void start(Stage primaryStage) throws Exception {
BorderPane root = new BorderPane();
root.setPadding(new Insets(15, 20, 10, 10));
// TOP
Button btnTop = new Button("Top");
btnTop.setPadding(new Insets(10, 10, 10, 10));
root.setTop(btnTop);
// Set margin for top area.
BorderPane.setMargin(btnTop, new Insets(10, 10, 10, 10));
// LEFT
Button btnLeft = new Button("Left");
btnLeft.setPadding(new Insets(5, 5, 5, 5));
root.setLeft(btnLeft);
// Set margin for left area.
BorderPane.setMargin(btnLeft, new Insets(10, 10, 10, 10));
// CENTER
Button btnCenter = new Button("Center");
btnCenter.setPadding(new Insets(5, 5, 5, 5));
root.setCenter(btnCenter);
// Alignment.
BorderPane.setAlignment(btnCenter, Pos.BOTTOM_CENTER);
// RIGHT
Button btnRight = new Button("Right");
btnRight.setPadding(new Insets(5, 5, 5, 5));
root.setRight(btnRight);
// Set margin for right area.
BorderPane.setMargin(btnRight, new Insets(10, 10, 10, 10));
// BOTTOM
Button btnBottom = new Button("Bottom");
btnBottom.setPadding(new Insets(5, 5, 5, 5));
root.setBottom(btnBottom);
// Alignment.
BorderPane.setAlignment(btnBottom, Pos.TOP_RIGHT);
// Set margin for bottom area.
BorderPane.setMargin(btnBottom, new Insets(10, 10, 10, 10));
Scene scene = new Scene(root, 550, 250);
primaryStage.setTitle("BorderPane Layout Demo");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Running the example:
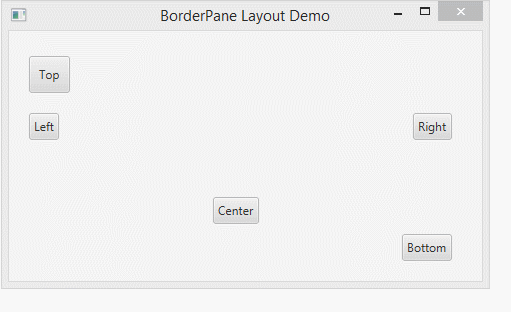
JavaFX Tutorials
- Open a new Window in JavaFX
- JavaFX ChoiceDialog Tutorial with Examples
- JavaFX Alert Dialogs Tutorial with Examples
- JavaFX TextInputDialog Tutorial with Examples
- Install e(fx)clipse for Eclipse (JavaFX Tooling)
- Install JavaFX Scene Builder for Eclipse
- JavaFX Tutorial for Beginners - Hello JavaFX
- JavaFX FlowPane Layout Tutorial with Examples
- JavaFX TilePane Layout Tutorial with Examples
- JavaFX HBox, VBox Layout Tutorial with Examples
- JavaFX BorderPane Layout Tutorial with Examples
- JavaFX AnchorPane Layout Tutorial with Examples
- JavaFX TitledPane Tutorial with Examples
- JavaFX Accordion Tutorial with Examples
- JavaFX ListView Tutorial with Examples
- JavaFX Group Tutorial with Examples
- JavaFX ComboBox Tutorial with Examples
- JavaFX Transformations Tutorial with Examples
- JavaFX Effects Tutorial with Examples
- JavaFX GridPane Layout Tutorial with Examples
- JavaFX StackPane Layout Tutorial with Examples
- JavaFX ScrollPane Tutorial with Examples
- JavaFX WebView and WebEngine Tutorial with Examples
- JavaFX HTMLEditor Tutorial with Examples
- JavaFX TableView Tutorial with Examples
- JavaFX TreeView Tutorial with Examples
- JavaFX TreeTableView Tutorial with Examples
- JavaFX Menu Tutorial with Examples
- JavaFX ContextMenu Tutorial with Examples
- JavaFX Image and ImageView Tutorial with Examples
- JavaFX Label Tutorial with Examples
- JavaFX Hyperlink Tutorial with Examples
- JavaFX Button Tutorial with Examples
- JavaFX ToggleButton Tutorial with Examples
- JavaFX RadioButton Tutorial with Examples
- JavaFX MenuButton and SplitMenuButton Tutorial with Examples
- JavaFX TextField Tutorial with Examples
- JavaFX PasswordField Tutorial with Examples
- JavaFX TextArea Tutorial with Examples
- JavaFX Slider Tutorial with Examples
- JavaFX Spinner Tutorial with Examples
- JavaFX ProgressBar and ProgressIndicator Tutorial with Examples
- JavaFX ChoiceBox Tutorial with Examples
- JavaFX Tooltip Tutorial with Examples
- JavaFX DatePicker Tutorial with Examples
- JavaFX ColorPicker Tutorial with Examples
- JavaFX FileChooser and DirectoryChooser Tutorial with Examples
- JavaFX PieChart Tutorial with Examples
- JavaFX AreaChart and StackedAreaChart Tutorial with Examples
- JavaFX BarChart and StackedBarChart Tutorial with Examples
- JavaFX Line Tutorial with Examples
- JavaFX Rectangle and Ellipse Tutorial with Examples
Show More