JavaFX AnchorPane Layout Tutorial with Examples
1. AnchorPane Layout
AnchorPane is a container, which is similar to BorderPane. BorderPane is divided into 5 separate areas in order to place subcomponent on while AnchorPane is divided into 5 areas in order to anchor on. Note that 5 areas of AnchorPane is 5 logic areas but no 5 real areas.
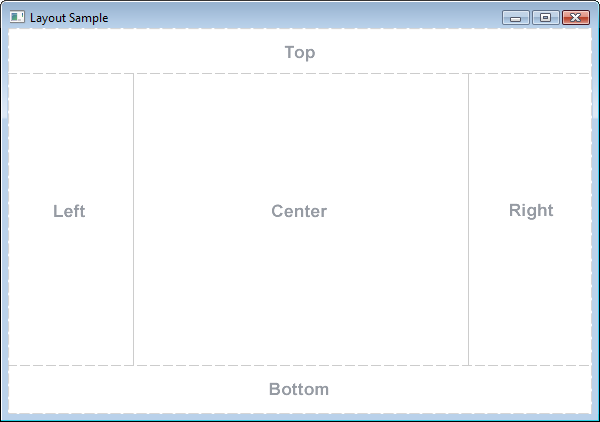
A subcomponent lying in AnchorPane can be anchored into one or more logic areas of AnchorPane.
The below image illustrates a subcomponent which lies in AnchorPane and is anchored on the the left and right sides of AnchorPane. When AnchorPane changes the length, the length of subcomponents will be also accordingly changed.
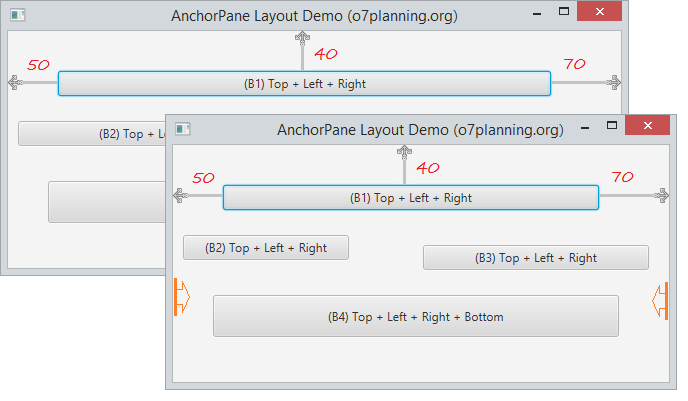
Subcomponents can be anchored on 4 sides of AnchorPane:
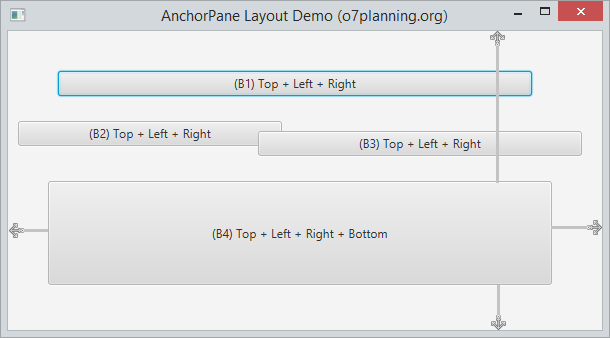
2. AnchorPane example
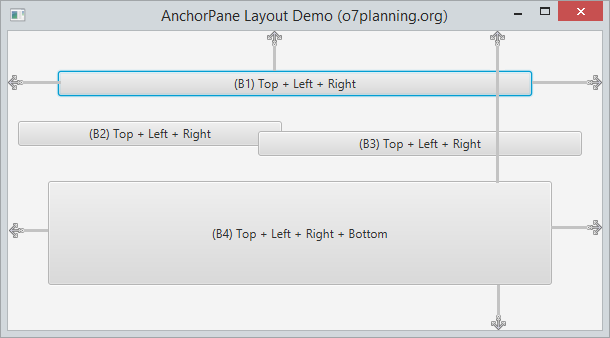
BorderPaneDemo.java
package org.o7planning.javafx.anchorpane;
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.AnchorPane;
import javafx.stage.Stage;
public class AnchorPaneDemo extends Application {
@Override
public void start(Stage primaryStage) throws Exception {
AnchorPane root = new AnchorPane();
Button button1 = new Button("(B1) Top + Left + Right");
Button button2 = new Button("(B2) Top + Left + Right");
Button button3 = new Button("(B3) Top + Left + Right");
Button button4 = new Button("(B4) Top + Left + Right + Bottom");
// (B1) Anchor to the Top + Left + Right
AnchorPane.setTopAnchor(button1, 40.0);
AnchorPane.setLeftAnchor(button1, 50.0);
AnchorPane.setRightAnchor(button1, 70.0);
// (B2) Anchor to the Top + Left + Right
AnchorPane.setTopAnchor(button2, 90.0);
AnchorPane.setLeftAnchor(button2, 10.0);
AnchorPane.setRightAnchor(button2, 320.0);
// (B3) Anchor to the Top + Left + Right
AnchorPane.setTopAnchor(button3, 100.0);
AnchorPane.setLeftAnchor(button3, 250.0);
AnchorPane.setRightAnchor(button3, 20.0);
// (B4) Anchor to the four sides of AnchorPane
AnchorPane.setTopAnchor(button4, 150.0);
AnchorPane.setLeftAnchor(button4, 40.0);
AnchorPane.setRightAnchor(button4, 50.0);
AnchorPane.setBottomAnchor(button4, 45.0);
// Add buttons to AnchorPane
root.getChildren().addAll(button1, button3, button2, button4);
Scene scene = new Scene(root, 550, 250);
primaryStage.setTitle("AnchorPane Layout Demo (o7planning.org)");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Running the example:
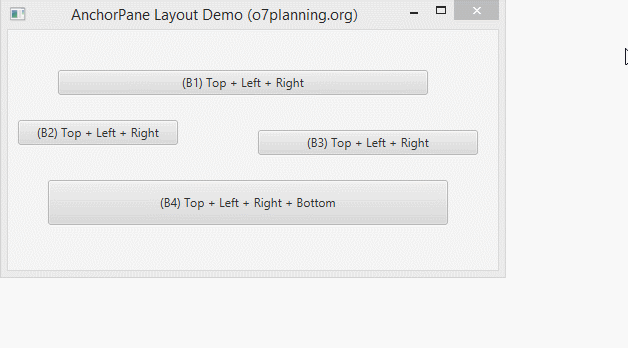
JavaFX Tutorials
- Open a new Window in JavaFX
- JavaFX ChoiceDialog Tutorial with Examples
- JavaFX Alert Dialogs Tutorial with Examples
- JavaFX TextInputDialog Tutorial with Examples
- Install e(fx)clipse for Eclipse (JavaFX Tooling)
- Install JavaFX Scene Builder for Eclipse
- JavaFX Tutorial for Beginners - Hello JavaFX
- JavaFX FlowPane Layout Tutorial with Examples
- JavaFX TilePane Layout Tutorial with Examples
- JavaFX HBox, VBox Layout Tutorial with Examples
- JavaFX BorderPane Layout Tutorial with Examples
- JavaFX AnchorPane Layout Tutorial with Examples
- JavaFX TitledPane Tutorial with Examples
- JavaFX Accordion Tutorial with Examples
- JavaFX ListView Tutorial with Examples
- JavaFX Group Tutorial with Examples
- JavaFX ComboBox Tutorial with Examples
- JavaFX Transformations Tutorial with Examples
- JavaFX Effects Tutorial with Examples
- JavaFX GridPane Layout Tutorial with Examples
- JavaFX StackPane Layout Tutorial with Examples
- JavaFX ScrollPane Tutorial with Examples
- JavaFX WebView and WebEngine Tutorial with Examples
- JavaFX HTMLEditor Tutorial with Examples
- JavaFX TableView Tutorial with Examples
- JavaFX TreeView Tutorial with Examples
- JavaFX TreeTableView Tutorial with Examples
- JavaFX Menu Tutorial with Examples
- JavaFX ContextMenu Tutorial with Examples
- JavaFX Image and ImageView Tutorial with Examples
- JavaFX Label Tutorial with Examples
- JavaFX Hyperlink Tutorial with Examples
- JavaFX Button Tutorial with Examples
- JavaFX ToggleButton Tutorial with Examples
- JavaFX RadioButton Tutorial with Examples
- JavaFX MenuButton and SplitMenuButton Tutorial with Examples
- JavaFX TextField Tutorial with Examples
- JavaFX PasswordField Tutorial with Examples
- JavaFX TextArea Tutorial with Examples
- JavaFX Slider Tutorial with Examples
- JavaFX Spinner Tutorial with Examples
- JavaFX ProgressBar and ProgressIndicator Tutorial with Examples
- JavaFX ChoiceBox Tutorial with Examples
- JavaFX Tooltip Tutorial with Examples
- JavaFX DatePicker Tutorial with Examples
- JavaFX ColorPicker Tutorial with Examples
- JavaFX FileChooser and DirectoryChooser Tutorial with Examples
- JavaFX PieChart Tutorial with Examples
- JavaFX AreaChart and StackedAreaChart Tutorial with Examples
- JavaFX BarChart and StackedBarChart Tutorial with Examples
- JavaFX Line Tutorial with Examples
- JavaFX Rectangle and Ellipse Tutorial with Examples
Show More