JavaFX Alert Dialogs Tutorial with Examples
1. JavaFX Alert
The Alert class subclasses the Dialog class, and provides support for a number of pre-built dialog types that can be easily shown to users to prompt for a response. Therefore, for many users, the Alert class is the most suited class for their needs (as opposed to using Dialog directly). Alternatively, users who want to prompt a user for text input or to make a choice from a list of options would be better served by using TextInputDialog and ChoiceDialog, respectively.
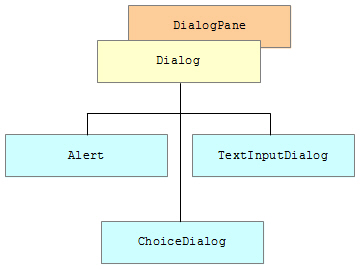
Alert is at default a window with the modelity of Modelity.WINDOW_MODAL. However, you can change by using alert.initModality(Modality).
By default, any window that opens an Alert dialog will be the parent window of this Alert.
You can see more explanation about the Modelity in the following posts:
- JavaFX Dialog
Below is the illustration of the Alert window structure:
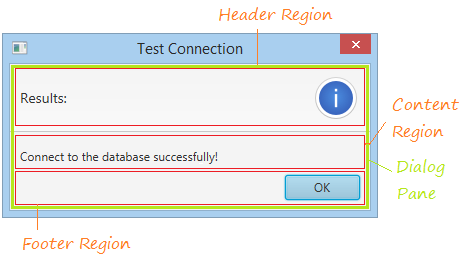
Header Region:
This region is used to display a short notification and icon.
Content Region:
By default, Content Region display a Label, you can set the text for this Label via alert.setContentText(String) method. You can also display another Node in the Content Region via alert.getDialogPane().setContent(Node).
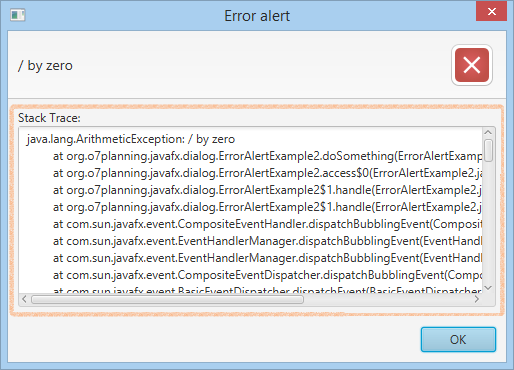
Footer Region:
This region is used to display Buttons. You can customize the displayed buttons.
2. Information Alert
Information Alert means a dialog window, displaying information. Below is an image of a standard Information Alert:
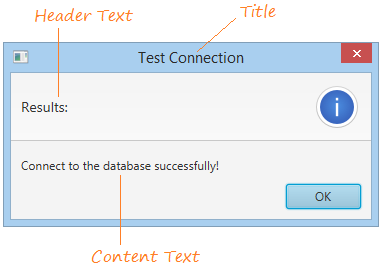
The image of an Information Alert with default Header Text:
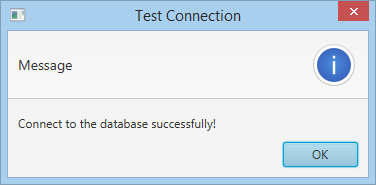
Image of an Information Alert without Header Text:
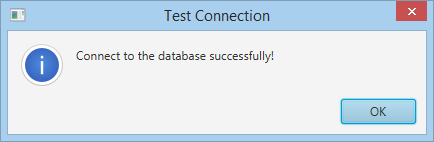
Example:
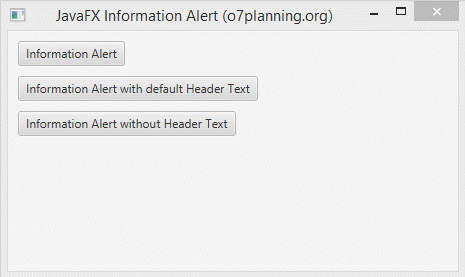
InformationAlertExample.java
package org.o7planning.javafx.alert;
import javafx.application.Application;
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
import javafx.geometry.Insets;
import javafx.scene.Scene;
import javafx.scene.control.Alert;
import javafx.scene.control.Alert.AlertType;
import javafx.scene.control.Button;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class InformationAlertExample extends Application {
// Show a Information Alert with header Text
private void showAlertWithHeaderText() {
Alert alert = new Alert(AlertType.INFORMATION);
alert.setTitle("Test Connection");
alert.setHeaderText("Results:");
alert.setContentText("Connect to the database successfully!");
alert.showAndWait();
}
// Show a Information Alert with default header Text
private void showAlertWithDefaultHeaderText() {
Alert alert = new Alert(AlertType.INFORMATION);
alert.setTitle("Test Connection");
// alert.setHeaderText("Results:");
alert.setContentText("Connect to the database successfully!");
alert.showAndWait();
}
// Show a Information Alert without Header Text
private void showAlertWithoutHeaderText() {
Alert alert = new Alert(AlertType.INFORMATION);
alert.setTitle("Test Connection");
// Header Text: null
alert.setHeaderText(null);
alert.setContentText("Connect to the database successfully!");
alert.showAndWait();
}
@Override
public void start(Stage stage) {
VBox root = new VBox();
root.setPadding(new Insets(10));
root.setSpacing(10);
Button button1 = new Button("Information Alert");
Button button2 = new Button("Information Alert with default Header Text");
Button button3 = new Button("Information Alert without Header Text");
button1.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
showAlertWithHeaderText();
}
});
button2.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
showAlertWithDefaultHeaderText();
}
});
button3.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
showAlertWithoutHeaderText();
}
});
root.getChildren().addAll(button1, button2, button3);
Scene scene = new Scene(root, 450, 250);
stage.setTitle("JavaFX Information Alert (o7planning.org)");
stage.setScene(scene);
stage.show();
}
public static void main(String args[]) {
launch(args);
}
}
3. Warning Alert
Warning Alert is similar to an Information Alert, except for its icon and intended use.The Warning Alert is used to warn an user for potential risks or problems, although it doesn't probably occur.
Image of a standard Warning Alert :
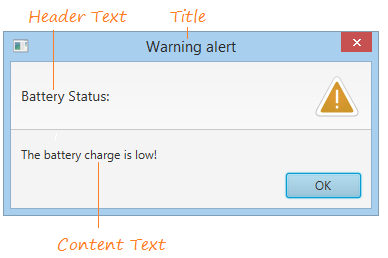
Image of a Warning Alert with default Header Text:
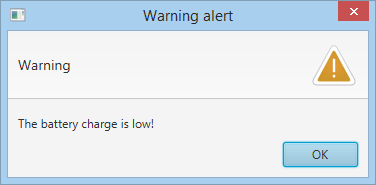
Image of a Warning Alert without Header Text:
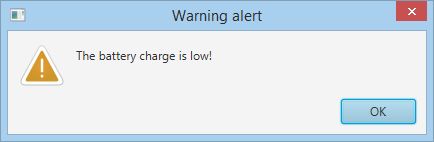
Example:
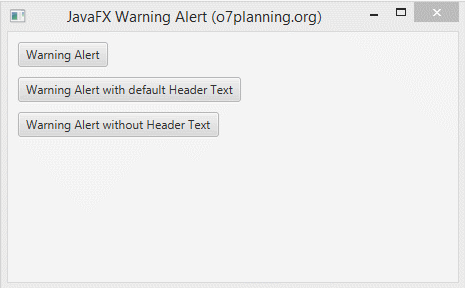
WarningAlertExample.java
package org.o7planning.javafx.alert;
import javafx.application.Application;
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
import javafx.geometry.Insets;
import javafx.scene.Scene;
import javafx.scene.control.Alert;
import javafx.scene.control.Alert.AlertType;
import javafx.scene.control.Button;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class WarningAlertExample extends Application {
// Show a Warning Alert with header Text
private void showAlertWithHeaderText() {
Alert alert = new Alert(AlertType.WARNING);
alert.setTitle("Warning alert");
alert.setHeaderText("Battery Status:");
alert.setContentText("The battery charge is low!");
alert.showAndWait();
}
// Show a Warning Alert with default header Text
private void showAlertWithDefaultHeaderText() {
Alert alert = new Alert(AlertType.WARNING);
alert.setTitle("Warning alert");
// alert.setHeaderText("Battery Status:");
alert.setContentText("The battery charge is low!");
alert.showAndWait();
}
// Show a Warning Alert without Header Text
private void showAlertWithoutHeaderText() {
Alert alert = new Alert(AlertType.WARNING);
alert.setTitle("Warning alert");
// Header Text: null
alert.setHeaderText(null);
alert.setContentText("The battery charge is low!");
alert.showAndWait();
}
@Override
public void start(Stage stage) {
VBox root = new VBox();
root.setPadding(new Insets(10));
root.setSpacing(10);
Button button1 = new Button("Warning Alert");
Button button2 = new Button("Warning Alert with default Header Text");
Button button3 = new Button("Warning Alert without Header Text");
button1.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
showAlertWithHeaderText();
}
});
button2.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
showAlertWithDefaultHeaderText();
}
});
button3.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
showAlertWithoutHeaderText();
}
});
root.getChildren().addAll(button1, button2, button3);
Scene scene = new Scene(root, 450, 250);
stage.setTitle("JavaFX Warning Alert (o7planning.org)");
stage.setScene(scene);
stage.show();
}
public static void main(String args[]) {
launch(args);
}
}
4. Error Alert
Error Alert is the same as Informtion Alert and Warning Alert, except for icon and intended use. The Error Alert is used to notify of occurence of a serious matter.
To create an Error Alert, you need to useAlertType.ERROR.
* Error Alert *
Alert alert = new Alert(AlertType.ERROR);
alert.setTitle("Error alert");
alert.setHeaderText("Can not add user");
alert.setContentText("The 'abc' user does not exists!");
alert.showAndWait();
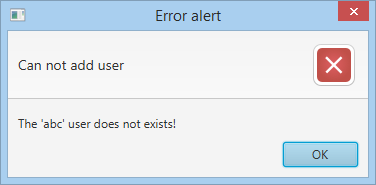
In fact, when an error takes place, you display an Error Alert for users to know. It consists of short information and probably details of error in which case, you need to set customizing content for the Content Region.
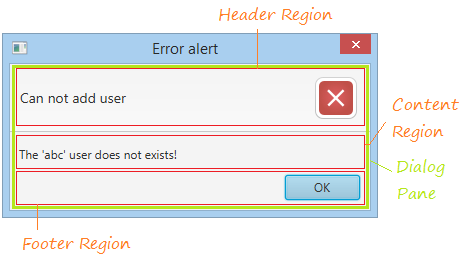
Example:
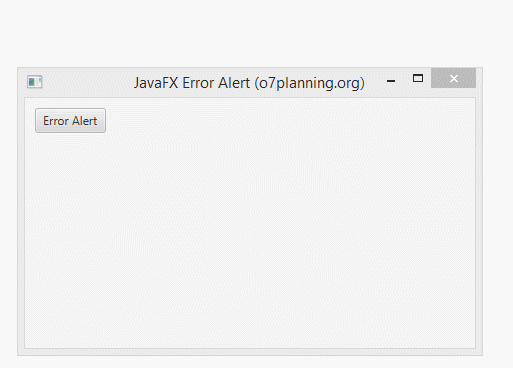
ErrorAlertExample2.java
package org.o7planning.javafx.alert;
import java.io.PrintWriter;
import java.io.StringWriter;
import javafx.application.Application;
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
import javafx.geometry.Insets;
import javafx.scene.Scene;
import javafx.scene.control.Alert;
import javafx.scene.control.Alert.AlertType;
import javafx.scene.control.Button;
import javafx.scene.control.Label;
import javafx.scene.control.TextArea;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class ErrorAlertExample2 extends Application {
private void showError(Exception e) {
Alert alert = new Alert(AlertType.ERROR);
alert.setTitle("Error alert");
alert.setHeaderText(e.getMessage());
VBox dialogPaneContent = new VBox();
Label label = new Label("Stack Trace:");
String stackTrace = this.getStackTrace(e);
TextArea textArea = new TextArea();
textArea.setText(stackTrace);
dialogPaneContent.getChildren().addAll(label, textArea);
// Set content for Dialog Pane
alert.getDialogPane().setContent(dialogPaneContent);
alert.showAndWait();
}
private void doSomething() {
try {
// An error has occurred here.
int a = 100 / 0;
} catch (Exception e) {
showError(e);
}
}
private String getStackTrace(Exception e) {
StringWriter sw = new StringWriter();
PrintWriter pw = new PrintWriter(sw);
e.printStackTrace(pw);
String s = sw.toString();
return s;
}
@Override
public void start(Stage stage) {
VBox root = new VBox();
root.setPadding(new Insets(10));
root.setSpacing(10);
Button button1 = new Button("Error Alert");
button1.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
doSomething();
}
});
root.getChildren().addAll(button1);
Scene scene = new Scene(root, 450, 250);
stage.setTitle("JavaFX Error Alert (o7planning.org)");
stage.setScene(scene);
stage.show();
}
public static void main(String args[]) {
launch(args);
}
}
5. Confirmation Alert
Confirmation Alert displays to ask users to confirm that an action will be done or not. It is defaulted that the Confirmation Alert will have 2 options for users such as OK or Cancel.
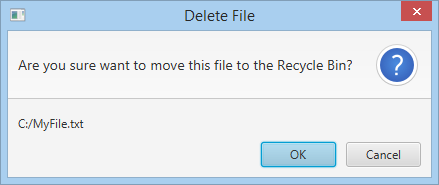
Example:
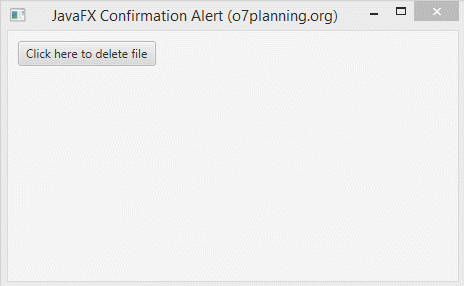
ConfirmationAlertExample.java
package org.o7planning.javafx.alert;
import java.util.Optional;
import javafx.application.Application;
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
import javafx.geometry.Insets;
import javafx.scene.Scene;
import javafx.scene.control.Alert;
import javafx.scene.control.Alert.AlertType;
import javafx.scene.control.Button;
import javafx.scene.control.ButtonType;
import javafx.scene.control.Label;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class ConfirmationAlertExample extends Application {
private Label label;
private void showConfirmation() {
Alert alert = new Alert(AlertType.CONFIRMATION);
alert.setTitle("Delete File");
alert.setHeaderText("Are you sure want to move this file to the Recycle Bin?");
alert.setContentText("C:/MyFile.txt");
// option != null.
Optional<ButtonType> option = alert.showAndWait();
if (option.get() == null) {
this.label.setText("No selection!");
} else if (option.get() == ButtonType.OK) {
this.label.setText("File deleted!");
} else if (option.get() == ButtonType.CANCEL) {
this.label.setText("Cancelled!");
} else {
this.label.setText("-");
}
}
@Override
public void start(Stage stage) {
VBox root = new VBox();
root.setPadding(new Insets(10));
root.setSpacing(10);
this.label = new Label();
Button button = new Button("Click here to delete file");
button.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
showConfirmation();
}
});
root.getChildren().addAll(button, label);
Scene scene = new Scene(root, 450, 250);
stage.setTitle("JavaFX Confirmation Alert (o7planning.org)");
stage.setScene(scene);
stage.show();
}
public static void main(String args[]) {
launch(args);
}
}
6. Custom Buttons
The Alert dialog allows you to customize buttons to be displayed on the Footer Region. Below is an example:
Example:
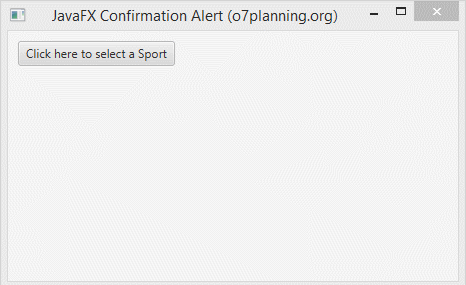
ConfirmationAlertExample2.java
package org.o7planning.javafx.alert;
import java.util.Optional;
import javafx.application.Application;
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
import javafx.geometry.Insets;
import javafx.scene.Scene;
import javafx.scene.control.Alert;
import javafx.scene.control.Alert.AlertType;
import javafx.scene.control.Button;
import javafx.scene.control.ButtonType;
import javafx.scene.control.Label;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class ConfirmationAlertExample2 extends Application {
private Label label;
private void showConfirmation() {
Alert alert = new Alert(AlertType.CONFIRMATION);
alert.setTitle("Select");
alert.setHeaderText("Choose the sport you like:");
ButtonType football = new ButtonType("Football");
ButtonType badminton = new ButtonType("Badminton");
ButtonType volleyball = new ButtonType("Volleyball");
// Remove default ButtonTypes
alert.getButtonTypes().clear();
alert.getButtonTypes().addAll(football, badminton, volleyball);
// option != null.
Optional<ButtonType> option = alert.showAndWait();
if (option.get() == null) {
this.label.setText("No selection!");
} else if (option.get() == football) {
this.label.setText("You like Football");
} else if (option.get() == badminton) {
this.label.setText("You like Badminton");
} else if (option.get() == volleyball) {
this.label.setText("You like Volleyball");
} else {
this.label.setText("-");
}
}
@Override
public void start(Stage stage) {
VBox root = new VBox();
root.setPadding(new Insets(10));
root.setSpacing(10);
this.label = new Label();
Button button = new Button("Click here to select a Sport");
button.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
showConfirmation();
}
});
root.getChildren().addAll(button, label);
Scene scene = new Scene(root, 450, 250);
stage.setTitle("JavaFX Confirmation Alert (o7planning.org)");
stage.setScene(scene);
stage.show();
}
public static void main(String args[]) {
launch(args);
}
}
JavaFX Tutorials
- Open a new Window in JavaFX
- JavaFX ChoiceDialog Tutorial with Examples
- JavaFX Alert Dialogs Tutorial with Examples
- JavaFX TextInputDialog Tutorial with Examples
- Install e(fx)clipse for Eclipse (JavaFX Tooling)
- Install JavaFX Scene Builder for Eclipse
- JavaFX Tutorial for Beginners - Hello JavaFX
- JavaFX FlowPane Layout Tutorial with Examples
- JavaFX TilePane Layout Tutorial with Examples
- JavaFX HBox, VBox Layout Tutorial with Examples
- JavaFX BorderPane Layout Tutorial with Examples
- JavaFX AnchorPane Layout Tutorial with Examples
- JavaFX TitledPane Tutorial with Examples
- JavaFX Accordion Tutorial with Examples
- JavaFX ListView Tutorial with Examples
- JavaFX Group Tutorial with Examples
- JavaFX ComboBox Tutorial with Examples
- JavaFX Transformations Tutorial with Examples
- JavaFX Effects Tutorial with Examples
- JavaFX GridPane Layout Tutorial with Examples
- JavaFX StackPane Layout Tutorial with Examples
- JavaFX ScrollPane Tutorial with Examples
- JavaFX WebView and WebEngine Tutorial with Examples
- JavaFX HTMLEditor Tutorial with Examples
- JavaFX TableView Tutorial with Examples
- JavaFX TreeView Tutorial with Examples
- JavaFX TreeTableView Tutorial with Examples
- JavaFX Menu Tutorial with Examples
- JavaFX ContextMenu Tutorial with Examples
- JavaFX Image and ImageView Tutorial with Examples
- JavaFX Label Tutorial with Examples
- JavaFX Hyperlink Tutorial with Examples
- JavaFX Button Tutorial with Examples
- JavaFX ToggleButton Tutorial with Examples
- JavaFX RadioButton Tutorial with Examples
- JavaFX MenuButton and SplitMenuButton Tutorial with Examples
- JavaFX TextField Tutorial with Examples
- JavaFX PasswordField Tutorial with Examples
- JavaFX TextArea Tutorial with Examples
- JavaFX Slider Tutorial with Examples
- JavaFX Spinner Tutorial with Examples
- JavaFX ProgressBar and ProgressIndicator Tutorial with Examples
- JavaFX ChoiceBox Tutorial with Examples
- JavaFX Tooltip Tutorial with Examples
- JavaFX DatePicker Tutorial with Examples
- JavaFX ColorPicker Tutorial with Examples
- JavaFX FileChooser and DirectoryChooser Tutorial with Examples
- JavaFX PieChart Tutorial with Examples
- JavaFX AreaChart and StackedAreaChart Tutorial with Examples
- JavaFX BarChart and StackedBarChart Tutorial with Examples
- JavaFX Line Tutorial with Examples
- JavaFX Rectangle and Ellipse Tutorial with Examples
Show More