JavaFX Accordion Tutorial with Examples
1. Javafx Accordion
In JavaFX, you can create an Accordion, using Accordion class.
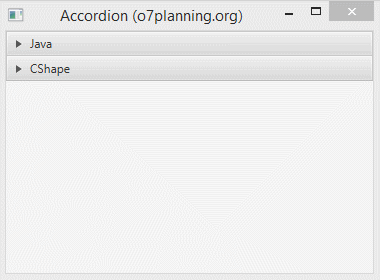
** Accordion **
Accordion root= new Accordion();
root.getPanes().addAll(firstTitledPane, secondTitledPane);
In fact, you can create the interface component which is the same as Accordion using the combination between TitledPane and VBox.
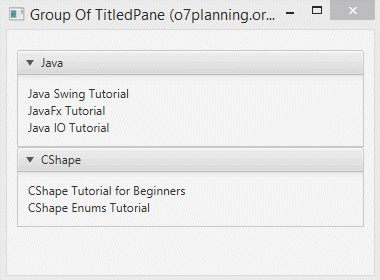
The image below illustrates a group of TitledPanes in a VBox.
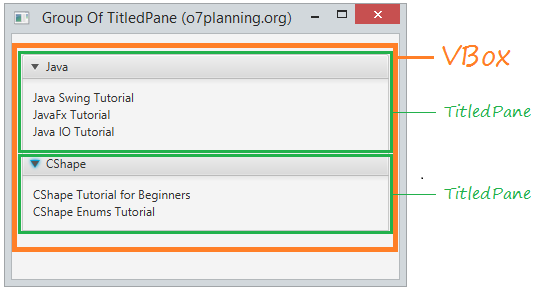
2. Accordion example
AccordionDemo.java
package org.o7planning.javafx.accordion;
import javafx.application.Application;
import javafx.geometry.Insets;
import javafx.scene.Scene;
import javafx.scene.control.Accordion;
import javafx.scene.control.Label;
import javafx.scene.control.TitledPane;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class AccordionDemo extends Application {
@Override
public void start(Stage stage) {
// Create First TitledPane.
TitledPane firstTitledPane = new TitledPane();
firstTitledPane.setText("Java");
VBox content1 = new VBox();
content1.getChildren().add(new Label("Java Swing Tutorial"));
content1.getChildren().add(new Label("JavaFx Tutorial"));
content1.getChildren().add(new Label("Java IO Tutorial"));
firstTitledPane.setContent(content1);
// Create Second TitledPane.
TitledPane secondTitledPane = new TitledPane();
secondTitledPane.setText("CShape");
VBox content2 = new VBox();
content2.getChildren().add(new Label("CShape Tutorial for Beginners"));
content2.getChildren().add(new Label("CShape Enums Tutorial"));
secondTitledPane.setContent(content2);
Accordion root= new Accordion();
root.getPanes().addAll(firstTitledPane, secondTitledPane);
Scene scene = new Scene(root, 300, 200);
stage.setTitle("Accordion (o7planning.org)");
stage.setScene(scene);
stage.show();
}
public static void main(String[] args) {
Application.launch(args);
}
}
Running the example:
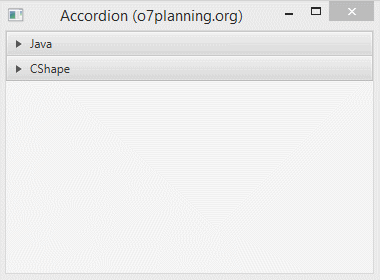
3. Group of TitledPane
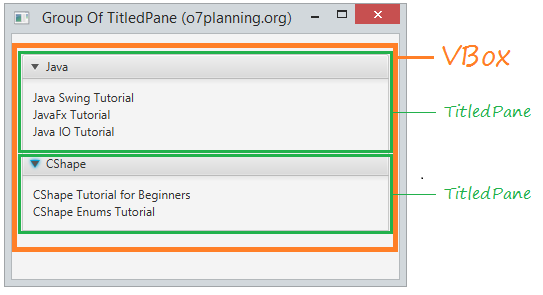
GroupOfTitledPane.java
package org.o7planning.javafx.accordion;
import javafx.application.Application;
import javafx.geometry.Insets;
import javafx.scene.Scene;
import javafx.scene.control.Label;
import javafx.scene.control.TitledPane;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class GroupOfTitledPane extends Application {
@Override
public void start(Stage stage) {
// Create First TitledPane.
TitledPane firstTitledPane = new TitledPane();
firstTitledPane.setText("Java");
VBox content1 = new VBox();
content1.getChildren().add(new Label("Java Swing Tutorial"));
content1.getChildren().add(new Label("JavaFx Tutorial"));
content1.getChildren().add(new Label("Java IO Tutorial"));
firstTitledPane.setContent(content1);
// Create Second TitledPane.
TitledPane secondTitledPane = new TitledPane();
secondTitledPane.setText("CShape");
VBox content2 = new VBox();
content2.getChildren().add(new Label("CShape Tutorial for Beginners"));
content2.getChildren().add(new Label("CShape Enums Tutorial"));
secondTitledPane.setContent(content2);
// Create Root Pane.
VBox root = new VBox();
root.setPadding(new Insets(20, 10, 10, 10));
root.getChildren().addAll(firstTitledPane, secondTitledPane);
Scene scene = new Scene(root, 300, 200);
stage.setTitle("Group Of TitledPane (o7planning.org)");
stage.setScene(scene);
stage.show();
}
public static void main(String[] args) {
Application.launch(args);
}
}
Running the example:
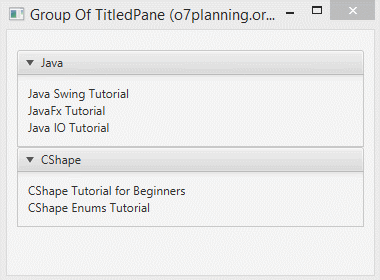
JavaFX Tutorials
- Open a new Window in JavaFX
- JavaFX ChoiceDialog Tutorial with Examples
- JavaFX Alert Dialogs Tutorial with Examples
- JavaFX TextInputDialog Tutorial with Examples
- Install e(fx)clipse for Eclipse (JavaFX Tooling)
- Install JavaFX Scene Builder for Eclipse
- JavaFX Tutorial for Beginners - Hello JavaFX
- JavaFX FlowPane Layout Tutorial with Examples
- JavaFX TilePane Layout Tutorial with Examples
- JavaFX HBox, VBox Layout Tutorial with Examples
- JavaFX BorderPane Layout Tutorial with Examples
- JavaFX AnchorPane Layout Tutorial with Examples
- JavaFX TitledPane Tutorial with Examples
- JavaFX Accordion Tutorial with Examples
- JavaFX ListView Tutorial with Examples
- JavaFX Group Tutorial with Examples
- JavaFX ComboBox Tutorial with Examples
- JavaFX Transformations Tutorial with Examples
- JavaFX Effects Tutorial with Examples
- JavaFX GridPane Layout Tutorial with Examples
- JavaFX StackPane Layout Tutorial with Examples
- JavaFX ScrollPane Tutorial with Examples
- JavaFX WebView and WebEngine Tutorial with Examples
- JavaFX HTMLEditor Tutorial with Examples
- JavaFX TableView Tutorial with Examples
- JavaFX TreeView Tutorial with Examples
- JavaFX TreeTableView Tutorial with Examples
- JavaFX Menu Tutorial with Examples
- JavaFX ContextMenu Tutorial with Examples
- JavaFX Image and ImageView Tutorial with Examples
- JavaFX Label Tutorial with Examples
- JavaFX Hyperlink Tutorial with Examples
- JavaFX Button Tutorial with Examples
- JavaFX ToggleButton Tutorial with Examples
- JavaFX RadioButton Tutorial with Examples
- JavaFX MenuButton and SplitMenuButton Tutorial with Examples
- JavaFX TextField Tutorial with Examples
- JavaFX PasswordField Tutorial with Examples
- JavaFX TextArea Tutorial with Examples
- JavaFX Slider Tutorial with Examples
- JavaFX Spinner Tutorial with Examples
- JavaFX ProgressBar and ProgressIndicator Tutorial with Examples
- JavaFX ChoiceBox Tutorial with Examples
- JavaFX Tooltip Tutorial with Examples
- JavaFX DatePicker Tutorial with Examples
- JavaFX ColorPicker Tutorial with Examples
- JavaFX FileChooser and DirectoryChooser Tutorial with Examples
- JavaFX PieChart Tutorial with Examples
- JavaFX AreaChart and StackedAreaChart Tutorial with Examples
- JavaFX BarChart and StackedBarChart Tutorial with Examples
- JavaFX Line Tutorial with Examples
- JavaFX Rectangle and Ellipse Tutorial with Examples
Show More