JavaFX Hyperlink Tutorial with Examples
1. JavaFX Hyperlink
JavaFX Hyperlink represents the hyper links similar to the anchor links on the HTML.
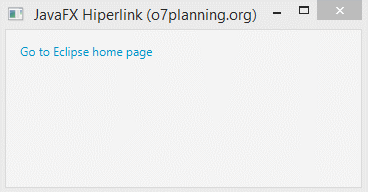
Hyperlink hyperlink = new Hyperlink("Go to Eclipse home page");
hyperlink.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
getHostServices().showDocument("https://eclipse.org");
}
});
By default JavaFX Hyperlink has 3 states is illustrated as shown below. Note that you can use CSS to change them.
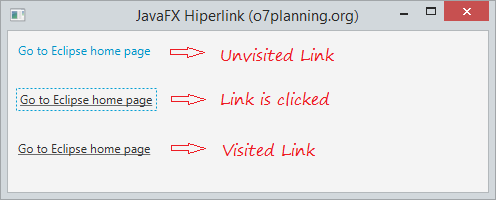
2. Hyperlink example
The following example creates a hyperlink that when you click on it, it go to the home page of Eclipse (https://eclipse.org).
HyperlinkDemo.java
package org.o7planning.javafx.hyperlink;
import javafx.application.Application;
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
import javafx.geometry.Insets;
import javafx.scene.Scene;
import javafx.scene.control.Hyperlink;
import javafx.scene.layout.FlowPane;
import javafx.stage.Stage;
public class HyperlinkDemo extends Application {
@Override
public void start(Stage stage) {
Hyperlink hyperlink = new Hyperlink("Go to Eclipse home page");
hyperlink.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
getHostServices().showDocument("https://eclipse.org");
}
});
FlowPane root = new FlowPane();
root.setPadding(new Insets(10));
root.getChildren().addAll(hyperlink);
Scene scene = new Scene(root);
stage.setTitle("JavaFX Hiperlink (o7planning.org)");
stage.setWidth(400);
stage.setHeight(200);
stage.setScene(scene);
stage.show();
}
public static void main(String[] args) {
launch(args);
}
}
3. Custom Hyperlink
With Hyperlink you have a few useful methods:
public final void setUnderline(boolean value)
public final boolean isUnderline()
public final void setVisited(boolean value)
public final boolean isVisited()
Example:
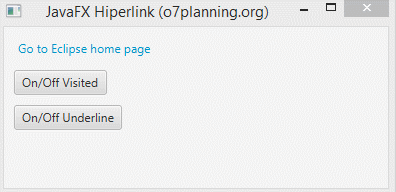
HyperlinkDemo2.java
package org.o7planning.javafx.hyperlink;
import javafx.application.Application;
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
import javafx.geometry.Insets;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.Hyperlink;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class HyperlinkDemo2 extends Application {
private final String TEXT = "Go to Eclipse home page";
@Override
public void start(Stage stage) {
Hyperlink hyperlink = new Hyperlink(TEXT);
hyperlink.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
getHostServices().showDocument("https://eclipse.org");
}
});
Button button1 = new Button("On/Off Visited");
button1.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
boolean visited = hyperlink.isVisited();
hyperlink.setVisited(!visited);
hyperlink.setText(TEXT+" (visited:"+ hyperlink.isVisible()+")");
}
});
Button button2 = new Button("On/Off Underline");
button2.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
boolean underline = hyperlink.isUnderline();
hyperlink.setUnderline(!underline);
hyperlink.setText(TEXT+" (underline:"+ hyperlink.isUnderline()+")");
}
});
VBox root = new VBox();
root.setPadding(new Insets(10));
root.setSpacing(10);
root.getChildren().addAll(hyperlink,button1,button2);
Scene scene = new Scene(root);
stage.setTitle("JavaFX Hiperlink (o7planning.org)");
stage.setWidth(400);
stage.setHeight(200);
stage.setScene(scene);
stage.show();
}
public static void main(String[] args) {
launch(args);
}
}
JavaFX Tutorials
- Install e(fx)clipse for Eclipse (JavaFX Tooling)
- Install JavaFX Scene Builder for Eclipse
- JavaFX Tutorial for Beginners - Hello JavaFX
- JavaFX FlowPane Layout Tutorial with Examples
- JavaFX TilePane Layout Tutorial with Examples
- JavaFX HBox, VBox Layout Tutorial with Examples
- JavaFX BorderPane Layout Tutorial with Examples
- JavaFX AnchorPane Layout Tutorial with Examples
- JavaFX GridPane Layout Tutorial with Examples
- JavaFX StackPane Layout Tutorial with Examples
- JavaFX TitledPane Tutorial with Examples
- JavaFX ScrollPane Tutorial with Examples
- JavaFX Accordion Tutorial with Examples
- JavaFX ListView Tutorial with Examples
- JavaFX Group Tutorial with Examples
- JavaFX ComboBox Tutorial with Examples
- JavaFX WebView and WebEngine Tutorial with Examples
- JavaFX HTMLEditor Tutorial with Examples
- JavaFX TableView Tutorial with Examples
- JavaFX TreeView Tutorial with Examples
- JavaFX TreeTableView Tutorial with Examples
- JavaFX Menu Tutorial with Examples
- JavaFX ContextMenu Tutorial with Examples
- JavaFX Image and ImageView Tutorial with Examples
- JavaFX Label Tutorial with Examples
- JavaFX Hyperlink Tutorial with Examples
- JavaFX Button Tutorial with Examples
- JavaFX ToggleButton Tutorial with Examples
- JavaFX RadioButton Tutorial with Examples
- JavaFX MenuButton and SplitMenuButton Tutorial with Examples
- JavaFX TextField Tutorial with Examples
- JavaFX PasswordField Tutorial with Examples
- JavaFX TextArea Tutorial with Examples
- JavaFX Slider Tutorial with Examples
- JavaFX Spinner Tutorial with Examples
- JavaFX ProgressBar and ProgressIndicator Tutorial with Examples
- JavaFX ChoiceBox Tutorial with Examples
- JavaFX Tooltip Tutorial with Examples
- JavaFX DatePicker Tutorial with Examples
- JavaFX ColorPicker Tutorial with Examples
- JavaFX FileChooser and DirectoryChooser Tutorial with Examples
- Open a new Window in JavaFX
- JavaFX Alert Dialogs Tutorial with Examples
- JavaFX TextInputDialog Tutorial with Examples
- JavaFX ChoiceDialog Tutorial with Examples
- JavaFX PieChart Tutorial with Examples
- JavaFX AreaChart and StackedAreaChart Tutorial with Examples
- JavaFX BarChart and StackedBarChart Tutorial with Examples
- JavaFX Line Tutorial with Examples
- JavaFX Rectangle and Ellipse Tutorial with Examples
- JavaFX Effects Tutorial with Examples
- JavaFX Transformations Tutorial with Examples
Show More