JavaFX HTMLEditor Tutorial with Examples
1. JavaFX HTMLEditor
The JavaFX HTMLEditor control is a full functional rich text editor. Its implementation is based on the document editing feature of HTML5 and includes the following editing functions:
- Text formatting including bold, italic, underline, and strike though styles
- Paragraph settings such as format, font family, and font size
- Foreground and background colors
- Text indent
- Bulleted and numbered lists
- Text alignment
- Adding a horizontal rule
- Copying and pasting text fragments
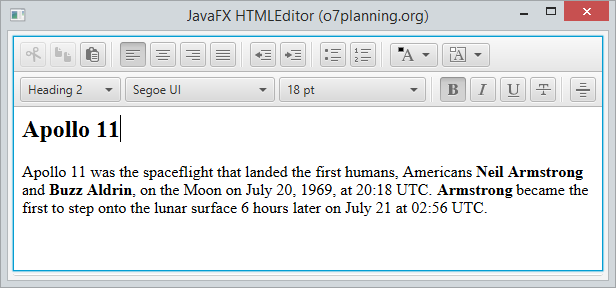
// Create HTMLEditor
HTMLEditor htmlEditor = new HTMLEditor();
htmlEditor.setPrefHeight(245);
String INITIAL_TEXT = "<h2>Apollo 11</h2>" //
+ "Apollo 11 was the spaceflight that landed the first humans,"//
+ " Americans <a href='http://en.wikipedia.org/wiki/Neil_Armstrong'>Neil Armstrong</a>"
+ " and <a href='http://en.wikipedia.org/wiki/Buzz_Aldrin'>Buzz Aldrin</a>,"//
+ " on the Moon on July 20, 1969, at 20:18 UTC."//
+ " <b>Armstrong</b> became the first to step onto"//
+ " the lunar surface 6 hours later on July 21 at 02:56 UTC.";
// Set HTML
htmlEditor.setHtmlText(INITIAL_TEXT);
// Get HTML
String html = htmlEditor.getHtmlText();
2. HTMLEditor Example
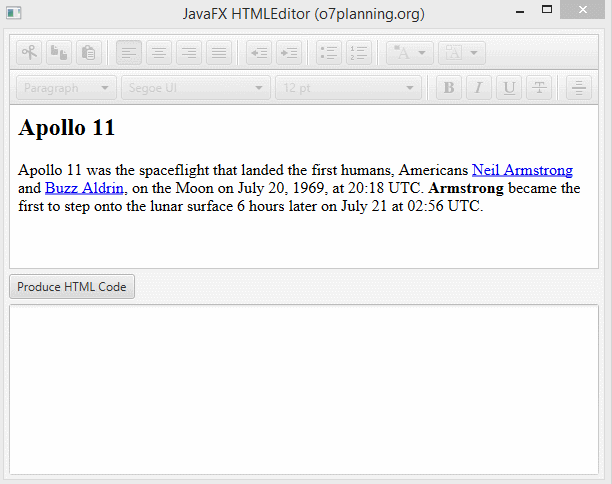
HTMLEditorDemo.java
package org.o7planning.javafx.htmleditor;
import javafx.application.Application;
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
import javafx.geometry.Insets;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.TextArea;
import javafx.scene.layout.VBox;
import javafx.scene.web.HTMLEditor;
import javafx.stage.Stage;
public class HTMLEditorDemo extends Application {
@Override
public void start(Stage stage) {
HTMLEditor htmlEditor = new HTMLEditor();
htmlEditor.setPrefHeight(245);
String INITIAL_TEXT = "<h2>Apollo 11</h2>" //
+ "Apollo 11 was the spaceflight that landed the first humans,"//
+ " Americans <a href='http://en.wikipedia.org/wiki/Neil_Armstrong'>Neil Armstrong</a>"
+ " and <a href='http://en.wikipedia.org/wiki/Buzz_Aldrin'>Buzz Aldrin</a>,"//
+ " on the Moon on July 20, 1969, at 20:18 UTC."//
+ " <b>Armstrong</b> became the first to step onto"//
+ " the lunar surface 6 hours later on July 21 at 02:56 UTC.";
htmlEditor.setHtmlText(INITIAL_TEXT);
Button showHTMLButton = new Button("Produce HTML Code");
TextArea textArea = new TextArea();
textArea.setWrapText(true);
//
showHTMLButton.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
textArea.setText(htmlEditor.getHtmlText());
}
});
VBox root = new VBox();
root.setPadding(new Insets(5));
root.setSpacing(5);
root.getChildren().addAll(htmlEditor, showHTMLButton, textArea);
Scene scene = new Scene(root, 600, 450);
stage.setTitle("JavaFX HTMLEditor (o7planning.org)");
stage.setScene(scene);
stage.show();
}
public static void main(String[] args) {
launch(args);
}
}
3. HTMLEditor and WebView
HTMLEditor is an HTML editor while WebView is a mini-browser. You can modify HTML content on HTMLEditor and display it on WebView. Let's see illustrative example below:
See more WebView:
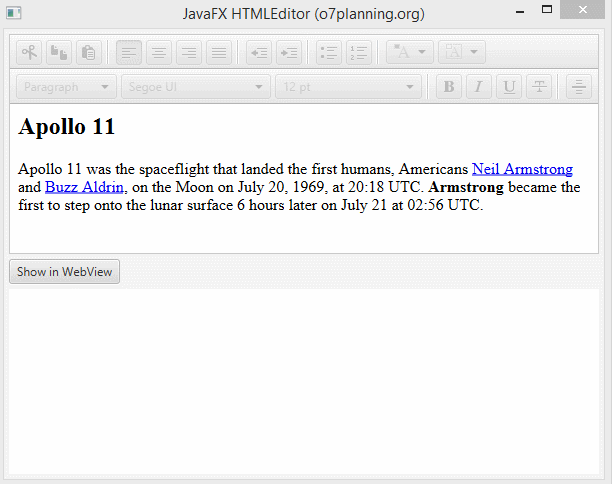
HTMLEditorWebViewDemo.java
package org.o7planning.javafx.htmleditor;
import javafx.application.Application;
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
import javafx.geometry.Insets;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.VBox;
import javafx.scene.web.HTMLEditor;
import javafx.scene.web.WebEngine;
import javafx.scene.web.WebView;
import javafx.stage.Stage;
public class HTMLEditorWebViewDemo extends Application {
@Override
public void start(Stage stage) {
// HTML Editor.
HTMLEditor htmlEditor = new HTMLEditor();
htmlEditor.setPrefHeight(245);
htmlEditor.setMinHeight(220);
String INITIAL_TEXT = "<h2>Apollo 11</h2>" //
+ "Apollo 11 was the spaceflight that landed the first humans,"//
+ " Americans <a href='http://en.wikipedia.org/wiki/Neil_Armstrong'>Neil Armstrong</a>"
+ " and <a href='http://en.wikipedia.org/wiki/Buzz_Aldrin'>Buzz Aldrin</a>,"//
+ " on the Moon on July 20, 1969, at 20:18 UTC."//
+ " <b>Armstrong</b> became the first to step onto"//
+ " the lunar surface 6 hours later on July 21 at 02:56 UTC.";
htmlEditor.setHtmlText(INITIAL_TEXT);
Button showHTMLButton = new Button("Show in WebView");
// WebView
WebView webView = new WebView();
WebEngine webEngine = webView.getEngine();
//
showHTMLButton.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
webEngine.loadContent(htmlEditor.getHtmlText(), "text/html");
}
});
VBox root = new VBox();
root.setPadding(new Insets(5));
root.setSpacing(5);
root.getChildren().addAll(htmlEditor, showHTMLButton, webView);
Scene scene = new Scene(root, 600, 450);
stage.setTitle("JavaFX HTMLEditor (o7planning.org)");
stage.setScene(scene);
stage.show();
}
public static void main(String[] args) {
launch(args);
}
}
JavaFX Tutorials
- Open a new Window in JavaFX
- JavaFX ChoiceDialog Tutorial with Examples
- JavaFX Alert Dialogs Tutorial with Examples
- JavaFX TextInputDialog Tutorial with Examples
- Install e(fx)clipse for Eclipse (JavaFX Tooling)
- Install JavaFX Scene Builder for Eclipse
- JavaFX Tutorial for Beginners - Hello JavaFX
- JavaFX FlowPane Layout Tutorial with Examples
- JavaFX TilePane Layout Tutorial with Examples
- JavaFX HBox, VBox Layout Tutorial with Examples
- JavaFX BorderPane Layout Tutorial with Examples
- JavaFX AnchorPane Layout Tutorial with Examples
- JavaFX TitledPane Tutorial with Examples
- JavaFX Accordion Tutorial with Examples
- JavaFX ListView Tutorial with Examples
- JavaFX Group Tutorial with Examples
- JavaFX ComboBox Tutorial with Examples
- JavaFX Transformations Tutorial with Examples
- JavaFX Effects Tutorial with Examples
- JavaFX GridPane Layout Tutorial with Examples
- JavaFX StackPane Layout Tutorial with Examples
- JavaFX ScrollPane Tutorial with Examples
- JavaFX WebView and WebEngine Tutorial with Examples
- JavaFX HTMLEditor Tutorial with Examples
- JavaFX TableView Tutorial with Examples
- JavaFX TreeView Tutorial with Examples
- JavaFX TreeTableView Tutorial with Examples
- JavaFX Menu Tutorial with Examples
- JavaFX ContextMenu Tutorial with Examples
- JavaFX Image and ImageView Tutorial with Examples
- JavaFX Label Tutorial with Examples
- JavaFX Hyperlink Tutorial with Examples
- JavaFX Button Tutorial with Examples
- JavaFX ToggleButton Tutorial with Examples
- JavaFX RadioButton Tutorial with Examples
- JavaFX MenuButton and SplitMenuButton Tutorial with Examples
- JavaFX TextField Tutorial with Examples
- JavaFX PasswordField Tutorial with Examples
- JavaFX TextArea Tutorial with Examples
- JavaFX Slider Tutorial with Examples
- JavaFX Spinner Tutorial with Examples
- JavaFX ProgressBar and ProgressIndicator Tutorial with Examples
- JavaFX ChoiceBox Tutorial with Examples
- JavaFX Tooltip Tutorial with Examples
- JavaFX DatePicker Tutorial with Examples
- JavaFX ColorPicker Tutorial with Examples
- JavaFX FileChooser and DirectoryChooser Tutorial with Examples
- JavaFX PieChart Tutorial with Examples
- JavaFX AreaChart and StackedAreaChart Tutorial with Examples
- JavaFX BarChart and StackedBarChart Tutorial with Examples
- JavaFX Line Tutorial with Examples
- JavaFX Rectangle and Ellipse Tutorial with Examples
Show More