JavaFX ScrollPane Tutorial with Examples
1. JavaFx ScrollPane
ScrollPane is a scrollable component used to display a large content in a limited space. It contains horizontal and vertical sroll bars.
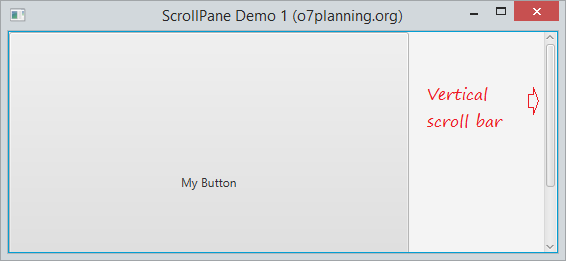
ScrollBar Policy
You can set up display policy for scroll bar:
- NEVER - Never display
- ALWAYS - Always display
- AS_NEEDED - Display if needed.
** ScrollBarPolicy **
// Setting a horizontal scroll bar is always display
scrollPane.setHbarPolicy(ScrollBarPolicy.ALWAYS);
// Setting vertical scroll bar is never displayed.
scrollPane.setVbarPolicy(ScrollBarPolicy.NEVER);
2. ScrollPane Example
ScrollPaneDemo1.java
package org.o7planning.javafx.scrollpane;
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.ScrollPane;
import javafx.scene.control.ScrollPane.ScrollBarPolicy;
import javafx.stage.Stage;
public class ScrollPaneDemo1 extends Application {
@Override
public void start(Stage primaryStage) {
// Create a ScrollPane
ScrollPane scrollPane = new ScrollPane();
Button button = new Button("My Button");
button.setPrefSize(400, 300);
// Set content for ScrollPane
scrollPane.setContent(button);
// Always show vertical scroll bar
scrollPane.setVbarPolicy(ScrollBarPolicy.ALWAYS);
// Horizontal scroll bar is only displayed when needed
scrollPane.setHbarPolicy(ScrollBarPolicy.AS_NEEDED);
primaryStage.setTitle("ScrollPane Demo 1 (o7planning.org)");
Scene scene = new Scene(scrollPane, 550, 200);
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Running the example:
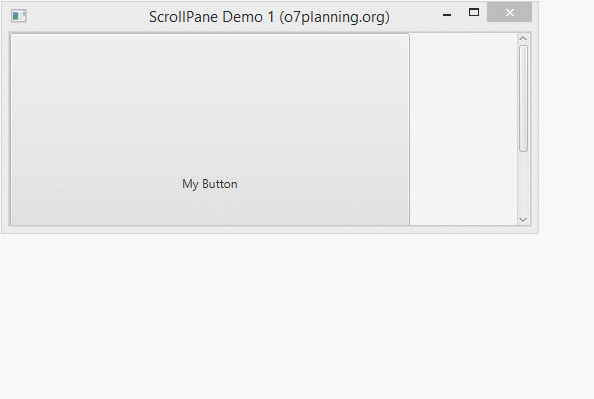
3. ScrollPane with Pannable example
ScrollPaneDemo2.java
package org.o7planning.javafx.scrollpane;
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.ScrollPane;
import javafx.scene.layout.FlowPane;
import javafx.stage.Stage;
public class ScrollPaneDemo2 extends Application {
@Override
public void start(Stage primaryStage) {
final FlowPane container = new FlowPane();
// Button 1
Button button1= new Button("Button 1");
button1.setPrefSize(350, 100);
container.getChildren().add(button1);
// Button 2
Button button2= new Button("Button 2");
button2.setPrefSize(245, 220);
container.getChildren().add(button2);
// ScrollPane
ScrollPane scrollPane = new ScrollPane();
scrollPane.setContent(container);
// Pannable.
scrollPane.setPannable(true);
primaryStage.setTitle("ScrollPane Demo 2 (o7planning.org)");
Scene scene = new Scene(scrollPane, 550, 200);
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Running the example:
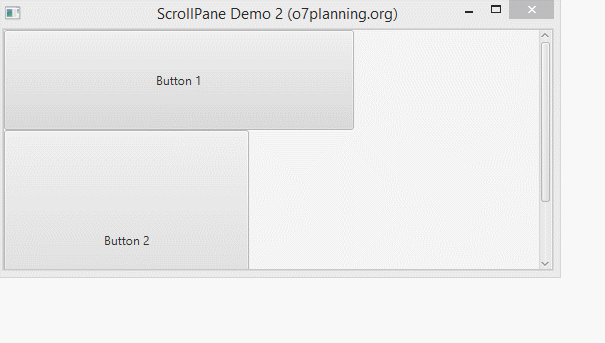
JavaFX Tutorials
- Open a new Window in JavaFX
- JavaFX ChoiceDialog Tutorial with Examples
- JavaFX Alert Dialogs Tutorial with Examples
- JavaFX TextInputDialog Tutorial with Examples
- Install e(fx)clipse for Eclipse (JavaFX Tooling)
- Install JavaFX Scene Builder for Eclipse
- JavaFX Tutorial for Beginners - Hello JavaFX
- JavaFX FlowPane Layout Tutorial with Examples
- JavaFX TilePane Layout Tutorial with Examples
- JavaFX HBox, VBox Layout Tutorial with Examples
- JavaFX BorderPane Layout Tutorial with Examples
- JavaFX AnchorPane Layout Tutorial with Examples
- JavaFX TitledPane Tutorial with Examples
- JavaFX Accordion Tutorial with Examples
- JavaFX ListView Tutorial with Examples
- JavaFX Group Tutorial with Examples
- JavaFX ComboBox Tutorial with Examples
- JavaFX Transformations Tutorial with Examples
- JavaFX Effects Tutorial with Examples
- JavaFX GridPane Layout Tutorial with Examples
- JavaFX StackPane Layout Tutorial with Examples
- JavaFX ScrollPane Tutorial with Examples
- JavaFX WebView and WebEngine Tutorial with Examples
- JavaFX HTMLEditor Tutorial with Examples
- JavaFX TableView Tutorial with Examples
- JavaFX TreeView Tutorial with Examples
- JavaFX TreeTableView Tutorial with Examples
- JavaFX Menu Tutorial with Examples
- JavaFX ContextMenu Tutorial with Examples
- JavaFX Image and ImageView Tutorial with Examples
- JavaFX Label Tutorial with Examples
- JavaFX Hyperlink Tutorial with Examples
- JavaFX Button Tutorial with Examples
- JavaFX ToggleButton Tutorial with Examples
- JavaFX RadioButton Tutorial with Examples
- JavaFX MenuButton and SplitMenuButton Tutorial with Examples
- JavaFX TextField Tutorial with Examples
- JavaFX PasswordField Tutorial with Examples
- JavaFX TextArea Tutorial with Examples
- JavaFX Slider Tutorial with Examples
- JavaFX Spinner Tutorial with Examples
- JavaFX ProgressBar and ProgressIndicator Tutorial with Examples
- JavaFX ChoiceBox Tutorial with Examples
- JavaFX Tooltip Tutorial with Examples
- JavaFX DatePicker Tutorial with Examples
- JavaFX ColorPicker Tutorial with Examples
- JavaFX FileChooser and DirectoryChooser Tutorial with Examples
- JavaFX PieChart Tutorial with Examples
- JavaFX AreaChart and StackedAreaChart Tutorial with Examples
- JavaFX BarChart and StackedBarChart Tutorial with Examples
- JavaFX Line Tutorial with Examples
- JavaFX Rectangle and Ellipse Tutorial with Examples
Show More