JavaFX Image and ImageView Tutorial with Examples
1. JavaFX Image
JavaFX allows you to work with all popular image formats. Let's use class javafx.scene.image.Image to load images from hard drive or a network image sources. In order to display images on JavaFX, you use ImageView class.
The Constructors of class Image help you to load image data:
** Image **
Image(InputStream inputStream)
Image(InputStream is, double requestedWidth, double requestedHeight,
boolean preserveRatio, boolean smooth)
Image(String url)
Image(String url, boolean backgroundLoading)
Image(String url, double requestedWidth, double requestedHeight,
boolean preserveRatio, boolean smooth)
Image(String url, double requestedWidth, double requestedHeight,
boolean preserveRatio, boolean smooth, boolean backgroundLoading)
Load images from URL:
String url = "http://somedomain/images/image.png";
boolean backgroundLoading = true;
// The image is being loaded in the background
Image image = new Image(url, backgroundLoading);
The most common is that you load an image on the hard drive.
// An image file on the hard drive.
File file = new File("C:/MyImages/myphoto.jpg");
// --> file:/C:/MyImages/myphoto.jpg
String localUrl = file.toURI().toURL().toString();
Image image = new Image(localUrl);
The images can be placed in a jar file or in a package of your Project. And you can use Image(InputStream) constructorto load it.
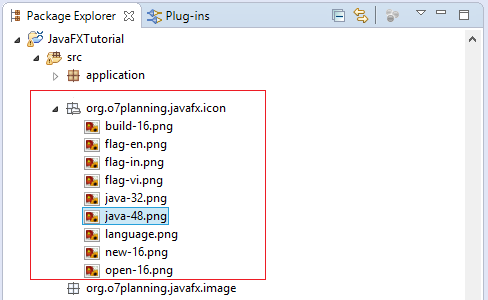
// MyClass is located in the same project with images,
// or in the same jar file with images.
Class<?> clazz = MyClass.class;
InputStream input = clazz.getResourceAsStream("/org/o7planning/javafx/icon/java-32.png");
Image image = new Image(input);
2. JavaFX ImageView
ImageView is a component which helps you to display images on JavaFX. You can also apply effects to display images such as rotate, zoom in and out,...
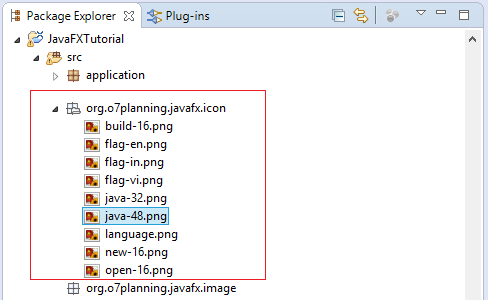
ImageViewDemo.java
package org.o7planning.javafx.image;
import java.io.InputStream;
import javafx.application.Application;
import javafx.geometry.Insets;
import javafx.scene.Scene;
import javafx.scene.image.Image;
import javafx.scene.image.ImageView;
import javafx.scene.layout.FlowPane;
import javafx.stage.Stage;
public class ImageViewDemo extends Application {
@Override
public void start(Stage primaryStage) throws Exception {
Class<?> clazz = this.getClass();
InputStream input = clazz.getResourceAsStream("/org/o7planning/javafx/icon/java-48.png");
Image image = new Image(input);
ImageView imageView = new ImageView(image);
InputStream input2 = clazz.getResourceAsStream("/org/o7planning/javafx/icon/java-48.png");
Image image2 = new Image(input2, 100, 200, false, true);
ImageView imageView2 = new ImageView(image2);
FlowPane root = new FlowPane();
root.setPadding(new Insets(20));
root.getChildren().addAll(imageView, imageView2);
Scene scene = new Scene(root, 400, 200);
primaryStage.setTitle("JavaFX ImageView (o7planning.org)");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
Application.launch(args);
}
}
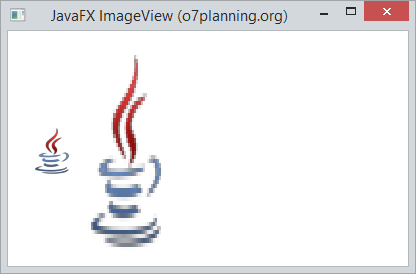
3. Rotate and Scale
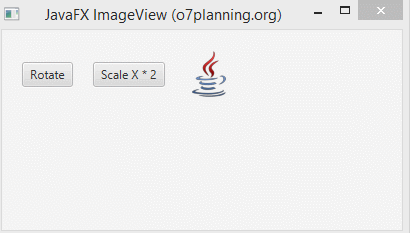
ImageViewDemo2.java
package org.o7planning.javafx.image;
import java.io.InputStream;
import javafx.application.Application;
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
import javafx.geometry.Insets;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.image.Image;
import javafx.scene.image.ImageView;
import javafx.scene.layout.FlowPane;
import javafx.stage.Stage;
public class ImageViewDemo2 extends Application {
@Override
public void start(Stage primaryStage) throws Exception {
Class<?> clazz = this.getClass();
InputStream input = clazz.getResourceAsStream("/org/o7planning/javafx/icon/java-48.png");
Image image = new Image(input);
ImageView imageView = new ImageView(image);
Button buttonRotate = new Button("Rotate");
buttonRotate.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
double value = imageView.getRotate();
imageView.setRotate(value + 30);
}
});
Button buttonScale = new Button("Scale X * 2");
buttonScale.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
imageView.setScaleX(2); ;
}
});
FlowPane root = new FlowPane();
root.setPadding(new Insets(20));
root.setHgap(20);
root.getChildren().addAll(buttonRotate,buttonScale, imageView);
Scene scene = new Scene(root, 400, 200);
primaryStage.setTitle("JavaFX ImageView (o7planning.org)");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
Application.launch(args);
}
}
JavaFX Tutorials
- Open a new Window in JavaFX
- JavaFX ChoiceDialog Tutorial with Examples
- JavaFX Alert Dialogs Tutorial with Examples
- JavaFX TextInputDialog Tutorial with Examples
- Install e(fx)clipse for Eclipse (JavaFX Tooling)
- Install JavaFX Scene Builder for Eclipse
- JavaFX Tutorial for Beginners - Hello JavaFX
- JavaFX FlowPane Layout Tutorial with Examples
- JavaFX TilePane Layout Tutorial with Examples
- JavaFX HBox, VBox Layout Tutorial with Examples
- JavaFX BorderPane Layout Tutorial with Examples
- JavaFX AnchorPane Layout Tutorial with Examples
- JavaFX TitledPane Tutorial with Examples
- JavaFX Accordion Tutorial with Examples
- JavaFX ListView Tutorial with Examples
- JavaFX Group Tutorial with Examples
- JavaFX ComboBox Tutorial with Examples
- JavaFX Transformations Tutorial with Examples
- JavaFX Effects Tutorial with Examples
- JavaFX GridPane Layout Tutorial with Examples
- JavaFX StackPane Layout Tutorial with Examples
- JavaFX ScrollPane Tutorial with Examples
- JavaFX WebView and WebEngine Tutorial with Examples
- JavaFX HTMLEditor Tutorial with Examples
- JavaFX TableView Tutorial with Examples
- JavaFX TreeView Tutorial with Examples
- JavaFX TreeTableView Tutorial with Examples
- JavaFX Menu Tutorial with Examples
- JavaFX ContextMenu Tutorial with Examples
- JavaFX Image and ImageView Tutorial with Examples
- JavaFX Label Tutorial with Examples
- JavaFX Hyperlink Tutorial with Examples
- JavaFX Button Tutorial with Examples
- JavaFX ToggleButton Tutorial with Examples
- JavaFX RadioButton Tutorial with Examples
- JavaFX MenuButton and SplitMenuButton Tutorial with Examples
- JavaFX TextField Tutorial with Examples
- JavaFX PasswordField Tutorial with Examples
- JavaFX TextArea Tutorial with Examples
- JavaFX Slider Tutorial with Examples
- JavaFX Spinner Tutorial with Examples
- JavaFX ProgressBar and ProgressIndicator Tutorial with Examples
- JavaFX ChoiceBox Tutorial with Examples
- JavaFX Tooltip Tutorial with Examples
- JavaFX DatePicker Tutorial with Examples
- JavaFX ColorPicker Tutorial with Examples
- JavaFX FileChooser and DirectoryChooser Tutorial with Examples
- JavaFX PieChart Tutorial with Examples
- JavaFX AreaChart and StackedAreaChart Tutorial with Examples
- JavaFX BarChart and StackedBarChart Tutorial with Examples
- JavaFX Line Tutorial with Examples
- JavaFX Rectangle and Ellipse Tutorial with Examples
Show More