JavaFX TextField Tutorial with Examples
1. JavaFX TextField
The TextField class implements a UI control that accepts and displays text input. It provides capabilities to receive text input from a user. Along with another text input control, PasswordField, this class extends the TextInput class.
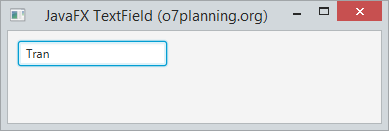
Review some helpful methods that you can use with text fields.
- clear() - Clear the text of TextField.
- copy() - transfers the currently selected range in the text to the clipboard, leaving the current selection.
- cut() - transfers the currently selected range in the text to the clipboard, removing the current selection.
- paste() - transfers the contents in the clipboard into this text, replacing the current selection.
2. TextField example
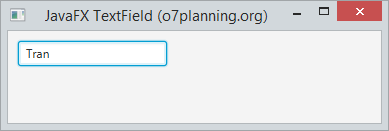
TextFieldDemo.java
package org.o7planning.javafx.textfield;
import javafx.application.Application;
import javafx.geometry.Insets;
import javafx.scene.Scene;
import javafx.scene.control.TextField;
import javafx.scene.layout.FlowPane;
import javafx.stage.Stage;
public class TextFieldDemo extends Application {
@Override
public void start(Stage primaryStage) throws Exception {
TextField textField = new TextField("Tran");
textField.setMinWidth(120);
FlowPane root = new FlowPane();
root.setPadding(new Insets(10));
root.getChildren().add(textField);
Scene scene = new Scene(root, 200, 100);
primaryStage.setTitle("JavaFX TextField (o7planning.org)");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
Application.launch(args);
}
}
3. Useful methods
The example below illustrates the usage of methods such as clear(), copy(), paste(), cut(), which are usefull methods of TextField.
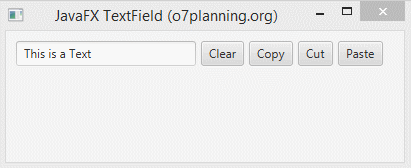
TextFieldDemo2.java
package org.o7planning.javafx.textfield;
import javafx.application.Application;
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
import javafx.geometry.Insets;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.TextField;
import javafx.scene.layout.FlowPane;
import javafx.stage.Stage;
public class TextFieldDemo2 extends Application {
@Override
public void start(Stage primaryStage) throws Exception {
TextField textField = new TextField("This is a Text");
textField.setMinWidth(180);
// Clear
Button buttonClear = new Button("Clear");
buttonClear.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
textField.clear();
}
});
// Copy
Button buttonCopy = new Button("Copy");
// Click this button without losing focus of the other component
buttonCopy.setFocusTraversable(false);
buttonCopy.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
textField.copy();
}
});
// Cut
Button buttonCut = new Button("Cut");
// Click this button without losing focus of the other component
buttonCut.setFocusTraversable(false);
buttonCut.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
textField.cut();
}
});
// Paste
Button buttonPaste = new Button("Paste");
buttonPaste.setFocusTraversable(false);
buttonPaste.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
textField.paste();
}
});
FlowPane root = new FlowPane();
root.setPadding(new Insets(10));
root.setVgap(5);
root.setHgap(5);
root.getChildren().addAll(textField, buttonClear,
buttonCopy, buttonCut, buttonPaste);
Scene scene = new Scene(root, 200, 100);
primaryStage.setTitle("JavaFX TextField (o7planning.org)");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
Application.launch(args);
}
}
JavaFX Tutorials
- Open a new Window in JavaFX
- JavaFX ChoiceDialog Tutorial with Examples
- JavaFX Alert Dialogs Tutorial with Examples
- JavaFX TextInputDialog Tutorial with Examples
- Install e(fx)clipse for Eclipse (JavaFX Tooling)
- Install JavaFX Scene Builder for Eclipse
- JavaFX Tutorial for Beginners - Hello JavaFX
- JavaFX FlowPane Layout Tutorial with Examples
- JavaFX TilePane Layout Tutorial with Examples
- JavaFX HBox, VBox Layout Tutorial with Examples
- JavaFX BorderPane Layout Tutorial with Examples
- JavaFX AnchorPane Layout Tutorial with Examples
- JavaFX TitledPane Tutorial with Examples
- JavaFX Accordion Tutorial with Examples
- JavaFX ListView Tutorial with Examples
- JavaFX Group Tutorial with Examples
- JavaFX ComboBox Tutorial with Examples
- JavaFX Transformations Tutorial with Examples
- JavaFX Effects Tutorial with Examples
- JavaFX GridPane Layout Tutorial with Examples
- JavaFX StackPane Layout Tutorial with Examples
- JavaFX ScrollPane Tutorial with Examples
- JavaFX WebView and WebEngine Tutorial with Examples
- JavaFX HTMLEditor Tutorial with Examples
- JavaFX TableView Tutorial with Examples
- JavaFX TreeView Tutorial with Examples
- JavaFX TreeTableView Tutorial with Examples
- JavaFX Menu Tutorial with Examples
- JavaFX ContextMenu Tutorial with Examples
- JavaFX Image and ImageView Tutorial with Examples
- JavaFX Label Tutorial with Examples
- JavaFX Hyperlink Tutorial with Examples
- JavaFX Button Tutorial with Examples
- JavaFX ToggleButton Tutorial with Examples
- JavaFX RadioButton Tutorial with Examples
- JavaFX MenuButton and SplitMenuButton Tutorial with Examples
- JavaFX TextField Tutorial with Examples
- JavaFX PasswordField Tutorial with Examples
- JavaFX TextArea Tutorial with Examples
- JavaFX Slider Tutorial with Examples
- JavaFX Spinner Tutorial with Examples
- JavaFX ProgressBar and ProgressIndicator Tutorial with Examples
- JavaFX ChoiceBox Tutorial with Examples
- JavaFX Tooltip Tutorial with Examples
- JavaFX DatePicker Tutorial with Examples
- JavaFX ColorPicker Tutorial with Examples
- JavaFX FileChooser and DirectoryChooser Tutorial with Examples
- JavaFX PieChart Tutorial with Examples
- JavaFX AreaChart and StackedAreaChart Tutorial with Examples
- JavaFX BarChart and StackedBarChart Tutorial with Examples
- JavaFX Line Tutorial with Examples
- JavaFX Rectangle and Ellipse Tutorial with Examples
Show More