JDBC Driver Libraries for different types of database in Java
1. Introduction
This document instructs you to download and using the JDBC library driver of some database. Here I instruct on types of database:
- Oracle
- MySQL
- SQL Server.
2. JDBC Driver for Oracle
Oracle Database library Driver is typically named ojdbc14.jar, ojdbc6.jar, ... The difference is the Java version packaged it.
- ojdbc14.jar: compiled and packaged by the Java version 1.4
- ojdbc6.jar: compiled and packaged by the Java version 1.6
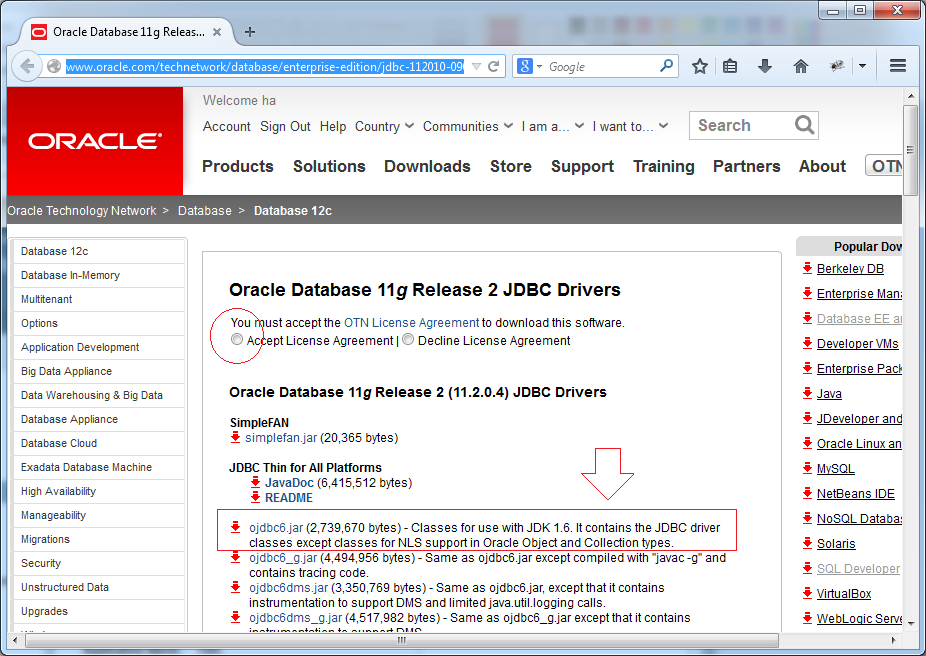
You can download ojdbc6.jar file, it can using for Oracle database of different versions (XE, 10g, 11g, 12). But almost all current Java applications use Java version 6 or newer.
To download at website of Oracle, you must have an Oracle account (Free registration).
For saving time, you can download at the following link:
To download at website of Oracle, you must have an Oracle account (Free registration).
For saving time, you can download at the following link:
Results downloaded:
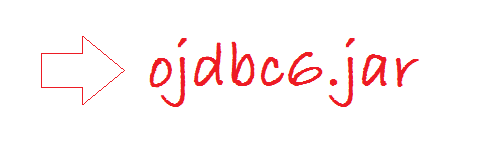
Maven for Oracle JDBC Driver
<repositories>
<!-- Repository for ORACLE ojdbc6. -->
<repository>
<id>codelds</id>
<url>https://code.lds.org/nexus/content/groups/main-repo</url>
</repository>
</repositories>
.......
<dependencies>
......
<!-- Oracle database driver -->
<dependency>
<groupId>com.oracle</groupId>
<artifactId>ojdbc6</artifactId>
<version>11.2.0.3</version>
</dependency>
.......
</dependencies>
How to use (ojdbc)
// Driver class:
oracle.jdbc.driver.OracleDriver
// URL Connection String: (SID)
String urlString ="jdbc:oracle:thin:@myhost:1521:mysid"
// URL Connection String: (Service Name)
String urlString ="jdbc:oracle:thin:username/pass@//myhost:1521/myservicename"
// Or:
String urlString ="jdbc:oracle:thin:@myhost:1521/myservicename";
For example JDBC connection to the Oracle database.
OracleConnUtils.java
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class OracleConnUtils {
public static Connection getOracleConnection()
throws ClassNotFoundException, SQLException {
String hostName = "localhost";
String sid = "db11g";
String userName = "learningsql";
String password = "1234";
return getOracleConnection(hostName, sid, userName, password);
}
public static Connection getOracleConnection(String hostName, String sid,
String userName, String password) throws ClassNotFoundException,
SQLException {
// Declare the class Driver for Oracle DB
// This is necessary with Java 5 (or older)
// Java6 (or newer) automatically find the appropriate driver.
// If you use Java> 6, then this line is not needed.
Class.forName("oracle.jdbc.driver.OracleDriver");
// Example: jdbc:oracle:thin:@localhost:1521:db11g
String connectionURL = "jdbc:oracle:thin:@" + hostName + ":1521:" + sid;
Connection conn = DriverManager.getConnection(connectionURL, userName,
password);
return conn;
}
}
3. JDBC Driver for MySQL
You can download the JDBC library for MySQL database at:
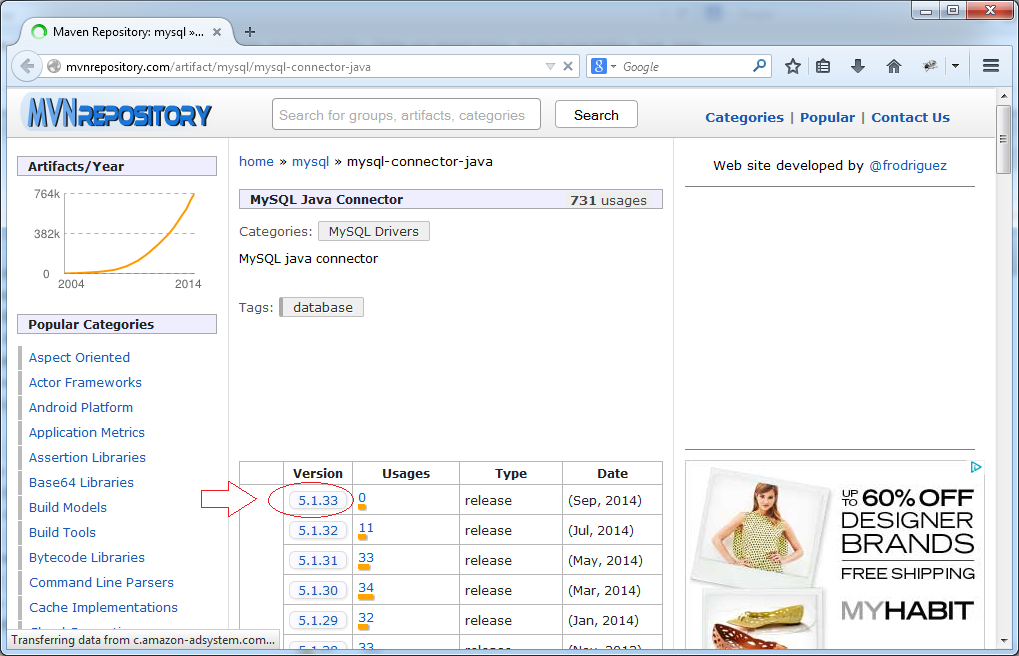
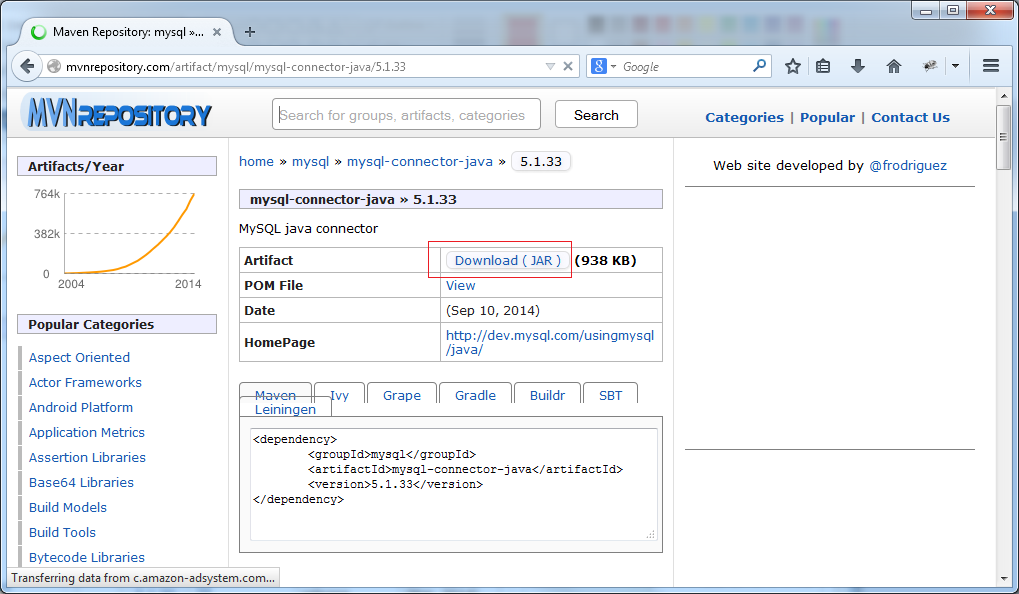
Results downloaded:

How to use
How to use: (JDBC for MySQL)
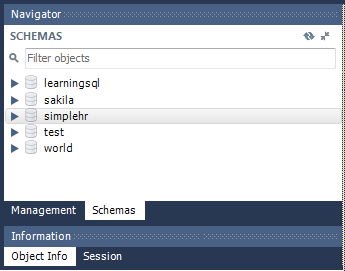
// Driver class:
com.mysql.jdbc.Driver
// URL Connection String:
String url = "jdbc:mysql://hostname:3306/dbname";
// Example:
String url = "jdbc:mysql://localhost:3306/simplehr";
For example, using JDBC to connect to the MySQL Database
MySQLConnUtils.java
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class MySQLConnUtils {
public static Connection getMySQLConnection()
throws ClassNotFoundException, SQLException {
String hostName = "localhost";
String dbName = "learningsql";
String userName = "root";
String password = "12345";
return getMySQLConnection(hostName, dbName, userName, password);
}
public static Connection getMySQLConnection(String hostName, String dbName,
String userName, String password) throws SQLException,
ClassNotFoundException {
// Declare the class Driver for Oracle DB
// This is necessary with Java 5 (or older)
// Java6 (or newer) automatically find the appropriate driver.
// If you use Java> 5, then this line is not needed.
Class.forName("com.mysql.jdbc.Driver");
// Ví dụ: jdbc:mysql://localhost:3306/simplehr
String connectionURL = "jdbc:mysql://" + hostName + ":3306/" + dbName;
Connection conn = DriverManager.getConnection(connectionURL, userName,
password);
return conn;
}
}
Some of trouble and how to fix it
In some cases, error happens with the connection of Java or another computer with MySQL. The cause you may not have configured MySQL server to allow connections from other machines.
You can review the configuration section in the document "Guide to installing and configuring MySQL Community".
4. JDBC Driver for SQLServer (JTDS)
JTDS is another JDBC library managing database SQLServer, it is a open source library.
jTDS is an open source 100% pure Java (type 4) JDBC 3.0 driver for Microsoft SQL Server (6.5, 7, 2000, 2005, 2008, 2012) and Sybase ASE (10, 11, 12, 15). jTDS is based on FreeTDS and is currently the fastest production-ready JDBC driver for SQL Server and Sybase. jTDS is 100% JDBC 3.0 compatible, supporting forward-only and scrollable/updateable ResultSets and implementing all the DatabaseMetaData and ResultSetMetaData methods.
You can download the JTDS at:
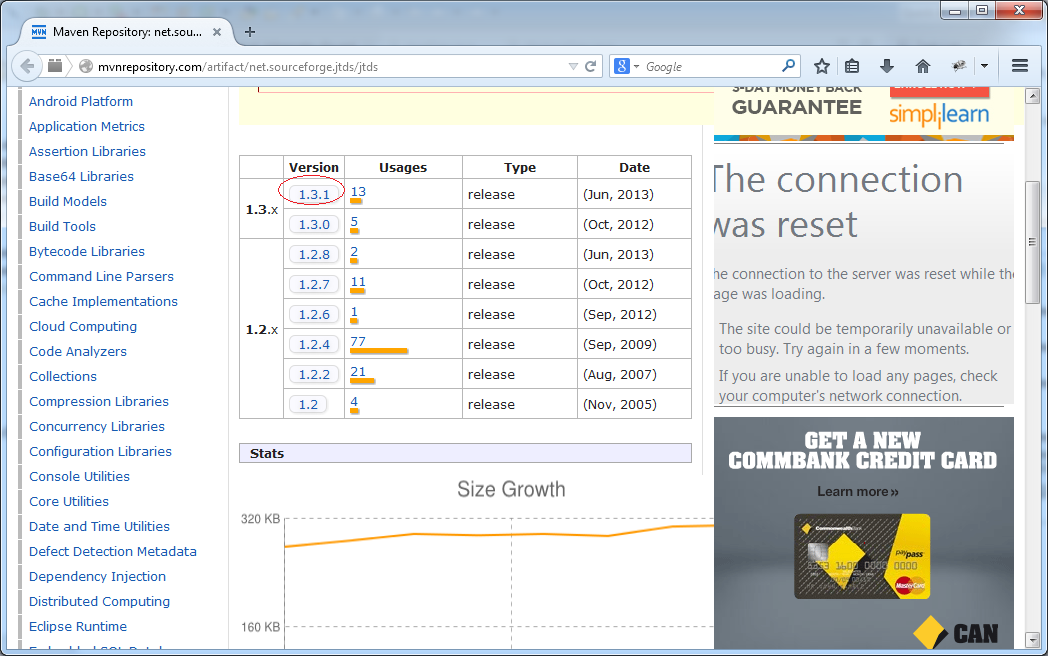
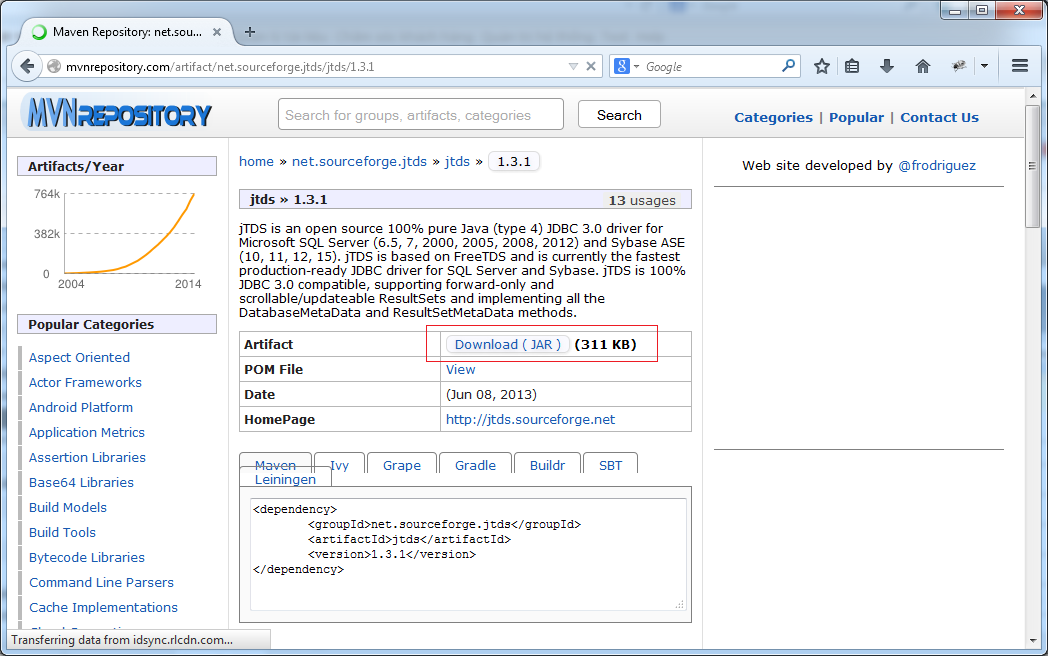
Results downloaded:
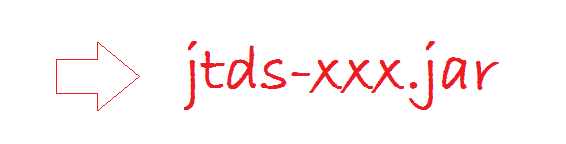
How to use (jtds)
How to use: (JDBC driver for SQL Server)
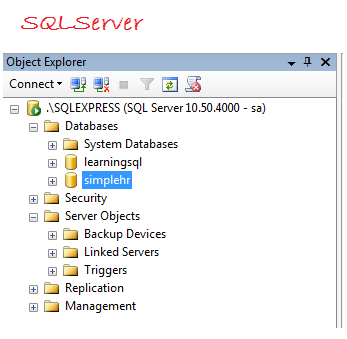
// Driver Class
net.sourceforge.jtds.jdbc.Driver
// Connection URL String:
jdbc:jtds:<server_type>://<server>[:<port>][/<database>][;<property>=<value>[;...]]
// Example 1:
String url = "jdbc:jtds:sqlserver://MYPC:1433/simplehr;instance=SQLEXPRESS;user=sa;password=s3cr3t";
getConnection(url);
// Example 2:
String url = "jdbc:jtds:sqlserver://MYPC:1433/simplehr;instance=SQLEXPRESS";
getConnection(url, "sa", "s3cr3t"):
For example, using JDBC to connect to the MySQL Database (with JTDS library)
SQLServerConnUtils_JTDS.java
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class SQLServerConnUtils_JTDS {
// Connect to SQLServer.
// (Using JTDS library)
public static Connection getSQLServerConnection_JTDS() throws SQLException,
ClassNotFoundException {
String hostName = "localhost";
String sqlInstanceName = "SQLEXPRESS";
String database = "simplehr";
String userName = "sa";
String password = "12345";
return getSQLServerConnection_JTDS(hostName, sqlInstanceName, database,
userName, password);
}
// JTDS & SQLServer.
private static Connection getSQLServerConnection_JTDS(String hostName,
String sqlInstanceName, String database, String userName,
String password) throws ClassNotFoundException, SQLException {
// Declare the class Driver for Oracle DB
// This is necessary with Java 5 (or older)
// Java6 (or newer) automatically find the appropriate driver.
// If you use Java> 5, then this line is not needed.
Class.forName("net.sourceforge.jtds.jdbc.Driver");
// Example:
// jdbc:jtds:sqlserver://localhost:1433/simplehr;instance=SQLEXPRESS
String connectionURL = "jdbc:jtds:sqlserver://" + hostName + ":1433/"
+ database + ";instance=" + sqlInstanceName;
Connection conn = DriverManager.getConnection(connectionURL, userName,
password);
return conn;
}
}
Some of trouble and how to fix it
In some situations, we connect to SQLServer and error happens:
Exception in thread "main" java.sql.SQLException: Server tran-vmware has no instance named SQLEXPRESS.
at net.sourceforge.jtds.jdbc.JtdsConnection.<init>(JtdsConnection.java:301)
at net.sourceforge.jtds.jdbc.Driver.connect(Driver.java:184)
at java.sql.DriverManager.getConnection(DriverManager.java:571)
at java.sql.DriverManager.getConnection(DriverManager.java:215)
at org.o7planning.tutorial.jdbc.ConnectionUtils.getSQLServerConnection_JTDS(ConnectionUtils.java:189)
at org.o7planning.tutorial.jdbc.ConnectionUtils.getSQLServerConnection_JTDS(ConnectionUtils.java:72)
at org.o7planning.tutorial.jdbc.ConnectionUtils.getMyConnection(ConnectionUtils.java:31)
at org.o7planning.tutorial.jdbc.TestConnection.main(TestConnection.java:20)
The above error exists because you do not Enable the TCP/IP service of SQLServer. You can reference the configuration section in the document: "Guide to installing and configuring SQLServer Express ..." at:
5. JDBC Driver for SQLServer (SQLJDBC)
SQLJDBC library is provided by Microsoft.
Download:
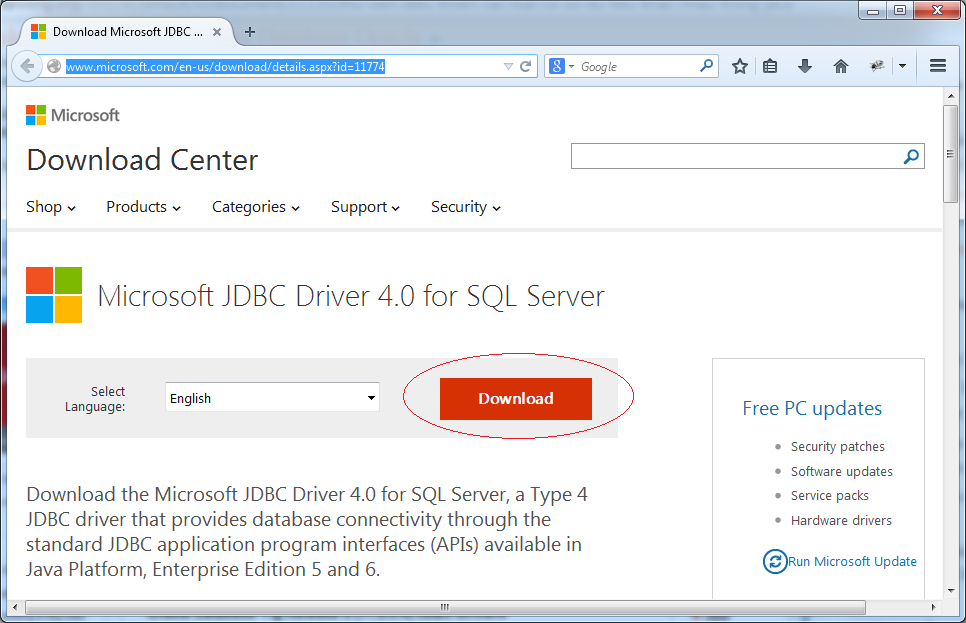
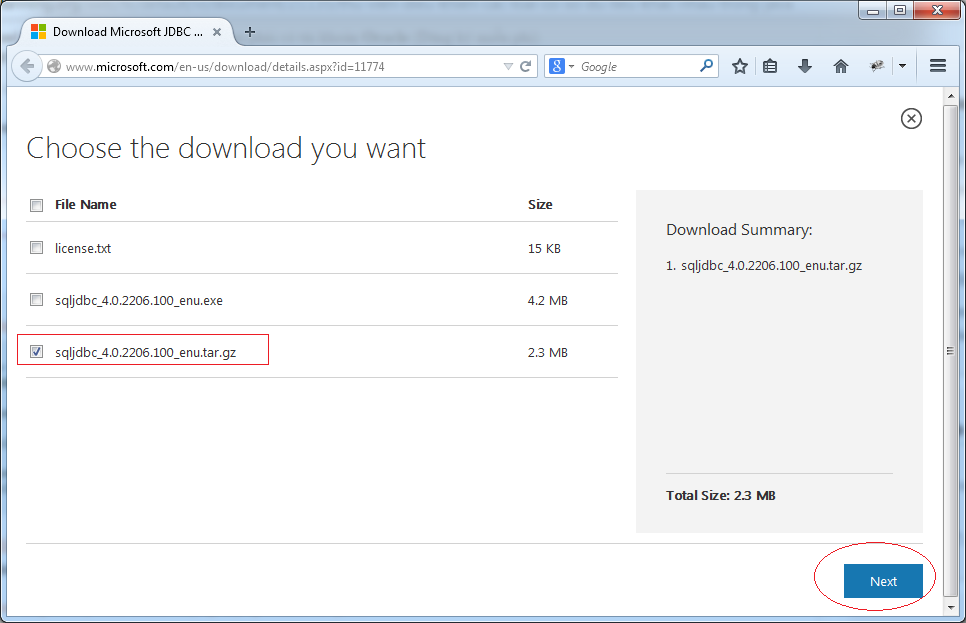
Unzip the file you just downloaded.
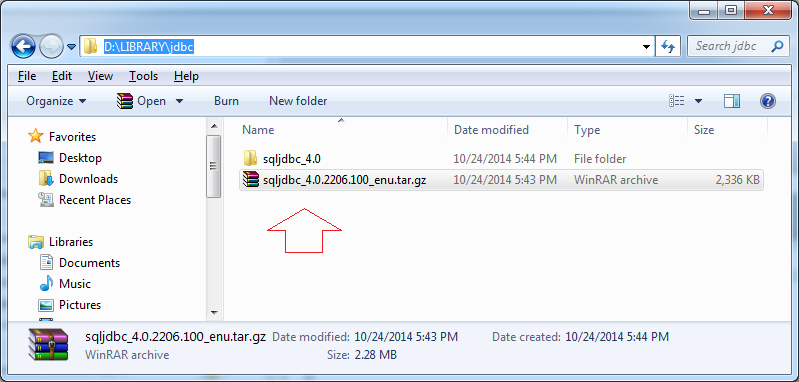
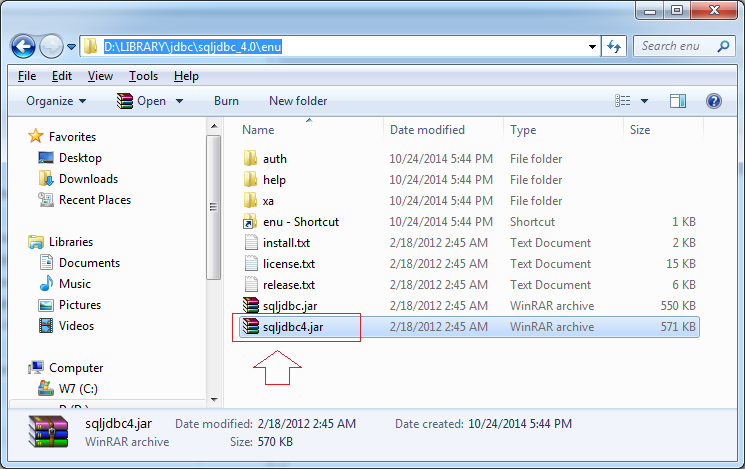
The result:
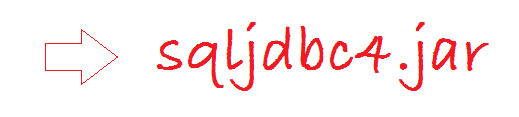
How to use (sqljdbc)
// Driver Class:
com.microsoft.sqlserver.jdbc.SQLServerDriver
Class.forName("com.microsoft.sqlserver.jdbc.SQLServerDriver");
// Url String:
String url = "jdbc:sqlserver://ServerIp;Instance=SQLEXPRESS;databaseName=simplehr";
// or
String url = "jdbc:sqlserver://ServerIp:1433;Instance=SQLEXPRESS;databaseName=simplehr";
String user = "dbUserID";
String pass = "dbUserPassword";
Connection connection = DriverManager.getConnection(url, user, pass);
For example, using JDBC to connect to the MySQL Database (with SQLJDBC library).
SQLServerConnUtils_SQLJDBC.java
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class SQLServerConnUtils_SQLJDBC {
// Connect to SQLServer.
// (Using SQLJDBC)
public static Connection getSQLServerConnection_SQLJDBC()
throws ClassNotFoundException, SQLException {
String hostName = "localhost";
String sqlInstanceName = "SQLEXPRESS";
String database = "learningsql";
String userName = "sa";
String password = "12345";
return getSQLServerConnection_SQLJDBC(hostName, sqlInstanceName,
database, userName, password);
}
// SQLServer & SQLJDBC.
private static Connection getSQLServerConnection_SQLJDBC(String hostName,
String sqlInstanceName, String database, String userName,
String password) throws ClassNotFoundException, SQLException {
// Declare the class Driver for Oracle DB
// This is necessary with Java 5 (or older)
// Java6 (or newer) automatically find the appropriate driver.
// If you use Java> 5, then this line is not needed.
Class.forName("com.microsoft.sqlserver.jdbc.SQLServerDriver");
// Example:
// jdbc:sqlserver://ServerIp:1433/SQLEXPRESS;databaseName=simplehr
String connectionURL = "jdbc:sqlserver://" + hostName + ":1433"
+ ";instance=" + sqlInstanceName + ";databaseName=" + database;
Connection conn = DriverManager.getConnection(connectionURL, userName,
password);
return conn;
}
}
Some of trouble and how to fix it
In some situations, we connect to SQLServer and error happens:
com.microsoft.sqlserver.jdbc.SQLServerException: The TCP/IP connection to the host localhost, port 1433 has failed. Error: "Connection refused: connect. Verify the connection properties. Make sure that an instance of SQL Server is running on the host and accepting TCP/IP connections at the port. Make sure that TCP connections to the port are not blocked by a firewall.".
at com.microsoft.sqlserver.jdbc.SQLServerException.makeFromDriverError(SQLServerException.java:190)
at com.microsoft.sqlserver.jdbc.SQLServerException.ConvertConnectExceptionToSQLServerException(SQLServerException.java:241)
at com.microsoft.sqlserver.jdbc.SocketFinder.findSocket(IOBuffer.java:2243)
at com.microsoft.sqlserver.jdbc.TDSChannel.open(IOBuffer.java:491)
at com.microsoft.sqlserver.jdbc.SQLServerConnection.connectHelper(SQLServerConnection.java:1309)
at com.microsoft.sqlserver.jdbc.SQLServerConnection.login(SQLServerConnection.java:991)
at com.microsoft.sqlserver.jdbc.SQLServerConnection.connect(SQLServerConnection.java:827)
at com.microsoft.sqlserver.jdbc.SQLServerDriver.connect(SQLServerDriver.java:1012)
at java.sql.DriverManager.getConnection(Unknown Source)
at java.sql.DriverManager.getConnection(Unknown Source)
...
The above error exists because you do not Enable the TCP/IP service of SQLServer. You can reference the configuration section in the document: "Guide to installing and configuring SQLServer Express ..." at:
If still errors:
Exception in thread "main" com.microsoft.sqlserver.jdbc.SQLServerException: The TCP/IP connection to the host tran-vmware, port 1433 has failed. Error: "Connection refused: connect. Verify the connection properties. Make sure that an instance of SQL Server is running on the host and accepting TCP/IP connections at the port. Make sure that TCP connections to the port are not blocked by a firewall.".
at com.microsoft.sqlserver.jdbc.SQLServerException.makeFromDriverError(SQLServerException.java:190)
at com.microsoft.sqlserver.jdbc.SQLServerException.ConvertConnectExceptionToSQLServerException(SQLServerException.java:241)
at com.microsoft.sqlserver.jdbc.SocketFinder.findSocket(IOBuffer.java:2243)
at com.microsoft.sqlserver.jdbc.TDSChannel.open(IOBuffer.java:491)
at com.microsoft.sqlserver.jdbc.SQLServerConnection.connectHelper(SQLServerConnection.java:1309)
at com.microsoft.sqlserver.jdbc.SQLServerConnection.login(SQLServerConnection.java:991)
at com.microsoft.sqlserver.jdbc.SQLServerConnection.connect(SQLServerConnection.java:827)
at com.microsoft.sqlserver.jdbc.SQLServerDriver.connect(SQLServerDriver.java:1012)
at java.sql.DriverManager.getConnection(DriverManager.java:571)
at java.sql.DriverManager.getConnection(DriverManager.java:215)
...
- TODO
- You should use JTDS
Java Basic
- Customize java compiler processing your Annotation (Annotation Processing Tool)
- Java Programming for team using Eclipse and SVN
- Java WeakReference Tutorial with Examples
- Java PhantomReference Tutorial with Examples
- Java Compression and Decompression Tutorial with Examples
- Configuring Eclipse to use the JDK instead of JRE
- Java String.format() and printf() methods
- Syntax and new features in Java 8
- Java Regular Expressions Tutorial with Examples
- Java Multithreading Programming Tutorial with Examples
- JDBC Driver Libraries for different types of database in Java
- Java JDBC Tutorial with Examples
- Get the values of the columns automatically increment when Insert a record using JDBC
- Java Stream Tutorial with Examples
- Java Functional Interface Tutorial with Examples
- Introduction to the Raspberry Pi
- Java Predicate Tutorial with Examples
- Abstract class and Interface in Java
- Access modifiers in Java
- Java Enums Tutorial with Examples
- Java Annotations Tutorial with Examples
- Comparing and Sorting in Java
- Java String, StringBuffer and StringBuilder Tutorial with Examples
- Java Exception Handling Tutorial with Examples
- Java Generics Tutorial with Examples
- Manipulating files and directories in Java
- Java BiPredicate Tutorial with Examples
- Java Consumer Tutorial with Examples
- Java BiConsumer Tutorial with Examples
- What is needed to get started with Java?
- History of Java and the difference between Oracle JDK and OpenJDK
- Install Java on Windows
- Install Java on Ubuntu
- Install OpenJDK on Ubuntu
- Install Eclipse
- Install Eclipse on Ubuntu
- Quick Learning Java for beginners
- History of bits and bytes in computer science
- Data Types in java
- Bitwise Operations
- if else statement in java
- Switch Statement in Java
- Loops in Java
- Arrays in Java
- JDK Javadoc in CHM format
- Inheritance and polymorphism in Java
- Java Function Tutorial with Examples
- Java BiFunction Tutorial with Examples
- Example of Java encoding and decoding using Apache Base64
- Java Reflection Tutorial with Examples
- Java remote method invocation - Java RMI Tutorial with Examples
- Java Socket Programming Tutorial with Examples
- Which Platform Should You Choose for Developing Java Desktop Applications?
- Java Commons IO Tutorial with Examples
- Java Commons Email Tutorial with Examples
- Java Commons Logging Tutorial with Examples
- Understanding Java System.identityHashCode, Object.hashCode and Object.equals
- Java SoftReference Tutorial with Examples
- Java Supplier Tutorial with Examples
- Java Aspect Oriented Programming with AspectJ (AOP)
Show More
- Java Servlet/Jsp Tutorials
- Java Collections Framework Tutorials
- Java API for HTML & XML
- Java IO Tutorials
- Java Date Time Tutorials
- Spring Boot Tutorials
- Maven Tutorials
- Gradle Tutorials
- Java Web Services Tutorials
- Java SWT Tutorials
- JavaFX Tutorials
- Java Oracle ADF Tutorials
- Struts2 Framework Tutorials
- Spring Cloud Tutorials