Java Annotations Tutorial with Examples
1. What is annotation
Java annotations are used to provide meta data for your Java code. Being meta data, the annotations do not directly affect the execution of your code, although some types of annotations can actually be used for that purpose.Java annotations were added to Java from Java 5.
Java annotations are typically used for the following purposes:
- Compiler instructions
- Build-time instructions
- Runtime instructions
Instructions for compiler
Java has 3 built-in annotations that you can use to give instructions to the Java compiler.
- @Deprecated
- @Override
- @SuppressWarnings
These annotations are explained in more detail later in this document.
Instruction in Build-time
Java annotations can be be used at build-time, when you build your software project. The build process includes generating source code, compiling the source, generating XML files (e.g. deployment descriptors), packaging the compiled code and files into a JAR file etc. Building the software is typically done by an automatic build tool like Apache Ant or Apache Maven. Build tools may scan your Java code for specific annotations and generate source code or other files based on these annotations.
Instructions in runtime
Normally, Java annotations are not present in your Java code after compilation. It is possible, however, to define your own annotations that are available at runtime. These annotations can then be accessed via Java Reflection, and used to give instructions to your program, or some third party API.
See more:
2. Build-in Annotations
There are 3 important available Annotation of Java
- @Deprecated
- @Override
- @SuppressWarnings
@Deprecated
This is an Annotation used to annotate something deprecated like class or method; and for the best, we should not use it any more.
If you use something deprecated, the compiler will notify you should use an alternative way . Or with programming IDE like Eclipse, it also shows you visual notifications.
If you use something deprecated, the compiler will notify you should use an alternative way . Or with programming IDE like Eclipse, it also shows you visual notifications.
DeprecatedMethodDemo.java
package org.o7planning.tutorial.ann.builtin;
import java.util.Date;
public class DeprecatedMethodDemo {
/**
* @deprecated replaced by {@link #todo(String,Date)}
*/
@Deprecated
public void todoJob(String jobName) {
System.out.println("Todo " + jobName);
}
public void todo(String jobName, Date atTime) {
System.out.println("Todo " + jobName + " at " + atTime);
}
public void todoNothing() {
System.out.println("Todo Nothing");
}
public static void main(String[] args) {
DeprecatedMethodDemo obj = new DeprecatedMethodDemo();
obj.todoJob("Java coding");
obj.todoNothing();
}
}
Here are picture, Eclipse inform you:
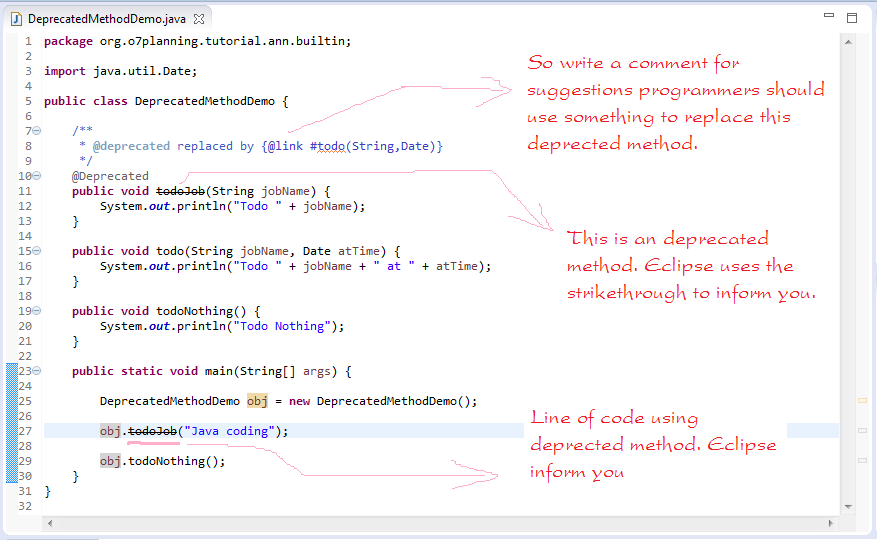
@Override
The @Override annotation is used above methods that override methods in a superclass. If the method does not match a method in the superclass, the compiler will give you an error.
The @Override annotation is not necessary in order to override a method in a superclass. It is a good idea to use it still, though. In case someone changed the name of the overridden method in the superclass, your subclass method would no longer override it. Without the @Override annotation you would not find out. With the @Override annotation the compiler would tell you that the method in the subclass is not overriding any method in the superclass.
The @Override annotation is not necessary in order to override a method in a superclass. It is a good idea to use it still, though. In case someone changed the name of the overridden method in the superclass, your subclass method would no longer override it. Without the @Override annotation you would not find out. With the @Override annotation the compiler would tell you that the method in the subclass is not overriding any method in the superclass.
See an Example:
Job.java
package org.o7planning.tutorial.ann.builtin;
public class Job {
// This is method of Job class.
public String getName() {
return null;
}
}
JavaCoding.java
package org.o7planning.tutorial.ann.builtin;
public class JavaCoding extends Job {
// This method is overridden method getName() of the superclass.
// @Override not required to annotate on this method.
// But it is necessary if someone changed the name of getName()
// method in superclass class, an error message will tell you.
@Override
public String getName() {
return "Java Coding";
}
}
And here is the alert of the Java Compiler:
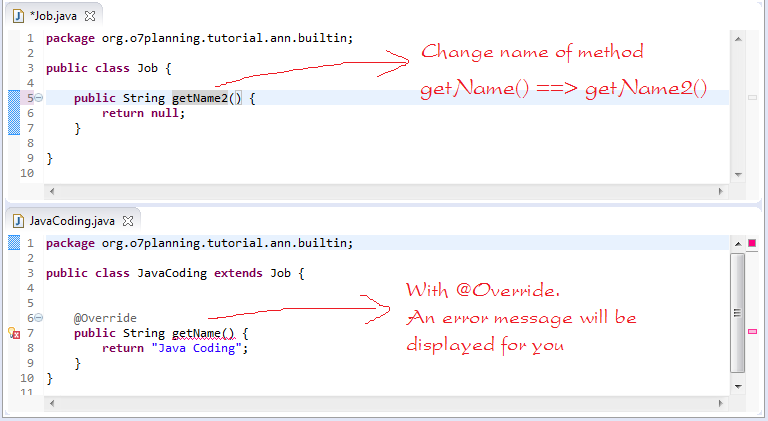
@SuppressWarnings
The @SuppressWarnings annotation makes the compiler suppress warnings for a given method. For instance, if a method calls a deprecated method, or makes an insecure type cast, the compiler may generate a warning. You can suppress these warnings by annotating the method containing the code with the @SuppressWarnings annotation.
See an Example:
SuppressWarningsDemo.java
package org.o7planning.tutorial.ann.builtin;
import java.util.Date;
public class SuppressWarningsDemo {
@SuppressWarnings("deprecation")
public Date getSomeDate() {
Date date = new Date(2014, 9, 25);
return date;
}
}
See the compiler warning:
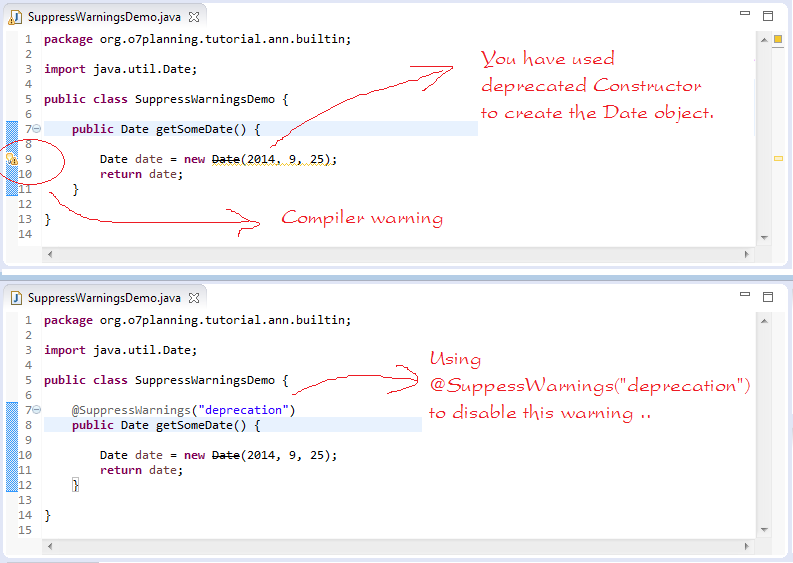
SuppressWarningsDemo2.java
package org.o7planning.tutorial.ann.builtin;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
public class SuppressWarningsDemo2 {
public List<?> getDatas() {
List<String> list = new ArrayList<String>();
list.add("One");
return list;
}
@SuppressWarnings({ "deprecation", "unused", "unchecked" })
public void processDatas() {
// You use deprecated Constructor.
// Variable 'date' was created, but not used
Date date = new Date(2014, 9, 25);
// Cast unsafe.
// Variable 'datas' is created, but not used in the code.
List<String> datas = (List<String>) this.getDatas();
}
}
The warning from the compiler:
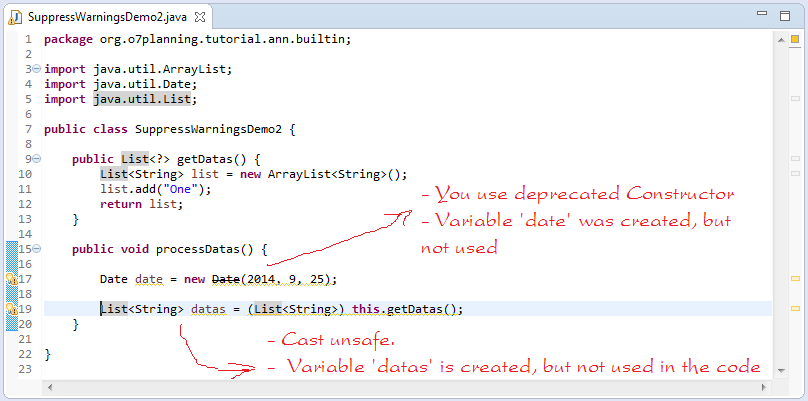
3. Write Your Annotation
Using @interface is a keyword of declaring an Annotation, and this annotation is quite similar to an interface. An annotation may have or not have elements in it.
Characteristics of elements of an annotation:
- There is no function body
- There is no functional parameter
- The returning declaration must be a specific type:
- Primitive type (boolean, int, float,...)
- Enum
- Annotation
- Class (eg: String.class)
- Element can have default values
Your first Annotation
MyFirstAnnotation.java
package org.o7planning.tutorial.ann1;
public @interface MyFirstAnnotation {
// Element 'name'.
public String name();
// Element 'description', default value is "".
public String description() default "";
}
Annotation can be used on:
- TYPE - Class, interface (including annotation type), or enum declaration
- FIELD - Field declaration (includes enum constants)
- METHOD - Method declaration
- PARAMETER - Parameter declaration
- CONSTRUCTOR - Constructor declaration
- LOCAL_VARIABLE - Local variable declaration
- ANNOTATION_TYPE - Annotation declaration
- PACKAGE - Package declaration
UsingMyFirstAnnotation.java
package org.o7planning.tutorial.ann1;
@MyFirstAnnotation(name = "Some name", description = "Some description")
public class UsingMyFirstAnnotation {
// Annotation is annotated on a Constructor.
// The value of the element 'name' is "John"
// The value element 'description' is "Write by John".
@MyFirstAnnotation(name = "John", description = "Write by John")
public UsingMyFirstAnnotation() {
}
// Annotation is annotated on a method.
// The value of the element 'name' is "Tom"
// 'description' element is not declared, it will be assigned a default value.
@MyFirstAnnotation(name = "Tom")
public void someMethod() {
}
// An Annotation is annotated on parameter of method.
public void todo(@MyFirstAnnotation(name = "none") String job) {
// An annotation is annotated on local variable.
@MyFirstAnnotation(name = "Some name")
int localVariable = 0;
}
}
Annotation with element value. (Special!)
An Annotation has an element named value that has some special characteristics:
AnnWithValue.java
package org.o7planning.tutorial.ann2;
public @interface AnnWithValue {
// Element named 'value'
// There is a bit special when using this element.
public int value();
// Element 'name'.
public String name() default "";
}
UsingAnnWithValue.java
package org.o7planning.tutorial.ann2;
public class UsingAnnWithValue {
// Initialize elements of Annotations in the usual way.
@AnnWithValue(name = "Name1", value = 100)
public void someMethod1() {
}
// Initialize the elements of Annotation in the usual way.
// Element 'name' using default.
@AnnWithValue(value = 100)
public void someMethod2() {
}
// The element named 'value' is special.
// Instead of writing @AnnWithValue (value = 100)
// You just write @AnnWithValue (100)
@AnnWithValue(100)
public void someMethod3() {
}
}
@Retention & @Target
@Retention & @Target is two available annotation of Java.
@Retention
@Retention: Used to note the existence level of some annotation.
Particularly, there are 3 levels of existence awareness of the noted thing:
Particularly, there are 3 levels of existence awareness of the noted thing:
- RetentionPolicy.SOURCE: Exists on source code, and free from recognition of compiler.
- RetentionPolicy.CLASS: Its existence is identified by the compiler, but not by the virtual machine at the Runtime.
- RetentionPolicyRUNTIME: reaches the highest level of existence, is identified by compiler and by the virtual machine at the Runtime.
@Target
@Target: is used to note another annotation and its using scope.
- ElementType.TYPE - attached on the declaration of Class, interface, enum, annotation.
- ElementType.FIELD -attached on the declaration of field and the enum constant.
- ElementType.METHOD -attached on the declaration of method.
- ElementType.PARAMETER - attached on the declaration of parameter
- ElementType.CONSTRUCTOR - attached on the declaration of constructor
- ElementType.LOCAL_VARIABLE - attached on the local variable
- ElementType.ANNOTATION_TYPE -attached on the declaration of Annotation
- ElementType.PACKAGE - attached on the declaration of package.
AnnFM.java
package org.o7planning.tutorial.ann3;
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
// This annotation says that AnnFM is only recognized on the source code.
// It will not be recognized by the compiler,
// And in the running time the virtual machine also does not know its existence.
@Retention(value = RetentionPolicy.SOURCE)
// This Annotation says:
// AnnFM will only be used on FIELD or METHOD.
@Target(value = { ElementType.FIELD, ElementType.METHOD })
public @interface AnnFM {
}
UsingAnnFM.java
package org.o7planning.tutorial.ann3;
public class UsingAnnFM {
// AnnFM is only allowed to annotate on FIELD or METHOD.
@AnnFM
protected int someField = 100;
// AnnFM is only allowed to annotate on FIELD or METHOD.
@AnnFM
public void someMethod() {
}
}
Annotation & Reflection
Note: If you have just started to learn Java, you can skip this section. Because it requires synthesized knowledge and learning it is unnecessary at the beginning level.
Java Reflection can identify things like Class, field, method annotated by some Annotation. And of course it can recognize only Annotation with @Retention(RetentionPolicy.RUNTIME)
See more:
The below example describes a program reading annotations on original Java files and creating Html files. Each class is equivalent to a html file.
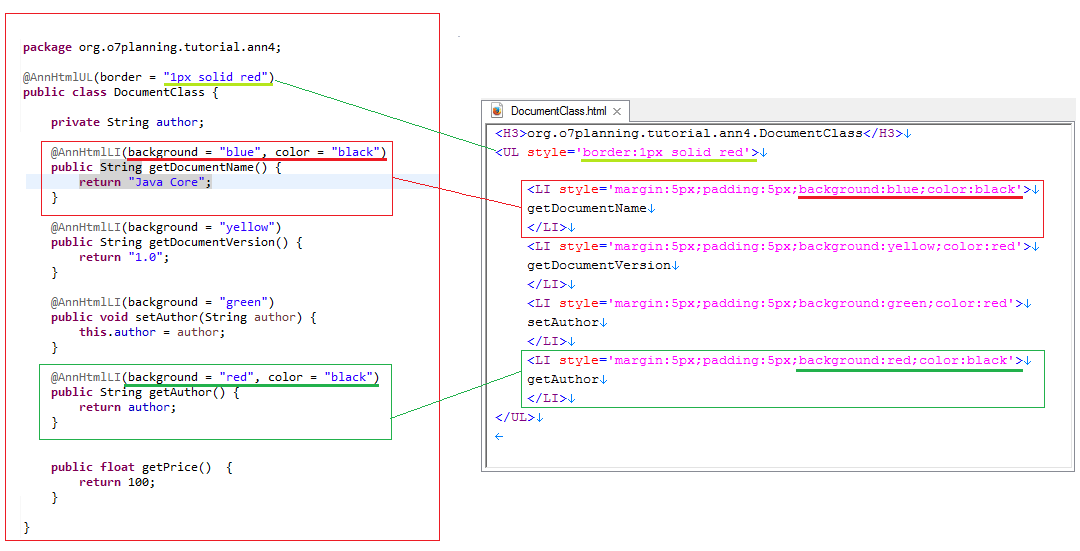
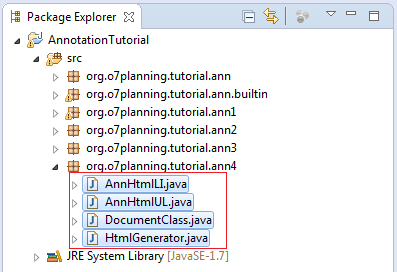
AnnHtmlUL.java
package org.o7planning.tutorial.ann4;
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
@Retention(RetentionPolicy.RUNTIME)
// This Annotation says:
// AnnHtmlUL is only used for Class, interface, annotation, enum.
@Target(value = { ElementType.TYPE })
// AnnHtmlUL: Simulating the <UL> tag in HTML.
public @interface AnnHtmlUL {
public String border() default "border:1px solid blue;";
}
AnnHtmlLI.java
package org.o7planning.tutorial.ann4;
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
@Retention(RetentionPolicy.RUNTIME)
@Target(value = { ElementType.FIELD, ElementType.METHOD })
// Simulating the <LI> tag in HTML.
public @interface AnnHtmlLI {
public String background();
public String color() default "red";
}
DocumentClass.java
package org.o7planning.tutorial.ann4;
@AnnHtmlUL(border = "1px solid red")
public class DocumentClass {
private String author;
@AnnHtmlLI(background = "blue", color = "black")
public String getDocumentName() {
return "Java Core";
}
@AnnHtmlLI(background = "yellow")
public String getDocumentVersion() {
return "1.0";
}
@AnnHtmlLI(background = "green")
public void setAuthor(String author) {
this.author = author;
}
@AnnHtmlLI(background = "red", color = "black")
public String getAuthor() {
return author;
}
// This method is not annotated by any annotation.
public float getPrice() {
return 100;
}
}
HtmlGenerator.java
package org.o7planning.tutorial.ann4;
import java.lang.reflect.Method;
public class HtmlGenerator {
public static void main(String[] args) {
Class<?> clazz = DocumentClass.class;
// Check if this class is annotated by AnnHtmlUL or not.
boolean isHtmlUL = clazz.isAnnotationPresent(AnnHtmlUL.class);
StringBuilder sb = new StringBuilder();
if (isHtmlUL) {
// Get out the AnnHtmlUL object, annotated on this class.
AnnHtmlUL annUL = clazz.getAnnotation(AnnHtmlUL.class);
sb.append("<H3>" + clazz.getName() + "</H3>");
sb.append("\n");
// Get the value of the element 'border'.
String border = annUL.border();
sb.append("<UL style='border:" + border + "'>");
// Add new line
sb.append("\n");
Method[] methods = clazz.getMethods();
for (Method method : methods) {
// Check if this method is annotated by Annotation AnnHtmlLI or not.
if (method.isAnnotationPresent(AnnHtmlLI.class)) {
// Get the annotation
AnnHtmlLI annLI = method.getAnnotation(AnnHtmlLI.class);
// Get the values of element.
String background = annLI.background();
String color = annLI.color();
sb.append("<LI style='margin:5px;padding:5px;background:" + background + ";color:" + color + "'>");
sb.append("\n");
sb.append(method.getName());
sb.append("\n");
sb.append("</LI>");
sb.append("\n");
}
}
sb.append("</UL>");
}
writeToFile(clazz.getSimpleName() + ".html", sb);
}
// Write to Console (Or file)
private static void writeToFile(String fileName, StringBuilder sb) {
System.out.println(sb);
}
}
Results of running the example:
<H3>org.o7planning.tutorial.ann4.DocumentClass</H3>
<UL style='border:1px solid red'>
<LI style='margin:5px;padding:5px;background:blue;color:black'>
getDocumentName
</LI>
<LI style='margin:5px;padding:5px;background:yellow;color:red'>
getDocumentVersion
</LI>
<LI style='margin:5px;padding:5px;background:green;color:red'>
setAuthor
</LI>
<LI style='margin:5px;padding:5px;background:red;color:black'>
getAuthor
</LI>
</UL>
Write to a html file:
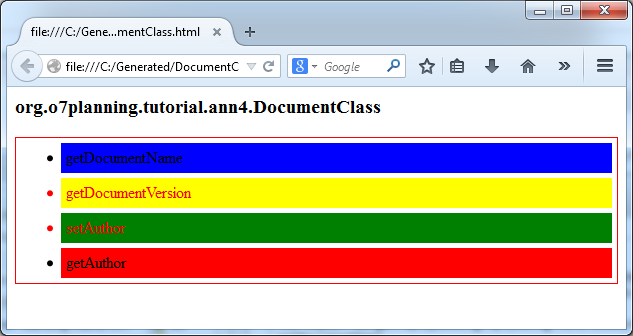
4. Annotation Processing Tool (Advanced Knowledge)
The situation is that:
You create your own Annotations and use them in your Java application. You set using rules for these Annotations. You want the Java Compiler to notify the error of applying the rules wrongly, if any, at the compile-time. If you use Eclipse for writing codes, you want Eclipse will notify the using error right on IDE.
You can see the study guide APT at:
Java Basic
- Customize java compiler processing your Annotation (Annotation Processing Tool)
- Java Programming for team using Eclipse and SVN
- Java WeakReference Tutorial with Examples
- Java PhantomReference Tutorial with Examples
- Java Compression and Decompression Tutorial with Examples
- Configuring Eclipse to use the JDK instead of JRE
- Java String.format() and printf() methods
- Syntax and new features in Java 8
- Java Regular Expressions Tutorial with Examples
- Java Multithreading Programming Tutorial with Examples
- JDBC Driver Libraries for different types of database in Java
- Java JDBC Tutorial with Examples
- Get the values of the columns automatically increment when Insert a record using JDBC
- Java Stream Tutorial with Examples
- Java Functional Interface Tutorial with Examples
- Introduction to the Raspberry Pi
- Java Predicate Tutorial with Examples
- Abstract class and Interface in Java
- Access modifiers in Java
- Java Enums Tutorial with Examples
- Java Annotations Tutorial with Examples
- Comparing and Sorting in Java
- Java String, StringBuffer and StringBuilder Tutorial with Examples
- Java Exception Handling Tutorial with Examples
- Java Generics Tutorial with Examples
- Manipulating files and directories in Java
- Java BiPredicate Tutorial with Examples
- Java Consumer Tutorial with Examples
- Java BiConsumer Tutorial with Examples
- What is needed to get started with Java?
- History of Java and the difference between Oracle JDK and OpenJDK
- Install Java on Windows
- Install Java on Ubuntu
- Install OpenJDK on Ubuntu
- Install Eclipse
- Install Eclipse on Ubuntu
- Quick Learning Java for beginners
- History of bits and bytes in computer science
- Data Types in java
- Bitwise Operations
- if else statement in java
- Switch Statement in Java
- Loops in Java
- Arrays in Java
- JDK Javadoc in CHM format
- Inheritance and polymorphism in Java
- Java Function Tutorial with Examples
- Java BiFunction Tutorial with Examples
- Example of Java encoding and decoding using Apache Base64
- Java Reflection Tutorial with Examples
- Java remote method invocation - Java RMI Tutorial with Examples
- Java Socket Programming Tutorial with Examples
- Which Platform Should You Choose for Developing Java Desktop Applications?
- Java Commons IO Tutorial with Examples
- Java Commons Email Tutorial with Examples
- Java Commons Logging Tutorial with Examples
- Understanding Java System.identityHashCode, Object.hashCode and Object.equals
- Java SoftReference Tutorial with Examples
- Java Supplier Tutorial with Examples
- Java Aspect Oriented Programming with AspectJ (AOP)
Show More
- Java Servlet/Jsp Tutorials
- Java Collections Framework Tutorials
- Java API for HTML & XML
- Java IO Tutorials
- Java Date Time Tutorials
- Spring Boot Tutorials
- Maven Tutorials
- Gradle Tutorials
- Java Web Services Tutorials
- Java SWT Tutorials
- JavaFX Tutorials
- Java Oracle ADF Tutorials
- Struts2 Framework Tutorials
- Spring Cloud Tutorials