Access modifiers in Java
1. Modifier in Java
There are two types of modifiers in java: access modifiers and non-access modifiers.
The access modifiers in java specifies accessibility (scope) of a data member, method, constructor or class.
There are 4 types of java access modifiers:
- private
- (Default)
- protected
- public
There are many non-access modifiers such as static, abstract, synchronized, native, volatile, transient etc. Here, we will learn access modifiers.
2. Overview of access modifiers
The table below illustrates give you an overview of how to use the access modifiers.
Access Modifier | Access within class | Access within package | Access outside package by subclass only | Access outside package and not in subclass. |
private | Y | |||
Default | Y | Y | ||
protected | Y | Y | Y | |
public | Y | Y | Y | Y |
You can see more details follow the example below:
3. private access modifier
The private access modifier is accessible only within class.
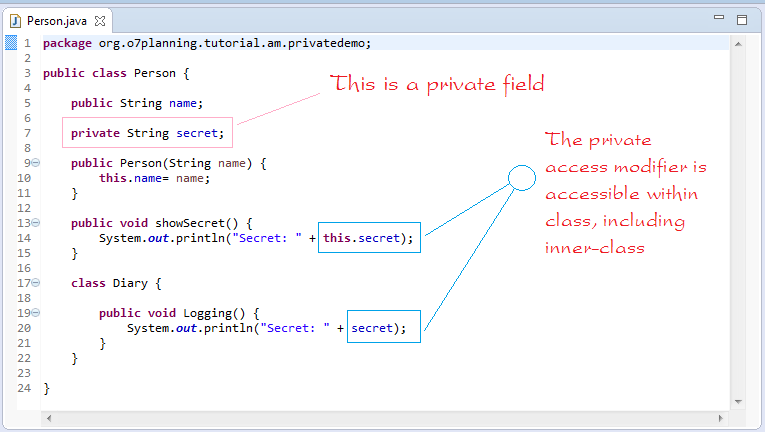
You cannot access to private field outside the class that defines that private. Java will notify error at the compile time of the class.
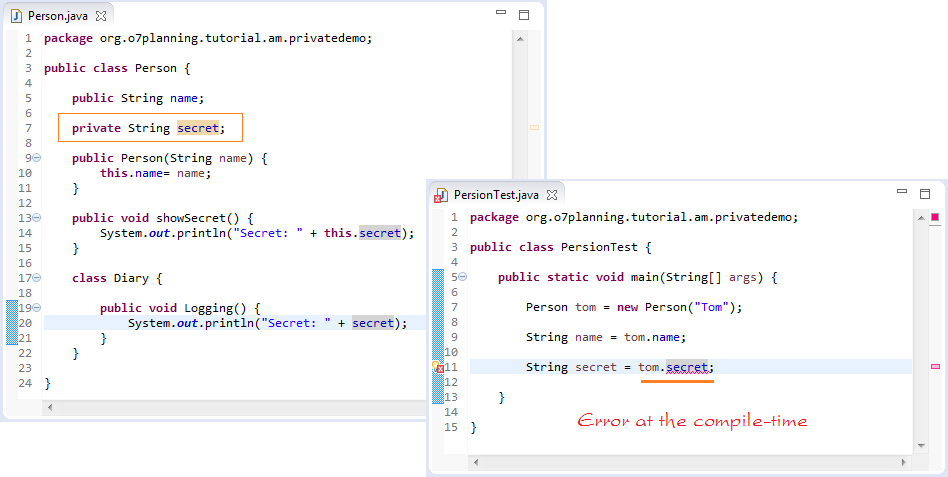
4. private constructor
If you create a class and have a private constructor, you cannot create a object of this class from that private constructor, outside this class. Let's see an illustrative example:
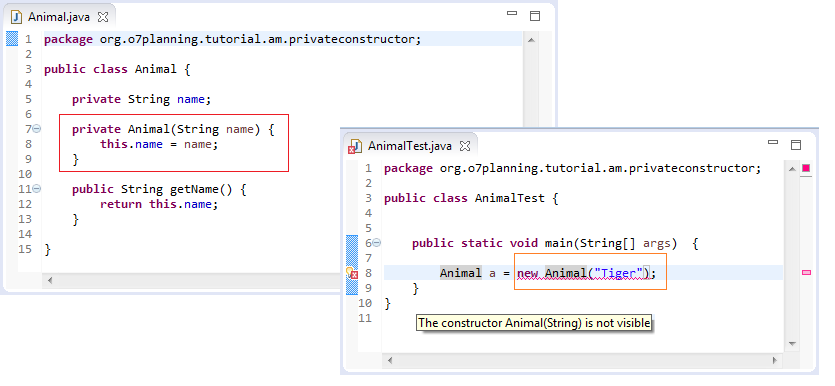
5. Default access modifier
In case you declare a field, method, constructor, but you do not clearly write down access modifier, it means it will be access modifier by default.
The accessing scope of access modifier by default is inside package.
The accessing scope of access modifier by default is inside package.
// A class with default access modifier
// (Not public).
class MyClass {
// A field with private access modifier.
private int myField;
// A field with default access modifier.
// (not specified public, protected, private).
String myField2;
// A method with default access modifier.
// (not specified public, protected, private).
void showMe() {
}
}
In the same package, you can access to members whose access modifier is default.
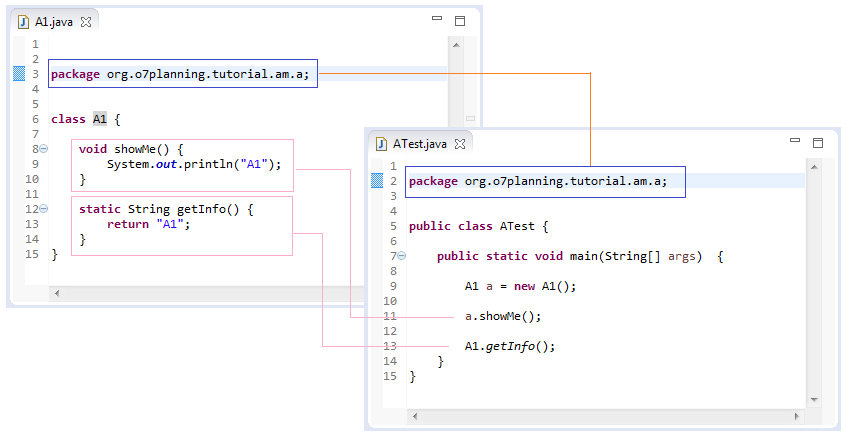
And, you cannot access to things outside package, even if they are in a subclass.
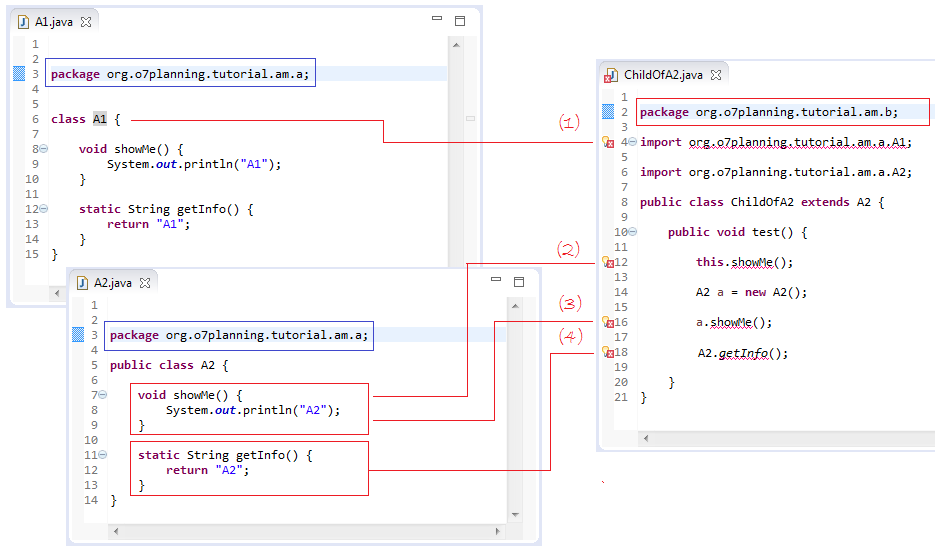
With Interface, when you declare a Field or a Method, you always have to declare the public. You also can declare the default mode, and Java considers it public.
public interface MyInterface {
// This is a field, you can not declare private or protected.
public static int MY_FIELD1 = 100;
// This is a field with default access modifier
// But Java considering this is a public.
static int MY_FIELD2 = 200;
// This is a method, with default access modifier
// But Java considering this is a public.
void showInfo();
}
6. protected access modifier
The protected access modifier is accessible within package and outside the package but through inheritance only.
The protected access modifier can be applied on the data member, method and constructor. It can't be applied on the class.
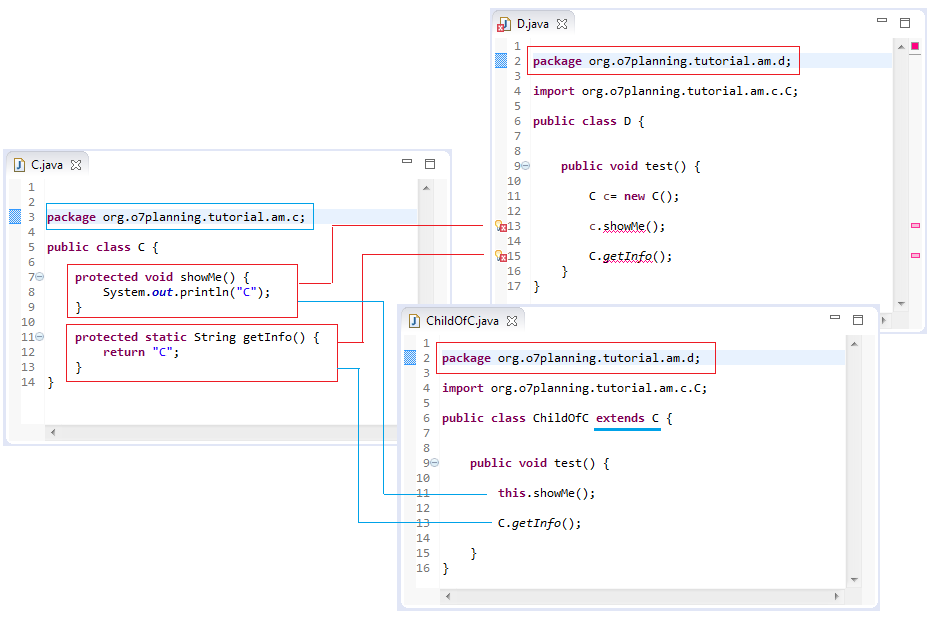
7. public access modifier
The public access modifier is accessible everywhere. It has the widest scope among all other modifiers.
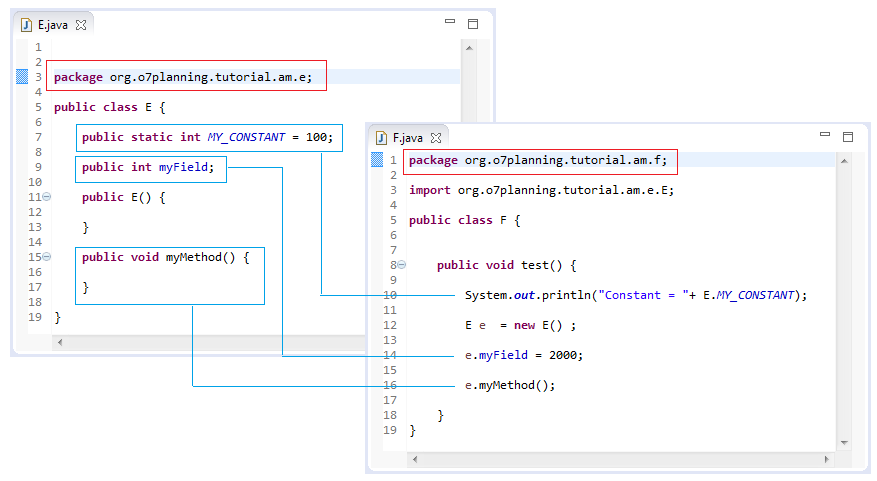
8. Override method
You can override a method of superclass with a method whose name and parameter are similar to those of a superclass. However, you cannot make the accessing scope of this method restrictive.
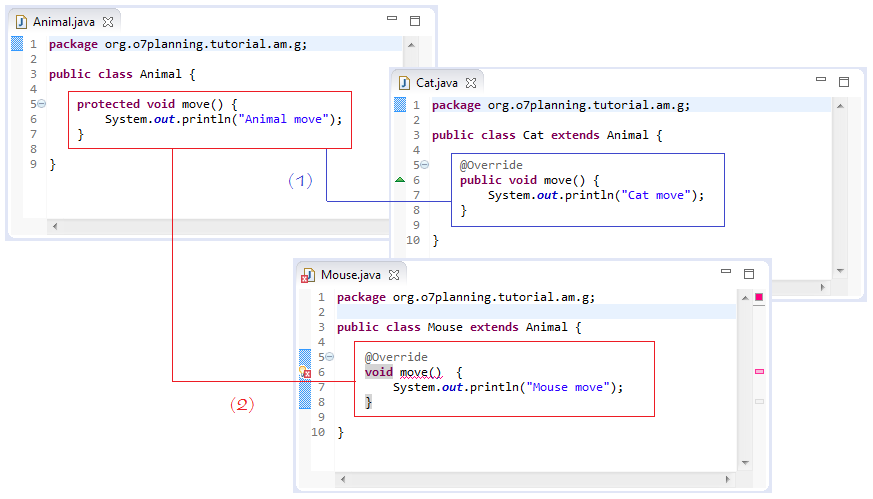
On the above illustrative image
- Cat class extends from Animal class and overrides move() method; the scope of overriding accession changes from protected to public mode. This is valid.
- Mouse class extends from Animal class and overrides move() method; the scope of overriding accession changes from protected to default mode. This reduces the accessing scope of the original method, so it is invalid.
Java Basic
- Customize java compiler processing your Annotation (Annotation Processing Tool)
- Java Programming for team using Eclipse and SVN
- Java WeakReference Tutorial with Examples
- Java PhantomReference Tutorial with Examples
- Java Compression and Decompression Tutorial with Examples
- Configuring Eclipse to use the JDK instead of JRE
- Java String.format() and printf() methods
- Syntax and new features in Java 8
- Java Regular Expressions Tutorial with Examples
- Java Multithreading Programming Tutorial with Examples
- JDBC Driver Libraries for different types of database in Java
- Java JDBC Tutorial with Examples
- Get the values of the columns automatically increment when Insert a record using JDBC
- Java Stream Tutorial with Examples
- Java Functional Interface Tutorial with Examples
- Introduction to the Raspberry Pi
- Java Predicate Tutorial with Examples
- Abstract class and Interface in Java
- Access modifiers in Java
- Java Enums Tutorial with Examples
- Java Annotations Tutorial with Examples
- Comparing and Sorting in Java
- Java String, StringBuffer and StringBuilder Tutorial with Examples
- Java Exception Handling Tutorial with Examples
- Java Generics Tutorial with Examples
- Manipulating files and directories in Java
- Java BiPredicate Tutorial with Examples
- Java Consumer Tutorial with Examples
- Java BiConsumer Tutorial with Examples
- What is needed to get started with Java?
- History of Java and the difference between Oracle JDK and OpenJDK
- Install Java on Windows
- Install Java on Ubuntu
- Install OpenJDK on Ubuntu
- Install Eclipse
- Install Eclipse on Ubuntu
- Quick Learning Java for beginners
- History of bits and bytes in computer science
- Data Types in java
- Bitwise Operations
- if else statement in java
- Switch Statement in Java
- Loops in Java
- Arrays in Java
- JDK Javadoc in CHM format
- Inheritance and polymorphism in Java
- Java Function Tutorial with Examples
- Java BiFunction Tutorial with Examples
- Example of Java encoding and decoding using Apache Base64
- Java Reflection Tutorial with Examples
- Java remote method invocation - Java RMI Tutorial with Examples
- Java Socket Programming Tutorial with Examples
- Which Platform Should You Choose for Developing Java Desktop Applications?
- Java Commons IO Tutorial with Examples
- Java Commons Email Tutorial with Examples
- Java Commons Logging Tutorial with Examples
- Understanding Java System.identityHashCode, Object.hashCode and Object.equals
- Java SoftReference Tutorial with Examples
- Java Supplier Tutorial with Examples
- Java Aspect Oriented Programming with AspectJ (AOP)
Show More
- Java Servlet/Jsp Tutorials
- Java Collections Framework Tutorials
- Java API for HTML & XML
- Java IO Tutorials
- Java Date Time Tutorials
- Spring Boot Tutorials
- Maven Tutorials
- Gradle Tutorials
- Java Web Services Tutorials
- Java SWT Tutorials
- JavaFX Tutorials
- Java Oracle ADF Tutorials
- Struts2 Framework Tutorials
- Spring Cloud Tutorials