Java Commons Email Tutorial with Examples
1. Introduction

Commons Email is a Java library of Apache involving Email. In fact, in order to work with Java Email, you can use JavaMail API integrated in JDK6. Commons Email only helps you to work with JavaMail API more easily, it can not be a substitute for JavaMail API.
Similarly, Commons IO is no substitute for JavaIO but includes a lot of classes and methods in order to work with Java IO more easily as well as to save code time for you.
2. Library
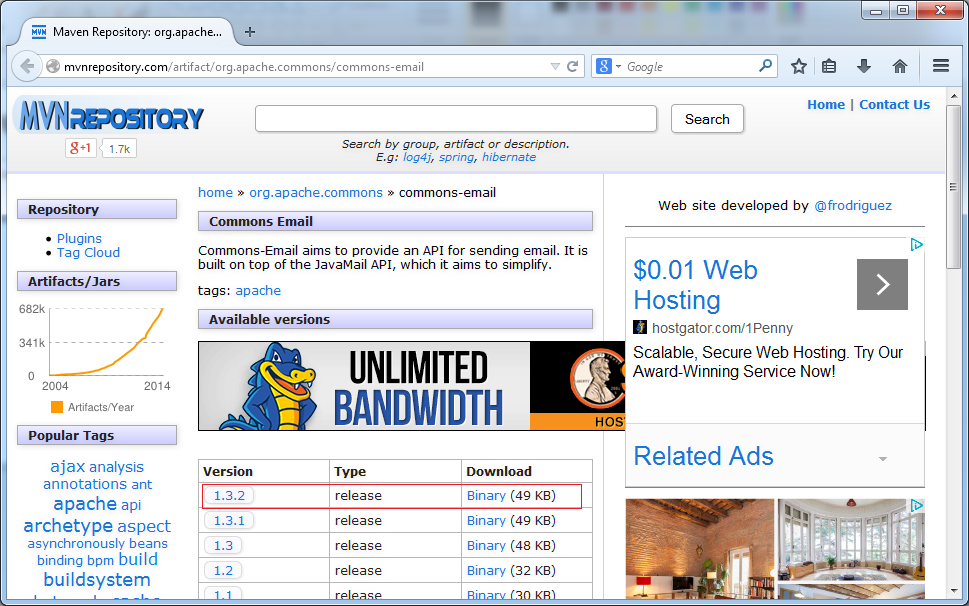
Maven:
** pom.xml **
<!-- http://mvnrepository.com/artifact/org.apache.commons/commons-email -->
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-email</artifactId>
<version>1.3.2</version>
</dependency>
See Also:
3. Note for Gmail
If you want to send email from a Java application that uses Gmail, you need to allow: less secure apps:
On your browser, sign in to your Gmail, and acccess the following link:
You will receive a Google Alert:Some apps and devices use less secure sign-in technology, which makes your account more vulnerable. You can turn off access for these apps, which we recommend, or turn on access if you want to use them despite the risks.
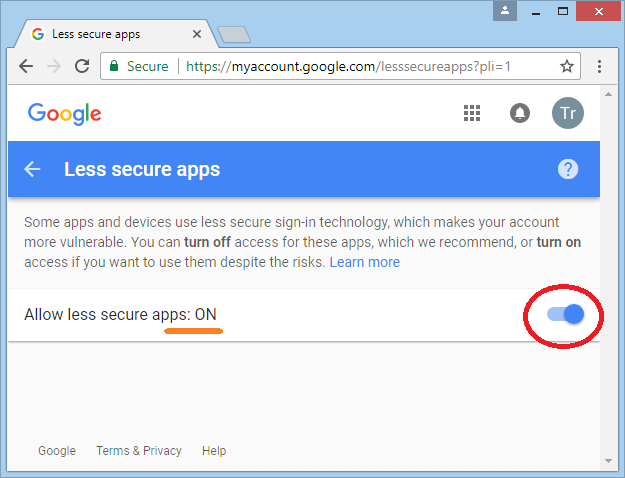
4. Example of sending a simple email
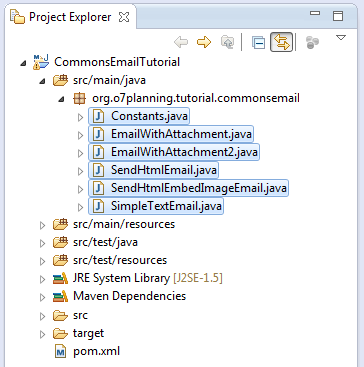
First of all, an example to send a simple Email with a Commons-Email
Constants.java
package org.o7planning.tutorial.commonsemail;
public class Constants {
public static final String MY_EMAIL = "yourEmail@gmail.com";
public static final String MY_PASSWORD ="your password";
public static final String FRIEND_EMAIL = "friendEmail@gmail.com";
}
SimpleTextEmail.java
package org.o7planning.tutorial.commonsemail;
import org.apache.commons.mail.DefaultAuthenticator;
import org.apache.commons.mail.Email;
import org.apache.commons.mail.SimpleEmail;
public class SimpleTextEmail {
public static void main(String[] args) {
try {
Email email = new SimpleEmail();
// Configuration
email.setHostName("smtp.googlemail.com");
email.setSmtpPort(465);
email.setAuthenticator(new DefaultAuthenticator(Constants.MY_EMAIL,
Constants.MY_PASSWORD));
// Required for gmail
email.setSSLOnConnect(true);
// Sender
email.setFrom(Constants.MY_EMAIL);
// Email title
email.setSubject("Test Email");
// Email message.
email.setMsg("This is a test mail ... :-)");
// Receiver
email.addTo(Constants.FRIEND_EMAIL);
email.send();
System.out.println("Sent!!");
} catch (Exception e) {
e.printStackTrace();
}
}
}
Running the example:
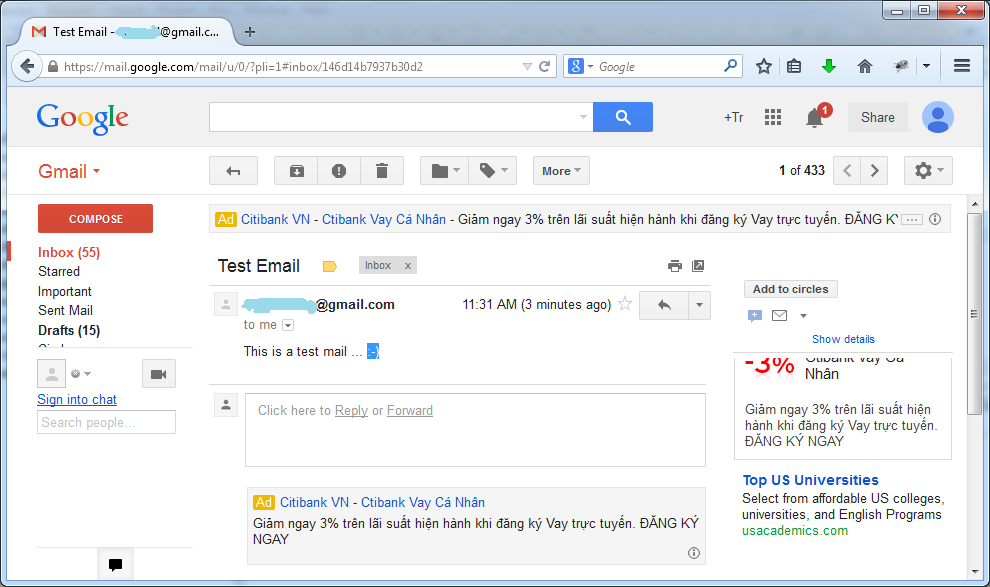
5. Send email with attachments
For example, sending email with a file attached on the hard disk.
EmailWithAttachment.java
package org.o7planning.tutorial.commonsemail;
import org.apache.commons.mail.DefaultAuthenticator;
import org.apache.commons.mail.EmailAttachment;
import org.apache.commons.mail.MultiPartEmail;
public class EmailWithAttachment {
public static void main(String[] args) {
try {
// Create the attachment
EmailAttachment attachment = new EmailAttachment();
attachment.setPath("C:/mypictures/map-vietnam.png");
attachment.setDisposition(EmailAttachment.ATTACHMENT);
attachment.setDescription("Vietnam Map");
attachment.setName("Map");
// Create the email message
MultiPartEmail email = new MultiPartEmail();
// Configuration
email.setHostName("smtp.googlemail.com");
email.setSmtpPort(465);
email.setSSLOnConnect(true);
email.setAuthenticator(new DefaultAuthenticator(Constants.MY_EMAIL,
Constants.MY_PASSWORD));
email.setFrom(Constants.MY_EMAIL, "TRAN");
email.addTo(Constants.FRIEND_EMAIL, "Hong");
email.setSubject("The Map");
email.setMsg("Here is the map you wanted");
// Add the attachment
email.attach(attachment);
// Send the email
email.send();
System.out.println("Sent!");
} catch (Exception e) {
e.printStackTrace();
}
}
}
Results:
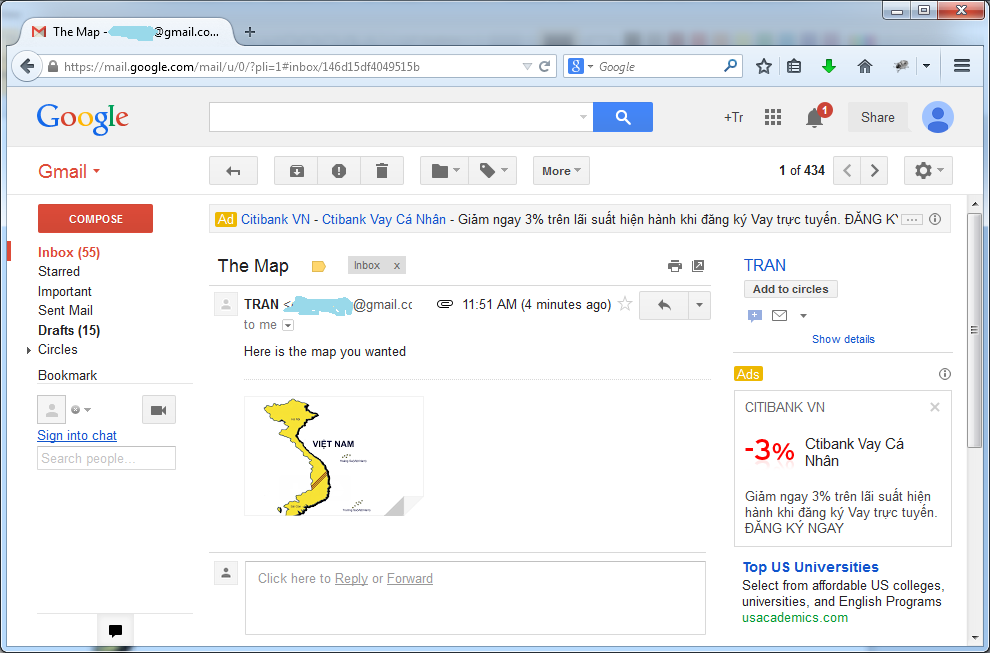
Another example is sending an email with attachments from a link.
EmailWithAttachment2.java
package org.o7planning.tutorial.commonsemail;
import java.net.URL;
import org.apache.commons.mail.DefaultAuthenticator;
import org.apache.commons.mail.EmailAttachment;
import org.apache.commons.mail.MultiPartEmail;
public class EmailWithAttachment2 {
public static void main(String[] args) {
try {
// Create the attachment
EmailAttachment attachment = new EmailAttachment();
attachment.setURL(new URL(
"http://www.apache.org/images/asf_logo_wide.gif"));
attachment.setDisposition(EmailAttachment.ATTACHMENT);
attachment.setDescription("Apache logo");
attachment.setName("Apache logo");
// Create the email message
MultiPartEmail email = new MultiPartEmail();
// Configuration
email.setHostName("smtp.googlemail.com");
email.setSmtpPort(465);
email.setSSLOnConnect(true);
email.setAuthenticator(new DefaultAuthenticator(
Constants.MY_EMAIL, Constants.MY_PASSWORD));
email.setFrom(Constants.MY_EMAIL, "TRAN");
email.addTo(Constants.FRIEND_EMAIL, "Hong");
email.setSubject("The logo");
email.setMsg("Here is Apache's logo");
// Add the attachment
email.attach(attachment);
// Send the email
email.send();
System.out.println("Sent!");
} catch (Exception e) {
e.printStackTrace();
}
}
}
Running the example:
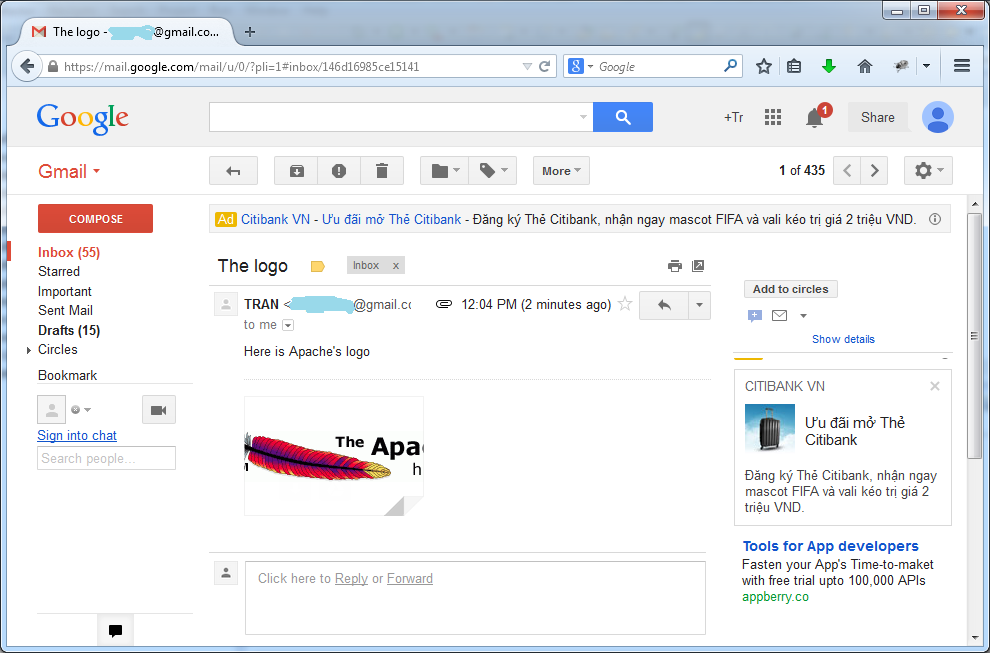
6. Send Email in HTML format
SendHtmlEmail.java
package org.o7planning.tutorial.commonsemail;
import java.net.URL;
import org.apache.commons.mail.DefaultAuthenticator;
import org.apache.commons.mail.HtmlEmail;
public class SendHtmlEmail {
public static void main(String[] args) {
try {
// Create the email message
HtmlEmail email = new HtmlEmail();
// Configuration
email.setHostName("smtp.googlemail.com");
email.setSmtpPort(465);
email.setAuthenticator(new DefaultAuthenticator(
Constants.MY_EMAIL,Constants.MY_PASSWORD));
email.setSSLOnConnect(true);
email.setFrom(Constants.MY_EMAIL, "TRAN");
// Receiver
email.addTo(Constants.FRIEND_EMAIL);
// Title
email.setSubject("Test Sending HTML formatted email");
// Embed the image and get the content id
URL url = new URL("http://www.apache.org/images/asf_logo_wide.gif");
String cid = email.embed(url, "Apache logo");
// Set the html message
email.setHtmlMsg("<html><h2>The apache logo</h2> <img src=\"cid:"
+ cid + "\"></html>");
// Set the alternative message
email.setTextMsg("Your email client does not support HTML messages");
// Send email
email.send();
System.out.println("Sent!!");
} catch (Exception e) {
e.printStackTrace();
}
}
}
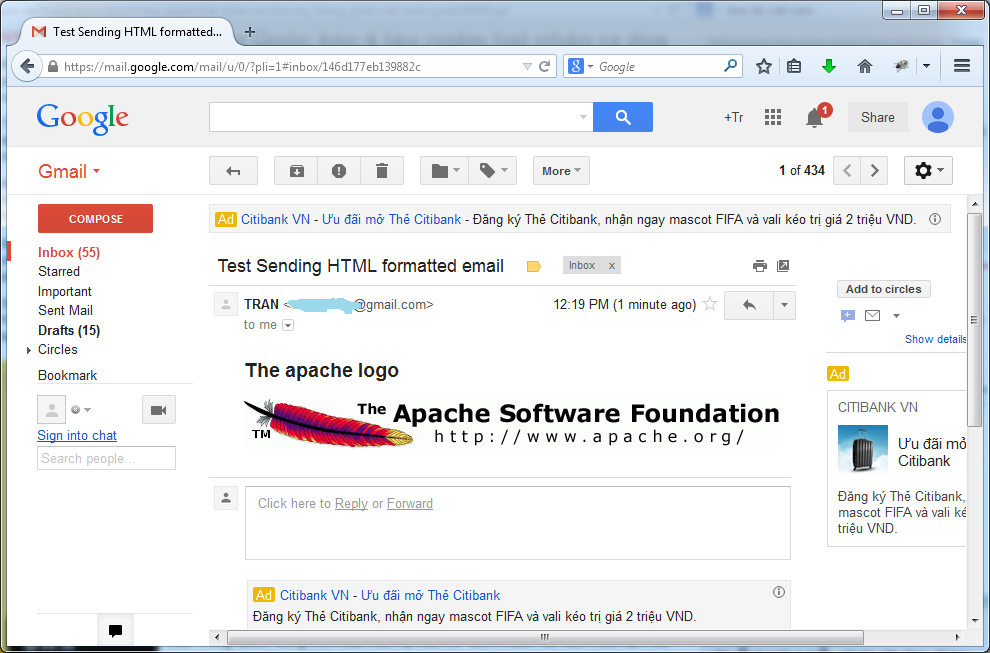
7. Send an Email in HTML format, embed photos
SendHtmlEmbedImageEmail.java
package org.o7planning.tutorial.commonsemail;
import java.net.URL;
import org.apache.commons.mail.DefaultAuthenticator;
import org.apache.commons.mail.ImageHtmlEmail;
import org.apache.commons.mail.resolver.DataSourceUrlResolver;
public class SendHtmlEmbedImageEmail {
public static void main(String[] args) {
try {
// Load your HTML email template
// Here, Img has relative location (**)
String htmlEmailTemplate = "<h2>Hello!</h2>"
+"This is Apache Logo <br/>"
+"<img src='proper/commons-email/images/commons-logo.png'/>";
// Create the email message
ImageHtmlEmail email = new ImageHtmlEmail();
// Configuration
email.setHostName("smtp.googlemail.com");
email.setSmtpPort(465);
email.setAuthenticator(new DefaultAuthenticator(
Constants.MY_EMAIL, Constants.MY_PASSWORD));
email.setSSLOnConnect(true);
email.setFrom(Constants.MY_EMAIL, "TRAN");
email.addTo(Constants.FRIEND_EMAIL);
email.setSubject("Sending HTML formatted email with embedded images");
// Define you base URL to resolve relative resource locations
// (Example - Img you see above ** )
URL url = new URL("http://commons.apache.org");
email.setDataSourceResolver(new DataSourceUrlResolver(url) );
// Set the html message
email.setHtmlMsg(htmlEmailTemplate);
// Set the alternative message
email.setTextMsg("Your email client does not support HTML messages");
// Send the email
email.send();
System.out.println("Sent!!");
} catch (Exception e) {
e.printStackTrace();
}
}
}
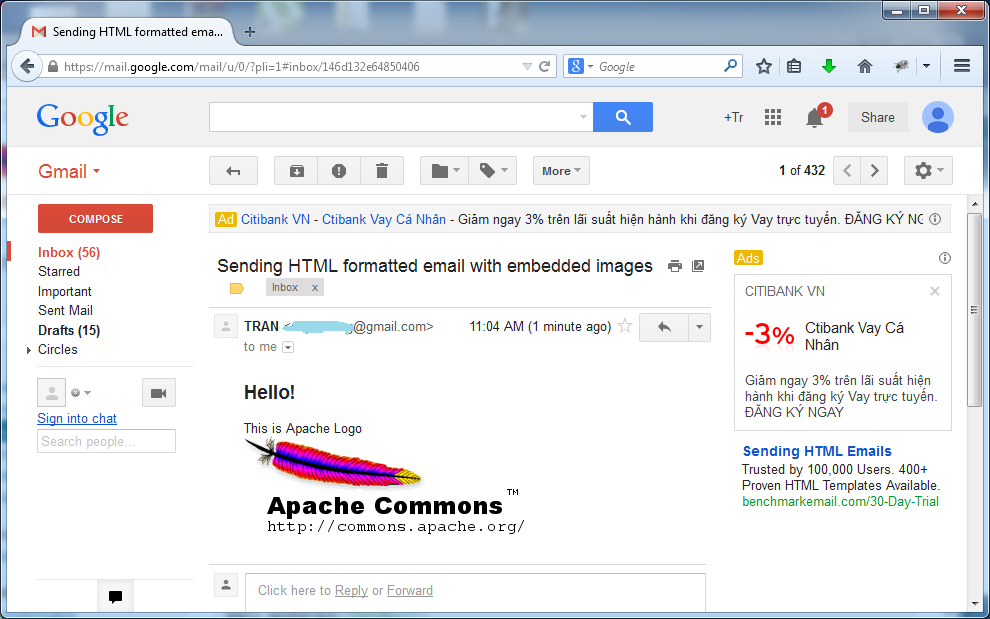
Java Basic
- Customize java compiler processing your Annotation (Annotation Processing Tool)
- Java Programming for team using Eclipse and SVN
- Java WeakReference Tutorial with Examples
- Java PhantomReference Tutorial with Examples
- Java Compression and Decompression Tutorial with Examples
- Configuring Eclipse to use the JDK instead of JRE
- Java String.format() and printf() methods
- Syntax and new features in Java 8
- Java Regular Expressions Tutorial with Examples
- Java Multithreading Programming Tutorial with Examples
- JDBC Driver Libraries for different types of database in Java
- Java JDBC Tutorial with Examples
- Get the values of the columns automatically increment when Insert a record using JDBC
- Java Stream Tutorial with Examples
- Java Functional Interface Tutorial with Examples
- Introduction to the Raspberry Pi
- Java Predicate Tutorial with Examples
- Abstract class and Interface in Java
- Access modifiers in Java
- Java Enums Tutorial with Examples
- Java Annotations Tutorial with Examples
- Comparing and Sorting in Java
- Java String, StringBuffer and StringBuilder Tutorial with Examples
- Java Exception Handling Tutorial with Examples
- Java Generics Tutorial with Examples
- Manipulating files and directories in Java
- Java BiPredicate Tutorial with Examples
- Java Consumer Tutorial with Examples
- Java BiConsumer Tutorial with Examples
- What is needed to get started with Java?
- History of Java and the difference between Oracle JDK and OpenJDK
- Install Java on Windows
- Install Java on Ubuntu
- Install OpenJDK on Ubuntu
- Install Eclipse
- Install Eclipse on Ubuntu
- Quick Learning Java for beginners
- History of bits and bytes in computer science
- Data Types in java
- Bitwise Operations
- if else statement in java
- Switch Statement in Java
- Loops in Java
- Arrays in Java
- JDK Javadoc in CHM format
- Inheritance and polymorphism in Java
- Java Function Tutorial with Examples
- Java BiFunction Tutorial with Examples
- Example of Java encoding and decoding using Apache Base64
- Java Reflection Tutorial with Examples
- Java remote method invocation - Java RMI Tutorial with Examples
- Java Socket Programming Tutorial with Examples
- Which Platform Should You Choose for Developing Java Desktop Applications?
- Java Commons IO Tutorial with Examples
- Java Commons Email Tutorial with Examples
- Java Commons Logging Tutorial with Examples
- Understanding Java System.identityHashCode, Object.hashCode and Object.equals
- Java SoftReference Tutorial with Examples
- Java Supplier Tutorial with Examples
- Java Aspect Oriented Programming with AspectJ (AOP)
Show More
- Java Servlet/Jsp Tutorials
- Java Collections Framework Tutorials
- Java API for HTML & XML
- Java IO Tutorials
- Java Date Time Tutorials
- Spring Boot Tutorials
- Maven Tutorials
- Gradle Tutorials
- Java Web Services Tutorials
- Java SWT Tutorials
- JavaFX Tutorials
- Java Oracle ADF Tutorials
- Struts2 Framework Tutorials
- Spring Cloud Tutorials