Java JDBC Tutorial with Examples
1. Database used in this document
This document guide you how to use interactive Java with database.
The Database model used in this document is "simplehr". You can see scripts of creating database at:
The Database model used in this document is "simplehr". You can see scripts of creating database at:
2. What is JDBC?
Java Database Connectivity (JDBC) is a standard Java API to interact with relational databases form Java. JDBC has set of classes and interfaces which can use from Java application and talk to database without learning RDBMS details and using Database Specific JDBC Drivers.
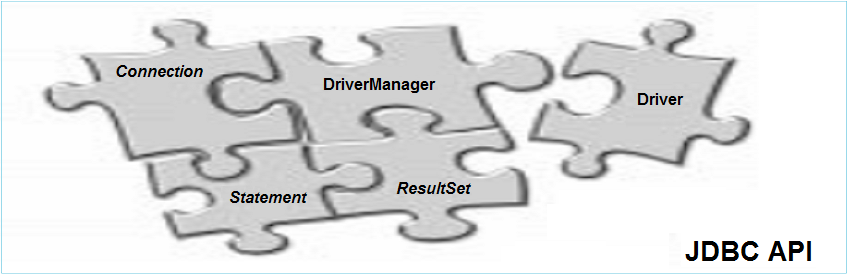
The components of JDBC Api basically include:
- DriverManager:
- Manages a list of database drivers. Matches connection requests from the java application with the proper database driver using communication subprotocol. The first driver that recognizes a certain subprotocol under JDBC will be used to establish a database Connection.
- Driver:
- The database communications link, handling all communication with the database. Normally, once the driver is loaded, the developer need not call it explicitly.
- Connection :
- Interface with all methods for contacting a database.The connection object represents communication context, i.e., all communication with database is through connection object only.
- Statement :
- Encapsulates an SQL statement which is passed to the database to be parsed, compiled, planned and executed.
- ResultSet:
- The ResultSet represents set of rows retrieved due to query execution.
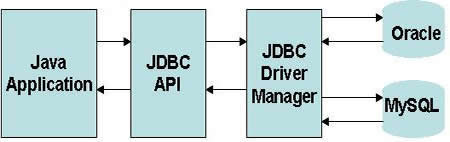
3. How to connect java with database
Java using JDBC to work with the database.
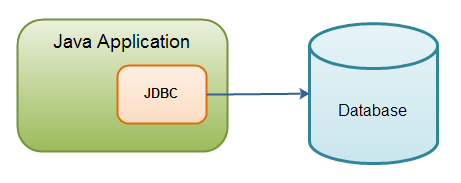
For example, when working with Oracle Database from Java you need to have a Driver (It is a class driving the connection with types of database you want). In JDBC API, we have java.sql.Driver, it is only an interface and it is available in JDK. Thus, you have to download library Driver compatible with the type of Database you want.
- For example, with Oracle, the class implements the Interface java.sql.Driver is oracle.jdbc.driver.OracleDriver
Please see the illustration below:
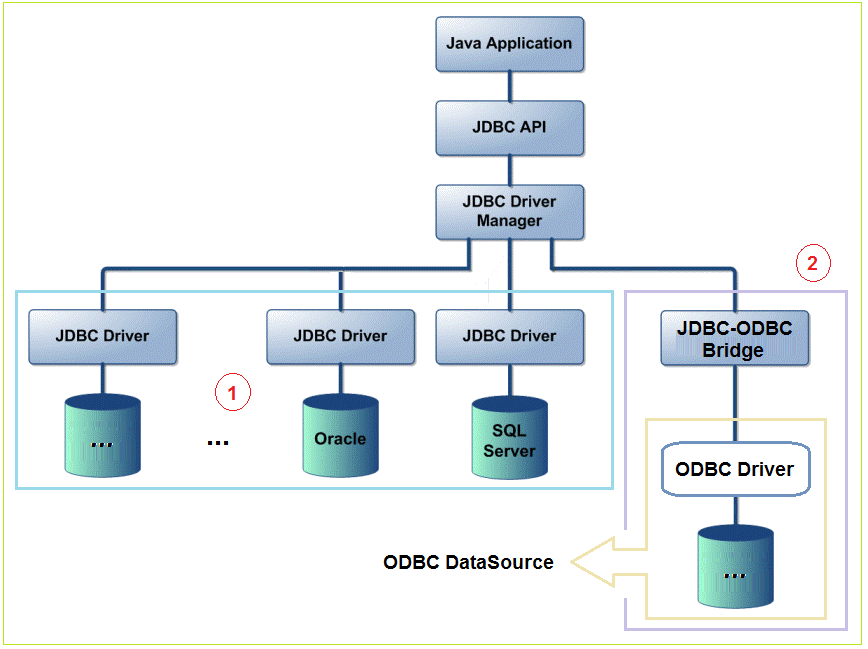
We have two manners to work with a particular database.
- Manner 1: You provide Library Driver of that type of database, this is the direct manner. If you use DB oracle (or other DB), you will have to download the specific JDBC library of this DB.
- Manner 2: Declare an "ODBC DataSource", and use the JDBC-ODBC Bridge for connecting with that "ODBC DataSource". The JDBC-ODBC Bridge is available in JDBC API.

The question for us is that what "ODBC DataSource" is.
ODBC - Open Database Connectivity: It is a set of open libraries that are able to connect to almost all types of various database, and it is free. It is provided by Microsoft.
ODBC DataSource: On Window operating system, you can declare an ODBC connection to some DB type. As a result, we have a Data Source.
In the JDBC API, a JDBC-ODBC Bridge has been set up so that JDBC can works with ODBC.
ODBC - Open Database Connectivity: It is a set of open libraries that are able to connect to almost all types of various database, and it is free. It is provided by Microsoft.
ODBC DataSource: On Window operating system, you can declare an ODBC connection to some DB type. As a result, we have a Data Source.
In the JDBC API, a JDBC-ODBC Bridge has been set up so that JDBC can works with ODBC.
As for speed, the first manner is faster than the second one, because the second one has to use bridge.
4. Download JDBC Driver
If you do not want to use JDBC-ODBC, you can directly connect with Database. In this case, you need to download the Driver compatible with each DB type. Here I instruct you to download a type of Driver for common Database:
- Oracle
- MySQL
- SQLServer
- ....
You can see instructions at:
The result we have a few files:
Database | Library |
Oracle | ojdbc6.jar |
MySQL | mysql-connector-java-x.jar |
SQL Server | jtds-x.jar |
SQL Server | sqljdbc4.jar |
5. Create project to start with JDBC
Create project JavaJdbcTutorial:
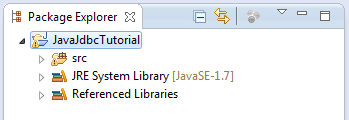
Create libs folder on project, copy libraries and connected them directly with types of database Oracle, MySQL, SQLServer you have just download. You can copy the whole or one of these libraries compatible with the DB type you use.
Note: You just need to download a Driver equivalent to the type of Database you are familiar to. You can get the Database used as the example in this document at:
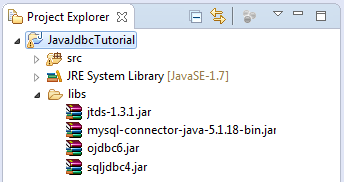
Right-click and select Properties Project:
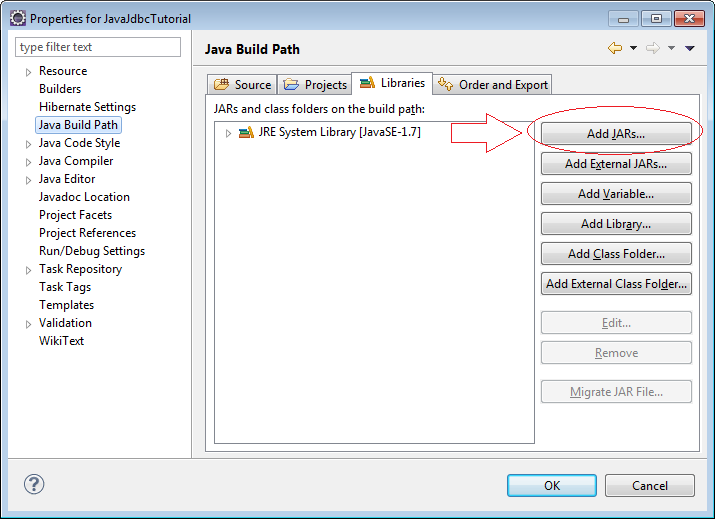
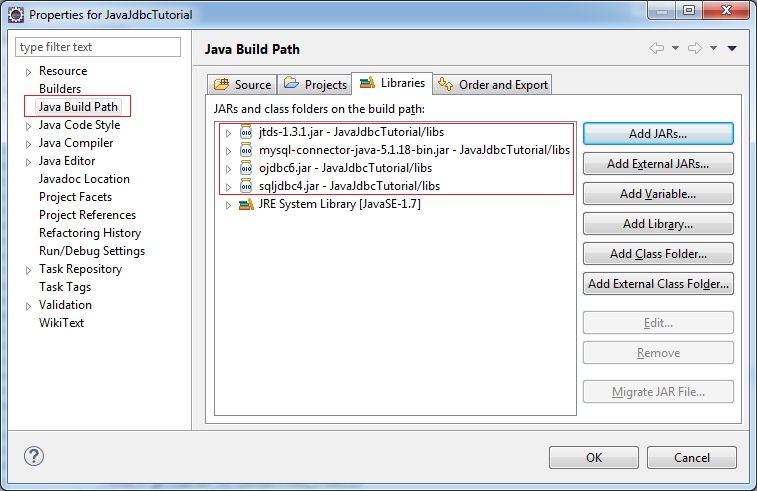
Now you are ready to work with one of Database (Oracle, MySQL, SQL Server):
6. Connection
In this instruction document, I will instruct you to connect to all three types of database:...
- MySQL
- SQLServer
- Oracle
Into practice, you just need work with the DB type you feel familiar to.
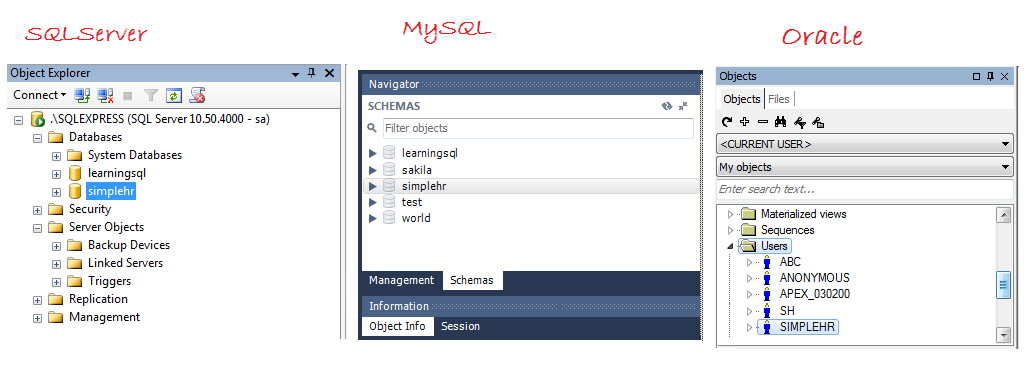
Create utility class ConnectionUtils to get Connection
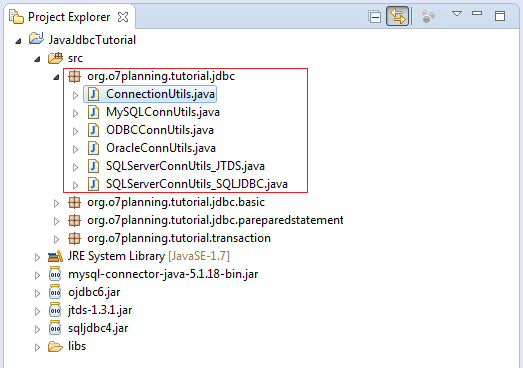
ConnectionUtils.java
package org.o7planning.tutorial.jdbc;
import java.sql.Connection;
import java.sql.SQLException;
public class ConnectionUtils {
public static Connection getMyConnection() throws SQLException,
ClassNotFoundException {
// Using Oracle
// You may be replaced by other Database.
return OracleConnUtils.getOracleConnection();
}
//
// Test Connection ...
//
public static void main(String[] args) throws SQLException,
ClassNotFoundException {
System.out.println("Get connection ... ");
// Get a Connection object
Connection conn = ConnectionUtils.getMyConnection();
System.out.println("Get connection " + conn);
System.out.println("Done!");
}
}
OracleConnUtils.java
package org.o7planning.tutorial.jdbc;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class OracleConnUtils {
// Connect to Oracle.
public static Connection getOracleConnection() throws SQLException,
ClassNotFoundException {
String hostName = "localhost";
String sid = "db11g";
String userName = "simplehr";
String password = "1234";
return getOracleConnection(hostName, sid, userName, password);
}
public static Connection getOracleConnection(String hostName, String sid,
String userName, String password) throws ClassNotFoundException,
SQLException {
// Declare the class Driver for ORACLE DB
// This is necessary with Java 5 (or older)
// Java6 (or newer) automatically find the appropriate driver.
// If you use Java> 5, then this line is not needed.
Class.forName("oracle.jdbc.driver.OracleDriver");
String connectionURL = "jdbc:oracle:thin:@" + hostName + ":1521:" + sid;
Connection conn = DriverManager.getConnection(connectionURL, userName,
password);
return conn;
}
}
MySQLConnUtils.java
package org.o7planning.tutorial.jdbc;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class MySQLConnUtils {
// Connect to MySQL
public static Connection getMySQLConnection() throws SQLException,
ClassNotFoundException {
String hostName = "localhost";
String dbName = "simplehr";
String userName = "root";
String password = "1234";
return getMySQLConnection(hostName, dbName, userName, password);
}
public static Connection getMySQLConnection(String hostName, String dbName,
String userName, String password) throws SQLException,
ClassNotFoundException {
// Declare the class Driver for MySQL DB
// This is necessary with Java 5 (or older)
// Java6 (or newer) automatically find the appropriate driver.
// If you use Java> 5, then this line is not needed.
Class.forName("com.mysql.jdbc.Driver");
String connectionURL = "jdbc:mysql://" + hostName + ":3306/" + dbName;
Connection conn = DriverManager.getConnection(connectionURL, userName,
password);
return conn;
}
}
SQLServerConnUtils_JTDS.java
package org.o7planning.tutorial.jdbc;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class SQLServerConnUtils_JTDS {
// Connect to SQLServer
// (Using JDBC Driver of JTDS library)
public static Connection getSQLServerConnection()
throws SQLException, ClassNotFoundException {
String hostName = "localhost";
String sqlInstanceName = "SQLEXPRESS";
String database = "simplehr";
String userName = "sa";
String password = "1234";
return getSQLServerConnection(hostName, sqlInstanceName, database,
userName, password);
}
// Connect to SQLServer & using JTDS library
public static Connection getSQLServerConnection(String hostName,
String sqlInstanceName, String database, String userName,
String password) throws ClassNotFoundException, SQLException {
// Declare the class Driver for SQLServer DB
// This is necessary with Java 5 (or older)
// Java6 (or newer) automatically find the appropriate driver.
// If you use Java> 5, then this line is not needed.
Class.forName("net.sourceforge.jtds.jdbc.Driver");
// Example:
// jdbc:jtds:sqlserver://localhost:1433/simplehr;instance=SQLEXPRESS
String connectionURL = "jdbc:jtds:sqlserver://" + hostName + ":1433/"
+ database + ";instance=" + sqlInstanceName;
Connection conn = DriverManager.getConnection(connectionURL, userName,
password);
return conn;
}
}
SQLServerConnUtils_SQLJDBC.java
package org.o7planning.tutorial.jdbc;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class SQLServerConnUtils_SQLJDBC {
// Connect to SQLServer
// (Using JDBC Driver: SQLJDBC)
public static Connection getSQLServerConnection()
throws SQLException, ClassNotFoundException {
String hostName = "localhost";
String sqlInstanceName = "SQLEXPRESS";
String database = "simplehr";
String userName = "sa";
String password = "1234";
return getSQLServerConnection(hostName, sqlInstanceName,
database, userName, password);
}
//
// Connect to SQLServer & using SQLJDBC Library.
public static Connection getSQLServerConnection(String hostName,
String sqlInstanceName, String database, String userName,
String password) throws ClassNotFoundException, SQLException {
// Declare the class Driver for SQLServer DB
// This is necessary with Java 5 (or older)
// Java6 (or newer) automatically find the appropriate driver.
// If you use Java> 5, then this line is not needed.
Class.forName("com.microsoft.sqlserver.jdbc.SQLServerDriver");
// jdbc:sqlserver://ServerIp:1433/SQLEXPRESS;databaseName=simplehr
String connectionURL = "jdbc:sqlserver://" + hostName + ":1433"
+ ";instance=" + sqlInstanceName + ";databaseName=" + database;
Connection conn = DriverManager.getConnection(connectionURL, userName,
password);
return conn;
}
}
ODBCConnUtils.java
package org.o7planning.tutorial.jdbc;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class ODBCConnUtils {
// Connect to ODBC Data Source named "simplehr-ds".
public static Connection getJdbcOdbcConnection() throws SQLException,
ClassNotFoundException {
String odbcDataSourceName = "simplehr-ds";
String userName = "simplehr";
String password = "simplehr";
return getJdbcOdbcConnection(odbcDataSourceName, userName, password);
}
public static Connection getJdbcOdbcConnection(String odbcDataSourceName,
String userName, String password) throws SQLException,
ClassNotFoundException {
// Declare the class Driver for JDBC-ODBC Bridge
// This is necessary with Java 5 (or older)
// Java6 (or newer) automatically find the appropriate driver.
// If you use Java> 5, then this line is not needed.
Class.forName("sun.jdbc.odbc.JdbcOdbcDriver");
String connectionURL = "jdbc:odbc:" + odbcDataSourceName;
Connection conn = DriverManager.getConnection(connectionURL, userName,
password);
return conn;
}
}
You can change ConnectionUtils class for connecting to familiar Database, and run this class for testing the connection.
Get connection ...
Get connection oracle.jdbc.driver.T4CConnection@5f54e92c
Done!
Note: If you use MySQL or SQL Server by default two Database prevent connecting to it from other IP address. You need to configure it so that this connection is allowed. You can see the instruction in the installing and configuration document of MySQL, SQL Server on the o7planning.Installing and Configuring MySQL Community:Installing and Configuring SQL Server:
7. Using the JDBC API to query data
This is a data in Employee table. We see how Java query data through an example:
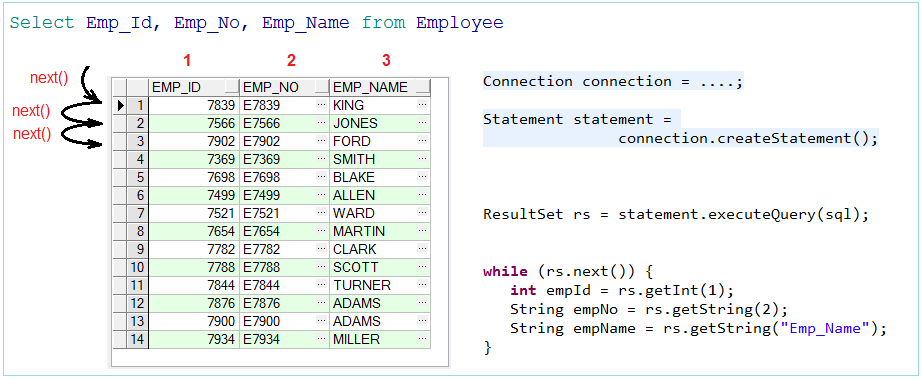
ResultSet is a Java object returned when you query data. Using ResultSet.next() for moving the cursor to the next records (Move by row). In a particular record, you use ResultSet.getXxx() methods for getting values in columns. Columns are arranged in ordinal number (1, 2, 3...).
** ResultSet **
public String getString(int columnIndex) throws SQLException;
public boolean getBoolean(int columnIndex) throws SQLException;
public int getInt(int columnIndex) throws SQLException;
public double getDouble(int columnIndex) throws SQLException;
...
public String getString(String columnLabel) throws SQLException;
public boolean getBoolean(String columnLabel) throws SQLException;
public int getInt(String columnLabel) throws SQLException;
public double getDouble(String columnLabel) throws SQLException;
....
Example:
QueryDataExample.java
package org.o7planning.tutorial.jdbc.basic;
import java.sql.Connection;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
import org.o7planning.tutorial.jdbc.ConnectionUtils;
public class QueryDataExample {
public static void main(String[] args) throws ClassNotFoundException,
SQLException {
// Get Connection
Connection connection = ConnectionUtils.getMyConnection();
// Create statement
Statement statement = connection.createStatement();
String sql = "Select Emp_Id, Emp_No, Emp_Name from Employee";
// Execute SQL statement returns a ResultSet object.
ResultSet rs = statement.executeQuery(sql);
// Fetch on the ResultSet
// Move the cursor to the next record.
while (rs.next()) {
int empId = rs.getInt(1);
String empNo = rs.getString(2);
String empName = rs.getString("Emp_Name");
System.out.println("--------------------");
System.out.println("EmpId:" + empId);
System.out.println("EmpNo:" + empNo);
System.out.println("EmpName:" + empName);
}
// Close connection.
connection.close();
}
}
Results of running the example:
EmpId:7900
EmpNo:E7900
EmpName:ADAMS
--------------------
EmpId:7934
EmpNo:E7934
EmpName:MILLER
8. ResultSet types
You have got accustomed with ResultSet through above examples. By default, when fetch data, The cursor only can move from top to bottom and from right to left. It means that with default ResultSet you can't call:
- ResultSet.previous() : step back a record.
- On the same record, you can't call ResultSet.getXxx(4) first, and then call ResultSet.getXxx(2).
public Statement createStatement(int resultSetType, int resultSetConcurrency)
throws SQLException;
// Example:
Statement statement = connection.createStatement(
ResultSet.TYPE_SCROLL_INSENSITIVE, ResultSet.CONCUR_READ_ONLY);
// ResultSet can scroll & readonly
ResultSet rs = statement.executeQuery(sql);
resultSetType | Meaning |
TYPE_FORWARD_ONLY | - The constant indicating the type for a ResultSet object whose cursor may move only forward. |
TYPE_SCROLL_INSENSITIVE | - The constant indicating the type for a ResultSet object that is scrollable but generally not sensitive to changes to the data that underlies the ResultSet. |
TYPE_SCROLL_SENSITIVE | - The constant indicating the type for a ResultSet object that is scrollable and generally sensitive to changes to the data that underlies the ResultSet. |
resultSetConcurrency | Meaning |
CONCUR_READ_ONLY | - The constant indicating the concurrency mode for a ResultSet object that may NOT be updated. |
CONCUR_UPDATABLE | - The constant indicating the concurrency mode for a ResultSet object that may be updated. |
ScrollableResultSetExample.java
package org.o7planning.tutorial.jdbc.basic;
import java.sql.Connection;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
import org.o7planning.tutorial.jdbc.ConnectionUtils;
public class ScrollableResultSetExample {
public static void main(String[] args) throws ClassNotFoundException,
SQLException {
// Get a Connection
Connection connection = ConnectionUtils.getMyConnection();
// Create a Statement object
// can be srolled, but not sensitive to changes under DB.
// ResultSet is readonly (Cannot update)
Statement statement = connection.createStatement(
ResultSet.TYPE_SCROLL_INSENSITIVE, ResultSet.CONCUR_READ_ONLY);
String sql = "Select Emp_Id, Emp_No, Emp_Name from Employee";
// Execute statement, return ResultSet.
ResultSet rs = statement.executeQuery(sql);
// Jump the cursor to last record.
boolean last = rs.last();
System.out.println("last : "+ last);
if(last) {
// Print out data of last record
System.out.println("EmpId:" + rs.getInt(1));
System.out.println("EmpNo:" + rs.getString(2));
System.out.println("EmpName:" + rs.getString(3));
}
System.out.println("--------------------");
// Move cursor to previous record
boolean previous =rs.previous();
System.out.println("Previous 1: "+ previous);
// Move cursor to previous record
previous =rs.previous();
System.out.println("Previous 2: "+ previous);
// Fetch in the ResultSet
while (rs.next()) {
// Get value of column 2
String empNo = rs.getString(2);
// Then get the value of column 1.
int empId = rs.getInt(1);
String empName = rs.getString("Emp_Name");
System.out.println("--------------------");
System.out.println("EmpId:" + empId);
System.out.println("EmpNo:" + empNo);
System.out.println("EmpName:" + empName);
}
// Close connection.
connection.close();
}
}
Results of running the example:
last : true
EmpId:7934
EmpNo:E7934
EmpName:MILLER
--------------------
Previous 1: true
Previous 2: true
--------------------
EmpId:7902
EmpNo:E7902
EmpName:FORD
--------------------
EmpId:7934
EmpNo:E7934
EmpName:MILLER
9. Insert example
InsertDataExample.java
package org.o7planning.tutorial.jdbc.basic;
import java.sql.Connection;
import java.sql.SQLException;
import java.sql.Statement;
import org.o7planning.tutorial.jdbc.ConnectionUtils;
public class InsertDataExample {
public static void main(String[] args) throws ClassNotFoundException,
SQLException {
// Get Connection
Connection connection = ConnectionUtils.getMyConnection();
Statement statement = connection.createStatement();
String sql = "Insert into Salary_Grade (Grade, High_Salary, Low_Salary) "
+ " values (2, 20000, 10000) ";
// Execute statement
// executeUpdate(String) using for Insert, Update, Delete statement.
int rowCount = statement.executeUpdate(sql);
System.out.println("Row Count affected = " + rowCount);
}
}
Results of running the example:
Row Count affected = 1
10. PreparedStatement
PreparedStatement is an extends Interface of Statement.
PreparedStatement is used for preparing SQL statement, reusing many times, and helping speed up the program.
PreparedStatement is used for preparing SQL statement, reusing many times, and helping speed up the program.
PrepareStatementExample.java
package org.o7planning.tutorial.jdbc.pareparedstatement;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import org.o7planning.tutorial.jdbc.ConnectionUtils;
public class PrepareStatementExample {
public static void main(String[] args) throws ClassNotFoundException,
SQLException {
// Get a connection
Connection connection = ConnectionUtils.getMyConnection();
// Create a SQL statement with two parameters (?)
String sql = "Select emp.Emp_Id, emp.Emp_No, emp.Emp_Name,"
+ " emp.Dept_Id from Employee emp "
+ " where emp.Emp_Name like ? and emp.Dept_Id = ? ";
// Create a PreparedStatement object.
PreparedStatement pstm = connection.prepareStatement(sql);
// Set value for the first parameter (First '?')
pstm.setString(1, "%S");
// Set value for the second parameter (Second '?')
pstm.setInt(2, 20);
ResultSet rs = pstm.executeQuery();
while (rs.next()) {
System.out.println(" ---- ");
System.out.println("EmpId : " + rs.getInt("Emp_Id"));
System.out.println("EmpNo : " + rs.getString(2));
System.out.println("EmpName : " + rs.getString("Emp_Name"));
}
System.out.println();
System.out.println("Set other parameters ..");
// Reuse PreparedStatement
// Set other values
pstm.setString(1, "KI%");
pstm.setInt(2,10);
// Execute statement.
rs = pstm.executeQuery();
while (rs.next()) {
System.out.println(" ---- ");
System.out.println("EmpId : " + rs.getInt("Emp_Id"));
System.out.println("EmpNo : " + rs.getString(2));
System.out.println("EmpName : " + rs.getString("Emp_Name"));
}
}
}
Results of running the example:
----
EmpId : 7566
EmpNo : E7566
EmpName : JONES
----
EmpId : 7876
EmpNo : E7876
EmpName : ADAMS
Set other parameters ...
----
EmpId : 7839
EmpNo : E7839
EmpName : KING
11. CallableStatement
CallableStatement used to call a stored procedure or SQL functions.
// Statement call procedure from Java
String sql = "{call procedure_name(?,?,?)}";
// Statement call function from Java.
String sql ="{? = call function_name(?,?,?)}";
For example with CallableStatement we need a function or a procedure in DB. With Oracle, MySQL or SQLServer you can quickly create a procedure as below:
ORACLE
Get_Employee_Info
-- This procedure retrieves information of an employee,
-- Input parameter: p_Emp_ID (Integer)
-- There are four output parameters v_Emp_No, v_First_Name, v_Last_Name, v_Hire_Date
Create Or Replace Procedure Get_Employee_Info(p_Emp_Id Integer
,v_Emp_No Out Varchar2
,v_First_Name Out Varchar2
,v_Last_Name Out Varchar2
,v_Hire_Date Out Date) Is
Begin
v_Emp_No := 'E' || p_Emp_Id;
--
v_First_Name := 'Michael';
v_Last_Name := 'Smith';
v_Hire_Date := Sysdate;
End Get_Employee_Info;
MySQL
Get_Employee_Info
-- This procedure retrieves information of an employee,
-- Input parameter: p_Emp_ID (Integer)
-- There are four output parameters v_Emp_No, v_First_Name, v_Last_Name, v_Hire_Date
CREATE PROCEDURE get_Employee_Info(p_Emp_ID Integer,
out v_Emp_No Varchar(50) ,
out v_First_Name Varchar(50) ,
Out v_Last_name Varchar(50) ,
Out v_Hire_date Date)
BEGIN
set v_Emp_No = concat( 'E' , Cast(p_Emp_Id as char(15)) );
--
set v_First_Name = 'Michael';
set v_Last_Name = 'Smith';
set v_Hire_date = curdate();
END
SQL Server
Get_Employee_Info
-- This procedure retrieves information of an employee,
-- Input parameter: p_Emp_ID (Integer)
-- There are four output parameters v_Emp_No, v_First_Name, v_Last_Name, v_Hire_Date
CREATE PROCEDURE Get_Employee_Info
@p_Emp_Id Integer ,
@v_Emp_No Varchar(50) OUTPUT,
@v_First_Name Varchar(50) OUTPUT,
@v_Last_Name Varchar(50) OUTPUT,
@v_Hire_Date Date OUTPUT
AS
BEGIN
set @v_Emp_No = 'E' + CAST( @p_Emp_Id as varchar) ;
--
set @v_First_Name = 'Michael';
set @v_Last_Name = 'Smith';
set @v_Hire_date = getdate();
END
CallableStatementExample.java
package org.o7planning.tutorial.jdbc.callablestatement;
import java.sql.CallableStatement;
import java.sql.Connection;
import java.sql.Date;
import java.sql.SQLException;
import org.o7planning.tutorial.jdbc.ConnectionUtils;
public class CallableStatementExample {
public static void main(String[] args) throws ClassNotFoundException,
SQLException {
// Get a connection
Connection connection = ConnectionUtils.getMyConnection();
String sql = "{call get_Employee_Info(?,?,?,?,?)}";
// Create a CallableStatement object.
CallableStatement cstm = connection.prepareCall(sql);
// Set parameter value for first question mark
// (p_Emp_ID)
cstm.setInt(1, 10);
// Registers the OUT parameter for 2st question mark.
// (v_Emp_No)
cstm.registerOutParameter(2, java.sql.Types.VARCHAR);
// Registers the OUT parameter for 3rd question mark.
// (v_First_Name)
cstm.registerOutParameter(3, java.sql.Types.VARCHAR);
// Registers the OUT parameter for 4th question mark.
// (v_Last_Name)
cstm.registerOutParameter(4, java.sql.Types.VARCHAR);
// Registers the OUT parameter for 5th question mark.
// (v_Hire_Date)
cstm.registerOutParameter(5, java.sql.Types.DATE);
// Execute statement.
cstm.executeUpdate();
String empNo = cstm.getString(2);
String firstName = cstm.getString(3);
String lastName = cstm.getString(4);
Date hireDate = cstm.getDate(5);
System.out.println("Emp No: " + empNo);
System.out.println("First Name: " + firstName);
System.out.println("Last Name: " + lastName);
System.out.println("Hire Date: " + hireDate);
}
}
Results of running the example:
Emp No : E10
First Name: Michael
Last Name: Smith
Hire Date: 2015-03-01
12. Control Transaction
Transaction is an important concept in SQL.
For example, A person send a sum of 1,000 dollar to B person's account, so there are two actions happening in Database:
And the transaction is considered successful if two above steps are implemented successfully. On the contrary, if one of two steps is fails, the transaction should be considered unsuccessfully and we will have to have a rollback from the early status.
For example, A person send a sum of 1,000 dollar to B person's account, so there are two actions happening in Database:
- Debit 1,000 dollar on the A person's account
- Credit 1,000 dollar on the B person's account.
And the transaction is considered successful if two above steps are implemented successfully. On the contrary, if one of two steps is fails, the transaction should be considered unsuccessfully and we will have to have a rollback from the early status.
TransactionExample.java
package org.o7planning.tutorial.transaction;
import java.sql.Connection;
import java.sql.SQLException;
import org.o7planning.tutorial.jdbc.ConnectionUtils;
public class TransactionExample {
private static void doJob1(Connection conn) {
// Todo something here.
}
private static void doJob2(Connection conn) {
// Todo something here.
}
public static void main(String[] args) throws ClassNotFoundException,
SQLException {
// Get a connection
Connection connection = ConnectionUtils.getMyConnection();
// Set auto-commit to false
connection.setAutoCommit(false);
try {
// Do something related to the DB.
doJob1(connection);
doJob2(connection);
// Explicitly commit statements to apply changes
connection.commit();
}
// Handling exception
catch (Exception e) {
e.printStackTrace();
// Rollback changes
connection.rollback();
}
// Close connection.
connection.close();
}
}
13. Execute a batch command
BatchExample.java
package org.o7planning.tutorial.transaction;
import java.sql.Connection;
import java.sql.SQLException;
import java.sql.Statement;
import org.o7planning.tutorial.jdbc.ConnectionUtils;
public class BatchExample {
public static void main(String[] args) throws SQLException,
ClassNotFoundException {
Connection conn = ConnectionUtils.getMyConnection();
try {
// Create statement object
Statement stmt = conn.createStatement();
// Set auto-commit to false
conn.setAutoCommit(false);
// Create SQL statement to insert into Employee table.
String sql1 = "Update Employee emp set emp.Salary = emp.Salary + 100 "
+ " where emp.Dept_Id = 10 ";
// Add above SQL statement in the batch.
stmt.addBatch(sql1);
// Create one more SQL statement
String sql2 = "Update Employee emp set emp.Salary = emp.Salary + 20 "
+ " where emp.Dept_Id = 20 ";
// Add above SQL statement in the batch.
stmt.addBatch(sql2);
// Create one more SQL statement
String sql3 = "Update Employee emp set emp.Salary = emp.Salary + 30 "
+ " where emp.Dept_Id = 30 ";
// Add above SQL statement in the batch.
stmt.addBatch(sql3);
// Create an int[] to hold returned values
int[] counts = stmt.executeBatch();
System.out.println("Sql1 count = " + counts[0]);
System.out.println("Sql2 count = " + counts[1]);
System.out.println("Sql3 count = " + counts[2]);
// Explicitly commit statements to apply changes
conn.commit();
} catch (Exception e) {
e.printStackTrace();
conn.rollback();
}
}
}
Results of running the example:
Sql1 count = 2
Sql2 count = 5
Sql3 count = 7
BatchExample2.java
package org.o7planning.tutorial.transaction;
import java.sql.Connection;
import java.sql.Date;
import java.sql.PreparedStatement;
import java.sql.SQLException;
import java.util.UUID;
import org.o7planning.tutorial.jdbc.ConnectionUtils;
public class BatchExample2 {
public static void main(String[] args) throws ClassNotFoundException,
SQLException {
Connection conn = ConnectionUtils.getMyConnection();
try {
String sql = "Insert into Timekeeper(Timekeeper_Id, Date_Time, In_Out, Emp_Id) "
+ " values (?,?,?,?) ";
// Create statement object
PreparedStatement stmt = conn.prepareStatement(sql);
// Set auto-commit to false
conn.setAutoCommit(false);
// Set values for parameters
stmt.setString(1, UUID.randomUUID().toString());
stmt.setDate(2, new Date(System.currentTimeMillis()));
stmt.setString(3, "I");
stmt.setInt(4, 7839);
// Add to batch
stmt.addBatch();
// Set other values for parameters.
stmt.setString(1, UUID.randomUUID().toString());
stmt.setDate(2, new Date(System.currentTimeMillis()));
stmt.setString(3, "I");
stmt.setInt(4, 7566);
// Add to batch.
stmt.addBatch();
// Create an int[] to hold returned values
int[] counts = stmt.executeBatch();
System.out.println("counts[0] = " + counts[0]);
System.out.println("counts[1] = " + counts[1]);
// Explicitly commit statements to apply changes
conn.commit();
} catch (Exception e) {
e.printStackTrace();
conn.rollback();
}
}
}
Results of running the example:
counts[0] = 1
counts[1] = 1
Java Basic
- Customize java compiler processing your Annotation (Annotation Processing Tool)
- Java Programming for team using Eclipse and SVN
- Java WeakReference Tutorial with Examples
- Java PhantomReference Tutorial with Examples
- Java Compression and Decompression Tutorial with Examples
- Configuring Eclipse to use the JDK instead of JRE
- Java String.format() and printf() methods
- Syntax and new features in Java 8
- Java Regular Expressions Tutorial with Examples
- Java Multithreading Programming Tutorial with Examples
- JDBC Driver Libraries for different types of database in Java
- Java JDBC Tutorial with Examples
- Get the values of the columns automatically increment when Insert a record using JDBC
- Java Stream Tutorial with Examples
- Java Functional Interface Tutorial with Examples
- Introduction to the Raspberry Pi
- Java Predicate Tutorial with Examples
- Abstract class and Interface in Java
- Access modifiers in Java
- Java Enums Tutorial with Examples
- Java Annotations Tutorial with Examples
- Comparing and Sorting in Java
- Java String, StringBuffer and StringBuilder Tutorial with Examples
- Java Exception Handling Tutorial with Examples
- Java Generics Tutorial with Examples
- Manipulating files and directories in Java
- Java BiPredicate Tutorial with Examples
- Java Consumer Tutorial with Examples
- Java BiConsumer Tutorial with Examples
- What is needed to get started with Java?
- History of Java and the difference between Oracle JDK and OpenJDK
- Install Java on Windows
- Install Java on Ubuntu
- Install OpenJDK on Ubuntu
- Install Eclipse
- Install Eclipse on Ubuntu
- Quick Learning Java for beginners
- History of bits and bytes in computer science
- Data Types in java
- Bitwise Operations
- if else statement in java
- Switch Statement in Java
- Loops in Java
- Arrays in Java
- JDK Javadoc in CHM format
- Inheritance and polymorphism in Java
- Java Function Tutorial with Examples
- Java BiFunction Tutorial with Examples
- Example of Java encoding and decoding using Apache Base64
- Java Reflection Tutorial with Examples
- Java remote method invocation - Java RMI Tutorial with Examples
- Java Socket Programming Tutorial with Examples
- Which Platform Should You Choose for Developing Java Desktop Applications?
- Java Commons IO Tutorial with Examples
- Java Commons Email Tutorial with Examples
- Java Commons Logging Tutorial with Examples
- Understanding Java System.identityHashCode, Object.hashCode and Object.equals
- Java SoftReference Tutorial with Examples
- Java Supplier Tutorial with Examples
- Java Aspect Oriented Programming with AspectJ (AOP)
Show More
- Java Servlet/Jsp Tutorials
- Java Collections Framework Tutorials
- Java API for HTML & XML
- Java IO Tutorials
- Java Date Time Tutorials
- Spring Boot Tutorials
- Maven Tutorials
- Gradle Tutorials
- Java Web Services Tutorials
- Java SWT Tutorials
- JavaFX Tutorials
- Java Oracle ADF Tutorials
- Struts2 Framework Tutorials
- Spring Cloud Tutorials