Manipulating files and directories in Java
1. Overview
In fact, you often have to manipulate files and folders in system including adding, deleting, renaming. Java provides you 2 classes to do this:
- java.io.File
- java.nio.file.Files
java.io.File
The java.io.File class represents a file or a directory of system and it has included in Java since version 1.0.
java.nio.file.Files
In Version 7.0, Java adds the java.nio.file.Files class, which simplifies the manipulation of files and directories, all the methods of the class are static and nature.
In the post, I just mention the use of the java.io.File class, you can see more about java.nio.file.Files here:
- Thao tác với tập tin và thư mục sử dụng Java NIO Files
2. java.io.File
The java.io.File class represents a file or a directory in the system, which represents a pathname. The example below creates an file representing a path on Windows or Unix:
// Window
File apath1 = new File("C:/mydocument/test.txt");
File apath2 = new File("C:/mydocument/java");
// Unix
File apath3 = new File("/mydocument/test.txt");
File apath3 = new File("/mydocument/java");
java.io.File can represent a path that may not actually exist on the system. If it exists, it may be a folder or a file.
The simple example below creates a File object that represents a path, and checks for its existence, print out basic information if that path actually exists:
FileInfoExample.java
package org.o7planning.filedirectory;
import java.io.File;
import java.util.Date;
public class FileInfoExample {
public static void main(String[] args) {
// Create a File represents a path.
File apath = new File("C:/test/mytext.txt");
// Check if exists.
System.out.println("Path exists? " + apath.exists());
if (apath.exists()) {
// Check if 'apath' is a directory.
System.out.println("Directory? " + apath.isDirectory());
// Check 'apath' is a Hidden path.
System.out.println("Hidden? " + apath.isHidden());
// Simple name.
System.out.println("Simple Name: " + apath.getName());
// Absolute path.
System.out.println("Absolute Path: " + apath.getAbsolutePath());
// Check file size (in bytes):
System.out.println("Length (bytes): " + apath.length());
// Last modify (in milli second)
long lastMofifyInMillis = apath.lastModified();
Date lastModifyDate = new Date(lastMofifyInMillis);
System.out.println("Last modify date: " + lastModifyDate);
}
}
}
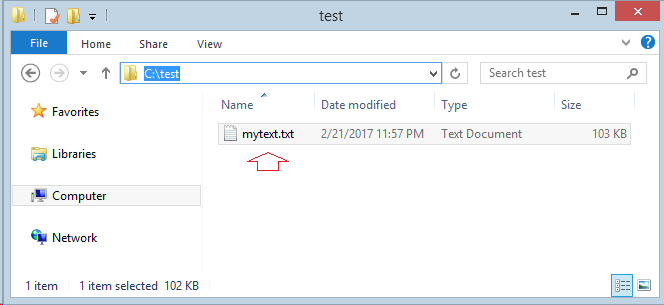
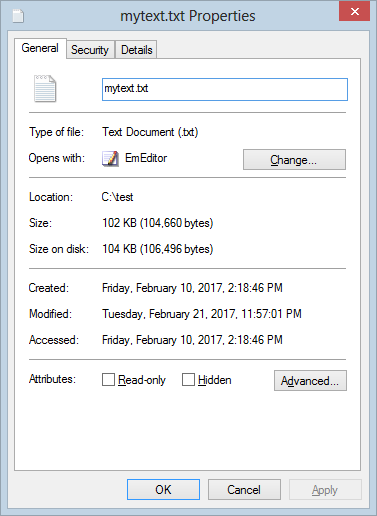
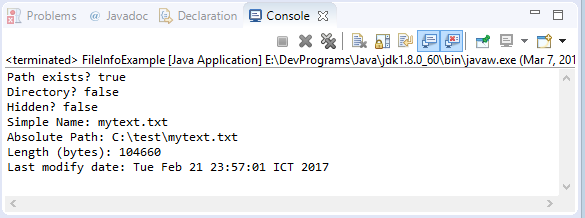
3. Create Directory
The File class provides 2 methods to create a directory:
Method | Description |
public boolean mkdir() | Create directory given by path. Note directory is only created if the parent directory exists. |
public boolean mkdirs() | Create the directory given by the path, including the parent directory if it does not exist. |
MakeDirExample.java
package org.o7planning.filedirectory;
import java.io.File;
public class MakeDirExample {
public static void main(String[] args) {
File dir1 = new File("C:/test2/test3");
System.out.println("Pathname: " + dir1.getAbsolutePath());
System.out.println("Path Exists? " + dir1.exists());
System.out.println("Parent Path Exists? " + dir1.getParentFile().exists());
// With mkdir(), the directory only created if parent directory exists.
boolean created = dir1.mkdir();
System.out.println("Directory created? " + created);
System.out.println("--------------------");
File dir2 = new File("C:/test2/test3/test4");
System.out.println("Pathname: " + dir2.getAbsolutePath());
System.out.println("Exists? " + dir2.exists());
//
created = dir1.mkdirs();
System.out.println("Directory created? " + created);
}
}
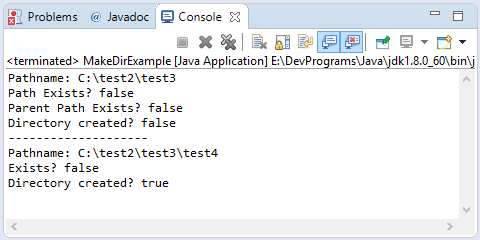
4. File Filter
The java.io.File provide some methods to retrieve subfiles and subdirectories in a directory. And use FileFilter to filer these files.
Phương thức | Mô tả |
static File[] listRoots() | Returns an array of File objects that represent the root directories. In Windows it will be the drives (C :, :D, ..), in Unix it is / |
File[] listFiles() | The method returns an array of pathnames for files and directories in the current directory. |
File[] listFiles(FilenameFilter filter) | The method returns an array of pathnames for files and directories in the current directory, and match the FilenameFilter filter on the parameter. |
File[] listFiles(FileFilter filter) | The method returns an array of pathnames for files and directories in the current directory, and match the FileFilter filter on the parameter. |
String[] list() | The method returns an array of paths for files and directories in the current directory. |
String[] list(FilenameFilter filter) | The method returns an array of paths for files and directories in the current directory, and match the FilenameFilter filter on the parameter. |
For example, list all of the root directory:
RootFilesExample.java
package org.o7planning.filedirectory;
import java.io.File;
public class RootFilesExample {
public static void main(String[] args) {
File[] roots = File.listRoots();
for(File root: roots) {
System.out.println(root.getAbsolutePath());
}
}
}
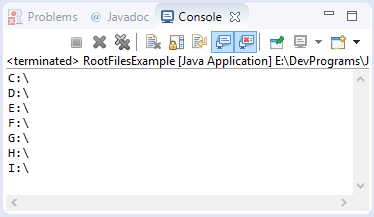
The example below lists all direct subfiles and subdirectories of a directory:
FileListExample.java
package org.o7planning.filedirectory;
import java.io.File;
public class FileListExample {
public static void main(String[] args) {
System.out.println("File[] listFiles():\n");
File dir = new File("C:/test");
File[] children = dir.listFiles();
for (File file : children) {
System.out.println(file.getAbsolutePath());
}
System.out.println("\n-----------------------");
System.out.println("String[] list():\n");
String[] paths = dir.list();
for (String path : paths) {
System.out.println(path);
}
}
}
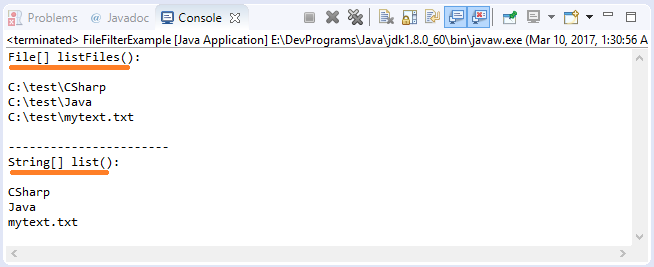
java.io.FileFilter
** FileFilter **
package java.io;
@FunctionalInterface
public interface FileFilter {
boolean accept(File pathname);
}
The simple example below uses FileFilter to filter out files in a directory with the "txt" extension:
TxtFileFilter.java
package org.o7planning.filedirectory;
import java.io.File;
import java.io.FileFilter;
public class TxtFileFilter implements FileFilter {
// Only accept 'pathname' as the file and extension is txt.
@Override
public boolean accept(File pathname) {
if (!pathname.isFile()) {
return false;
}
if (pathname.getAbsolutePath().endsWith(".txt")) {
return true;
}
return false;
}
}
FileFilterExample.java
package org.o7planning.filedirectory;
import java.io.File;
public class FileFilterExample {
public static void main(String[] args) {
File dir = new File("C:/test");
File[] txtFiles = dir.listFiles(new TxtFileFilter());
for (File txtFile : txtFiles) {
System.out.println(txtFile.getAbsolutePath());
}
}
}
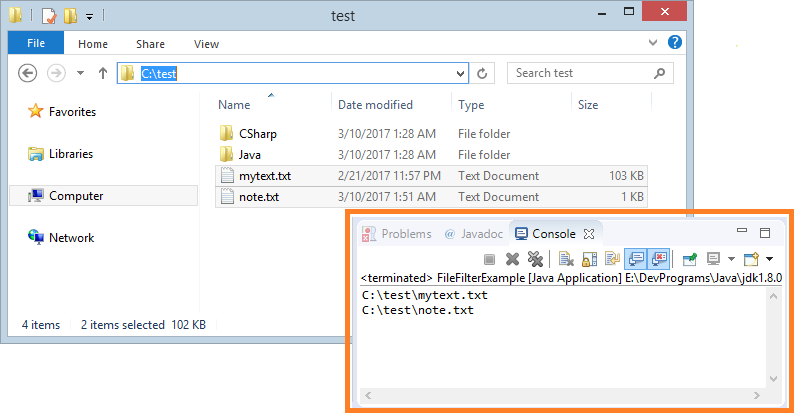
java.io.FilenameFilter
** FilenameFilter **
package java.io;
@FunctionalInterface
public interface FilenameFilter {
/**
* Tests if a specified file should be included in a file list.
*
* @param dir the directory in which the file was found.
* @param name the name of the file.
* @return <code>true</code> if and only if the name should be
* included in the file list; <code>false</code> otherwise.
*/
boolean accept(File dir, String name);
}
The example of using FilenameFilter:
TxtFilenameFilter.java
package org.o7planning.filedirectory;
import java.io.File;
import java.io.FilenameFilter;
public class TxtFilenameFilter implements FilenameFilter {
// Accept paths end withs '.txt'
@Override
public boolean accept(File dir, String name) {
if (name.endsWith(".txt")) {
return true;
}
return false;
}
}
FilenameFilterExample.java
package org.o7planning.filedirectory;
import java.io.File;
public class FilenameFilterExample {
public static void main(String[] args) {
File dir = new File("C:/test");
File[] txtFiles = dir.listFiles(new TxtFilenameFilter());
for (File txtFile : txtFiles) {
System.out.println(txtFile.getAbsolutePath());
}
}
}
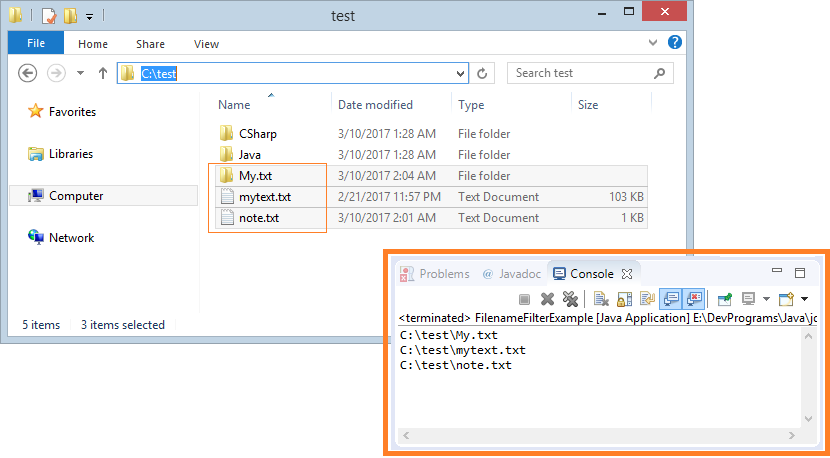
5. Listing Files
The java.io.File class provides you with some methods to list directories and files are "direct children" of the current directory. The example below use recursion to list all descendant directories and descendant files of a directory.
RecursiveFileExample.java
package org.o7planning.filedirectory;
import java.io.File;
public class RecursiveFileExample {
private void fetchChild(File file) {
System.out.println(file.getAbsolutePath());
if (file.isDirectory()) {
File[] children = file.listFiles();
for (File child : children) {
// Recursive
this.fetchChild(child);
}
}
}
public static void main(String[] args) {
RecursiveFileExample example = new RecursiveFileExample();
File dir = new File("C:/test");
example.fetchChild(dir);
}
}
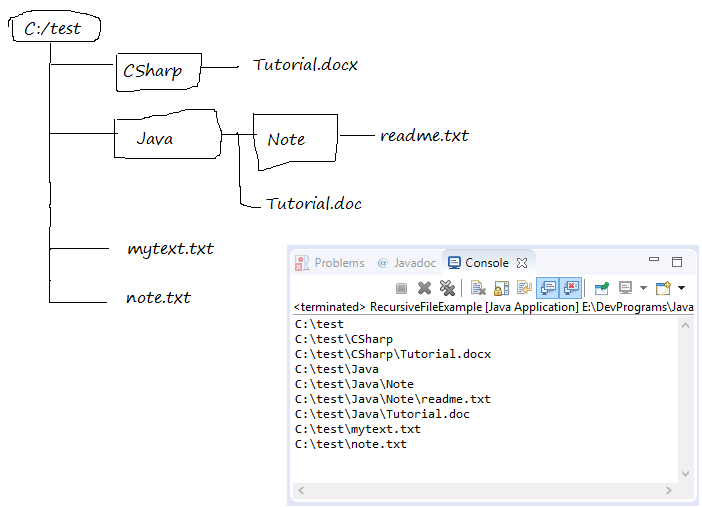
6. Rename
When using the rename() method of the File class, you can change name (Or the path) of a file or a directory. If you change the path, make sure that your new parent directory has already existed.
Rename
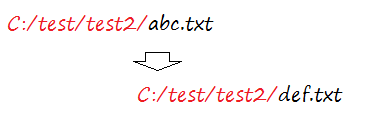
The example below renames a file (Or directory) but does not change the parent paths.
SimpleRenameExample.java
package org.o7planning.filedirectory;
import java.io.File;
public class SimpleRenameExample {
public static void main(String[] args) {
File srcFile = new File("C:/test/test2/abc.txt");
File destFile = new File("C:/test/test2/def.txt");
boolean renamed = srcFile.renameTo(destFile);
System.out.println("Renamed: "+ renamed);
}
}
Renaming includes the parent path:
Renaming and changing the parent path are similar to the "Cut" action of a file or a directory to another directory and then renaming it.
Note: For Windows OS, the File.rename() method will not work if you change from the path to another drive.
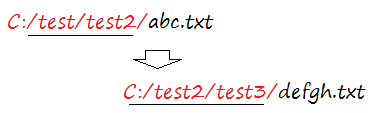
RenameAndPathExample.java
package org.o7planning.filedirectory;
import java.io.File;
public class RenameAndPathExample {
public static void main(String[] args) {
// Source file.
File srcFile = new File("C:/test/test2/abc.txt");
// Destination file.
File destFile = new File("C:/test2/test3/defgh.txt");
if (!srcFile.exists()) {
System.out.println("Src File doest not exists");
return;
}
// Create parent directory of target file.
destFile.getParentFile().mkdirs();
boolean renamed = srcFile.renameTo(destFile);
System.out.println("Renamed: " + renamed);
}
}
Java Basic
- Customize java compiler processing your Annotation (Annotation Processing Tool)
- Java Programming for team using Eclipse and SVN
- Java WeakReference Tutorial with Examples
- Java PhantomReference Tutorial with Examples
- Java Compression and Decompression Tutorial with Examples
- Configuring Eclipse to use the JDK instead of JRE
- Java String.format() and printf() methods
- Syntax and new features in Java 8
- Java Regular Expressions Tutorial with Examples
- Java Multithreading Programming Tutorial with Examples
- JDBC Driver Libraries for different types of database in Java
- Java JDBC Tutorial with Examples
- Get the values of the columns automatically increment when Insert a record using JDBC
- Java Stream Tutorial with Examples
- Java Functional Interface Tutorial with Examples
- Introduction to the Raspberry Pi
- Java Predicate Tutorial with Examples
- Abstract class and Interface in Java
- Access modifiers in Java
- Java Enums Tutorial with Examples
- Java Annotations Tutorial with Examples
- Comparing and Sorting in Java
- Java String, StringBuffer and StringBuilder Tutorial with Examples
- Java Exception Handling Tutorial with Examples
- Java Generics Tutorial with Examples
- Manipulating files and directories in Java
- Java BiPredicate Tutorial with Examples
- Java Consumer Tutorial with Examples
- Java BiConsumer Tutorial with Examples
- What is needed to get started with Java?
- History of Java and the difference between Oracle JDK and OpenJDK
- Install Java on Windows
- Install Java on Ubuntu
- Install OpenJDK on Ubuntu
- Install Eclipse
- Install Eclipse on Ubuntu
- Quick Learning Java for beginners
- History of bits and bytes in computer science
- Data Types in java
- Bitwise Operations
- if else statement in java
- Switch Statement in Java
- Loops in Java
- Arrays in Java
- JDK Javadoc in CHM format
- Inheritance and polymorphism in Java
- Java Function Tutorial with Examples
- Java BiFunction Tutorial with Examples
- Example of Java encoding and decoding using Apache Base64
- Java Reflection Tutorial with Examples
- Java remote method invocation - Java RMI Tutorial with Examples
- Java Socket Programming Tutorial with Examples
- Which Platform Should You Choose for Developing Java Desktop Applications?
- Java Commons IO Tutorial with Examples
- Java Commons Email Tutorial with Examples
- Java Commons Logging Tutorial with Examples
- Understanding Java System.identityHashCode, Object.hashCode and Object.equals
- Java SoftReference Tutorial with Examples
- Java Supplier Tutorial with Examples
- Java Aspect Oriented Programming with AspectJ (AOP)
Show More
- Java Servlet/Jsp Tutorials
- Java Collections Framework Tutorials
- Java API for HTML & XML
- Java IO Tutorials
- Java Date Time Tutorials
- Spring Boot Tutorials
- Maven Tutorials
- Gradle Tutorials
- Java Web Services Tutorials
- Java SWT Tutorials
- JavaFX Tutorials
- Java Oracle ADF Tutorials
- Struts2 Framework Tutorials
- Spring Cloud Tutorials