Abstract class and Interface in Java
1. Introduction
In this document, I will instruct you in Interface and Abstract Class, also analyze the similarities and differences between them.
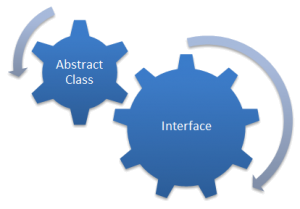
2. Abstract Class
Abstract class. for example:
// This is an abstract class.
// It must be declared as abstract because it has an abstract method.
public abstract class ClassA {
// This is an abstract method
// It has no body.
// Its access modifier is public
public abstract void doSomething();
// The access modifier of this method is protected.
protected abstract String doNothing();
// This method does not declare access modifier
// It has the default access modifier.
abstract void todo() ;
}
// This is an abstract class.
// It is declared as abstract,
// though it does not have any abstract methods.
public abstract class ClassB {
}
Characteristics of an abstract class is:
- It is declared abstract.
- It can declare 0, 1 or more of inside abstract methods.
- Unable to initialize one object directly from an abstract class.
3. Abstract class, the examples
See illustration:
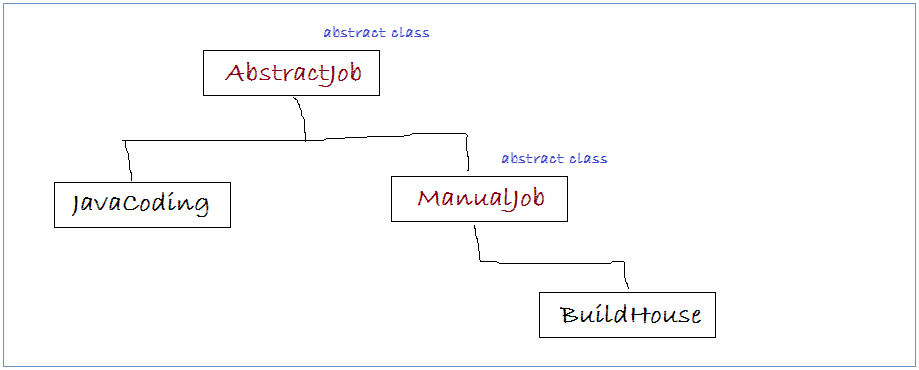
AbstractJob.java
package org.o7planning.tutorial.abs;
// An abstract class (Simulating a job).
// There are two methods declared abstract.
public abstract class AbstractJob {
public AbstractJob() {
}
// This is an abstract method.
// return Job Name.
public abstract String getJobName();
// This is an abstract method.
public abstract void doJob();
}
JavaCoding.java
package org.o7planning.tutorial.abs;
// Class implements all abstract methods of the parent class.
public class JavaCoding extends AbstractJob {
public JavaCoding() {
}
// Implements abstract method of parent class.
@Override
public void doJob() {
System.out.println("Coding Java...");
}
// Implements abstract method of parent.
// Method with body.
// Return name of Job.
@Override
public String getJobName() {
return "Coding Java";
}
}
ManualJob.java
package org.o7planning.tutorial.abs;
// AbstractJob class has two abstract methods.
// This class implements only one abstract method of parent class.
// Therefore it must be declared as abstract.
public abstract class ManualJob extends AbstractJob {
public ManualJob() {
}
// Implements abstract method of parent class.
@Override
public String getJobName() {
return "Manual Job";
}
}
BuildHouse.java
package org.o7planning.tutorial.abs;
// This class inherited from ManualJob
// BuildHouse is not declared as abstract.
// so it must implements all remaining abstract methods.
public class BuildHouse extends ManualJob {
public BuildHouse() {
}
// Implements abstract methods of the parent class.
@Override
public void doJob() {
System.out.println("Build a House");
}
}
Demo
JobDemo.java
package org.o7planning.tutorial.abs;
public class JobDemo {
public static void main(String[] args) {
// Create AbstractJob object
// from Constructor of JavaCoding.
AbstractJob job1 = new JavaCoding();
// Call doJob() method.
job1.doJob();
// getJobName is the abstract method in AbstractJob class.
// But it was implemented in a certain subclass (JavaCoding).
// So can call it.
String jobName = job1.getJobName();
System.out.println("Job Name 1= " + jobName);
// Create AbstractJob object
// from constructor of BuildHouse.
AbstractJob job2 = new BuildHouse();
job2.doJob();
String jobName2 = job2.getJobName();
System.out.println("Job Name 2= " + jobName2);
}
}
Running the example:
Coding Java...
Job Name 1= Coding Java
Build a House
Job Name 2= Manual Job
4. Overview of the interface
We know that a class can extends from only one parent class.
// B is subclass of class A, or in other words B is extended from A.
// Java only allows a class to extends from only one another class.
public class B extends A {
// ....
}
// In the absence of specifying class B extends from a particular class.
// By default, understand that B extends from the Object class.
public class B {
}
// This declaration, and the above is equivalent.
public class B extends Object {
}
But a class can extend from multiple Interfaces.
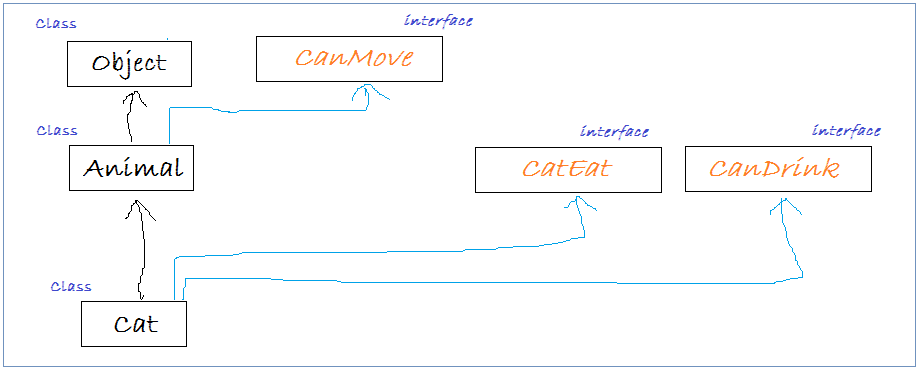
// A class can extends from only one parent class.
// But can implements (extends) from multiple Interfaces.
public class Cat extends Animal implements CanEat, CanDrink {
// ....
}
Các đặc điểm của interface
- Interface always has modifier: public interface, whether you declare clearly or not.
- If there are fields, they are: public static final, whether you declare clearly or not.
- Its methods are abstract method, ie without function body, and contains modifier is: public abstract, whether you declare or not.
- Interface have no Constructor.
5. Structure of an Interface
NoAccessModifierInterface.java
package org.o7planning.tutorial.itf;
// This is an interface not specified access modifier.
// Its access modifier is default.
// Only classes in the same package can implements this interface.
interface NoAccessModifierInterface {
}
CanMove.java
package org.o7planning.tutorial.itf;
// This interface defines a standard,
// about things capable of moving.
public interface CanMove {
// The methods in Interface are always abstract and public.
public abstract void run();
// Even if you do not specify 'public abstract', java always understands.
void back();
// Velocity.
public int getVelocity();
}
CanDrink.java
package org.o7planning.tutorial.itf;
// This interface defines a standard,
// about things capable of drinking.
public interface CanDrink {
// Fields in Interface are always 'public static final'.
// Whether you declare it or not.
public static final String PEPSI = "PEPSI";
final String NUMBER_ONE = "NUMBER ONE";
String SEVENUP = "SEVEN UP";
public void drink();
}
CanEat.java
package org.o7planning.tutorial.itf;
// This interface defines a standard,
// about things capable of eating.
public interface CanEat {
public void eat();
}
6. Class implements Interface
Animal.java
package org.o7planning.tutorial.cls;
import org.o7planning.tutorial.itf.CanMove;
// This class extends from Object.
// And implements CanMove interface.
// CanMove has 3 abstract methods.
// This class implements only one abstract method of CanMove.
// Therefore it must be declared as abstract.
// The remaining abstract methods to be implemented in the subclasses.
public abstract class Animal implements CanMove {
// Implements run() method of CanMove.
@Override
public void run() {
System.out.println("Animal run...");
}
}
Cat.java
package org.o7planning.tutorial.cls;
import org.o7planning.tutorial.itf.CanDrink;
import org.o7planning.tutorial.itf.CanEat;
// This class extends Animal and implements CanEat, CanDrink interfaces.
// This class is not declared as abstract.
// So it must implements all abstract methods of the interfaces.
public class Cat extends Animal implements CanEat, CanDrink {
private String name;
public Cat(String name) {
this.name = name;
}
public String getName() {
return this.name;
}
// Implements method of CanMove
@Override
public void back() {
System.out.println(name + " cat back ...");
}
// Implements method of CanMove.
@Override
public int getVelocity() {
return 110;
}
// Implements method of CanEat.
@Override
public void eat() {
System.out.println(name + " cat eat ...");
}
// Implements method of CanDrink.
@Override
public void drink() {
System.out.println(name + " cat drink ...");
}
}
Mouse.java
package org.o7planning.tutorial.cls;
import org.o7planning.tutorial.itf.CanDrink;
import org.o7planning.tutorial.itf.CanEat;
public class Mouse extends Animal implements CanEat, CanDrink {
@Override
public void back() {
System.out.println("Mouse back ...");
}
@Override
public int getVelocity() {
return 85;
}
@Override
public void drink() {
System.out.println("Mouse drink ...");
}
@Override
public void eat() {
System.out.println("Mouse eat ...");
}
}
AnimalDemo.java
package org.o7planning.tutorial.cls;
import org.o7planning.tutorial.itf.CanEat;
public class AnimalDemo {
public static void main(String[] args) {
// Inherit static field from CanDrink interface.
System.out.println("Drink " + Cat.SEVENUP);
// Create CanEat object
// via constructor of Cat.
CanEat canEat1 = new Cat("Tom");
// An object declared as CanEat.
// But in fact is Mouse.
CanEat canEat2 = new Mouse();
// Polymorphism shown here.
// Java know the actual types of objects.
// ==> Tom cat eat ...
canEat1.eat();
// ==> Mouse eat ...
canEat2.eat();
boolean isCat = canEat1 instanceof Cat;
System.out.println("catEat1 is Cat? " + isCat);
// Cast
if (canEat2 instanceof Mouse) {
Mouse mouse = (Mouse) canEat2;
// Call drink method (Inherited from CanDrink).
mouse.drink();
}
}
}
Running the example:
Drink SEVEN UP
Tom cat eat ...
Mouse eat ...
catEat1 is Cat? true
Mouse drink ...
Java Basic
- Customize java compiler processing your Annotation (Annotation Processing Tool)
- Java Programming for team using Eclipse and SVN
- Java WeakReference Tutorial with Examples
- Java PhantomReference Tutorial with Examples
- Java Compression and Decompression Tutorial with Examples
- Configuring Eclipse to use the JDK instead of JRE
- Java String.format() and printf() methods
- Syntax and new features in Java 8
- Java Regular Expressions Tutorial with Examples
- Java Multithreading Programming Tutorial with Examples
- JDBC Driver Libraries for different types of database in Java
- Java JDBC Tutorial with Examples
- Get the values of the columns automatically increment when Insert a record using JDBC
- Java Stream Tutorial with Examples
- Java Functional Interface Tutorial with Examples
- Introduction to the Raspberry Pi
- Java Predicate Tutorial with Examples
- Abstract class and Interface in Java
- Access modifiers in Java
- Java Enums Tutorial with Examples
- Java Annotations Tutorial with Examples
- Comparing and Sorting in Java
- Java String, StringBuffer and StringBuilder Tutorial with Examples
- Java Exception Handling Tutorial with Examples
- Java Generics Tutorial with Examples
- Manipulating files and directories in Java
- Java BiPredicate Tutorial with Examples
- Java Consumer Tutorial with Examples
- Java BiConsumer Tutorial with Examples
- What is needed to get started with Java?
- History of Java and the difference between Oracle JDK and OpenJDK
- Install Java on Windows
- Install Java on Ubuntu
- Install OpenJDK on Ubuntu
- Install Eclipse
- Install Eclipse on Ubuntu
- Quick Learning Java for beginners
- History of bits and bytes in computer science
- Data Types in java
- Bitwise Operations
- if else statement in java
- Switch Statement in Java
- Loops in Java
- Arrays in Java
- JDK Javadoc in CHM format
- Inheritance and polymorphism in Java
- Java Function Tutorial with Examples
- Java BiFunction Tutorial with Examples
- Example of Java encoding and decoding using Apache Base64
- Java Reflection Tutorial with Examples
- Java remote method invocation - Java RMI Tutorial with Examples
- Java Socket Programming Tutorial with Examples
- Which Platform Should You Choose for Developing Java Desktop Applications?
- Java Commons IO Tutorial with Examples
- Java Commons Email Tutorial with Examples
- Java Commons Logging Tutorial with Examples
- Understanding Java System.identityHashCode, Object.hashCode and Object.equals
- Java SoftReference Tutorial with Examples
- Java Supplier Tutorial with Examples
- Java Aspect Oriented Programming with AspectJ (AOP)
Show More
- Java Servlet/Jsp Tutorials
- Java Collections Framework Tutorials
- Java API for HTML & XML
- Java IO Tutorials
- Java Date Time Tutorials
- Spring Boot Tutorials
- Maven Tutorials
- Gradle Tutorials
- Java Web Services Tutorials
- Java SWT Tutorials
- JavaFX Tutorials
- Java Oracle ADF Tutorials
- Struts2 Framework Tutorials
- Spring Cloud Tutorials