Inheritance and polymorphism in Java
1. Introduction
Inheritance and polymorphism - this is a very important concept in Java. You must understand it better if you want to learn java.
2. Class, Constructor and instance
You need to understand explicitly about class, constructor and instance before starting to learn inheritance in java. We consider the class Person, describes a person with information full name, year of birth, place of birth.
Person.java
package org.o7planning.tutorial.inheritance.basic;
public class Person {
// Field name - name of person.
private String name;
// Field bornYear
private Integer bornYear;
// Field placeOfBirth.
private String placeOfBirth;
// Constructor has 3 parameters.
// The aim is to assign values to the fields of Person.
// Specify the name, year of birth, place of birth of a person.
public Person(String name, Integer bornYear, String placeOfBirth) {
this.name = name;
this.bornYear = bornYear;
this.placeOfBirth = placeOfBirth;
}
// Constructor has 2 parameters.
// The aim is to assign values to the name, born year fileds of the Person.
// The place of birth is not assigned.
public Person(String name, Integer bornYear) {
this.name = name;
this.bornYear = bornYear;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Integer getBornYear() {
return bornYear;
}
public void setBornYear(Integer bornYear) {
this.bornYear = bornYear;
}
public String getPlaceOfBirth() {
return placeOfBirth;
}
public void setPlaceOfBirth(String placeOfBirth) {
this.placeOfBirth = placeOfBirth;
}
}
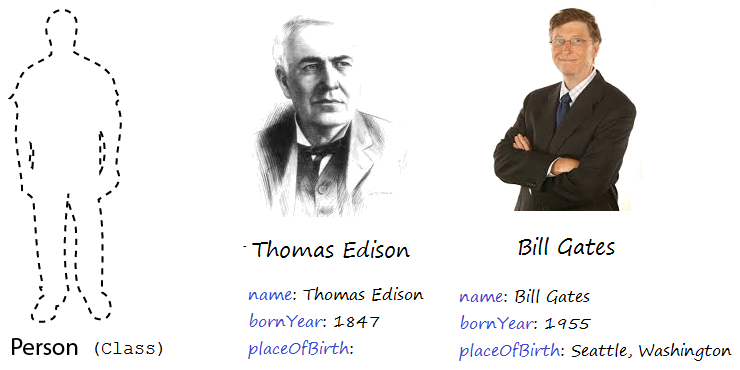
PersonDemo.java
package org.o7planning.tutorial.inheritance.basic;
public class PersonDemo {
public static void main(String[] args) {
// Instance: Thomas Edison.
Person edison = new Person("Thomas Edison", 1847);
System.out.println("Info:");
System.out.println("Name: " + edison.getName());
System.out.println("Born Year: " + edison.getBornYear());
System.out.println("Place Of Birth: " + edison.getPlaceOfBirth() );
// Instance: Bill Gates
Person billGates = new Person("Bill Gate", 1955, "Seattle, Washington");
System.out.println("Info:");
System.out.println("Name: " + billGates.getName());
System.out.println("Born Year: " + billGates.getBornYear());
System.out.println("Place Of Birth: " + billGates.getPlaceOfBirth());
}
}
Distinguishing Class,constructor and instance:
Person simulate a class of people, it is something abstract, but it has information, in the example above, information is the name, year of birth, place of birth.
Constructor
Constructor
- Constructor always have the same name as the class
- One class has one or more constructors.
- Constructor with or without parameters, no parameters constructor called default constructor.
- Constructor using for create a instance of class.
See example, create a object (instance) from Constructor.
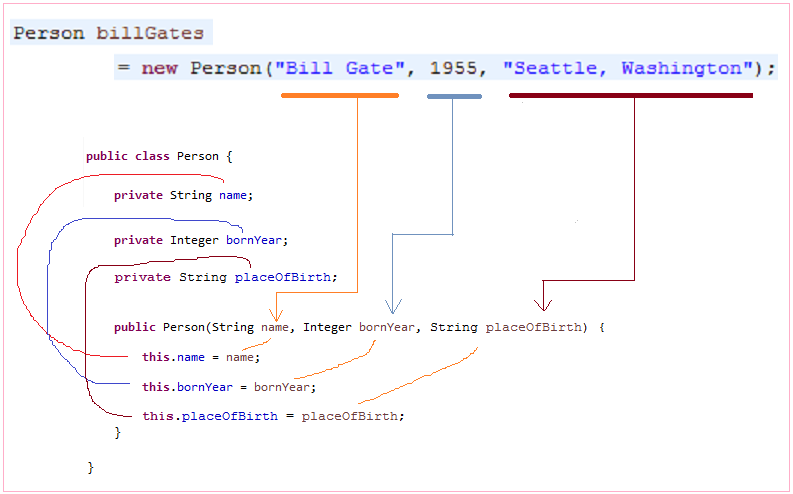
3. Inheritance in java
We need a few classes to participate in examples.
- Animal: Class simulate an animal.
- Duck: Subclass of Animal.
- Cat: Subclass of Animal.
- Mouse: Subclass of Animal.
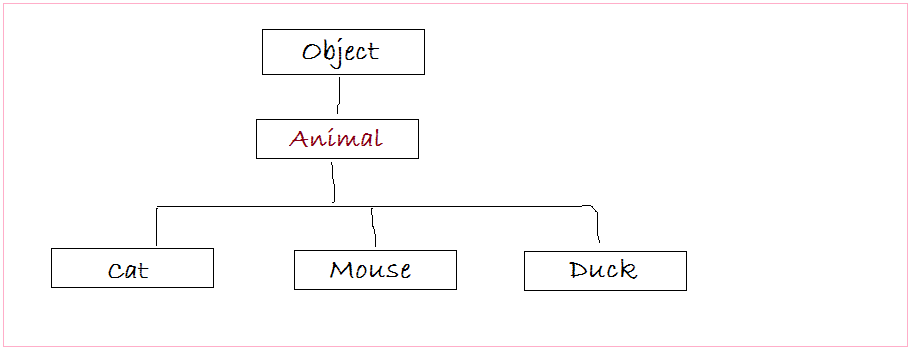
Here we have the Animal class, it has a method without body.
This method is an abstract method, in the subclass you need to declare and implement its body.
Animal class has at least one abstract method, it must be declared as abstract. Class abstract has constructor, but you can not create instance from it.
- public abstract String getAnimalName();
This method is an abstract method, in the subclass you need to declare and implement its body.
Animal class has at least one abstract method, it must be declared as abstract. Class abstract has constructor, but you can not create instance from it.
- In essence means that you want to create an object of animal, you need to create from a specific animal species, in this case, you must create from constructor of Cat, Mouse or Duck.
Animal.java
package org.o7planning.tutorial.inheritance.animal;
// The class has at least one abstract method must be declared as abstract.
public abstract class Animal {
// The Name, for example, Tom Cat, Jerry Mouse.
private String name;
// Default constructor.
public Animal() {
// Assign the default value to 'name'.
this.name = this.getAnimalName();
}
public Animal(String name) {
this.name = name;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
// This is an abstract method.
// Returns the name of this animal.
// The specific content of this method will be implemented in the subclass.
public abstract String getAnimalName();
}
Next see Cat class, inherited from Animal.
Cat also has constructor, and also has fields. In the first line of the constructor must call super(..), means that call to constructor of parent class, to assign value for fields of the parent class.
If you do not call call super(..), java auto insert super() into first line of constructor at compile time.
If you do not call call super(..), java auto insert super() into first line of constructor at compile time.
Cat.java
package org.o7planning.tutorial.inheritance.animal;
public class Cat extends Animal {
private int age;
private int height;
public Cat(int age, int height) {
// Call the default Constructor of the parent class (Animal).
// For the purpose of assigning values to fields of superclass.
super();
// Next, Assign values to its fields.
this.age = age;
this.height = height;
}
public Cat(String name, int age, int height) {
// Call the default Constructor of the parent class (Animal)
// For the purpose of assigning values to fields of superclass.
super(name);
// Next, Assign values to its fields.
this.age = age;
this.height = height;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public int getHeight() {
return height;
}
public void setHeight(int height) {
this.height = height;
}
// Implement content for abstract method declared in the parent class.
@Override
public String getAnimalName() {
return "Cat";
}
}
When you create a Cat object, what happens?
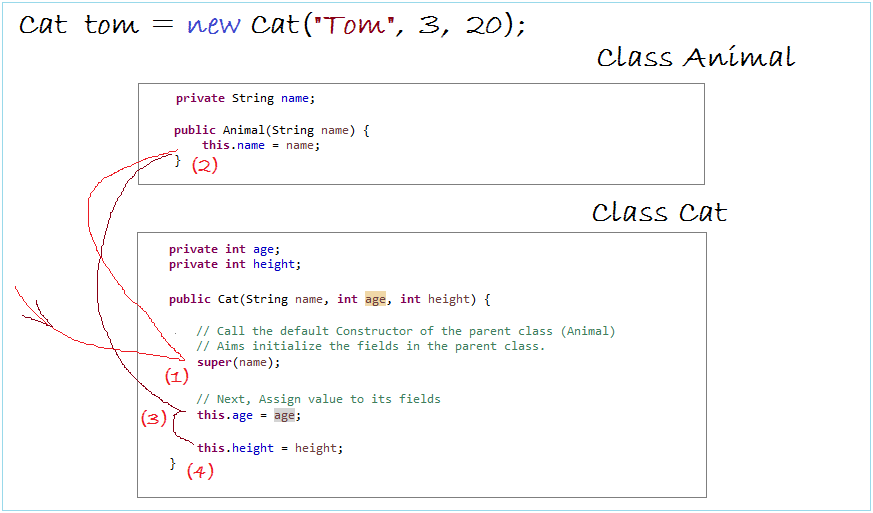
View Mouse class, subclass of Animal.
Mouse.java
package org.o7planning.tutorial.inheritance.animal;
public class Mouse extends Animal {
private int weight;
// Default constructor.
public Mouse() {
// Gọi tới cấu tử Mouse(int)
// Call to constructor Mouse(int)
this(100);
}
// The Constructor has one parameter.
public Mouse(int weight) {
// If you do not call any super (..).
// Java will call a default super().
// super();
this.weight = weight;
}
// The Constructor has 2 parameters.
public Mouse(String name, int weight) {
super(name);
this.weight = weight;
}
public int getWeight() {
return weight;
}
public void setWeight(int weight) {
this.weight = weight;
}
@Override
public String getAnimalName() {
return "Mouse";
}
}
InstanceofDemo.java
package org.o7planning.tutorial.inheritance.demo;
import org.o7planning.tutorial.inheritance.animal.Animal;
import org.o7planning.tutorial.inheritance.animal.Cat;
import org.o7planning.tutorial.inheritance.animal.Mouse;
public class InstanceofDemo {
public static void main(String[] args) {
// Create an Animal object.
// Animal is abstract class,
// you can not create an object from Animal's Constructor.
Animal tom = new Cat("Tom", 3, 20);
System.out.println("name: " + tom.getName());
System.out.println("animalName: " + tom.getAnimalName());
// Using 'instanceof' operator to
// check if an object is an instance of a specific class.
boolean isMouse = tom instanceof Mouse;
System.out.println("Tom is mouse? " + isMouse);
boolean isCat = tom instanceof Cat;
System.out.println("Tom is cat? " + isCat);
boolean isAnimal = tom instanceof Animal;
System.out.println("Tom is animal? " + isAnimal);
}
}
Results of running the example:
name: Tom
animalName: Cat
Tom is mouse? false
Tom is cat? true
Tom is animal? true
InheritMethodDemo.java
package org.o7planning.tutorial.inheritance.demo;
import org.o7planning.tutorial.inheritance.animal.Cat;
public class InheritMethodDemo {
public static void main(String[] args) {
// Create instance of Cat.
Cat tom = new Cat("Tom", 3, 20);
// Call the methods inherited from the parent class (Animal).
System.out.println("name: " + tom.getName());
System.out.println("animalName: " + tom.getAnimalName());
System.out.println("-----------------");
// Call the methods declared in the Cat class.
System.out.println("Age: " + tom.getAge());
System.out.println("Height: " + tom.getHeight());
}
}
Results of running the example:
name: Tom
animalName: Cat
-----------------
Age: 3
Height: 20
Casting in Java
CastDemo.java
package org.o7planning.tutorial.inheritance.demo;
import java.util.Random;
import org.o7planning.tutorial.inheritance.animal.Animal;
import org.o7planning.tutorial.inheritance.animal.Cat;
import org.o7planning.tutorial.inheritance.animal.Mouse;
public class CastDemo {
// This method returns a random animal.
public static Animal getRandomAnimal() {
// Returns a random value 0 or 1.
int random = new Random().nextInt(2);
Animal animal = null;
if (random == 0) {
animal = new Cat("Tom", 3, 20);
} else {
animal = new Mouse("Jerry", 5);
}
return animal;
}
public static void main(String[] args) {
Animal animal = getRandomAnimal();
if (animal instanceof Cat) {
// Cast to Cat
Cat cat = (Cat) animal;
// And call a method of the Cat class.
System.out.println("Cat height: " + cat.getHeight());
} else if (animal instanceof Mouse) {
// Cast to Mouse.
Mouse mouse = (Mouse) animal;
// And call method of the Mouse class.
System.out.println("Mouse weight: " + mouse.getWeight());
}
}
}
4. Polymorphism in Java
You have a cat Asian Cat, you can call it is a Cat or call it is an Animal which is an aspect of the polymorphism.
Or another example: On your resume records that you're an Asian man, while you actually are a Vietnamese.
The example below shows you how to behave between declaring and reality
Or another example: On your resume records that you're an Asian man, while you actually are a Vietnamese.
The example below shows you how to behave between declaring and reality
AsianCat is a subclass of the class Cat.
AsianCat.java
package org.o7planning.tutorial.inheritance.animal;
public class AsianCat extends Cat {
public AsianCat(String name, int age, int height) {
super(name, age, height);
}
// Override the method of the parent class (Cat)
@Override
public String getAnimalName() {
return "Asian Cat";
}
}
Polymorphism of Java is described in the following illustration:
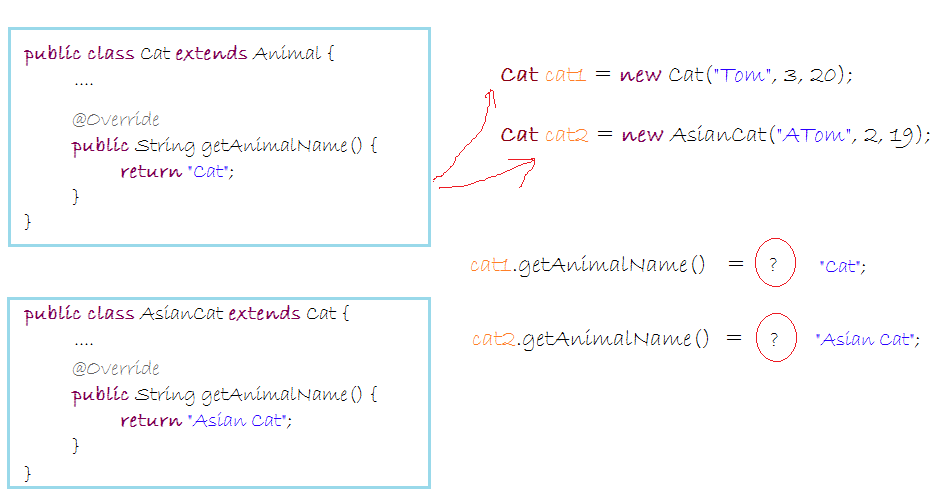
PolymorphismDemo.java
package org.o7planning.tutorial.polymorphism;
import org.o7planning.tutorial.inheritance.animal.AsianCat;
import org.o7planning.tutorial.inheritance.animal.Cat;
public class PolymorphismDemo {
public static void main(String[] args) {
Cat cat1 = new Cat("Tom", 3, 20);
Cat cat2 = new AsianCat("ATom", 2, 19);
System.out.println("Animal Name of cat1: " + cat1.getAnimalName());
System.out.println("Animal Name of cat2: " + cat2.getAnimalName());
}
}
Results of running the example:
Animal Name of cat1: Cat
Animal Name of cat2: Asian Cat
Java Basic
- Customize java compiler processing your Annotation (Annotation Processing Tool)
- Java Programming for team using Eclipse and SVN
- Java WeakReference Tutorial with Examples
- Java PhantomReference Tutorial with Examples
- Java Compression and Decompression Tutorial with Examples
- Configuring Eclipse to use the JDK instead of JRE
- Java String.format() and printf() methods
- Syntax and new features in Java 8
- Java Regular Expressions Tutorial with Examples
- Java Multithreading Programming Tutorial with Examples
- JDBC Driver Libraries for different types of database in Java
- Java JDBC Tutorial with Examples
- Get the values of the columns automatically increment when Insert a record using JDBC
- Java Stream Tutorial with Examples
- Java Functional Interface Tutorial with Examples
- Introduction to the Raspberry Pi
- Java Predicate Tutorial with Examples
- Abstract class and Interface in Java
- Access modifiers in Java
- Java Enums Tutorial with Examples
- Java Annotations Tutorial with Examples
- Comparing and Sorting in Java
- Java String, StringBuffer and StringBuilder Tutorial with Examples
- Java Exception Handling Tutorial with Examples
- Java Generics Tutorial with Examples
- Manipulating files and directories in Java
- Java BiPredicate Tutorial with Examples
- Java Consumer Tutorial with Examples
- Java BiConsumer Tutorial with Examples
- What is needed to get started with Java?
- History of Java and the difference between Oracle JDK and OpenJDK
- Install Java on Windows
- Install Java on Ubuntu
- Install OpenJDK on Ubuntu
- Install Eclipse
- Install Eclipse on Ubuntu
- Quick Learning Java for beginners
- History of bits and bytes in computer science
- Data Types in java
- Bitwise Operations
- if else statement in java
- Switch Statement in Java
- Loops in Java
- Arrays in Java
- JDK Javadoc in CHM format
- Inheritance and polymorphism in Java
- Java Function Tutorial with Examples
- Java BiFunction Tutorial with Examples
- Example of Java encoding and decoding using Apache Base64
- Java Reflection Tutorial with Examples
- Java remote method invocation - Java RMI Tutorial with Examples
- Java Socket Programming Tutorial with Examples
- Which Platform Should You Choose for Developing Java Desktop Applications?
- Java Commons IO Tutorial with Examples
- Java Commons Email Tutorial with Examples
- Java Commons Logging Tutorial with Examples
- Understanding Java System.identityHashCode, Object.hashCode and Object.equals
- Java SoftReference Tutorial with Examples
- Java Supplier Tutorial with Examples
- Java Aspect Oriented Programming with AspectJ (AOP)
Show More
- Java Servlet/Jsp Tutorials
- Java Collections Framework Tutorials
- Java API for HTML & XML
- Java IO Tutorials
- Java Date Time Tutorials
- Spring Boot Tutorials
- Maven Tutorials
- Gradle Tutorials
- Java Web Services Tutorials
- Java SWT Tutorials
- JavaFX Tutorials
- Java Oracle ADF Tutorials
- Struts2 Framework Tutorials
- Spring Cloud Tutorials