Java Compression and Decompression Tutorial with Examples
1. Read write the zip file using java.util.zip
To compress and decompress files, JDK give you package 'java.util.zip' with some class to do this.
Unfortunately, this library can not compress and decompress the popular formats like RAR, or 7zip. For the use of the format rar, 7zip, .. you need a different library. In this document I have to refer to the library to do this.
Unfortunately, this library can not compress and decompress the popular formats like RAR, or 7zip. For the use of the format rar, 7zip, .. you need a different library. In this document I have to refer to the library to do this.
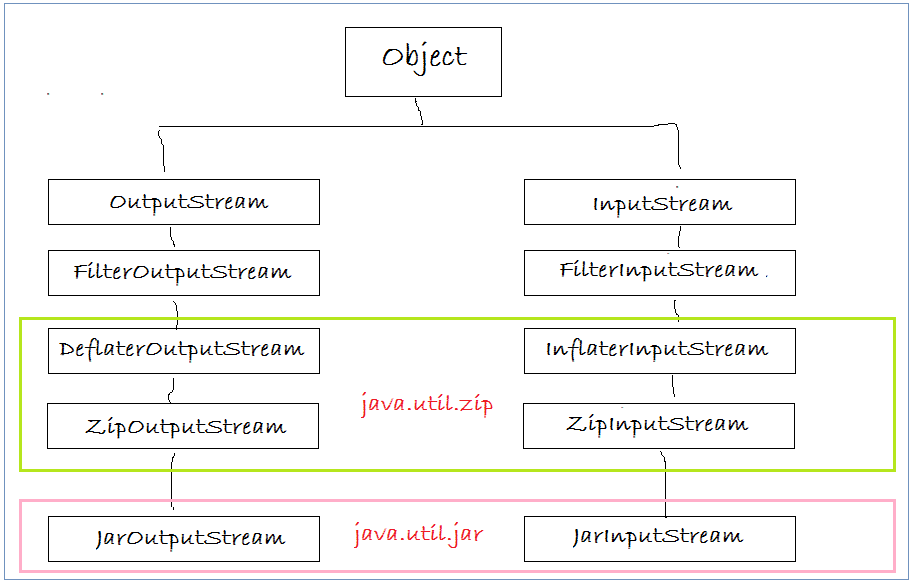
View a zip file is opened using WinRAR tool.
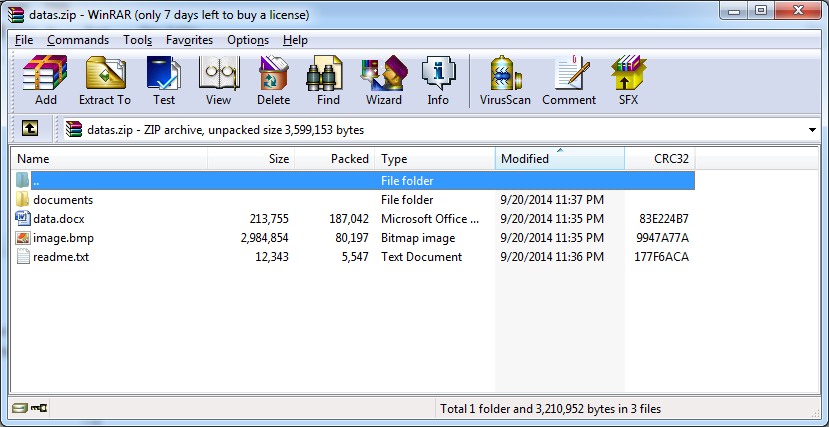
java.util.zip define files in the zip file is the ZipEntry.
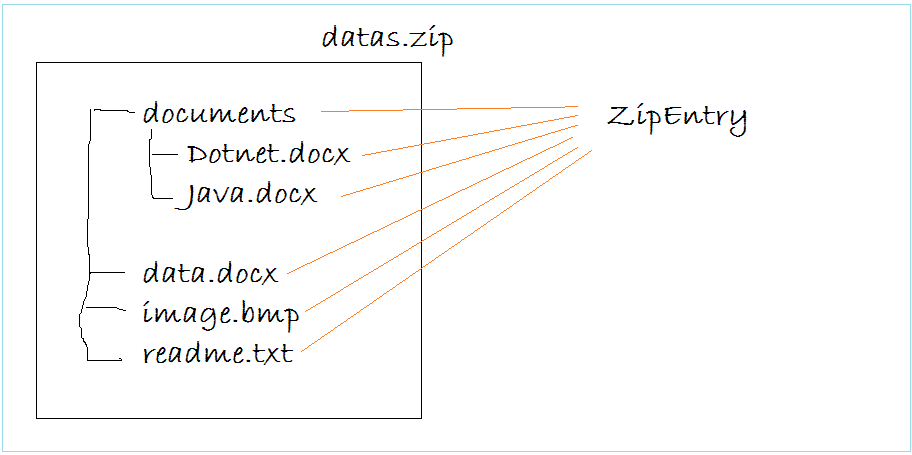
ListZipEntriesDemo.java
package org.o7planning.tutorial.javaiozip;
import java.io.FileInputStream;
import java.util.zip.ZipEntry;
import java.util.zip.ZipInputStream;
public class ListZipEntriesDemo {
public static void main(String[] args) {
String FILE_PATH = "C:/test/datas.zip";
ZipInputStream zipIs = null;
try {
// Create ZipInputStream object to read zip file.
zipIs = new ZipInputStream(new FileInputStream(FILE_PATH));
ZipEntry entry = null;
// Fetch entries (From Top to Bottom)
while ((entry = zipIs.getNextEntry()) != null) {
if (entry.isDirectory()) {
System.out.print("Directory: ");
} else {
System.out.print("File: ");
}
System.out.println(entry.getName());
}
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
zipIs.close();
} catch (Exception e) {
}
}
}
}
Results of running the example:
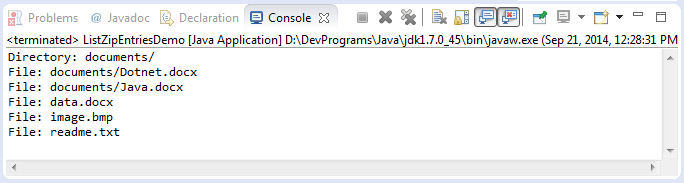
For example, unzip the zip file to a specific folder:
UnZipDemo.java
package org.o7planning.tutorial.javaiozip;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.util.zip.ZipEntry;
import java.util.zip.ZipInputStream;
public class UnZipDemo {
public static void main(String[] args) {
final String OUTPUT_FOLDER = "C:/output";
String FILE_PATH = "C:/test/datas.zip";
// Create Output folder if it does not exists.
File folder = new File(OUTPUT_FOLDER);
if (!folder.exists()) {
folder.mkdirs();
}
// Create a buffer.
byte[] buffer = new byte[1024];
ZipInputStream zipIs = null;
try {
// Create ZipInputStream object to read a file from path.
zipIs = new ZipInputStream(new FileInputStream(FILE_PATH));
ZipEntry entry = null;
// Read ever Entry (From top to bottom until the end)
while ((entry = zipIs.getNextEntry()) != null) {
String entryName = entry.getName();
String outFileName = OUTPUT_FOLDER + File.separator + entryName;
System.out.println("Unzip: " + outFileName);
if (entry.isDirectory()) {
// Make directories.
new File(outFileName).mkdirs();
} else {
// Create Stream to write file.
FileOutputStream fos = new FileOutputStream(outFileName);
int len;
// Read the data on the current entry.
while ((len = zipIs.read(buffer)) > 0) {
fos.write(buffer, 0, len);
}
fos.close();
}
}
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
zipIs.close();
} catch (Exception e) {
}
}
}
}
Results of running the example:
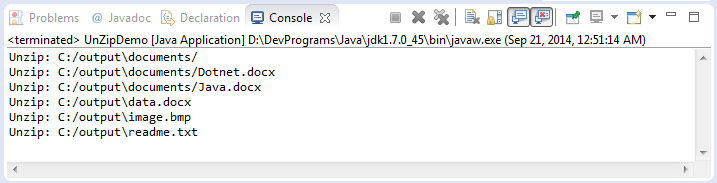
Compress a directory
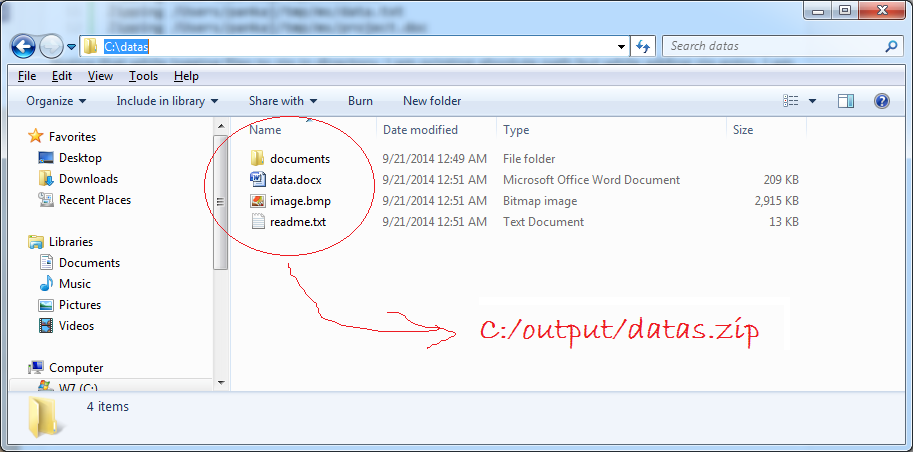
ZipDirectory.java
package org.o7planning.tutorial.javaiozip;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.OutputStream;
import java.util.ArrayList;
import java.util.List;
import java.util.zip.ZipEntry;
import java.util.zip.ZipOutputStream;
public class ZipDirectory {
public ZipDirectory() {
}
// A method to Compress a directory.
public void zipDirectory(File inputDir, File outputZipFile) {
// Create parent directory for the output file.
outputZipFile.getParentFile().mkdirs();
String inputDirPath = inputDir.getAbsolutePath();
byte[] buffer = new byte[1024];
FileOutputStream fileOs = null;
ZipOutputStream zipOs = null;
try {
List<File> allFiles = this.listChildFiles(inputDir);
// Create ZipOutputStream object to write to the zip file
fileOs = new FileOutputStream(outputZipFile);
//
zipOs = new ZipOutputStream(fileOs);
for (File file : allFiles) {
String filePath = file.getAbsolutePath();
System.out.println("Zipping " + filePath);
// entryName: là một đường dẫn tương đối.
String entryName = filePath.substring(inputDirPath.length() + 1);
ZipEntry ze = new ZipEntry(entryName);
// Put new entry into zip file.
zipOs.putNextEntry(ze);
// Read the file and write to ZipOutputStream.
FileInputStream fileIs = new FileInputStream(filePath);
int len;
while ((len = fileIs.read(buffer)) > 0) {
zipOs.write(buffer, 0, len);
}
fileIs.close();
}
} catch (IOException e) {
e.printStackTrace();
} finally {
closeQuite(zipOs);
closeQuite(fileOs);
}
}
private void closeQuite(OutputStream out) {
try {
out.close();
} catch (Exception e) {
}
}
// This method returns the list of files,
// including the children, grandchildren files of the input folder.
private List<File> listChildFiles(File dir) throws IOException {
List<File> allFiles = new ArrayList<File>();
File[] childFiles = dir.listFiles();
for (File file : childFiles) {
if (file.isFile()) {
allFiles.add(file);
} else {
List<File> files = this.listChildFiles(file);
allFiles.addAll(files);
}
}
return allFiles;
}
public static void main(String[] args) {
ZipDirectory zipDir = new ZipDirectory();
File inputDir = new File("C:/datas");
File outputZipFile = new File("C:/output/datas.zip");
zipDir.zipDirectory(inputDir, outputZipFile);
}
}
Results of running the example:
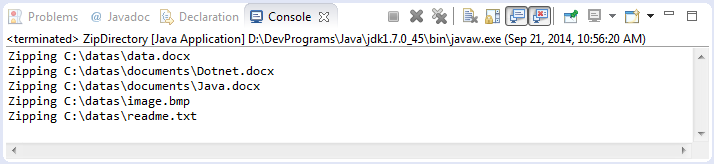
2. Read write the jar file using java.util.jar
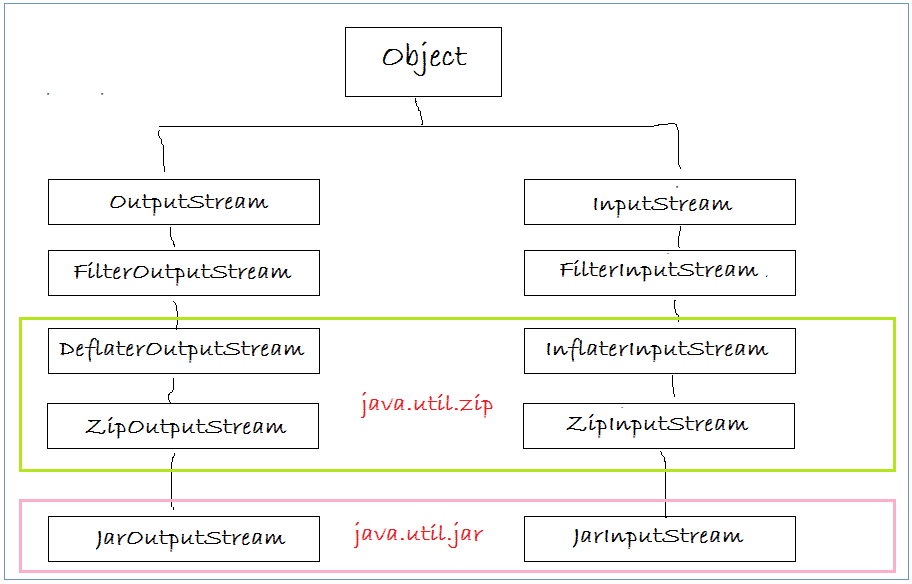
Basically read, write jar file is not any different than reading, writing Zip file.
- JarInputStream extends from ZipInputStream, supports more methods to read MANIFEST information.
- JarOutputStream extends from ZipOutputStream,supports more more method to write MANIFEST information
Example Manifest:
META-INF/MANIFEST.MF
Manifest-Version: 1.0
Bundle-ManifestVersion: 2
Bundle-Name: RAPWorkbenchTutorial
Bundle-SymbolicName: RAPWorkbenchTutorial;singleton:=true
Bundle-Version: 1.0.0.qualifier
Bundle-Activator: rapworkbenchtutorial.Activator
Require-Bundle: org.eclipse.rap.ui;bundle-version="2.3.0",
org.apache.felix.gogo.command;bundle-version="0.10.0",
org.apache.felix.gogo.runtime;bundle-version="0.10.0",
org.apache.felix.gogo.shell;bundle-version="0.10.0",
org.eclipse.equinox.console;bundle-version="1.1.0",
org.eclipse.equinox.http.jetty;bundle-version="3.0.200",
org.eclipse.equinox.ds;bundle-version="1.4.200",
org.eclipse.rap.rwt.osgi;bundle-version="2.3.0",
org.eclipse.rap.design.example;bundle-version="2.3.0"
Bundle-RequiredExecutionEnvironment: JavaSE-1.7
Bundle-ActivationPolicy: lazy
For example, open a jar file with Winrar:
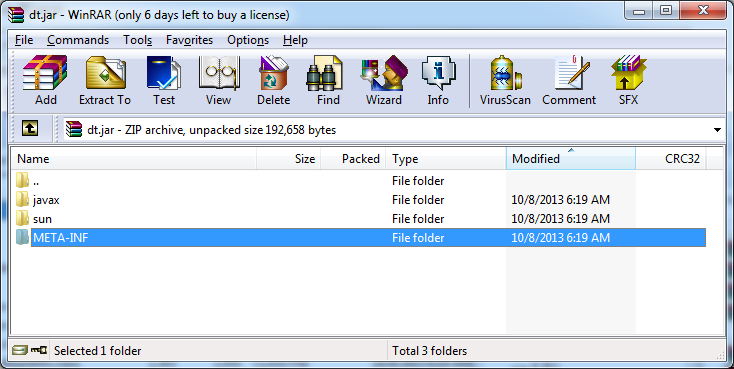
The entities in the jar file is considered to be the JarEntry.
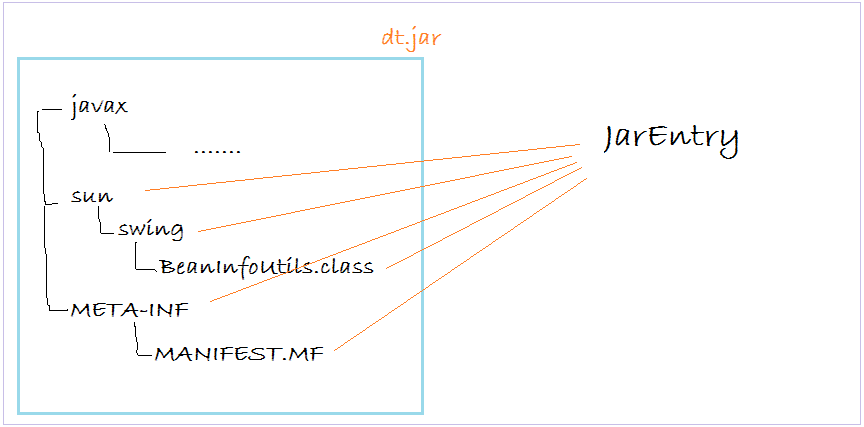
A example of reading information MANIFEST file. Here are the contents of a simple Manifest file.
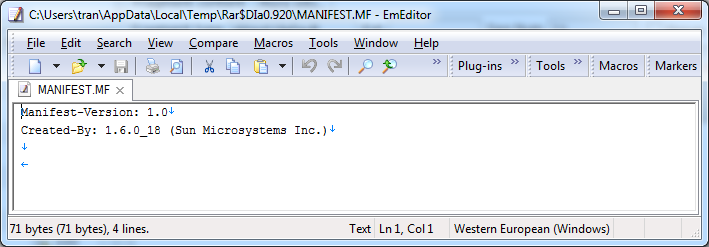
ReadJarFileDemo.java
package org.o7planning.tutorial.jar;
import java.io.FileInputStream;
import java.util.jar.Attributes;
import java.util.jar.JarEntry;
import java.util.jar.JarInputStream;
import java.util.jar.Manifest;
public class ReadJarFileDemo {
public static void main(String[] args) {
String FILE_PATH = "C:/DevPrograms/Java/jdk1.7.0_45/lib/dt.jar";
JarInputStream zipIs = null;
try {
// Create JarInputStream object to read jar file.
zipIs = new JarInputStream(new FileInputStream(FILE_PATH));
// Read manifest information:
Manifest manifest = zipIs.getManifest();
Attributes atts = manifest.getMainAttributes();
String version = atts.getValue("Manifest-Version");
String createdBy = atts.getValue("Created-By");
System.out.println("Manifest-Version:" + version);
System.out.println("Created-By:" + createdBy);
System.out.println("========================");
JarEntry entry = null;
// Read ever Entry (From top to bottom until the end)
while ((entry = zipIs.getNextJarEntry()) != null) {
if (entry.isDirectory()) {
System.out.print("Folder: ");
} else {
System.out.print("File: ");
}
System.out.println(entry.getName());
}
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
zipIs.close();
} catch (Exception e) {
}
}
}
}
Results of running the example:
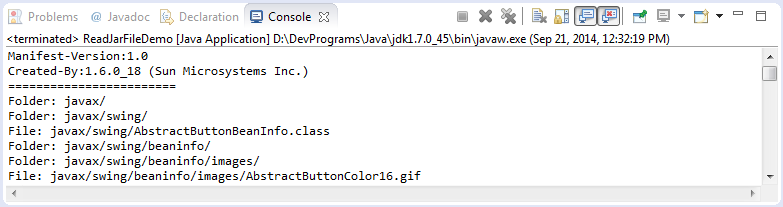
3. Handle RAR file
To handle RAR file you need a open source code library. You can use one of the following libraries which is arranged in order of quality priority.
Java Basic
- Customize java compiler processing your Annotation (Annotation Processing Tool)
- Java Programming for team using Eclipse and SVN
- Java WeakReference Tutorial with Examples
- Java PhantomReference Tutorial with Examples
- Java Compression and Decompression Tutorial with Examples
- Configuring Eclipse to use the JDK instead of JRE
- Java String.format() and printf() methods
- Syntax and new features in Java 8
- Java Regular Expressions Tutorial with Examples
- Java Multithreading Programming Tutorial with Examples
- JDBC Driver Libraries for different types of database in Java
- Java JDBC Tutorial with Examples
- Get the values of the columns automatically increment when Insert a record using JDBC
- Java Stream Tutorial with Examples
- Java Functional Interface Tutorial with Examples
- Introduction to the Raspberry Pi
- Java Predicate Tutorial with Examples
- Abstract class and Interface in Java
- Access modifiers in Java
- Java Enums Tutorial with Examples
- Java Annotations Tutorial with Examples
- Comparing and Sorting in Java
- Java String, StringBuffer and StringBuilder Tutorial with Examples
- Java Exception Handling Tutorial with Examples
- Java Generics Tutorial with Examples
- Manipulating files and directories in Java
- Java BiPredicate Tutorial with Examples
- Java Consumer Tutorial with Examples
- Java BiConsumer Tutorial with Examples
- What is needed to get started with Java?
- History of Java and the difference between Oracle JDK and OpenJDK
- Install Java on Windows
- Install Java on Ubuntu
- Install OpenJDK on Ubuntu
- Install Eclipse
- Install Eclipse on Ubuntu
- Quick Learning Java for beginners
- History of bits and bytes in computer science
- Data Types in java
- Bitwise Operations
- if else statement in java
- Switch Statement in Java
- Loops in Java
- Arrays in Java
- JDK Javadoc in CHM format
- Inheritance and polymorphism in Java
- Java Function Tutorial with Examples
- Java BiFunction Tutorial with Examples
- Example of Java encoding and decoding using Apache Base64
- Java Reflection Tutorial with Examples
- Java remote method invocation - Java RMI Tutorial with Examples
- Java Socket Programming Tutorial with Examples
- Which Platform Should You Choose for Developing Java Desktop Applications?
- Java Commons IO Tutorial with Examples
- Java Commons Email Tutorial with Examples
- Java Commons Logging Tutorial with Examples
- Understanding Java System.identityHashCode, Object.hashCode and Object.equals
- Java SoftReference Tutorial with Examples
- Java Supplier Tutorial with Examples
- Java Aspect Oriented Programming with AspectJ (AOP)
Show More
- Java Servlet/Jsp Tutorials
- Java Collections Framework Tutorials
- Java API for HTML & XML
- Java IO Tutorials
- Java Date Time Tutorials
- Spring Boot Tutorials
- Maven Tutorials
- Gradle Tutorials
- Java Web Services Tutorials
- Java SWT Tutorials
- JavaFX Tutorials
- Java Oracle ADF Tutorials
- Struts2 Framework Tutorials
- Spring Cloud Tutorials