Java Functional Interface Tutorial with Examples
1. Functional Interface
Functional Interface is a new concept introduced in Java since version 8, which is an interface with only one abstract method. Lambda expressions are often used to quickly create an object of a Functional Interface.
Here is a Functional Interface, with a single abstract method:
package org.o7planning.ex;
public interface Greeting {
String greeting(String name);
default String hello(String name) {
return "Hello " + name;
}
}
If an interface is designed for the purpose of a functional interface, you should use @FunctionalInterface annotation to annotate it. Although this is not required, it makes it easier for others to understand your design idea. It also assists compiler to report your errors during application development. For example, compiler will flag error if there is an interface annotated by @FunctionalInterface but has more than one abstract method.
package org.o7planning.ex;
@FunctionalInterface
public interface Greeting {
String greeting(String name);
default String hello(String name) {
return "Hello " + name;
}
}
Continue with the above example. Before Java 8, to create a Greeting object you had to create a class and then implement Greeting's abstract method, which is quite verbose.
UsingGreetingBeforeJ8.java
package org.o7planning.ex;
public class UsingGreetingBeforeJ8 {
public static void main(String[] args) {
// Create Greeting object:
Greeting obj = new Greeting() {
@Override
public String greeting(String name) {
return "Hi " + name;
}
};
System.out.println(obj.greeting("Tran"));
System.out.println(obj.hello("Tran"));
}
}
Basically, Functional Interface includes only 1 abstract method, so you only need to implement this method. Java 8 Lambda Expression helps you to rewrite the previous example more concisely.
UsingGreetingJ8A.java
package org.o7planning.ex;
public class UsingGreetingJ8A {
public static void main(String[] args) {
// Create Greeting object with Lambda expression:
Greeting obj = (String name) -> {
return "Hi " + name;
};
System.out.println(obj.greeting("Tran"));
System.out.println(obj.hello("Tran"));
}
}
Datatypes of the parameters in Greeting's abstract method are known, so there's no need to rewrite them. We can rewrite the previous example more succinctly:
Greeting obj = (name) -> {
return "Hi " + name;
};
If abstract method has only one parameter, you probably don't need parentheses () in Lambda expression:
Greeting obj = name -> {
return "Hi " + name;
};
If the method's contents consist of only one expression, you may not need braces {} nor need to write keyword "return".
Greeting obj = name -> "Hi " + name;
Finally, code of previous example is much shorter:
UsingGreetingJ8.java
package org.o7planning.ex;
public class UsingGreetingJ8 {
public static void main(String[] args) {
// Create Greeting object with Lambda expression:
Greeting obj = name -> "Hi " + name;
System.out.println(obj.greeting("Tran"));
System.out.println(obj.hello("Tran"));
}
}
Output:
Hi Tran
Hello Tran
2. Examples
Next, we will look at some Functional Interface(s) including valid and invalid, and explain why they are invalid:
The following IEquals interface is not a functional interface because the method "public boolean equals (Object)" is a member inherited from Object class. Thus IEquals is considered to have no abstract methods and is ineligible for a functional interface.
IEquals.java (Invalid!)
package org.o7planning.j8fi;
@FunctionalInterface
public interface IEquals {
boolean equals(Object other);
}
Valid:
IComparator.java (OK, Valid!)
package org.o7planning.j8fi;
@FunctionalInterface
public interface IComparator<T> {
int compareTwoObject(T obj, T other);
boolean equals(Object other);
}
clone() method of Object class is protected (Not public). Therefore the following IWithClone interface is considered a valid functional interface.
IWithClone.java (OK, Valid)
package org.o7planning.j8fi;
@FunctionalInterface
public interface IWithClone {
Object clone();
}
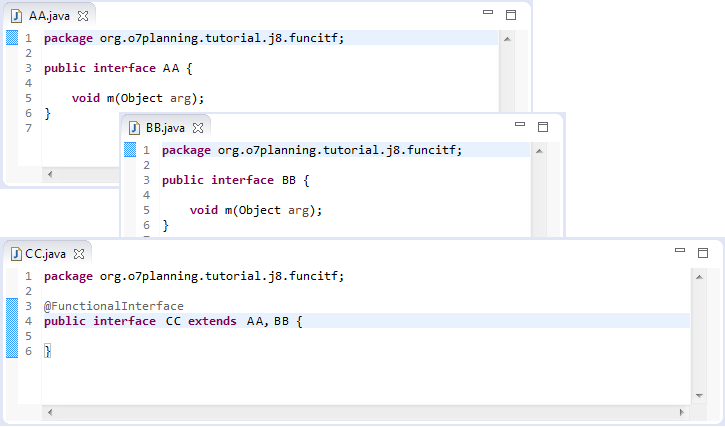
CC interface in the above illustration is a valid functional interface because abstract methods that it inherits from AA and BB are the same.
Java Basic
- Customize java compiler processing your Annotation (Annotation Processing Tool)
- Java Programming for team using Eclipse and SVN
- Java WeakReference Tutorial with Examples
- Java PhantomReference Tutorial with Examples
- Java Compression and Decompression Tutorial with Examples
- Configuring Eclipse to use the JDK instead of JRE
- Java String.format() and printf() methods
- Syntax and new features in Java 8
- Java Regular Expressions Tutorial with Examples
- Java Multithreading Programming Tutorial with Examples
- JDBC Driver Libraries for different types of database in Java
- Java JDBC Tutorial with Examples
- Get the values of the columns automatically increment when Insert a record using JDBC
- Java Stream Tutorial with Examples
- Java Functional Interface Tutorial with Examples
- Introduction to the Raspberry Pi
- Java Predicate Tutorial with Examples
- Abstract class and Interface in Java
- Access modifiers in Java
- Java Enums Tutorial with Examples
- Java Annotations Tutorial with Examples
- Comparing and Sorting in Java
- Java String, StringBuffer and StringBuilder Tutorial with Examples
- Java Exception Handling Tutorial with Examples
- Java Generics Tutorial with Examples
- Manipulating files and directories in Java
- Java BiPredicate Tutorial with Examples
- Java Consumer Tutorial with Examples
- Java BiConsumer Tutorial with Examples
- What is needed to get started with Java?
- History of Java and the difference between Oracle JDK and OpenJDK
- Install Java on Windows
- Install Java on Ubuntu
- Install OpenJDK on Ubuntu
- Install Eclipse
- Install Eclipse on Ubuntu
- Quick Learning Java for beginners
- History of bits and bytes in computer science
- Data Types in java
- Bitwise Operations
- if else statement in java
- Switch Statement in Java
- Loops in Java
- Arrays in Java
- JDK Javadoc in CHM format
- Inheritance and polymorphism in Java
- Java Function Tutorial with Examples
- Java BiFunction Tutorial with Examples
- Example of Java encoding and decoding using Apache Base64
- Java Reflection Tutorial with Examples
- Java remote method invocation - Java RMI Tutorial with Examples
- Java Socket Programming Tutorial with Examples
- Which Platform Should You Choose for Developing Java Desktop Applications?
- Java Commons IO Tutorial with Examples
- Java Commons Email Tutorial with Examples
- Java Commons Logging Tutorial with Examples
- Understanding Java System.identityHashCode, Object.hashCode and Object.equals
- Java SoftReference Tutorial with Examples
- Java Supplier Tutorial with Examples
- Java Aspect Oriented Programming with AspectJ (AOP)
Show More
- Java Servlet/Jsp Tutorials
- Java Collections Framework Tutorials
- Java API for HTML & XML
- Java IO Tutorials
- Java Date Time Tutorials
- Spring Boot Tutorials
- Maven Tutorials
- Gradle Tutorials
- Java Web Services Tutorials
- Java SWT Tutorials
- JavaFX Tutorials
- Java Oracle ADF Tutorials
- Struts2 Framework Tutorials
- Spring Cloud Tutorials