CSS visibility Tutorial with Examples
1. CSS Visibility
CSS Visibility is used to hide or display an element without changing document Layout. It can also hide a row or a row group, or a column or a column group of a table.
/* Example: */
visibility: visible|hidden|collapse|initial|inherit;
The possible values of CSS Visibility:
Value | Description |
visible | (default), "Visible" elements are default. |
hidden | Make an element invisible, but it still takes up space. |
collapse | This value is used for only the elements <thead>, <tbody>, <tfoot>, <tr>, <col>, <colgroup> (of a table) to hide this element, and release space occupied by it. If you use this value for other elements, it will work as similarly as the "hidden" value does. |
initial | Set this property to default value. |
inherit | Its value will be inherited from the parent element. |
CSS {display:none} also hides an element, but it releases the space which it occupies, so it can change document layout.
2. CSS {visibility:hidden}
CSS {visibility:hidden} makes an element invisible, but it doesn't release the space which the element occupies, so it doesn't change document layout.
visibility-hidden-example.html
<!DOCTYPE html>
<html>
<head>
<title>CSS {visibility:hidden}</title>
<meta charset="UTF-8"/>
<script>
function showHideImage(event) {
var myImage = document.getElementById("myImage");
var visibilityValue = myImage.style.visibility;
if(visibilityValue !== "hidden") {
myImage.style.visibility = "hidden";
} else {
myImage.style.visibility = "visible";
}
}
</script>
</head>
<body>
<h2>CSS {visibility:hidden}</h2>
<button onClick="showHideImage(event)">Show/Hide Image</button>
<div style="padding:5px; margin-top:10px; background:#eee;">
<img src="../images/flower.png" id= "myImage"/>
Apollo 11 was the spaceflight that landed the first humans,
Americans Neil Armstrong and Buzz Aldrin,
on the Moon on July 20, 1969, at 20:18 UTC.
Armstrong became the first to step onto the lunar
surface 6 hours later on July 21 at 02:56 UTC.
</div>
</body>
</html>
3. CSS {visibility:collapse}
CSS {visibility:collapse} is used for only one of the elements <thead>, <tbody>, <tfoot>, <tr>, <col>, <colgroup> (of a table) to hide this element, and release the space occupied by it. If you use this value for other elements, it will work as similarly as the "hidden" value does.
<thead>, <tbody>, <tfoot>, <tr>
CSS {visibility: hidden | collapse} works well with the Rows and Row groups of a table, specifically, the elements <thead>, <tbody>, <tfoot>, <tr>.
visibility-collapse-row-example.html
<!DOCTYPE html>
<html>
<head>
<title>CSS {visibility:collapse}</title>
<meta charset="UTF-8"/>
<style>
table {
border: 1px solid;
margin-top: 10px;
}
th, td {
border: 1px solid;
padding: 5px;
}
#myRow {
background: lightgreen;
}
</style>
<script>
function setVisibilityValue(newValue) {
var myRow = document.getElementById("myRow");
myRow.style.visibility = newValue;
var myNote = document.getElementById("myNote");
myNote.innerHTML = "{visibility: " + newValue+"}";
}
</script>
</head>
<body>
<h2>CSS {visibility:visible | hidden | collapse}</h2>
<button onClick="setVisibilityValue('visible')">Visible</button>
<button onClick="setVisibilityValue('hidden')">Hidden</button>
<button onClick="setVisibilityValue('collapse')">Collapse</button>
<p style="color:blue;">
Works for elements: TR, THEAD, TBODY, TFOOT.
</p>
<p id="myNote" style="color:red; font-style:italic;">
{visibility:visible}
</p>
<table>
<thead>
<tr>
<th>No</th>
<th>First Name</th>
<th>Last Name</th>
</tr>
</thead>
<tbody>
<tr id="myRow">
<td>1</td>
<td>Hillary</td>
<td>Clinton</td>
</tr>
<tr>
<td>2</td>
<td>Donald</td>
<td>Trump</td>
</tr>
</tbody>
</table>
</body>
</html>
<colgroup>, <col>
CSS {visibility: collapse} can work well with the Columns and Column groups of a table in some browsers. Other values will be ignored, or treated as {visibility: visible}.
<col>, <colgroup> | CSS {visibility:hidden} | CSS {visibility:collapse} |
Firefox | ![]() | |
Chrome |
visibility-collapse-col-example.html
<!DOCTYPE html>
<html>
<head>
<title>CSS {visibility:collapse}</title>
<meta charset="UTF-8"/>
<style>
table {
border: 1px solid;
margin-top: 10px;
}
th, td {
border: 1px solid;
padding: 5px;
}
</style>
<script>
function setVisibilityValue(newValue) {
var myColGroup = document.getElementById("myColGroup");
myColGroup.style.visibility = newValue;
var myNote = document.getElementById("myNote");
myNote.innerHTML = "{visibility: " + newValue+"}";
}
</script>
</head>
<body>
<h2>CSS {visibility:visible | hidden | collapse}</h2>
<button onClick="setVisibilityValue('visible')">Visible</button>
<button onClick="setVisibilityValue('hidden')">Hidden</button>
<button onClick="setVisibilityValue('collapse')">Collapse</button>
<h3>COL, COLGROUP Elements</h3>
<p id="myNote" style="color:red; font-style:italic;">
{visibility:visible}
</p>
<table>
<colgroup>
<col style="background-color:lightgreen">
</colgroup>
<colgroup id="myColGroup">
<col span="2" style="background-color:red">
<col style="background-color:yellow">
</colgroup>
<tr>
<th>No</th>
<th>ISBN</th>
<th>Title</th>
<th>Price</th>
<th>Description</th>
</tr>
<tr>
<td>1</td>
<td>3476896</td>
<td>My first HTML</td>
<td>$53</td>
<td>..</td>
</tr>
<tr>
<td>2</td>
<td>5869207</td>
<td>My first CSS</td>
<td>$49</td>
<td>..</td>
</tr>
</table>
<p style="color:red;">
CSS {visibility:collapse} works with COL, COLGROUP elements in Firefox (Not Chrome).<br/>
CSS {visibility:hidden} does not work with COL, COLGROUP elements in Firefox and Chrome.
</p>
</body>
</html>
Show/Hide Columns?
If you want to hide one or more columns of a table, it is best to hide all the cells of those columns, which is supported by all browsers.
hide-table-col-example.html
<!DOCTYPE html>
<html>
<head>
<title>CSS {visibility:collapse}</title>
<meta charset="UTF-8"/>
<style>
table {
border: 1px solid;
margin-top: 10px;
}
th, td {
border: 1px solid;
padding: 5px;
}
.my-cell {
background-color: lightgreen;
}
.cell-hide {
display: none;
}
</style>
<script src="hide-table-col-example.js"></script>
</head>
<body>
<h2>Show/Hide Table Columns</h2>
<button onClick="showOrHideCells(false)">Hide</button>
<button onClick="showOrHideCells(true)">Show</button>
<table>
<tr>
<th>No</th>
<th class="my-cell">ISBN</th>
<th>Title</th>
<th>Price</th>
<th>Description</th>
</tr>
<tr>
<td>1</td>
<td class="my-cell">3476896</td>
<td>My first HTML</td>
<td>$53</td>
<td>..</td>
</tr>
<tr>
<td>2</td>
<td class="my-cell">5869207</td>
<td>My first CSS</td>
<td>$49</td>
<td>..</td>
</tr>
</table>
</body>
</html>
hide-table-col-example.js
function showOrHideCells(show) {
var elements = document.getElementsByClassName("my-cell");
var copiedElements = [... elements];
for(var i=0; i< copiedElements.length; i++) {
if(show) {
copiedElements[i].classList.remove("cell-hide");
} else {
copiedElements[i].classList.add("cell-hide");
}
}
}
Below is a more complex example provided by the community, hiding / showing a table column:
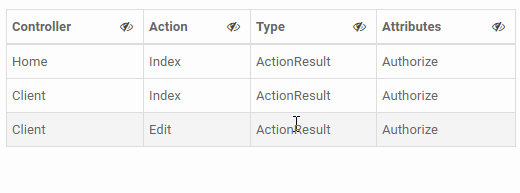
CSS Tutorials
- Units in CSS
- Basic CSS Selectors Tutorial with Examples
- CSS Attribute Selector Tutorial with Examples
- CSS combinator Selectors Tutorial with Examples
- CSS Backgrounds Tutorial with Examples
- CSS Opacity Tutorial with Examples
- CSS Padding Tutorial with Examples
- CSS Margins Tutorial with Examples
- CSS Borders Tutorial with Examples
- CSS Outline Tutorial with Examples
- CSS box-sizing Tutorial with Examples
- CSS max-width and min-width Tutorial with Examples
- The keywords min-content, max-content, fit-content, stretch in CSS
- CSS Links Tutorial with Examples
- CSS Fonts Tutorial with Examples
- Understanding Generic Font Family Names in CSS
- CSS @font-face Tutorial with Examples
- CSS Align Tutorial with Examples
- CSS Cursors Tutorial with Examples
- CSS Overflow Tutorial with Examples
- CSS Lists Tutorial with Examples
- CSS Tables Tutorial with Examples
- CSS visibility Tutorial with Examples
- CSS Display Tutorial with Examples
- CSS Grid Layout Tutorial with Examples
- CSS Float and Clear Tutorial with Examples
- CSS Position Tutorial with Examples
- CSS line-height Tutorial with Examples
- CSS text-align Tutorial with Examples
- CSS text-decoration Tutorial with Examples
Show More