CSS Lists Tutorial with Examples
1. HTML Lists
In HTML, there are two types of List: Unordered Lists and Ordered Lists.
- Unordered Lists - Use bullets to mark items on the list.
- Ordered Lists - Use numbers (Regular numbers, or Roman numbers), or letters to mark items on a list.
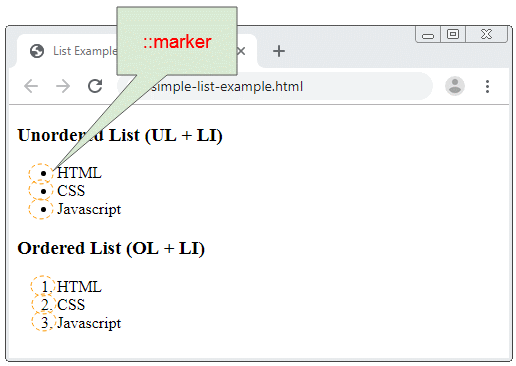
CSS List properties allow you to set the following:
- Allow choose the type of ::marker that will be seen in the Unordered List.
- Allow choose the type of ::marker that will be seen in the Ordered List.
- Use an image as an ::marker for an Unordered List.
- Sets the location of ::marker.
2. CSS list-style-type
The CSS list-style-type property is used for the <UL>(Unordered List) tag to set the style for the items of the list.
The CSS list-style-type can take one of the values:
- disc
- circle
- square
- none
ul-list-style-type-example.html
<h3>list-style-type: dist (Default)</h3>
<ul style="list-style-type: dist;">
<li>HTML</li>
<li>CSS</li>
<li>Javascript</li>
</ul>
<h3>list-style-type: circle</h3>
<ul style="list-style-type: circle;">
<li>HTML</li>
<li>CSS</li>
<li>Javascript</li>
</ul>
<h3>list-style-type: square</h3>
<ul style="list-style-type: square;">
<li>HTML</li>
<li>CSS</li>
<li>Javascript</li>
</ul>
<h3>list-style-type: none</h3>
<ul style="list-style-type: none;">
<li>HTML</li>
<li>CSS</li>
<li>Javascript</li>
</ul>
CSS list-style-type can also be applied to <OL> (Ordered List), its values can be:
- none
- disc
- circle
- square
- armenian
- decimal
- cjk-ideographic
- decimal-leading-zero
- georgian
- hebrew
- hiragana
- hiragana-iroha
- katakana
- katakana-iroha
- lower-alpha
- lower-greek
- lower-latin
- lower-roman
- upper-alpha
- upper-greek
- upper-latin
- upper-roman
list-style-type-example.html
<!DOCTYPE html>
<html>
<head>
<title>List Styles Example</title>
<meta charset="UTF-8">
<style>
table {
border-collapse: collapse;
border: 1px solid black;
width:100%;
}
td {
border: 1px solid black;
padding: 5px;
vertical-align: top;
white-space: nowrap;
}
</style>
<script src="list-style-type-example.js"></script>
</head>
<body onLoad="initRadios()">
<table>
<tr>
<td id="radio-container"></td>
<td>
<h3 id ="my-info">list-style-type: none</h3>
<ol style="list-style-type: none;" id="my-list">
<li>HTML</li>
<li>CSS</li>
<li>Javascript</li>
</ol>
</td>
<tr>
</table>
</body>
</html>
list-style-type-example.js
var types= [
"none",
"disc",
"circle",
"square",
"armenian",
"decimal",
"cjk-ideographic",
"decimal-leading-zero",
"georgian",
"hebrew",
"hiragana",
"hiragana-iroha",
"katakana",
"katakana-iroha ",
"lower-alpha",
"lower-greek",
"lower-latin",
"lower-roman",
"upper-alpha",
"upper-greek",
"upper-latin",
"upper-roman"];
function initRadios() {
var radioContainer = document.getElementById("radio-container");
for(var i = 0; i< types.length; i++) {
var radioElement = document.createElement("input");
radioElement.type = "radio";
radioElement.name = "type";
radioElement.value = types[i];
var spanElement = document.createElement("span");
spanElement.innerHTML = types[i];
spanElement.style.marginRight = "5px";
var brElement = document.createElement("br");
radioElement.addEventListener("click", function(event) {
var infoElement = document.getElementById("my-info");
infoElement.innerHTML = "{ list-style-type: " + event.target.value + " }";
var listElement = document.getElementById("my-list");
listElement.style.listStyleType = event.target.value;
});
radioContainer.appendChild(radioElement);
radioContainer.appendChild(spanElement);
radioContainer.appendChild(brElement);
}
}
3. CSS list-style-image
The CSS list-style-image property is used for the <UL> tag to display an alternative image for ::markers.
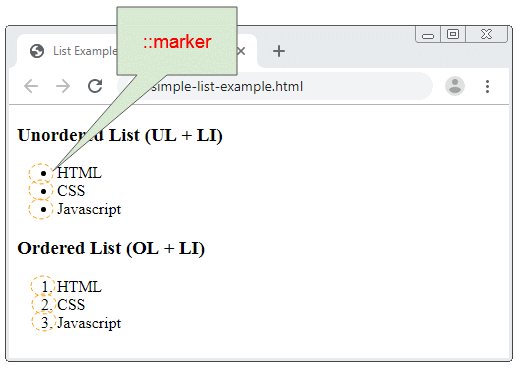
Notes: the list-style-image takes precedence over the list-style-type. If the image provided by list-style-image does not exist or cannot display list-style-type to be used.
<ul style="list-style-image: URL('../right-arrow-16.png')">
<li>HTML</li>
<li>CSS</li>
<li>Javascript</li>
</ul>
4. CSS list-style-position
The CSS list-style-position property is used for the <UL> and the <OL> tag to set the position of the ::markers.
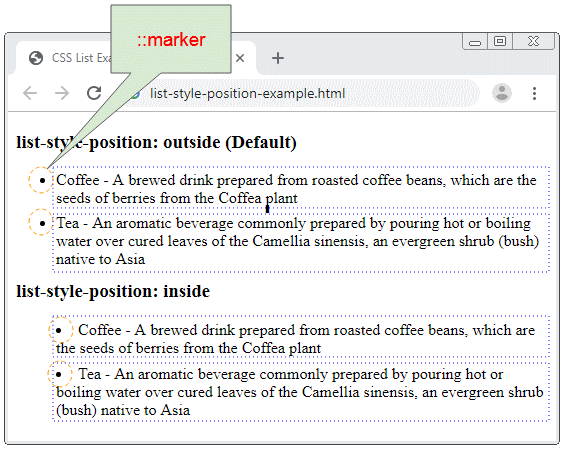
Possible values of the CSS list-style-position are:
- outside
- inside
- initial
- inherit
list-style-position | Description |
outside | ::marker is located outside of the items of the list. |
inside | ::marker is located inside of the items of the list. |
list-style-position-example.html
<h3>list-style-position: outside (Default)</h3>
<ul style="list-style-position: outside">
<li>
Coffee - A brewed drink prepared from roasted coffee beans,
which are the seeds of berries from the Coffea plant
</li>
<li>
Tea - An aromatic beverage commonly prepared by pouring hot
or boiling water over cured leaves of the Camellia sinensis,
an evergreen shrub (bush) native to Asia
</li>
</ul>
<h3>list-style-position: inside</h3>
<ul style="list-style-position: inside">
<li>
Coffee - A brewed drink prepared from roasted coffee beans,
which are the seeds of berries from the Coffea plant
</li>
<li>
Tea - An aromatic beverage commonly prepared by pouring hot
or boiling water over cured leaves of the Camellia sinensis,
an evergreen shrub (bush) native to Asia
</li>
</ul>
5. CSS margin, padding
The image below shows the HTML List structure, and you can use CSS to change its margin/padding.
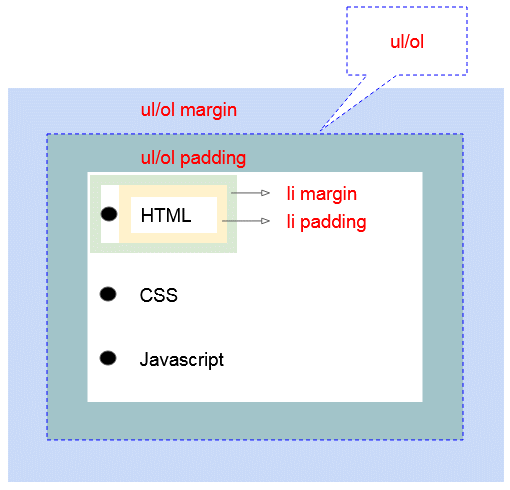
Here is an example of the customized margin/padding of the HTML List:
margin-padding-example.css
ul {
background: #3399ff;
padding: 20px;
}
ul li {
background: #cce5ff;
margin: 5px;
}
ol {
background: #ff9999;
padding: 20px;
}
ol li {
background: #ffe5e5;
padding: 5px;
margin-left: 35px;
}
margin-padding-example.html
<!DOCTYPE html>
<html>
<head>
<title>CSS List</title>
<meta charset="UTF-8">
<link rel="stylesheet" type="text/css" href="margin-padding-example.css" />
</head>
<body>
<h3>ul {padding: 20px;} ul li {margin: 5px}</h3>
<ul>
<li>HTML</li>
<li>CSS</li>
<li>Javascript</li>
</ul>
<h3>ol {padding: 20px;} ol li {margin-left: 35px; padding: 5px;}</h3>
<ol>
<li>HTML</li>
<li>CSS</li>
<li>Javascript</li>
</ol>
</body>
</html>
CSS Tutorials
- Units in CSS
- Basic CSS Selectors Tutorial with Examples
- CSS Attribute Selector Tutorial with Examples
- CSS combinator Selectors Tutorial with Examples
- CSS Backgrounds Tutorial with Examples
- CSS Opacity Tutorial with Examples
- CSS Padding Tutorial with Examples
- CSS Margins Tutorial with Examples
- CSS Borders Tutorial with Examples
- CSS Outline Tutorial with Examples
- CSS box-sizing Tutorial with Examples
- CSS max-width and min-width Tutorial with Examples
- The keywords min-content, max-content, fit-content, stretch in CSS
- CSS Links Tutorial with Examples
- CSS Fonts Tutorial with Examples
- Understanding Generic Font Family Names in CSS
- CSS @font-face Tutorial with Examples
- CSS Align Tutorial with Examples
- CSS Cursors Tutorial with Examples
- CSS Overflow Tutorial with Examples
- CSS Lists Tutorial with Examples
- CSS Tables Tutorial with Examples
- CSS visibility Tutorial with Examples
- CSS Display Tutorial with Examples
- CSS Grid Layout Tutorial with Examples
- CSS Float and Clear Tutorial with Examples
- CSS Position Tutorial with Examples
- CSS line-height Tutorial with Examples
- CSS text-align Tutorial with Examples
- CSS text-decoration Tutorial with Examples
Show More